diff --git a/docs/readme.md b/docs/readme.md
new file mode 100644
index 000000000000..08c2ea21722c
--- /dev/null
+++ b/docs/readme.md
@@ -0,0 +1,114 @@
+This directory contains useful documentation, examples (keep reading),
+and [recipes](./recipes/) to get you started. For an overview of Lighthouse's
+internals, see [Lighthouse Architecture](architecture.md).
+
+## Using programmatically
+
+The example below shows how to run Lighthouse programmatically as a Node module. It
+assumes you've installed Lighthouse as a dependency (`yarn add --dev lighthouse`).
+
+```javascript
+const lighthouse = require('lighthouse');
+const chromeLauncher = require('lighthouse/chrome-launcher/chrome-launcher');
+
+function launchChromeAndRunLighthouse(url, flags, config = null) {
+ return chromeLauncher.launch().then(chrome => {
+ flags.port = chrome.port;
+ return lighthouse(url, flags, config).then(results =>
+ chrome.kill().then(() => results)
+ );
+ });
+}
+
+const flags = {output: 'json'};
+
+// Usage:
+launchChromeAndRunLighthouse('https://example.com', flags).then(results => {
+ // Use results!
+});
+```
+
+### Turn on logging
+
+If you want to see log output as Lighthouse runs, include the `log` module
+and set an appropriate logging level in your code. You'll also need to pass
+the `logLevel` flag when calling `lighthouse`.
+
+```javascript
+const log = require('lighthouse/lighthouse-core/lib/log');
+
+const flags = {logLevel: 'info', output: 'json'};
+log.setLevel(flags.logLevel);
+
+launchChromeAndRunLighthouse('https://example.com', flags).then(...);
+```
+
+## Testing on a site with authentication
+
+When installed globally via `npm i -g lighthouse` or `yarn global add lighthouse`,
+`chrome-debug` is added to your `PATH`. This binary launches a standalone Chrome
+instance with an open debugging port.
+
+- Run `chrome-debug`
+- navigate to and log in to your site
+- in a separate terminal tab `lighthouse http://mysite.com`
+
+## Testing on a mobile device
+
+Lighthouse can run against a real mobile device. You can follow the [Remote Debugging on Android (Legacy Workflow)](https://developer.chrome.com/devtools/docs/remote-debugging-legacy) up through step 3.3, but the TL;DR is install & run adb, enable USB debugging, then port forward 9222 from the device to the machine with Lighthouse.
+
+You'll likely want to use the CLI flags `--disable-device-emulation --disable-cpu-throttling` and potentially `--disable-network-throttling`.
+
+```sh
+$ adb kill-server
+
+$ adb devices -l
+* daemon not running. starting it now on port 5037 *
+* daemon started successfully *
+00a2fd8b1e631fcb device usb:335682009X product:bullhead model:Nexus_5X device:bullhead
+
+$ adb forward tcp:9222 localabstract:chrome_devtools_remote
+
+$ lighthouse --disable-device-emulation --disable-cpu-throttling https://mysite.com
+```
+
+## Lighthouse as trace processor
+
+Lighthouse can be used to analyze trace and performance data collected from other tools (like WebPageTest and ChromeDriver). The `traces` and `devtoolsLogs` artifact items can be provided using a string for the absolute path on disk. The `devtoolsLogs` array is captured from the `Network` and `Page` domains (a la ChromeDriver's [enableNetwork and enablePage options]((https://sites.google.com/a/chromium.org/chromedriver/capabilities#TOC-perfLoggingPrefs-object)).
+
+As an example, here's a trace-only run that's reporting on user timings and critical request chains:
+
+### `config.json`
+
+```json
+{
+ "audits": [
+ "user-timings",
+ "critical-request-chains"
+ ],
+
+ "artifacts": {
+ "traces": {
+ "defaultPass": "/User/me/lighthouse/lighthouse-core/test/fixtures/traces/trace-user-timings.json"
+ },
+ "devtoolsLogs": {
+ "defaultPass": "/User/me/lighthouse/lighthouse-core/test/fixtures/traces/perflog.json"
+ }
+ },
+
+ "aggregations": [{
+ "name": "Performance Metrics",
+ "description": "These encapsulate your app's performance.",
+ "scored": false,
+ "categorizable": false,
+ "items": [{
+ "audits": {
+ "user-timings": { "expectedValue": 0, "weight": 1 },
+ "critical-request-chains": { "expectedValue": 0, "weight": 1}
+ }
+ }]
+ }]
+}
+```
+
+Then, run with: `lighthouse --config-path=config.json http://www.random.url`
diff --git a/docs/recipes/custom-audit/readme.md b/docs/recipes/custom-audit/readme.md
index e9848366425d..84cc2a43e1ef 100644
--- a/docs/recipes/custom-audit/readme.md
+++ b/docs/recipes/custom-audit/readme.md
@@ -1,11 +1,32 @@
-# Basic custom audit recipe for Lighthouse
+# Basic Custom Audit Recipe
-A hypothetical site measures the time from navigation start to when the page has initialized and the main search box is ready to be used. It saves that value in a global variable, `window.myLoadMetrics.searchableTime`.
+> **Tip**: see [Lighthouse Architecture](../../../docs/architecture.md) for information
+on terminology and architecture.
-This Lighthouse [gatherer](searchable-gatherer.js)/[audit](searchable-audit.js) pair will take that value from the context of the page and test whether or not it stays below a test threshold.
+## What this example does
-The config file tells Lighthouse where to find the gatherer and audit files, when to run them, and how to incorporate their output into the Lighthouse report.
+This example shows how to write a custom Lighthouse audit for a hypothetical search page. The page is considered fully initialized when the main search box (the page's "hero element") is ready to be used. When this happens, the page uses `performance.now()` to find the time since navigation start and saves the value in a global variable called `window.myLoadMetrics.searchableTime`.
-## Run
-With site running:
-`lighthouse --config-path=custom-config.js https://test-site.url`
+## The Audit, Gatherer, and Config
+
+- [searchable-gatherer.js](searchable-gatherer.js) - a [Gatherer](https://github.com/GoogleChrome/lighthouse/blob/master/docs/architecture.md#components--terminology) that collects `window.myLoadMetrics.searchableTime`
+from the context of the page.
+
+- [searchable-audit.js](searchable-audit.js) - an [Audit](https://github.com/GoogleChrome/lighthouse/blob/master/docs/architecture.md#components--terminology) that tests whether or not `window.myLoadMetrics.searchableTime`
+stays below a 4000ms threshold. In other words, Lighthouse will consider the audit "passing"
+in the report if the search box initializes within 4s.
+
+- [custom-config.js](custom-config.js) - this file tells Lighthouse where to
+find the gatherer and audit files, when to run them, and how to incorporate their
+output into the Lighthouse report. This example extends [Lighthouse's
+default configuration](https://github.com/GoogleChrome/lighthouse/blob/master/lighthouse-core/config/default.js).
+
+**Note**: when extending the default configuration file, passes with the same name are merged together, all other arrays will be concatenated, and primitive values will override the defaults.
+
+## Run the configuration
+
+Run Lighthouse with the custom audit by using the `--config-path` flag with your configuration file:
+
+```sh
+lighthouse --config-path=custom-config.js https://example.com
+```
diff --git a/readme.md b/readme.md
index bfac9bcd662c..78cc216d0d97 100644
--- a/readme.md
+++ b/readme.md
@@ -1,42 +1,42 @@
-# Lighthouse [](https://travis-ci.org/GoogleChrome/lighthouse) [](https://coveralls.io/github/GoogleChrome/lighthouse?branch=master)
+# Lighthouse [](https://travis-ci.org/GoogleChrome/lighthouse) [](https://coveralls.io/github/GoogleChrome/lighthouse?branch=master) [](https://npmjs.org/package/lighthouse)
> Lighthouse analyzes web apps and web pages, collecting modern performance metrics and insights on developer best practices.
-**Lighthouse requires Chrome 56 or later.**
+_Lighthouse requires Chrome [stable or later](https://googlechrome.github.io/current-versions/)._
-## Installation
+## Using Lighthouse in Chrome DevTools
-### Chrome extension
+Lighthouse is integrated directly into the Chrome Developer Tools, under the "Audits" panel.
-[Install from the Chrome Web Store](https://chrome.google.com/webstore/detail/lighthouse/blipmdconlkpinefehnmjammfjpmpbjk)
+**Installation**: install [Chrome Canary](https://www.google.com/chrome/browser/canary.html).
-### Node CLI [](https://npmjs.org/package/lighthouse)
+**Run it**: open Chrome DevTools, select the Audits panel, and hit "Perform an Audit...".
-**Requires Node v6+.**
+
-```sh
-npm install -g lighthouse
-# or use yarn:
-# yarn global add lighthouse
-```
+## Using the Chrome extension
-## Running Lighthouse
+**Installation**: [install the extension](https://chrome.google.com/webstore/detail/lighthouse/blipmdconlkpinefehnmjammfjpmpbjk) from the Chrome Web Store.
-### Chrome extension
+**Run it**: follow the [extension quick-start guide](https://developers.google.com/web/tools/lighthouse/#extension).
-Check out the quick-start guide:
+## Using the Node CLI
-### CLI
+_Lighthouse requires Node 6 or later._
-Kick off a run by passing `lighthouse` the URL to audit:
+**Installation**:
```sh
-lighthouse https://airhorner.com/
+npm install -g lighthouse
+# or use yarn:
+# yarn global add lighthouse
```
+**Run it**: `lighthouse https://airhorner.com/`
+
By default, Lighthouse writes the report to an HTML file. You can control the output format by passing flags.
-#### CLI options
+### CLI options
```sh
$ lighthouse --help
@@ -128,71 +128,13 @@ NOTE: specifying an output path with multiple formats ignores your specified ext
* `./_.report.html`
* `./_.artifacts.log`
-### Testing on a site with authentication
-
- - `chrome-debug`
- - open and login to your site
- - in a separate terminal tab `lighthouse http://mysite.com`
-
-## Testing on a mobile device
-
-Lighthouse can run against a real mobile device. You can follow the [Remote Debugging on Android (Legacy Workflow)](https://developer.chrome.com/devtools/docs/remote-debugging-legacy) up through step 3.3, but the TL;DR is install & run adb, enable USB debugging, then port forward 9222 from the device to the machine with Lighthouse.
-
-You'll likely want to use the CLI flags `--disable-device-emulation --disable-cpu-throttling` and potentially `--disable-network-throttling`.
-
-```sh
-$ adb kill-server
-
-$ adb devices -l
-* daemon not running. starting it now on port 5037 *
-* daemon started successfully *
-00a2fd8b1e631fcb device usb:335682009X product:bullhead model:Nexus_5X device:bullhead
-
-$ adb forward tcp:9222 localabstract:chrome_devtools_remote
-
-$ lighthouse --disable-device-emulation --disable-cpu-throttling https://mysite.com
-```
-
-## Using programmatically
-
-The example below shows how to setup and run Lighthouse programmatically as a Node module. It
-assumes you've installed Lighthouse as a dependency (`yarn add --dev lighthouse`).
-
-```javascript
-const lighthouse = require('lighthouse');
-const chromeLauncher = require('lighthouse/chrome-launcher/chrome-launcher');
-
-function launchChromeAndRunLighthouse(url, flags, config = null) {
- return chromeLauncher.launch().then(chrome => {
- flags.port = chrome.port;
- return lighthouse(url, flags, config).then(results =>
- chrome.kill().then(() => results)
- );
- });
-}
-
-// In use:
-const flags = {output: 'json'};
-launchChromeAndRunLighthouse('https://example.com', flags)
- .then(results => console.log(results));
-```
-
-### Recipes
-> Helpful for CI integration
-
- - [gulp](docs/recipes/gulp)
-
## Viewing a report
-Lighthouse can produce a report as JSON, HTML, or stdout CLI output.
+Lighthouse can produce a report as JSON or HTML.
HTML report:
-
-
-Default CLI output:
-
-
+
### Online Viewer
@@ -207,21 +149,40 @@ right corner and signing in to GitHub.
> **Note**: shared reports are stashed as a secret Gist in GitHub, under your account.
-## Related Projects
+## Docs & Recipes
-* [webpack-lighthouse-plugin](https://github.com/addyosmani/webpack-lighthouse-plugin) - run Lighthouse from a Webpack build.
-* [lighthouse-mocha-example](https://github.com/justinribeiro/lighthouse-mocha-example) - gathers performance metrics via Lighthouse and tests them in Mocha
-* [pwmetrics](https://github.com/paulirish/pwmetrics/) - gather performance metrics
-* [lighthouse-hue](https://github.com/ebidel/lighthouse-hue) - Lighthouse score setting the color of Philips Hue lights
-* [lighthouse-batch](https://www.npmjs.com/package/lighthouse-batch) - Run Lighthouse over a number of sites in sequence and generating a summary report including all of their scores.
-* [lighthouse-cron](https://github.com/thearegee/lighthouse-cron) - Cron multiple batch Lighthouse audits and emit results for sending to remote server.
+Useful documentation, examples, and recipes to get you started.
+
+**Docs**
+
+- [Using Lighthouse programmatically](./docs/readme.md#using-programmatically)
+- [Testing a site with authentication](./docs/readme.md#testing-on-a-site-with-authentication)
+- [Testing on a mobile device](./docs/readme.md#testing-on-a-mobile-device)
+- [Lighthouse Architecture](./docs/architecture.md)
+
+**Recipes**
+
+- [gulp](docs/recipes/gulp) - helpful for CI integration
+- [Custom Audit example](./docs/recipes/custom-audit) - extend Lighthouse, run your own audits
+
+**Videos**
+
+The session from Google I/O 2017 covers architecture, writing custom audits,
+Github/Travis/CI integration, headless Chrome, and more:
+
+[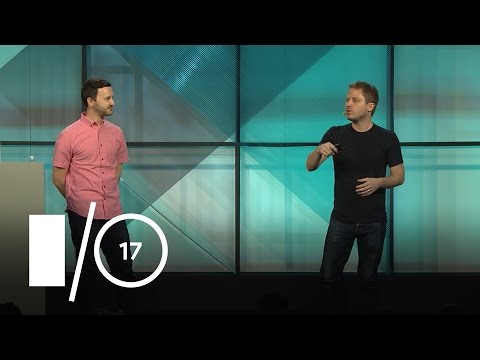](https://www.youtube.com/watch?v=NoRYn6gOtVo)
+
+_click to watch the video_
## Develop
+Read on for the basics of hacking on Lighthouse. Also see [Contributing](./CONTRIBUTING.md)
+for detailed information.
+
### Setup
```sh
-# yarn should be installed, first
+# yarn should be installed first
git clone https://github.com/GoogleChrome/lighthouse
@@ -229,13 +190,13 @@ cd lighthouse
yarn install-all
yarn build-all
-# The CLI is authored in TypeScript and requires compilation.
+# The CLI and Chrome Launcher are authored in TypeScript and require compilation.
# If you need to make changes to the CLI, run the TS compiler in watch mode:
# cd lighthouse-cli && yarn dev
+# similarly, run the TS compiler for the launcher:
+# cd chrome-launcher && yarn dev
```
-See [Contributing](./CONTRIBUTING.md) for more information.
-
### Run
```sh
@@ -247,52 +208,7 @@ through the entire app. See [Debugging Node.js with Chrome
DevTools](https://medium.com/@paul_irish/debugging-node-js-nightlies-with-chrome-devtools-7c4a1b95ae27#.59rma3ukm)
for more info.
-## Creating custom audits & gatherers
-
-The audits and gatherers checked into the lighthouse repo are available to any configuration. If you're interested in writing your own audits or gatherers, you can use them with Lighthouse without necessarily contributing upstream.
-
-Better docs coming soon, but in the meantime look at [PR #593](https://github.com/GoogleChrome/lighthouse/pull/593), and the tests [valid-custom-audit.js](https://github.com/GoogleChrome/lighthouse/blob/3f5c43f186495a7f3ecc16c012ab423cd2bac79d/lighthouse-core/test/fixtures/valid-custom-audit.js) and [valid-custom-gatherer.js](https://github.com/GoogleChrome/lighthouse/blob/3f5c43f186495a7f3ecc16c012ab423cd2bac79d/lighthouse-core/test/fixtures/valid-custom-gatherer.js). If you have questions, please file an issue and we'll help out!
-
-> **Tip**: see [Lighthouse Architecture](./docs/architecture.md) for more information on Audits and Gatherers.
-
-### Custom configurations for runs
-
-You can supply your own run configuration to customize what audits you want details on. Copy the [default.js](https://github.com/GoogleChrome/lighthouse/blob/master/lighthouse-core/config/default.js) and start customizing. Then provide to the CLI with `lighthouse --config-path=myconfig.js `
-
-If you are simply adding additional audits/gatherers or tweaking flags, you can extend the default configuration without having to copy the default and maintain it. Passes with the same name will be merged together, all other arrays will be concatenated, and primitive values will override the defaults. See the example below that adds a custom gatherer to the default pass and an audit.
-
-```json
-{
- "extends": true,
- "passes": [
- {
- "passName": "defaultPass",
- "gatherers": ["path/to/custom/gatherer.js"]
- }
- ],
- "audits": ["path/to/custom/audit.js"],
- "aggregations": [
- {
- "name": "Custom Section",
- "description": "Enter description here.",
- "scored": false,
- "categorizable": false,
- "items": [
- {
- "name": "My Custom Audits",
- "audits": {
- "name-of-custom-audit": {}
- }
- }
- ]
- }
- ]
-}
-```
-
-## Tests
-
-Some basic unit tests forked are in `/test` and run via mocha. eslint is also checked for style violations.
+### Tests
```sh
# lint and test all files
@@ -302,9 +218,10 @@ yarn test
# Requires http://entrproject.org : brew install entr
yarn watch
-## run linting and unit tests separately
+## run linting, unit, and smoke tests separately
yarn lint
yarn unit
+yarn smoke
## run closure compiler (on whitelisted files)
yarn closure
@@ -312,50 +229,18 @@ yarn closure
yarn compile-devtools
```
-## Lighthouse as trace processor
-
-Lighthouse can be used to analyze trace and performance data collected from other tools (like WebPageTest and ChromeDriver). The `traces` and `devtoolsLogs` artifact items can be provided using a string for the absolute path on disk. The devtoolsLogs array is captured from the Network domain (a la ChromeDriver's [`enableNetwork` option](https://sites.google.com/a/chromium.org/chromedriver/capabilities#TOC-perfLoggingPrefs-object)) and reformatted slightly. As an example, here's a trace-only run that's reporting on user timings and critical request chains:
-
-### `config.json`
-
-```json
-{
- "audits": [
- "user-timings",
- "critical-request-chains"
- ],
-
- "artifacts": {
- "traces": {
- "defaultPass": "/User/me/lighthouse/lighthouse-core/test/fixtures/traces/trace-user-timings.json"
- },
- "devtoolsLogs": {
- "defaultPass": "/User/me/lighthouse/lighthouse-core/test/fixtures/traces/perflog.json"
- }
- },
-
- "aggregations": [{
- "name": "Performance Metrics",
- "description": "These encapsulate your app's performance.",
- "scored": false,
- "categorizable": false,
- "items": [{
- "audits": {
- "user-timings": { "expectedValue": 0, "weight": 1 },
- "critical-request-chains": { "expectedValue": 0, "weight": 1}
- }
- }]
- }]
-}
-```
-
-Then, run with: `lighthouse --config-path=config.json http://www.random.url`
+## Related Projects
-The traceviewer-based trace processor from [node-big-rig](https://github.com/GoogleChrome/node-big-rig/tree/master/lib) was forked into Lighthouse. Additionally, the [DevTools' Timeline Model](https://github.com/paulirish/devtools-timeline-model) is available as well. There may be advantages for using one model over another.
+* [webpack-lighthouse-plugin](https://github.com/addyosmani/webpack-lighthouse-plugin) - run Lighthouse from a Webpack build.
+* [lighthouse-mocha-example](https://github.com/justinribeiro/lighthouse-mocha-example) - gather performance metrics via Lighthouse and tests them in Mocha
+* [pwmetrics](https://github.com/paulirish/pwmetrics/) - gather performance metrics
+* [lighthouse-hue](https://github.com/ebidel/lighthouse-hue) - set the color of Philips Hue lights based on a Lighthouse score
+* [lighthouse-batch](https://www.npmjs.com/package/lighthouse-batch) - run Lighthouse over a number of sites and generate a summary of their metrics/scores.
+* [lighthouse-cron](https://github.com/thearegee/lighthouse-cron) - Cron multiple batch Lighthouse audits and emit results for sending to remote server.
## FAQ
-### What is the architecture?
+### How does Lighthouse work?
See [Lighthouse Architecture](./docs/architecture.md).
@@ -374,13 +259,22 @@ If you'd like to contribute, check the [list of issues](https://github.com/Googl
Nope. Lighthouse runs locally, auditing a page using a local version of the Chrome browser installed the
machine. Report results are never processed or beaconed to a remote server.
-### Videos
+### How do I author custom audits to extend Lighthouse?
-Our session from Google I/O 2017: architecture, writing custom audits, Github/Travis/CI integration, and more:
+> **Tip**: see [Lighthouse Architecture](./docs/architecture.md) for more information
+on terminology and architecture.
-[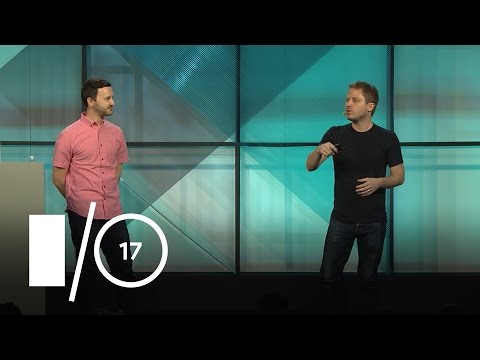](https://www.youtube.com/watch?v=NoRYn6gOtVo)
+Lighthouse can be extended to run custom audits and gatherers that you author.
+This is great if you're already tracking performance metrics in your site and
+want to surface those metrics within a Lighthouse report.
-_click to watch the video_
+If you're interested in running your own custom audits, check out our
+[Custom Audit Example](./docs/recipes/custom-audit) over in recipes.
+
+### How do I contribute?
+
+We'd love help writing audits, fixing bugs, and making the tool more useful!
+See [Contributing](./CONTRIBUTING.md) to get started.
---