-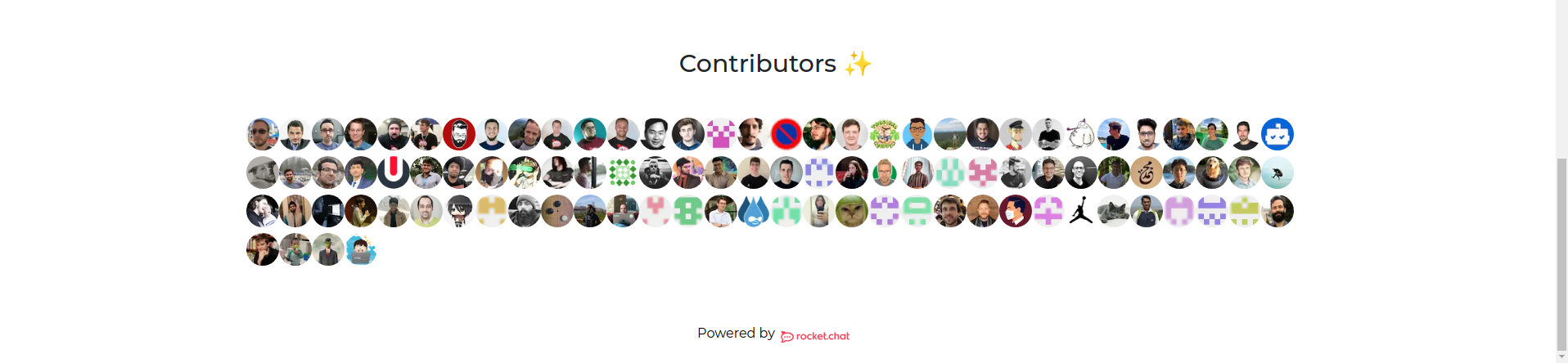
-## Steps to setup your own GitHub Components:
+### 2. Issues
-### CMS
+
+
+
-1. We need to add cron jobs to handle the fetch requests to GitHub, as frequent fetch request will forbid the IP address to fetch data. You can find `cron.js` file under `cms/config/functions` folder.
- This example means after each 1 hour we will re-fetch and update our data.
- 
-2. These functions exist at `github.js` file under the same functions directory. If you will check, you need to provide `owner` and `repo` to get data. Simply, owner = organisation or the user and repo = repository.
-3. Just one more change and we are good to go! As the cron job will populate and re-populate data after 1 hour interval. We can't just wait for the first one hour to work with the data right? So we need an initial fetch!
-4. Go to `fetchData.js` file which is also in the same functions directory and change the `owner` and `repo` here so that when we first call `INITIALIZE_DATA=true npm run develop` it will call these functions and populate the data for us!
- 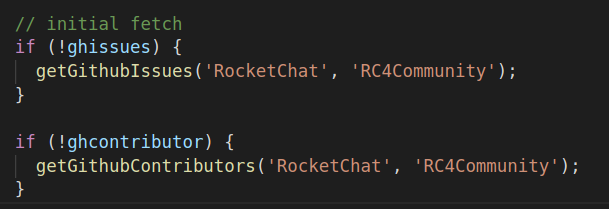
+### 3. Contributors
-### Frontend
+
+
+
+
+### 4. Pull Requests
-1. All the GitHub Components reside in the `app/components/github` folder. You can just pull any one of them and use however you like.
-2. There are helper functions in `lib/github.js` file, so that you don't worry much about setting up things or handling errors and focus on instant results.
-3. Now that you know where stuffs exist, let's get started:
-4. While using `getStaticProps` you can fetch data through the helper functions we talked about in _point 2_. So in any page you wish to render them you just need to,
+
+
+
-```javascript
-export async function getStaticProps({ params }) {
- ...
- // owner is (organisation/user), repo is (repository)
- const issues = await getIssues('RocketChat', 'RC4Community');
- const contributors = await getContributors();
- return {
- props: { issues, contributors }
- }
+
+
+All the componets use the same `` tag and the same data fetchig library with additional paramters.
+
+## Github Tag Props
+
+We use our helper function `githubKitData(repoName,ownerName,[... needs]);` to fetch the data for the component. The returned object can be directly passed to the component and it will render data based on the passed paramters
+
+| Prop Name | Description | Type |
+| ------------- |------------------------- | -----|
+| type | This specifies the `type` of github kit components we wish to use. If `type` is not specified, by default the `repository overview` component is rendered. Type can be set to : `issues` , `pulls` or `contributors` | string |
+| githubData | This will contain the data which will be rendered by the component | json |
+
+# Usage Examples
+
+## #Example 1 : Using GitHub Repository Overview , Issues, Contributors and Pull Request all at once.
+
+### Using the component
+
+```
+import Head from "next/head";
+import { Github } from '../components/github';
+import { githubKitData } from '../lib/github';
+
+export default function Leaderboardpage(props){
+ return (
+
+
+ GSOC2022 LeaderBoard
+
+
+
+ Repository Overview
+
+
+
+
+
+ GitHub Issues
+
+
+
+
+
+
+ GitHub Pull Requests
+
+
+
+
+
+
+ Contributors ✨
+
+
+
+
+ );
+}
+
+export async function getStaticProps(){
+
+ const githubData = await githubKitData('RocketChat','RC4Community',['issues','pulls','contributors']);
+ const topNavItems = await fetchAPI("/top-nav-item");
+
+ return {
+ props: {
+ leaderboardProps,
+ githubData
+ },
+ revalidate: 30,
+ };
}
```
-5. Now use them like any react component (make sure to import them),
+### Setting up component data in CMS
+
+1. Open the your cron.js ( This can be located in the following path : `RC4Community/cms/config/functions/cron.js` ).
+2. We need to add cron jobs to handle the fetch requests to GitHub, as frequent fetch request will forbid the IP address to fetch data.
+ This example means after each 60 seconds we will re-fetch and update our data.
+
+ 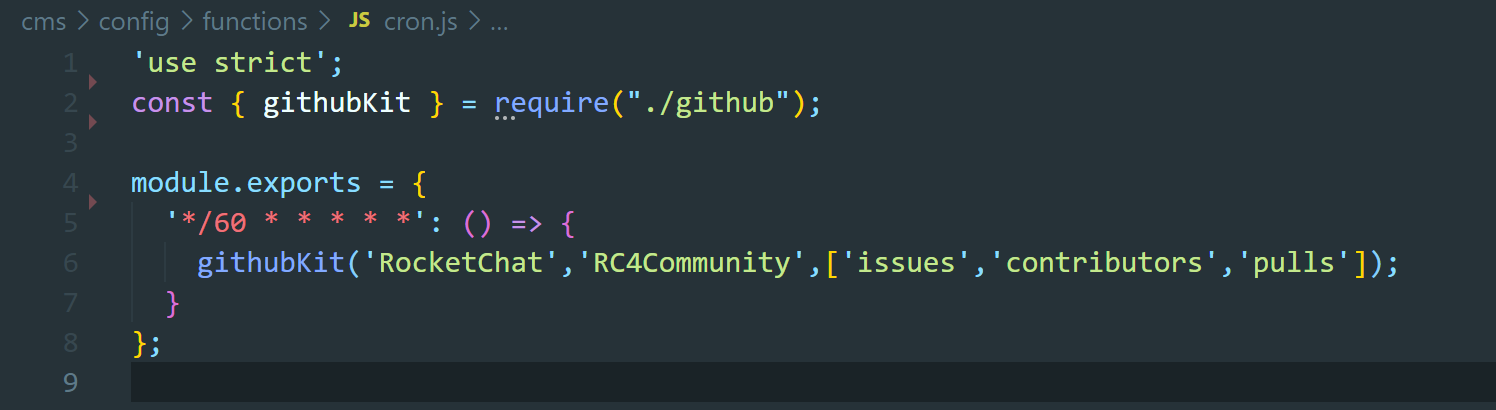
+
+3. The `githubKit()` function takes three arguments:
+ 1. The github user name of the owner of the repostory.
+ 2. Name of the repository.
+ 3. The components we wish to use, as it fetches data accordigly. This will be an array of strinng and can take a combinationof values : `issues`, `contributors`,`pulls` depending on our usecase.
+
+4. Just one more change and we are good to go! As the cron job will populate and re-populate data after some time interval. We can't just wait for the first one hour to work with the data right? So we need an initial fetch!
+
+5. Go to `fetchData.js` file which is also in the same functions directory and change the `owner` and `repo` here so that when we first call `INITIALIZE_DATA=true npm run develop` it will call these functions and populate the data for us!
+
+
+
+## #Example 2 : Using Contributors and PullRequest components for the Rocket.Chat repository of RocketChat.
+
+### Using the component
-```jsx
-
-or
-
```
+import Head from "next/head";
+import { Github } from '../components/github';
+import { githubKitData } from '../lib/github';
+
+export default function Leaderboardpage(props){
+ return (
+
+
+ GSOC2022 LeaderBoard
+
+
+
+ GitHub Pull Requests
+
+
+
+
+
+
+ Contributors ✨
+
+
+
+
+ );
+}
+
+export async function getStaticProps(){
+
+ const githubData = await githubKitData('RocketChat','RC4Community',['contributors','pulls']);
+ const topNavItems = await fetchAPI("/top-nav-item");
+
+ return {
+ props: {
+ leaderboardProps,
+ githubData
+ },
+ revalidate: 30,
+ };
+}
+```
+
+### Setting up component data in CMS
+
+
+1. Cron Jobs 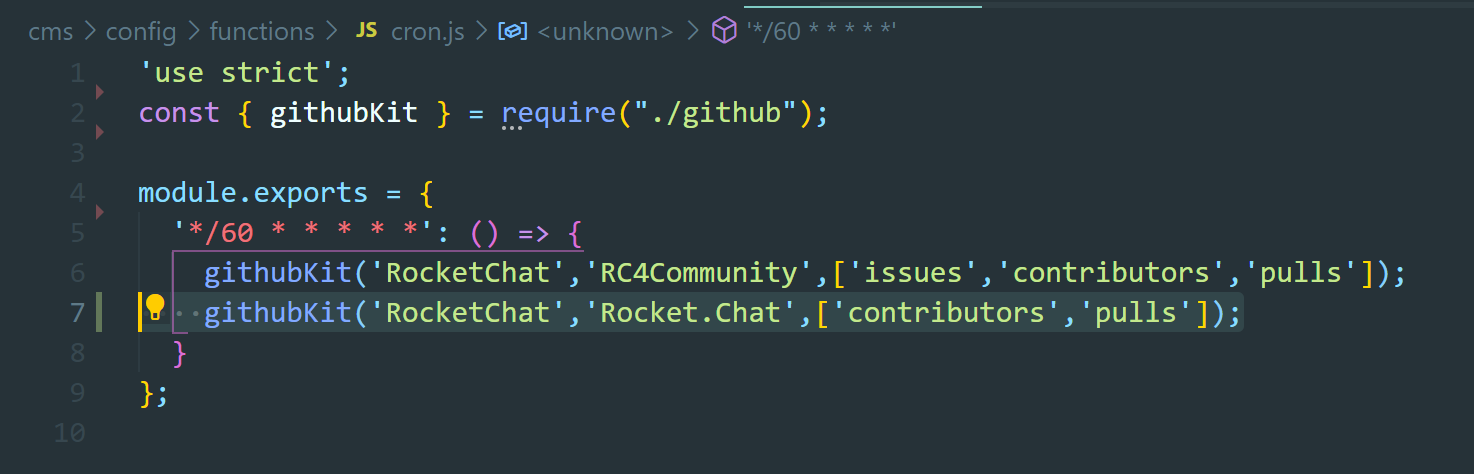
+
+2. Initial Fetch 
+
+
+## #Example 3 : Using Repository Overview Component only.
+
+Note : the repository overview component can be used by default. We don not need to specify any `type` in Github component or add anything to `needed` in `githubKitData`
+or in `githubKit` in the cron job.
+
+### Using the component
+
+```
+import Head from "next/head";
+import { Github } from '../components/github';
+import { githubKitData } from '../lib/github';
+
+export default function Leaderboardpage(props){
+ return (
+
+
+ GSOC2022 LeaderBoard
+
+
+
+ Repository Overview
+
+
+
+
+ );
+}
+
+export async function getStaticProps(){
+
+ const githubData = await githubKitData('RocketChat','RC4Community');
+ const topNavItems = await fetchAPI("/top-nav-item");
+
+ return {
+ props: {
+ leaderboardProps,
+ githubData
+ },
+ revalidate: 30,
+ };
+}
+```
+
+### Setting up component data in CMS
+
+
+1. Cron Jobs 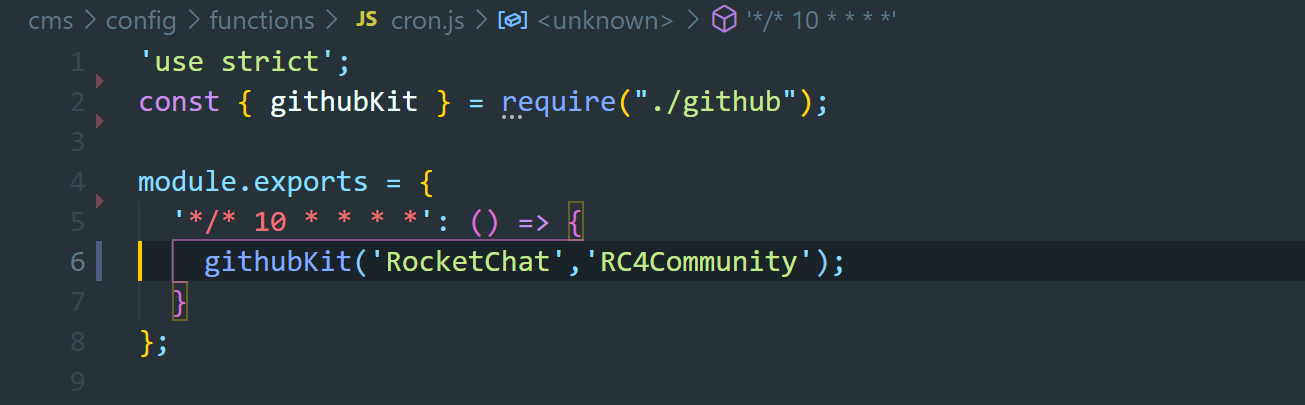
+
+2. Initial Fetch 
+
+
+
----
-### :arrow_left: Explore More Components
+### :arrow_left: Explore More Components
\ No newline at end of file