diff --git a/docs/DeveloperGuide.md b/docs/DeveloperGuide.md
index 3b429d436d..d383cbe15e 100644
--- a/docs/DeveloperGuide.md
+++ b/docs/DeveloperGuide.md
@@ -4,13 +4,36 @@
{list here sources of all reused/adapted ideas, code, documentation, and third-party libraries -- include links to the original source as well}
-## Design & implementation
+## Design
### Architecture
+Given below is a higher-level overview of the main components for the app to work.
+
+```Main``` has methods which are responsible for:
+1. App launch: Initialises the various classes needed and starting up the game
+2. App running: Calls the various methods in other Classes to run the game
+3. App shutdown: Saves the game state
+
+listed below are a collection of classes used by multiple components which will be generalised as ```Commons```.
+1. ```TextBox``` which is used to set all the messages and narrations for the user.
+2. ```FileReader``` to read our design.txt files in order to print certain screens.
+3. ```PlayerStatus``` which stores the status and inventory of the player.
+
+```Ui``` responsible for displaying the game's UI, interactions and narrations to the user.
+```Storage``` responsible for saving the current state of the game when quitting the app.
+```Parser``` is a collection of classes that converts the user's commands and starts the command execution process.
+
+Below is how some of the architecture components would interact with each other when the user inputs the command to move.
+
+The section below gives more details of each component.
### UI Component
-# UserCommand feature
+### Parser
+
+## Implementation
+
+### User Command Component
User can type command to do things on the Map.
@@ -20,8 +43,6 @@ Command back to the main based on the command type. The Final step is to call th
is the type of `fightCommand`, we will call the execute function with one parameter `Scanner`. For all other
conditions, we will call the execute function with no parameter.
-
-
### Map Component
The API of this component is defined in BaseMap.java.
@@ -41,21 +62,15 @@ At the moment the `MapGenerator` class allows the positions of enemies to be pla
The reason why the player's map(FirstMap), the shop's interface and the battle interface all extend off of the `BaseMap`
class is because during the game loop, these maps are being cycled through as the main screen the user will view.
-
-
-
-{Describe the design and implementation of the product. Use UML diagrams and short code snippets where applicable.}
-
### Interacting with Environment Component
The API of this component is defined in InteractingCommand.java
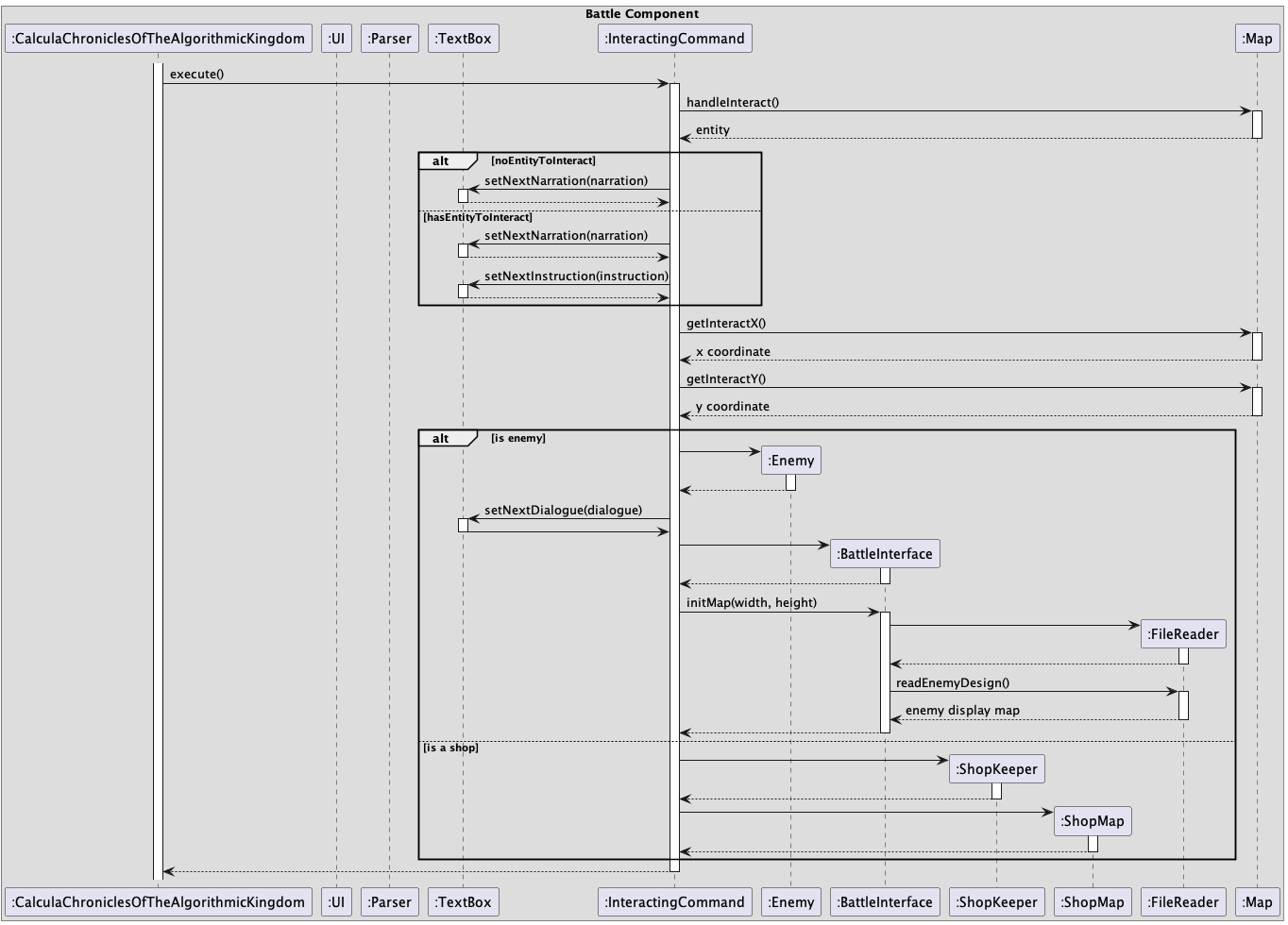
-This component happens when the user chooses to key the interact command.
+This component happens when the user chooses to key the interact command ```e```.
Here is how it works:
-1. When the command is passed by the user, the ```CalculaChroniclesOfTheAlgorithmicKingdom``` object calls the ```Parser``` object
- to parse the command to call the respective commands. Here, the ```InteractingCommand``` object is created.
+1. When the user chooses to fight, the command is parsed.
2. The ```CalculaChroniclesOfTheAlgorithmicKingdom``` component then calls the execute() method in ```InteractingCommand```.
3. It executes the method and creates other objects like ```Enemy``` and ```ShopKeaper``` components which are responsible for the
entity classes in the game and also ```BattleInterface``` and ```ShopMap``` which are responsible for displaying these entities among other things.
@@ -69,10 +84,10 @@ The API of this component is defined in FightCommand.java.
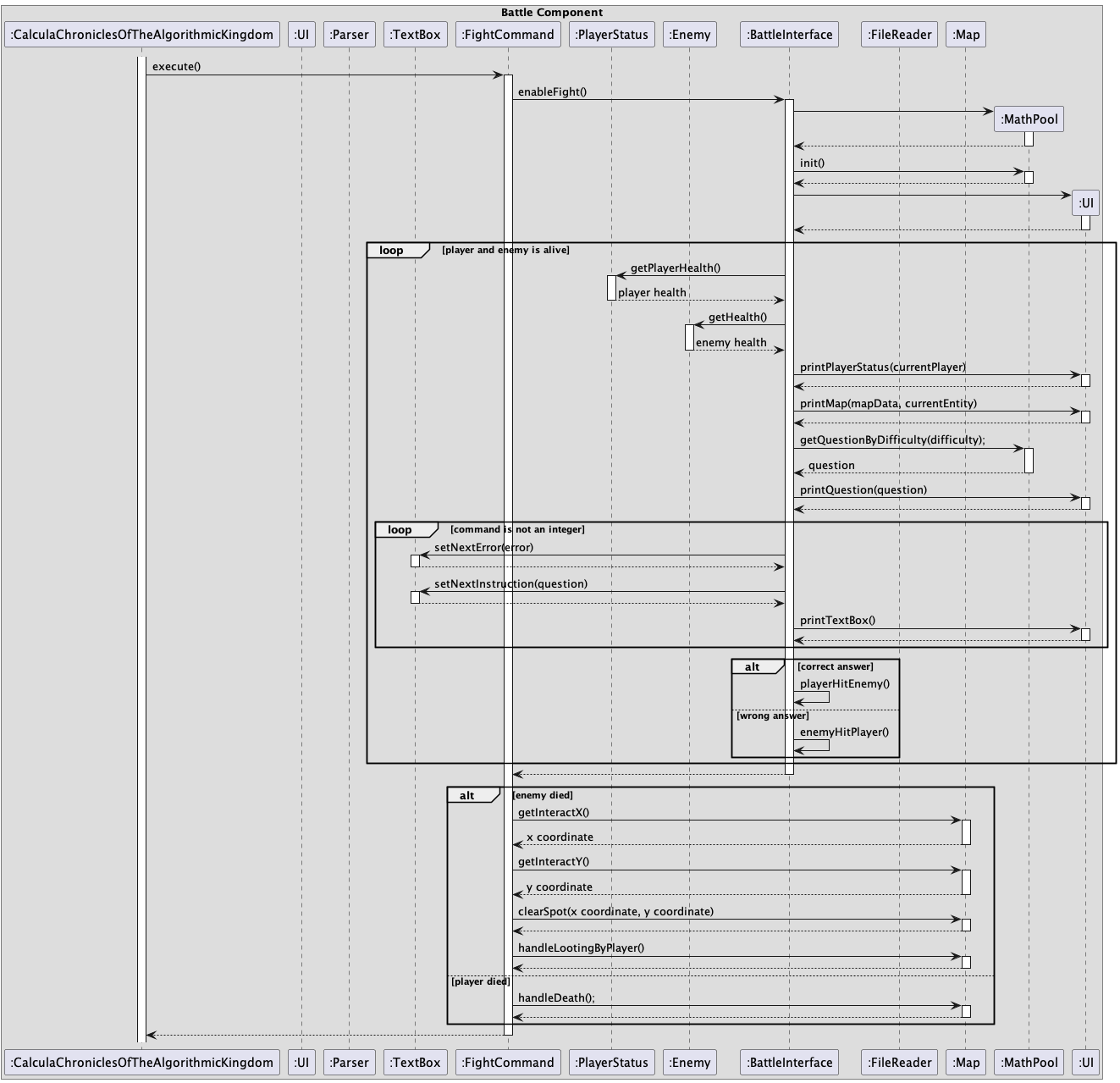
-This component happens when the user chooses to fight an enemy.
+This component occurs when the user chooses to fight an enemy after interacting with it using the command
+```f``` or ```fight```.
Here is how it works:
-1. When the user chooses to fight, the ```CalculaChroniclesOfTheAlgorithmicKingdom``` object calls the ```Parser``` object
- to parse the command to call the respective commands. Here, the ```FightCommand``` object is created.
+1. When the user chooses to fight, the command is parsed.
2. The ```CalculaChroniclesOfTheAlgorithmicKingdom``` object then calls the execute() method in ```FightCommand``` and enables the fighting.
3. The ```MathPool``` object is created, which is responsible for the math questions to answer and another ```Ui``` object is created to interact with the user.
4. In the enableFight() method, it has a loop which asks the user math questions to answer until the player or enemy dies. The player takes damage for
@@ -82,21 +97,62 @@ until the user gives an answer which is a valid integer.
6. Once either the player or enemy dies, it then exits and runs the relevant checks to eventually print the output to be shown to
the user after battle, handled by ```CalculaChroniclesOfTheAlgorithmicKingdom```.
+### Item Usage Component
+
+The API of the following component is defined in OpenInventoryCommand.java.
+
+This component occurs when the user decides to open up the inventory. The user opens up the inventory using the command ```i```
+or ```inventory```.
+Here is how it works:
+1. When the user chooses to open the inventory, the command is parsed.
+2. The ```CalculaChroniclesOfTheAlgorithmicKingdom``` object then calls the execute() method in ```OpenInventoryCommand```to get
+the inventory from the stored maps in ```BaseMap```.
+3. The inventory would then be printed on the Ui for display.
+
+The API of the following component is defined in UseCommand.java.
+
+This component occurs when the user decides to use an item after navigating to the inventory page containing consumable items.
+Here is how it works:
+1. When the user chooses to use an item after navigating to the consumable items page, the command is parsed.
+2. The ```CalculaChroniclesOfTheAlgorithmicKingdom``` object then calls the execute() method
+3. The method goes through the necessary checks to check if the item intended to use has been indicated as stated in the UserGuide.
+4. If any of the checks fail, an error message would be displayed to flag out what went wrong.
+5. If all the checks passes, the inventory is obtained from the ```PlayerStatus``` object. The inventory is then searched to check if it
+contains the item.
+6. The method useItem(item) in the ```PlayerInventory``` object is called if the item is found. Subsequently,
+an error message is printed outlining the error.
+
## Product scope
### Target user profile
-{Describe the target user profile}
+Our target users are young students who are hoping the revise their mathematical skills.
### Value proposition
-{Describe the value proposition: what problem does it solve?}
+It allows the target user to supplement their existing revision with a more fun and interacting way to revise their mathematics knowledge.
## User Stories
-| Version | As a ... | I want to ... | So that I can ... |
-|---------|----------|---------------------------|-------------------------------------------------------------|
-| v1.0 | new user | see usage instructions | refer to them when I forget how to use the application |
-| v2.0 | user | find a to-do item by name | locate a to-do without having to go through the entire list |
+| Priority | As a ... | I want to ... | So that I can ... |
+|----------|----------------|-------------------------------------------------------------|---------------------------------------------------------|
+| *** | new player | to get access to a help menu | refer to them when I don't know the commands to proceed |
+| *** | player | to see a map of the play area | see the map and location in real-time |
+| *** | player | to move around at will | explore the world as I want to |
+| *** | player | to have an ending to the game | win the game |
+| *** | player | to be able to track stats/items | gauge how my characters progress |
+| *** | player | save my game | come back and finish it when I have time |
+| *** | player | To have a death and restart mechanic | add challenge to the game |
+| ** | player | To be able to fight entities | battle in and interactive way |
+| ** | player | To collect items | enhance my character |
+| ** | player | To interact with things in the environment | be more immersed into the game |
+| ** | player | See an actual image of the characters | know what I am fighting against |
+| ** | student player | To have variations in the questions asked | revise more stuff rather than the same questions |
+| ** | player | to have clear distinctions between entities I interact with | have a clearer picture of what I'm doing |
+| ** | student player | refresh my knowledge of math | revise as I play at the same time |
+| * | player | To know the background of this game | follow the storyline |
+| * | player | to see funny and engaging dialogue | enjoy the story |
+| * | player | To have access to hints to the questions | make calculations easier |
+
## Non-Functional Requirements
diff --git a/graph/BattleInterface.puml b/graph/BattleInterface.puml
index cbd4482463..f736d6a18f 100644
--- a/graph/BattleInterface.puml
+++ b/graph/BattleInterface.puml
@@ -1,7 +1,7 @@
@startuml
box Battle Component
-participant "CalculaChroniclesOfTheAlgorithmicKingdom" as main
+participant ":CalculaChroniclesOfTheAlgorithmicKingdom" as main
participant ":UI" as ui
participant ":Parser" as parser
participant ":TextBox" as text
@@ -13,17 +13,17 @@ participant ":FileReader" as fileReader
participant ":Map" as map
participant ":MathPool" as mathpool
-activate main
-main -> parser : parseCommand(userCommandText)
-activate parser
-create f
-parser -> f
-activate f
-f --> parser
-deactivate f
-parser --> main : command class
-deactivate parser
-main -> main : executeCommand()
+'activate main
+'main -> parser : parseCommand(userCommandText)
+'activate parser
+'create f
+'parser -> f
+'activate f
+'f --> parser
+'deactivate f
+'parser --> main : command class
+'deactivate parser
+'main -> main : executeCommand()
activate main
main -> f : execute()
activate f
@@ -116,21 +116,7 @@ end
f --> main
deactivate f
deactivate main
-main -> main : printMessageUnderMap(userCommand, ui, playerStatus, textBox)
-activate main
-alt not calling help menu or quitting game
- alt show battle interface
- main -> ui : printEnemy(currentMap)
- activate ui
- ui --> main
- deactivate ui
- else
- main -> ui : printMap(currentMap)
- activate ui
- ui --> main
- deactivate ui
- end
-end
+
end box
diff --git a/graph/Interaction.puml b/graph/Interaction.puml
index 6edf083941..69898d2d18 100644
--- a/graph/Interaction.puml
+++ b/graph/Interaction.puml
@@ -1,7 +1,7 @@
@startuml
box Battle Component
-participant "CalculaChroniclesOfTheAlgorithmicKingdom" as main
+participant ":CalculaChroniclesOfTheAlgorithmicKingdom" as main
participant ":UI" as ui
participant ":Parser" as parser
participant ":TextBox" as text
@@ -14,17 +14,17 @@ participant ":FileReader" as fileReader
participant ":Map" as map
activate main
-main -> parser : parseCommand(userCommandText)
-activate parser
-create iCommand
-parser -> iCommand
-activate iCommand
-iCommand --> parser
-deactivate iCommand
-parser --> main : command class
-deactivate parser
-main -> main : executeCommand()
-activate main
+'main -> parser : parseCommand(userCommandText)
+'activate parser
+'create iCommand
+'parser -> iCommand
+'activate iCommand
+'iCommand --> parser
+'deactivate iCommand
+'parser --> main : command class
+'deactivate parser
+'main -> main : executeCommand()
+'activate main
main -> iCommand : execute()
activate iCommand
iCommand -> map : handleInteract()
@@ -96,22 +96,22 @@ else is a shop
end
iCommand --> main
deactivate iCommand
-deactivate main
-main -> main : printMessageUnderMap(userCommand, ui, playerStatus, textBox)
-activate main
-alt not calling help menu or quitting game
- alt show battle interface
- main -> ui : printEnemy(currentMap)
- activate ui
- ui --> main
- deactivate ui
- else
- main -> ui : printMap(currentMap)
- activate ui
- ui --> main
- deactivate ui
- end
-end
+'deactivate main
+'main -> main : printMessageUnderMap(userCommand, ui, playerStatus, textBox)
+'activate main
+'alt not calling help menu or quitting game
+' alt show battle interface
+' main -> ui : printEnemy(currentMap)
+' activate ui
+' ui --> main
+' deactivate ui
+' else
+' main -> ui : printMap(currentMap)
+' activate ui
+' ui --> main
+' deactivate ui
+' end
+'end
end box
diff --git a/graph/ItemUsage.puml b/graph/ItemUsage.puml
index 7deb2045cc..3672e3dc4d 100644
--- a/graph/ItemUsage.puml
+++ b/graph/ItemUsage.puml
@@ -35,27 +35,11 @@ text --> use
deactivate text
use --> main
end
-opt item name was provided
-use -> use : findItem(itemString)
-activate use
-use -> status : getPlayerInventory()
-activate status
-
-status --> use : inventory
-deactivate status
-alt item is in list
-use -> inventory : useItem(item)
-activate inventory
-inventory --> use
-deactivate inventory
-else
+opt item index was not a valid integer
use -> text : setNextError(error)
activate text
text --> use
deactivate text
-deactivate use
-end
-use --> main
end
use -> status : getPlayerInventory()
activate status
diff --git a/picture/BattleInterface.png b/picture/BattleInterface.png
index e93052ed5e..0582edb896 100644
Binary files a/picture/BattleInterface.png and b/picture/BattleInterface.png differ
diff --git a/picture/Interaction.png b/picture/Interaction.png
index 9b314e30e3..6e2c2f0123 100644
Binary files a/picture/Interaction.png and b/picture/Interaction.png differ
diff --git a/picture/ItemUsage.png b/picture/ItemUsage.png
index ac0fe06739..6c53663630 100644
Binary files a/picture/ItemUsage.png and b/picture/ItemUsage.png differ
diff --git a/src/main/java/command/CommandType.java b/src/main/java/command/CommandType.java
index 5980c2feb9..e2ba9cf604 100644
--- a/src/main/java/command/CommandType.java
+++ b/src/main/java/command/CommandType.java
@@ -10,14 +10,11 @@ public enum CommandType {
INTERACT("(?i)\\h*(e)\\h*"),
QUIT("(?i)\\h*(q|quit)\\h*"),
HELP("(?i)\\h*(h|help)\\h*"),
- EXIT("(?i)\\h*(exit)\\h*"), // New command: EXIT
+ EXIT("(?i)\\h*(exit)\\h*"),
ERROR(""),
INVENTORY("(?i)\\h*(i|inventory)\\h*"),
- INV_NEXT("(?i)\\h*(n|next)\\h*"),
- INV_PREV("(?i)\\h*(p|prev|previous)\\h*"),
USE_ITEM("(?i)\\h*(use)(\\h+\\d+|\\h+\\w+)?\\h*"),
- SELL_ITEM("(?i)\\h*(sell)(\\h+\\d+|\\h+\\w+)?\\h*"),
- CLOSE_INV("(?i)\\h*(c|close)\\h*"); // to delete aft sihan implements
+ CLOSE_INV("(?i)\\h*(close)\\h*");
final String regExpression;
CommandType(String regExpression) {
diff --git a/src/main/java/command/inventory/NextPageCommand.java b/src/main/java/command/inventory/NextPageCommand.java
deleted file mode 100644
index e42bfb577d..0000000000
--- a/src/main/java/command/inventory/NextPageCommand.java
+++ /dev/null
@@ -1,10 +0,0 @@
-package command.inventory;
-
-import command.Command;
-
-public class NextPageCommand extends Command {
- @Override
- public void execute() {
- playerStatus.getPlayerInventory().listNextInventoryPage();
- }
-}
diff --git a/src/main/java/command/inventory/OpenInventoryCommand.java b/src/main/java/command/inventory/OpenInventoryCommand.java
index 134b30a1e0..546a04d5ab 100644
--- a/src/main/java/command/inventory/OpenInventoryCommand.java
+++ b/src/main/java/command/inventory/OpenInventoryCommand.java
@@ -14,6 +14,7 @@ public OpenInventoryCommand() {
@Override
public void execute() {
BaseMap.currentMap = mapIndex.get(INVENTORY_IDENTITY);
- textBox.setNextNarration("Here's your inventory. Navigate the pages using [next] or [prev]");
+ textBox.setNextNarration("Here's your inventory! You can use an item by keying in [use] followed by it's " +
+ "index with a space between them.");
}
}
diff --git a/src/main/java/command/inventory/PrevPageCommand.java b/src/main/java/command/inventory/PrevPageCommand.java
deleted file mode 100644
index b4e15d1161..0000000000
--- a/src/main/java/command/inventory/PrevPageCommand.java
+++ /dev/null
@@ -1,10 +0,0 @@
-package command.inventory;
-
-import command.Command;
-
-public class PrevPageCommand extends Command {
- @Override
- public void execute() {
- playerStatus.getPlayerInventory().listPreviousInventoryPage();
- }
-}
diff --git a/src/main/java/command/inventory/SellCommand.java b/src/main/java/command/inventory/SellCommand.java
index ab1b34d90b..ffc2ef8ecf 100644
--- a/src/main/java/command/inventory/SellCommand.java
+++ b/src/main/java/command/inventory/SellCommand.java
@@ -31,8 +31,7 @@ public void findItem(String item, ArrayList- itemList) {
@Override
public void execute() {
PlayerInventory inventory = playerStatus.getPlayerInventory();
- int listIndex = inventory.getCurrentItemPageNumber();
- ArrayList
- list = playerStatus.getPlayerInventory().getAllItemsList().get(listIndex);
+ ArrayList
- list = playerStatus.getPlayerInventory().getGeneralItems();
if (list.isEmpty()) {
textBox.setNextError("The item does not exist");
return;
diff --git a/src/main/java/command/inventory/UseCommand.java b/src/main/java/command/inventory/UseCommand.java
index 0dffa0fd3e..d433a772fd 100644
--- a/src/main/java/command/inventory/UseCommand.java
+++ b/src/main/java/command/inventory/UseCommand.java
@@ -5,8 +5,6 @@
import inventoryitems.Item;
import map.PlayerInventory;
-import java.util.stream.Collectors;
-
import static java.lang.Integer.parseInt;
public class UseCommand extends Command {
@@ -16,27 +14,9 @@ public UseCommand(String userCommand) {
this.userCommand = userCommand;
}
- public void findItem(String item) {
- PlayerInventory inventory = playerStatus.getPlayerInventory();
- Item consumable;
- try {
- consumable = inventory.getConsumableItems().stream().filter(x -> x.getName().equalsIgnoreCase(item))
- .collect(Collectors.toList()).get(0);
- inventory.useItem((Consumable) consumable);
- } catch (Exception e) {
- textBox.setNextError("Please enter a valid item number or item name");
- }
- }
-
@Override
public void execute() {
- if (playerStatus.getPlayerInventory().getInventoryNames().indexOf("Consumable") !=
- playerStatus.getPlayerInventory().getCurrentItemPageNumber()) {
- textBox.setNextInstruction("Enter [next] or [prev] to navigate the inventory page.");
- textBox.setNextError("You only can use an item in the consumables page");
- return;
- }
- if (playerStatus.getPlayerInventory().getConsumableItems().isEmpty()) {
+ if (playerStatus.getPlayerInventory().getGeneralItems().isEmpty()) {
textBox.setNextError("The item does not exist");
return;
}
@@ -46,16 +26,16 @@ public void execute() {
itemString = userCommand.split(" ", 2)[1];
itemIndex = parseInt(itemString);
} catch (ArrayIndexOutOfBoundsException e) {
- textBox.setNextError("Please enter an item number or item name");
+ textBox.setNextError("Please enter an item index");
return;
} catch (NumberFormatException e) {
- findItem(itemString);
+ textBox.setNextError("Please enter a valid integer for the item index");
return;
}
Item item;
PlayerInventory inventory = playerStatus.getPlayerInventory();
try {
- item = inventory.getConsumableItems().get(itemIndex - 1);
+ item = inventory.getGeneralItems().get(itemIndex - 1);
} catch (Exception e) {
textBox.setNextError("The item does not exist");
return;
diff --git a/src/main/java/filereader/MapStorage.java b/src/main/java/filereader/MapStorage.java
index 134c3a7561..98a6e0a973 100644
--- a/src/main/java/filereader/MapStorage.java
+++ b/src/main/java/filereader/MapStorage.java
@@ -12,11 +12,9 @@
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.Scanner;
-import java.util.concurrent.TimeUnit;
import static filereader.filepath.MapFilePath.BASE_MAP_PATH;
import static filereader.filepath.MapFilePath.MAP_PROPERTY_PATH;
-import static map.BaseMap.storedMaps;
public class MapStorage {
@@ -95,6 +93,7 @@ private BaseMap getBaseMap(File mapPicture, File mapProperty) throws FileNotFoun
case 3:
map.setPlayerY(currentData);
break;
+ default:
}
round++;
}
diff --git a/src/main/java/filereader/PlayerStatusStorage.java b/src/main/java/filereader/PlayerStatusStorage.java
index 3ac536a071..3e504dfa95 100644
--- a/src/main/java/filereader/PlayerStatusStorage.java
+++ b/src/main/java/filereader/PlayerStatusStorage.java
@@ -57,6 +57,7 @@ public PlayerStatus readPlayerStatus() throws FileNotFoundException {
case 3:
startDamage = playerStatusData;
break;
+ default:
}
round++;
}
diff --git a/src/main/java/main/CalculaChroniclesOfTheAlgorithmicKingdom.java b/src/main/java/main/CalculaChroniclesOfTheAlgorithmicKingdom.java
index f91d191997..360f85ca72 100644
--- a/src/main/java/main/CalculaChroniclesOfTheAlgorithmicKingdom.java
+++ b/src/main/java/main/CalculaChroniclesOfTheAlgorithmicKingdom.java
@@ -6,7 +6,7 @@
import hint.HintHandler;
import command.Command;
import map.BaseMap;
-import map.FirstMap;
+import map.ShopMap;
import map.PlayerInventory;
import map.battleinterface.BattleInterface;
import parser.Parser;
@@ -18,7 +18,6 @@
import java.io.IOException;
import java.util.Scanner;
-import map.MapGenerator;
import static map.BaseMap.mapIndex;
import static map.BaseMap.storedMaps;
import static map.BaseMap.currentMap;
@@ -29,7 +28,7 @@
public class CalculaChroniclesOfTheAlgorithmicKingdom {
public static final int START_HEALTH = 100;
- public static final int START_MONEY = 0;
+ public static final int START_MONEY = 200;
public static final int START_EXP = 0;
public static final int START_DAMAGE = 5;
public static final PlayerInventory PLAYER_INVENTORY = new PlayerInventory();
@@ -112,12 +111,12 @@ private static void printMessageUnderMap(Command userCommand, Ui ui, PlayerStatu
if (!userCommand.getCommandDescription().equals("HelpMe!!") &&
!userCommand.getCommandDescription().equals("TIRED")) {
ui.printPlayerStatus(playerStatus);
- if (storedMaps.get(currentMap) instanceof BattleInterface) {
+ if (storedMaps.get(currentMap) instanceof BattleInterface ||
+ storedMaps.get(currentMap) instanceof ShopMap) {
ui.printEnemy(storedMaps.get(currentMap));
} else if (storedMaps.get(currentMap) instanceof PlayerInventory){
- int listIndex = playerStatus.getPlayerInventory().getCurrentItemPageNumber();
- ui.printInventory(playerStatus.getPlayerInventory().getAllItemsList().get(listIndex),
- playerStatus.getPlayerInventory().getInventoryNames().get(listIndex),
+ ui.printInventory(playerStatus.getPlayerInventory().getGeneralItems(),
+ playerStatus.getPlayerInventory().getInventoryNames().get(0),
storedMaps.get(currentMap).getWidth(), storedMaps.get(currentMap).getHeight());
} else {
ui.printMap(storedMaps.get(currentMap));
diff --git a/src/main/java/map/BaseMap.java b/src/main/java/map/BaseMap.java
index a6c78e9f65..b02b17588d 100644
--- a/src/main/java/map/BaseMap.java
+++ b/src/main/java/map/BaseMap.java
@@ -14,6 +14,14 @@ public abstract class BaseMap {
public static HashMap mapIndex = new HashMap<>();
protected int width;
protected int height;
+ protected ArrayList> mapData;
+ protected int playerX;
+ protected int playerY;
+ protected String mapName;
+ protected TextBox textBox;
+
+ public BaseMap() {
+ }
public void setWidth(int width) {
this.width = width;
@@ -31,15 +39,6 @@ public void setPlayerY(int playerY) {
this.playerY = playerY;
}
- protected ArrayList> mapData;
- protected int playerX;
- protected int playerY;
- protected String mapName;
- protected TextBox textBox;
-
- public BaseMap() {
- }
-
public abstract void enableFight();
public void enableFight(Scanner in) {
diff --git a/src/main/java/map/BattleInterface/BattleInterface.java b/src/main/java/map/BattleInterface/BattleInterface.java
index b0560ecef0..719219a56b 100644
--- a/src/main/java/map/BattleInterface/BattleInterface.java
+++ b/src/main/java/map/BattleInterface/BattleInterface.java
@@ -76,7 +76,7 @@ public void initMap(int givenWidth, int givenHeight) {
try {
mapData = fileReader.readEnemyDesign();
} catch (Exception e) {
- // display exception, see how sihan wants to do.
+ currentTextBox.setNextError("Unable to read file from local");
}
}
diff --git a/src/main/java/map/PlayerInventory.java b/src/main/java/map/PlayerInventory.java
index c6eb07e167..2ad0746e2f 100644
--- a/src/main/java/map/PlayerInventory.java
+++ b/src/main/java/map/PlayerInventory.java
@@ -1,110 +1,51 @@
package map;
import inventoryitems.Consumable;
-import inventoryitems.Gear;
import inventoryitems.Item;
import textbox.PlayerStatus;
import textbox.TextBox;
import ui.Ui;
import java.util.ArrayList;
+import java.util.List;
+import java.util.stream.Collectors;
public class PlayerInventory extends BaseMap {
- protected static final int FIRST_PAGE_INDEX = 0;
- protected static final int LAST_PAGE_INDEX = 2;
- protected ArrayList> allItemsList;
protected ArrayList inventoryNames;
protected ArrayList
- generalItems;
- protected ArrayList
- consumableItems;
- protected ArrayList
- equippableItems;
- protected int currentItemPageNumber;
protected Ui ui;
protected TextBox currentTextBox;
protected PlayerStatus playerStatus;
public PlayerInventory() {
- this.currentItemPageNumber = 0;
this.generalItems = new ArrayList<>();
- this.consumableItems = new ArrayList<>();
- this.equippableItems = new ArrayList<>();
- this.allItemsList = new ArrayList<>();
this.inventoryNames = new ArrayList<>();
- allItemsList.add(generalItems);
- allItemsList.add(consumableItems);
- allItemsList.add(equippableItems);
inventoryNames.add("General");
- inventoryNames.add("Consumable");
- inventoryNames.add("Equipment");
this.ui = new Ui();
width = 59;
height = 8;
}
- public void resetInventoryPage() {
- currentItemPageNumber = FIRST_PAGE_INDEX;
- }
-
- public void listNextInventoryPage() {
- int size = allItemsList.size();
- if (currentItemPageNumber + 1 >= size) {
- resetInventoryPage();
- } else {
- currentItemPageNumber += 1;
- }
- }
-
- public void listPreviousInventoryPage() {
- if (currentItemPageNumber <= 0) {
- currentItemPageNumber = LAST_PAGE_INDEX;
- } else {
- currentItemPageNumber -= 1;
- }
- }
-
- public void addItems(Item item) {
+ public void addItems(Consumable item) {
if (generalItems.isEmpty()) {
+ item.setQuantity(1);
generalItems.add(item);
return;
}
- int indexOfItem = generalItems.indexOf(item);
- if (indexOfItem < 0) {
+ List
- filteredList = generalItems.stream().filter(x -> x.getName().equalsIgnoreCase(
+ item.getName())).collect(Collectors.toList());
+ if (filteredList.isEmpty()) {
generalItems.add(item);
return;
}
- generalItems.get(indexOfItem).addQuantity(item.getQuantity());
- }
-
- public void addItems(Consumable item) {
- if (consumableItems.isEmpty()) {
- consumableItems.add(item);
- return;
- }
- int indexOfItem = consumableItems.indexOf(item);
- if (indexOfItem < 0) {
- consumableItems.add(item);
- return;
- }
- consumableItems.get(indexOfItem).addQuantity(item.getQuantity());
- }
-
- public void addItems(Gear item) {
- if (equippableItems.isEmpty()) {
- equippableItems.add(item);
- return;
- }
- int indexOfItem = equippableItems.indexOf(item);
- if (indexOfItem < 0) {
- equippableItems.add(item);
- return;
- }
- equippableItems.get(indexOfItem).addQuantity(item.getQuantity());
+ filteredList.get(0).addQuantity(1);
}
public void useItem(Consumable item) {
item.use(playerStatus, item, generalItems);
int leftover = item.getQuantity() - 1;
if (leftover <= 0) {
- consumableItems.remove(item);
+ generalItems.remove(item);
} else {
item.setQuantity(item.getQuantity() - 1);
}
@@ -119,10 +60,9 @@ public void sellItem(Item item) {
} catch (Exception e) {
currentTextBox.setNextError("This item cannot be sold");
}
- ArrayList
- list = allItemsList.get(currentItemPageNumber);
int leftover = item.getQuantity() - 1;
if (leftover <= 0) {
- list.remove(item);
+ generalItems.remove(item);
} else {
item.setQuantity(item.getQuantity() - 1);
}
@@ -130,10 +70,6 @@ public void sellItem(Item item) {
currentTextBox.setNextDialogue("Congrats, you just sold a " + item.getName() + " for $" + item.getSellPrice());
}
- public void loadInventory() { // for when loading game aft save
-
- }
-
public ArrayList
- getGeneralItems() {
return generalItems;
}
@@ -142,34 +78,6 @@ public void setGeneralItems(ArrayList
- items) {
this.generalItems = items;
}
- public ArrayList
- getConsumableItems() {
- return consumableItems;
- }
-
- public void setConsumableItems(ArrayList
- consumableItems) {
- this.consumableItems = consumableItems;
- }
-
- public ArrayList
- getEquippableItems() {
- return equippableItems;
- }
-
- public void setEquippableItems(ArrayList
- equippableItems) {
- this.equippableItems = equippableItems;
- }
-
- public ArrayList> getAllItemsList() {
- return allItemsList;
- }
-
- public int getCurrentItemPageNumber() {
- return currentItemPageNumber;
- }
-
- public void setCurrentItemPageNumber(int currentItemPageNumber) {
- this.currentItemPageNumber = currentItemPageNumber;
- }
-
public ArrayList getInventoryNames() {
return inventoryNames;
}
diff --git a/src/main/java/map/ShopMap.java b/src/main/java/map/ShopMap.java
index 8a595f6ca5..d1b6b5f65e 100644
--- a/src/main/java/map/ShopMap.java
+++ b/src/main/java/map/ShopMap.java
@@ -2,6 +2,7 @@
import interactable.ShopKeeper;
import filereader.FileReader;
+import inventoryitems.Consumable;
import inventoryitems.ShopItem;
import textbox.PlayerStatus;
import textbox.TextBox;
@@ -80,7 +81,7 @@ public void enableFight(Scanner in) {
int currentMoney = currentPlayer.getPlayerMoney();
currentPlayer.setPlayerMoney(currentMoney - item.getPrice());
currentTextBox.setNextNarration("NEW ITEM ADDED TO INVENTORY");
- inventory.addItems(item);
+ inventory.addItems((Consumable) item);
} else {
currentTextBox.setNextNarration("The cat silently judged your broke ass.\n");
}
diff --git a/src/main/java/math/MathPool.java b/src/main/java/math/MathPool.java
index fc34a0c86e..035b5fc53e 100644
--- a/src/main/java/math/MathPool.java
+++ b/src/main/java/math/MathPool.java
@@ -77,24 +77,25 @@ public void init() {
addMathQuestion("How many sides does a hexagon have?", 6, 2);
addMathQuestion("What is the area of a square with side length 5?", 25, 2);
addMathQuestion("What is the perimeter of a rectangle with sides 4 and 6?", 20, 2);
-// Difficulty 3
+ // Difficulty 3
addMathQuestion("What is 2 times the square root of 64?", 16, 3);
addMathQuestion("How many degrees are in a right angle?", 90, 3);
addMathQuestion("If a square has an area of 25 square units, what is the length of one side?", 5, 3);
addMathQuestion("What is the sum of the first 10 positive integers?", 55, 3);
addMathQuestion("How many edges does a cube have?", 12, 3);
-// Difficulty 4
+ // Difficulty 4
addMathQuestion("What is the value of 5 factorial (5!)?", 120, 4);
addMathQuestion("What is the next prime number after 31?", 37, 4);
addMathQuestion("How many vertices does a tetrahedron have?", 4, 4);
addMathQuestion("How many diagonals does a hexagon have?", 9, 4);
addMathQuestion("How many millimeters are in a meter?", 1000, 4);
-// Difficulty 5
+ // Difficulty 5
addMathQuestion("What is the value of 7 choose 3 (7C3)?", 35, 5);
addMathQuestion("How many faces does a dodecahedron have?", 12, 5);
- addMathQuestion("How many sides does a regular polygon have if each exterior angle measures 30 degrees?", 12, 5);
+ addMathQuestion("How many sides does a regular polygon have if each exterior angle measures 30 " +
+ "degrees?", 12, 5);
addMathQuestion("What is the 20th Fibonacci number?", 6765, 5);
addMathQuestion("What is the value of 2^10?", 1024, 5);
diff --git a/src/main/java/parser/Parser.java b/src/main/java/parser/Parser.java
index df3e84bca9..c5f5c564a7 100644
--- a/src/main/java/parser/Parser.java
+++ b/src/main/java/parser/Parser.java
@@ -3,12 +3,9 @@
import command.CommandType;
import command.fight.FightingCommand;
import command.fight.RunningCommand;
-import command.inventory.SellCommand;
import command.inventory.OpenInventoryCommand;
import command.inventory.CloseInventoryCommand;
import command.inventory.UseCommand;
-import command.inventory.NextPageCommand;
-import command.inventory.PrevPageCommand;
import command.mapmove.InteractingCommand;
import command.mapmove.MovingDownwardCommand;
import command.mapmove.MovingForwardCommand;
@@ -97,21 +94,11 @@ public Command parseCommand(String userCommand) {
command = (currentMap == mapIndex.get(FIRST_MAP_IDENTITY)) ?
new OpenInventoryCommand() : new ErrorCommand();
break;
- case INV_NEXT:
- command = (currentMap == mapIndex.get(INVENTORY_IDENTITY)) ? new NextPageCommand() : new ErrorCommand();
- break;
- case INV_PREV:
- command = (currentMap == mapIndex.get(INVENTORY_IDENTITY)) ? new PrevPageCommand() : new ErrorCommand();
- break;
case USE_ITEM:
command = (currentMap == mapIndex.get(INVENTORY_IDENTITY)) ?
new UseCommand(userCommand) : new ErrorCommand();
break;
- case SELL_ITEM:
- command = (currentMap == mapIndex.get(INVENTORY_IDENTITY)) ?
- new SellCommand(userCommand) : new ErrorCommand();
- break;
- case CLOSE_INV: // to delete aft sihan implements
+ case CLOSE_INV:
command = (currentMap == mapIndex.get(INVENTORY_IDENTITY)) ?
new CloseInventoryCommand() : new ErrorCommand();
break;
diff --git a/src/main/java/ui/Ui.java b/src/main/java/ui/Ui.java
index f3507f2067..c6072202d7 100644
--- a/src/main/java/ui/Ui.java
+++ b/src/main/java/ui/Ui.java
@@ -224,7 +224,7 @@ public void printDeathMessage(){
}
public void insertOutOfBoundsMessage(TextBox box){
- box.setNextNarration("You run straight into a wall");
+ box.setNextNarration("You ran straight into a wall");
}
public void insertObjectObstructionMessage(TextBox box){
diff --git a/tp b/tp
deleted file mode 160000
index c09c893058..0000000000
--- a/tp
+++ /dev/null
@@ -1 +0,0 @@
-Subproject commit c09c8930583e29acfed98006856f7d60e784d603