diff --git a/.github/README.md.sha256 b/.github/README.md.sha256
index ebad115d75..d71c50f91c 100644
--- a/.github/README.md.sha256
+++ b/.github/README.md.sha256
@@ -1 +1 @@
-87828c8747d7ddab7f5617287553c11a296487e8c7df4881d6bf2210faae96c9
\ No newline at end of file
+7b7fd89b960a597a68ba5ae3def6618e5f5da9002c0fe695cbd50eadedf78e4c
\ No newline at end of file
diff --git a/README.md b/README.md
index d7bd03082e..8a257b7e45 100644
--- a/README.md
+++ b/README.md
@@ -1,46 +1,75 @@
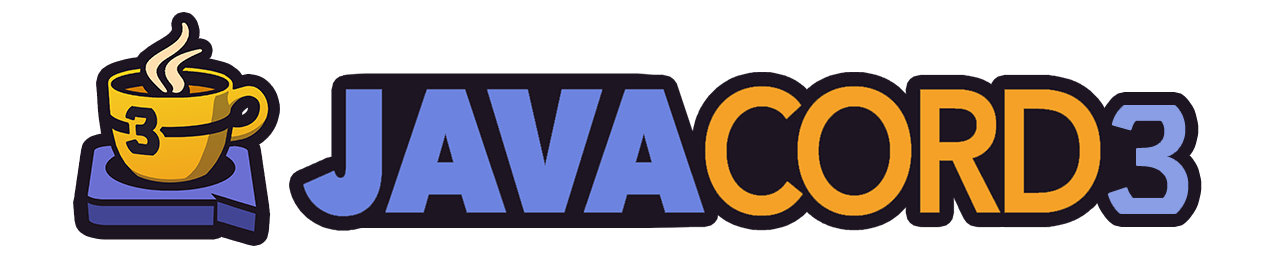
# Javacord [](https://github.com/Javacord/Javacord/releases/latest) [](https://docs.javacord.org/api/v/latest/) [](https://javacord.org/wiki/) [](https://discord.gg/0qJ2jjyneLEgG7y3)
+
An easy to use multithreaded library for creating Discord bots in Java.
-## Feature Coverage
+Javacord is a modern library that focuses on simplicity and speed ????.
+By reducing itself to standard Java classes and features like [`Optional`](https://javacord.org/wiki/essential-knowledge/working-with-optionals/)s and [`CompletableFuture`](https://javacord.org/wiki/essential-knowledge/working-with-futures/)s, it is extremely easy to use for every Java developer, as it does not require you to learn any new frameworks or complex abstractions.
+It has rich [documentation](#-documentation) and an [awesome community on Discord](#-support) that loves to help with any specific problems and questions.
+
+## ???? Basic Usage
+
+The following example logs the bot in and replies to every "!ping" message with "Pong!".
+
+```java
+public class MyFirstBot {
+
+ public static void main(String[] args) {
+ // Insert your bot's token here
+ String token = "your token";
+
+ DiscordApi api = new DiscordApiBuilder().setToken(token).login().join();
+
+ // Add a listener which answers with "Pong!" if someone writes "!ping"
+ api.addMessageCreateListener(event -> {
+ if (event.getMessageContent().equalsIgnoreCase("!ping")) {
+ event.getChannel().sendMessage("Pong!");
+ }
+ });
+
+ // Print the invite url of your bot
+ System.out.println("You can invite the bot by using the following url: " + api.createBotInvite());
+ }
-Javacord supports every action a Discord bot is able to perform.
-Some of these features include, but are not limited to:
+}
+```
-- sending messages
-- attaching files
-- event listening
-- user management
-- server administration
-- *... **and many others***
+
-**Sending voice will be added in an upcoming release**.
-New features introduced by Discord are typically added in less than one week, depending on scale.
+More sophisticated examples can be found at the [end of the README](#-more-examples).
+You can also check out the [example bot](https://github.com/Javacord/Example-Bot) for a fully functional bot.
-## Download / Installation
+## ???? Download / Installation
The recommended way to get Javacord is to use a build manager, like Gradle or Maven.
-If you are not familiar with build managers, you can follow this [Setup Guide](#ide-setup)
-or download Javacord directly from [GitHub](https://github.com/Javacord/Javacord/releases/latest).
+If you are not familiar with build managers, you can follow this [setup guide](#-ide-setup) or download Javacord directly from [GitHub](https://github.com/Javacord/Javacord/releases/latest).
### Javacord Dependency
#### Gradle
+
```groovy
repositories { mavenCentral() }
-dependencies { implementation 'org.javacord:javacord:3.0.5' }
+dependencies { implementation 'org.javacord:javacord:3.0.6' }
```
#### Maven
+
```xml
org.javacord
javacord
- 3.0.5
+ 3.0.6
pom
```
+#### Sbt
+
+```scala
+libraryDependencies ++= Seq("org.javacord" % "javacord" % "3.0.6")
+```
+
### Optional Logger Dependency
Any Log4j-2-compatible logging framework can be used to provide a more sophisticated logging experience
@@ -51,100 +80,156 @@ For example, Log4j Core in Gradle
```groovy
dependencies { runtimeOnly 'org.apache.logging.log4j:log4j-core:2.11.0' }
```
-Take a look at the [Logger Configuration](https://javacord.org/wiki/basic-tutorials/logger-configuration/) wiki article for further information.
+Take a look at the [logger configuration](https://javacord.org/wiki/basic-tutorials/logger-configuration/) wiki article for further information.
-## IDE Setup
+## ???? IDE Setup
If you have never used Gradle or Maven before, you should take a look at one of the setup tutorials:
* **[IntelliJ & Gradle](https://javacord.org/wiki/getting-started/intellij-gradle/)** _(recommended)_
* **[IntelliJ & Maven](https://javacord.org/wiki/getting-started/intellij-maven/)**
* **[Eclipse & Maven](https://javacord.org/wiki/getting-started/eclipse-maven/)**
-## Support
+## ???? Support
Javacord's Discord community is an excellent resource if you have questions about the library.
-* **[The Javacord Server](https://discord.gg/0qJ2jjyneLEgG7y3)**
+* **[The Javacord server](https://discord.gg/0qJ2jjyneLEgG7y3)**
-## Documentation
+## ???? Documentation
-* The [Javacord Wiki](https://javacord.org/wiki/) is a great place to get started
-* Additional documentation can be found in the [JavaDoc](https://docs.javacord.org/api/v/latest/)
+* The [Javacord wiki](https://javacord.org/wiki/) is a great place to get started.
+* Additional documentation can be found in the [JavaDoc](https://docs.javacord.org/api/v/latest/).
-## Logging in
+## ???? How to Create a Bot User and Get Its Token
-Logging in is very simple
-```java
-public class MyFirstBot {
+* **[Creating a Bot User Account](https://javacord.org/wiki/essential-knowledge/creating-a-bot-account/)**
- public static void main(String[] args) {
- // Insert your bot's token here
- String token = "your token";
+## ???? Version Numbers
- DiscordApi api = new DiscordApiBuilder().setToken(token).login().join();
+The version number has a 3-digit format: `major.minor.trivial`
+* `major`: Increased extremely rarely to mark a major release (usually a rewrite affecting very huge parts of the library).
+* `minor`: Any backward incompatible change to the api.
+* `trivial`: A backward compatible change to the **api**. This is usually an important bugfix (or a bunch of smaller ones)
+ or a backwards compatible feature addition.
+
+## ???? Deprecation Policy
- // Add a listener which answers with "Pong!" if someone writes "!ping"
- api.addMessageCreateListener(event -> {
- if (event.getMessageContent().equalsIgnoreCase("!ping")) {
- event.getChannel().sendMessage("Pong!");
- }
- });
+A method or class that is marked as deprecated can be removed with the next minor release (but it will usually stay for
+several minor releases). A minor release might remove a class or method without having it deprecated, but we will do our
+best to deprecate it before removing it. We are unable to guarantee this though, because we might have to remove / replace
+something due to changes made by Discord, which we are unable to control. Usually you can expect a deprecated method or
+class to stay for at least 6 months before it finally gets removed, but this is not guaranteed.
- // Print the invite url of your bot
- System.out.println("You can invite the bot by using the following url: " + api.createBotInvite());
+## ???? Large Bots Using Javacord
+
+Javacord is used by many large bots. Here are just a few of them:
+* [**Yunite**](https://yunite.xyz/): A bot for Fortnite which runs on over 30,000 servers with over five million users.
+* [**Beemo**](https://beemo.gg/): A bot that prevents raids of many large servers such as [discord.gg/LeagueOfLegends](https://discord.gg/LeagueOfLegends), [discord.gg/VALORANT](https://discord.gg/VALORANT), and many more.
+
+If you own a large bot that uses Javacord, feel free to add it to the list in a pull request!
+
+## ???? More Examples
+
+### Using the MessageBuilder ????
+
+
+
+The following example uses the built-in `MessageBuilder`. It is very useful to construct complex messages with images, code-blocks, embeds, or attachments.
+
+```java
+new MessageBuilder()
+ .append("Look at these ")
+ .append("awesome", MessageDecoration.BOLD, MessageDecoration.UNDERLINE)
+ .append(" animal pictures! ????")
+ .appendCode("java", "System.out.println(\"Sweet!\");")
+ .addAttachment(new File("C:/Users/JohnDoe/Pictures/kitten.jpg"))
+ .addAttachment(new File("C:/Users/JohnDoe/Pictures/puppy.jpg"))
+ .setEmbed(new EmbedBuilder()
+ .setTitle("WOW")
+ .setDescription("Really cool pictures!")
+ .setColor(Color.ORANGE))
+ .send(channel);
+```
+
+### Listeners in Their Own Class ????
+
+All the examples use inline listeners for simplicity. For better readability it is also possible to have listeners in their own class:
+
+```java
+public class MyListener implements MessageCreateListener {
+
+ @Override
+ public void onMessageCreate(MessageCreateEvent event) {
+ Message message = event.getMessage();
+ if (message.getContent().equalsIgnoreCase("!ping")) {
+ event.getChannel().sendMessage("Pong!");
+ }
}
}
```
-
-You can also login non-blocking asynchronously
```java
-public class MyFirstBot {
+api.addListener(new MyListener());
+```
- public static void main(String[] args) {
- // Insert your bot's token here
- String token = "your token";
+For commands, you have the option of using one of the many existing command frameworks such as
+* [**Command Framework**](https://github.com/Vampire/command-framework) by [@Vampire](https://github.com/Vampire)
+* [**Sdcf4j**](https://github.com/Bastian/sdcf4j) by [@Bastian](https://github.com/Bastian)
- new DiscordApiBuilder().setToken(token).login().thenAccept(api -> {
- // Add a listener which answers with "Pong!" if someone writes "!ping"
- api.addMessageCreateListener(event -> {
- if (event.getMessageContent().equalsIgnoreCase("!ping")) {
- event.getChannel().sendMessage("Pong!");
- }
- });
-
- // Print the invite url of your bot
- System.out.println("You can invite the bot by using the following url: " + api.createBotInvite());
- })
- // Log any exceptions that happened
- .exceptionally(ExceptionLogger.get());
- }
+or even write your own!
+
+### Attach Listeners to Objects ????
+
+You can even attach listeners to objects.
+Let's say you have a very sensitive bot.
+As soon as someone reacts with a ???? within the first 30 minutes of message creation, it deletes its own message.
+```java
+api.addMessageCreateListener(event -> {
+ if (event.getMessageContent().equalsIgnoreCase("!ping")) {
+ event.getChannel().sendMessage("Pong!").thenAccept(message -> {
+ // Attach a listener directly to the message
+ message.addReactionAddListener(reactionEvent -> {
+ if (reactionEvent.getEmoji().equalsEmoji("????")) {
+ reactionEvent.deleteMessage();
+ }
+ }).removeAfter(30, TimeUnit.MINUTES);
+ });
+ }
}
```
+The result then looks like this:
-Check out the [Example Bot](https://github.com/Javacord/Example-Bot) to learn more.
+
-## How to create a bot user and get its token
+### Creating a Temporary Voice Channel ????????
-* **[Creating a Bot User Account](https://javacord.org/wiki/essential-knowledge/creating-a-bot-account/)**
+This example creates a temporary voice channel that gets deleted when the last user leaves it or if nobody joins it within the first 30 seconds after creation.
-## Version numbers
+```java
+Server server = ...;
+ServerVoiceChannel channel = new ServerVoiceChannelBuilder(server)
+ .setName("tmp-channel")
+ .setUserlimit(10)
+ .create()
+ .join();
+
+// Delete the channel if the last user leaves
+channel.addServerVoiceChannelMemberLeaveListener(event -> {
+ if (event.getChannel().getConnectedUserIds().isEmpty()) {
+ event.getChannel().delete();
+ }
+});
-The version number has a 3-digit format: `major.minor.trivial`
-* `major`: Increased extremely rarely to mark a major release (usually a rewrite affecting very huge parts of the library).
- You can expect this digit to not change for several years.
-* `minor`: Any backwards incompatible change to the api. You can expect this digit to change about 1-3 times per year.
-* `trivial`: A backwards compatible change to the **api**. This is usually an important bugfix (or a bunch of smaller ones)
- or a backwards compatible feature addition. You can expect this digit to change 1-2 times per month.
-
-## Deprecation policy
+// Delete the channel if no user joined in the first 30 seconds
+api.getThreadPool().getScheduler().schedule(() -> {
+ if (channel.getConnectedUserIds().isEmpty()) {
+ channel.delete();
+ }
+}, 30, TimeUnit.SECONDS);
+```
-A method or class that is marked as deprecated can be removed with the next minor release (but it will usually stay for
-several minor releases). A minor release might remove a class or method without having it deprecated, but we will do our
-best to deprecate it before removing it. We are unable to guarantee this though, because we might have to remove / replace
-something due to changes made by Discord, which we are unable to control. Usually you can expect a deprecated method or
-class to stay for at least 6 months before it finally gets removed, but this is not guaranteed.
+> **Note**: You should also make sure to remove the channels on bot shutdown (or startup)
-## Discord Server
+## ???? License
-Join the [Javacord Server](https://discord.gg/0qJ2jjyneLEgG7y3) for support, status updates, or just chatting with other users.
+Javacord is distributed under the [Apache license version 2.0](./LICENSE).
diff --git a/gradle.properties b/gradle.properties
index 899abb2904..c599dd7b1e 100644
--- a/gradle.properties
+++ b/gradle.properties
@@ -1,2 +1,2 @@
org.gradle.caching = true
-version = 3.0.6-SNAPSHOT
+version = 3.0.6