diff --git a/.markdownlint.json b/.markdownlint.json
index 145d285..5602e44 100644
--- a/.markdownlint.json
+++ b/.markdownlint.json
@@ -2,5 +2,6 @@
"MD013": false,
"MD024": false,
"MD033": false,
- "MD041": false
+ "MD041": false,
+ "MD046": false
}
\ No newline at end of file
diff --git a/.vscode/settings.json b/.vscode/settings.json
new file mode 100644
index 0000000..12ecef8
--- /dev/null
+++ b/.vscode/settings.json
@@ -0,0 +1,13 @@
+{
+ "yaml.schemas": {
+ "https://squidfunk.github.io/mkdocs-material/schema.json": "mkdocs.yml"
+ },
+ "yaml.customTags": [
+ "!ENV scalar",
+ "!ENV sequence",
+ "!relative scalar",
+ "tag:yaml.org,2002:python/name:material.extensions.emoji.to_svg",
+ "tag:yaml.org,2002:python/name:material.extensions.emoji.twemoji",
+ "tag:yaml.org,2002:python/name:pymdownx.superfences.fence_code_format"
+ ]
+ }
\ No newline at end of file
diff --git a/docs/alternatives.md b/docs/alternatives.md
index 938af5e..d3d05d9 100644
--- a/docs/alternatives.md
+++ b/docs/alternatives.md
@@ -2,11 +2,6 @@
title: Alternatives
description: Alternatives to Satchel along with pros and cons.
icon: material/card-multiple-outline
-comments: true
-tags:
- - Others
- - Comparison
- - Third-party
---
Satchel isn't the best backpack system out there or the only one but it does offer some advantages against others. Some free and open-souce alternaves of Satchel.
diff --git a/docs/api-reference.md b/docs/api-reference.md
index 3ac1d69..bf47fdd 100644
--- a/docs/api-reference.md
+++ b/docs/api-reference.md
@@ -2,15 +2,56 @@
title: API Reference
description: Satchel is a reskin of the default BackpackGui. Satchel acts very similar to the default backpack and is based on a fork on the default backpack.
icon: material/book-outline
-comments: true
-tags:
- - API
- - Summary
- - Documentation
---
+
+
Satchel is a reskin of the default BackpackGui located in [CoreGui](https://create.roblox.com/docs/reference/engine/classes/CoreGui). Satchel acts very similar to the default backpack and is based on a fork on the default backpack. Behaviors between the two should remain the same with both of them managing the [Backpack](https://create.roblox.com/docs/reference/engine/classes/Backpack).
+#### Code Samples
+
+This code sample makes a TextButton that toggles the inventory when clicked.
+
+```lua title="Toggle Satchel" linenums="1"
+local ReplicatedStorage = game:GetService("ReplicatedStorage")
+local Satchel = require(ReplicatedStorage:WaitForChild("Satchel"))
+
+local button = Instance.new("TextButton")
+button.AnchorPoint = Vector2.new(0.5, 0.5)
+button.Position = UDim2.new(0.5, 0, 0.5, 0)
+button.Text = "Toggle Inventory"
+button.MouseButton1Click:Connect(function()
+ if Satchel:GetBackpackEnabled() then
+ Satchel.SetBackpackEnabled(false)
+ else
+ Satchel.SetBackpackEnabled(true)
+ end
+end)
+```
+
+This code sample detects when the inventory is opened or closed.
+
+```lua title="Detect Inventory State" linenums="1"
+local ReplicatedStorage = game:GetService("ReplicatedStorage")
+local Satchel = require(ReplicatedStorage:WaitForChild("Satchel"))
+
+Satchel.GetStateChangedEvent():Connect(function(isOpened: boolean)
+ if isOpened then
+ print("Inventory opened")
+ else
+ print("Inventory closed")
+ end
+end)
+```
+
## Summary
### Attributes
@@ -52,26 +93,38 @@ Satchel is a reskin of the default BackpackGui located in [CoreGui](https://crea
### BackgroundColor3
+[`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3)
+
Determines the background color of the default inventory window and slots. Changing this will update the background color for all elements excluding the search box background for visibility purposes.
### BackgroundTransparency
+[`number`](https://create.roblox.com/docs/luau/numbers)
+
Determines the background transparency of the default inventory window and slots. This will change how the hot bar looks in its locked state and the inventory background.
### CornerRadius
+[`UDim`](https://create.roblox.com/docs/reference/engine/datatypes/UDim)
+
Determines the radius, in pixels, of the default inventory window and slots. This will affect all elements with a visible rounded corner. The corner radius for the search bar is calculated automatically based on this value.
### EquipBorderColor3
+[`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3)
+
Determines the color of the equip border when a slot is equipped. The drag outline color of the slot will not changed by this.
### EquipBorderSizePixel
+[`number`](https://create.roblox.com/docs/luau/numbers)
+
Determines the pixel width of the equip border when a slot is equipped. This additionally controls the padding of tool icons.
### FontFace
+[`Enum.Font`](https://create.roblox.com/docs/reference/engine/enums/Font)
+
Determines the font of the default inventory window and slots. This includes all text in the Satchel UI.
!!! bug
@@ -80,26 +133,38 @@ Determines the font of the default inventory window and slots. This includes all
### InsetIconPadding
+[`bool`](https://create.roblox.com/docs/luau/booleans)
+
Determines whether or not the tool icon is padded in the default inventory window and slots. Changing this will change how the tool icon is padded in the slot or not.
### OutlineEquipBorder
+[`bool`](https://create.roblox.com/docs/luau/booleans)
+
Determines whether or not the equip border is outline or inset when a slot is equipped. Changing this will make the equip border either border will outline or inset the slot.
### TextColor3
+[`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3)
+
Determines the color of the text in default inventory window and slots. This will change the color of all text.
### TextSize
+[`number`](https://create.roblox.com/docs/luau/numbers)
+
Determines the size of the text in the default inventory window and slots. This will change the text size of the tool names and will not change other text like search text, hotkey number, and gamepad hints.
### TextStrokeColor3
+[`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3)
+
Determines the color of the text stroke of text in default inventory window and slots. This will change the color of all text strokes which are visible.
### TextStrokeTransparency
+[`number`](https://create.roblox.com/docs/luau/numbers)
+
Determines the transparency of the text stroke of text in default chat window and slots. This will change all text strokes in which text strokes are visible.
## Methods
@@ -108,34 +173,105 @@ Determines the transparency of the text stroke of text in default chat window an
Returns whether the inventory is opened or not.
+#### Code Samples
+
+This code sample will return whether the inventory is opened or not.
+
+```lua title="Is Opened" linenums="1"
+local ReplicatedStorage = game:GetService("ReplicatedStorage")
+local Satchel = require(ReplicatedStorage:WaitForChild("Satchel"))
+
+local isOpened = Satchel.IsOpened()
+```
+
#### Returns
-```boolean```
+
### GetStateChangedEvent
Returns a signal that fires when the inventory is opened or closed.
+#### Code Samples
+
+This code sample detects when the inventory is opened or closed.
+
+```lua title="Detect Inventory State" linenums="1"
+local ReplicatedStorage = game:GetService("ReplicatedStorage")
+local Satchel = require(ReplicatedStorage:WaitForChild("Satchel"))
+
+Satchel.GetStateChangedEvent():Connect(function(isOpened: boolean)
+ if isOpened then
+ print("Inventory opened")
+ else
+ print("Inventory closed")
+ end
+end)
+```
+
#### Returns
-```RBXScriptSignal```
+
diff --git a/docs/app.webmanifest b/docs/app.webmanifest
new file mode 100644
index 0000000..30f1064
--- /dev/null
+++ b/docs/app.webmanifest
@@ -0,0 +1,24 @@
+{
+ "name": "Satchel Documentation",
+ "short_name": "Satchel",
+ "icons": [
+ {
+ "src": "/icons/512.png",
+ "type": "image/png",
+ "sizes": "512x512"
+ },
+ {
+ "src": "/icons/512-maskable.png",
+ "type": "image/png",
+ "sizes": "512x512",
+ "purpose": "maskable"
+ }
+ ],
+ "start_url": "/",
+ "display": "standalone",
+ "theme_color": "#00a2ff",
+ "background_color": "#00a2ff",
+ "lang": "en",
+ "description": "Documentation for Satchel, a modern open-source alternative to Roblox's default backpack",
+ "categories": ["utilities", "reference", "developer"]
+}
diff --git a/docs/assets/satchel-black.svg b/docs/assets/satchel-black.svg
new file mode 100644
index 0000000..4b9701d
--- /dev/null
+++ b/docs/assets/satchel-black.svg
@@ -0,0 +1,3 @@
+
diff --git a/docs/assets/satchel-white.svg b/docs/assets/satchel-white.svg
new file mode 100644
index 0000000..af3bf4c
--- /dev/null
+++ b/docs/assets/satchel-white.svg
@@ -0,0 +1 @@
+
\ No newline at end of file
diff --git a/docs/benchmarks.md b/docs/benchmarks.md
index ca4607c..b0130fb 100644
--- a/docs/benchmarks.md
+++ b/docs/benchmarks.md
@@ -2,11 +2,6 @@
title: Benchmarks
description: Satchel benchmarks on performance and speed.
icon: material/speedometer
-comments: true
-tags:
- - Tests
- - Speed
- - Performance
---
Built for speed, optimized scripting allows Satchel to be blazingly fast. As faster or even faster than the default speed.
diff --git a/docs/contributing.md b/docs/contributing.md
new file mode 100644
index 0000000..a6bdd43
--- /dev/null
+++ b/docs/contributing.md
@@ -0,0 +1,35 @@
+---
+title: Contributing
+description: Learn how to contribute to our project.
+icon: material/heart-multiple-outline
+---
+
+Thank you for investing your time in contributing to our project!
+
+Please read our [Code of Conduct](https://github.com/RyanLua/Satchel#coc-ov-file) to keep our community approachable and respectable.
+
+When you contribute, you become a project contributor and both are shown on [our repository](https://github.com/RyanLua/Satchel), [contributors graph](https://github.com/RyanLua/Satchel/graphs/contributors), and [your contribution activity](https://docs.github.com/en/account-and-profile/setting-up-and-managing-your-github-profile/managing-contribution-settings-on-your-profile/viewing-contributions-on-your-profile#contribution-activity).
+
+## GitHub Codespaces
+
+We are fully integrated with [GitHub Codespaces](https://docs.github.com/en/codespaces). When you create a codespace on our repository, all required extensions and dependencies are automatically installed to make contributing easier. The following will be automatically available:
+
+* [Rojo](https://github.com/rojo-rbx/rojo) sync (If editing in Visual Studio Code from your local desktop client. Requires [Visual Studio Code](https://code.visualstudio.com/download/) with the [GitHub Codespaces](https://marketplace.visualstudio.com/items?itemName=GitHub.codespaces) extension)
+* [Rojo](https://github.com/rojo-rbx/rojo), [Selene](https://github.com/Kampfkarren/selene), and [StyLua](https://github.com/JohnnyMorganz/StyLua) Extensions
+* [Wally](https://github.com/UpliftGames/wally/) packages
+
+Additionally, our codespaces are preconfigured with [pre-builds](https://docs.github.com/en/codespaces/prebuilding-your-codespaces/about-github-codespaces-prebuilds) and [dev containers](https://docs.github.com/en/codespaces/setting-up-your-project-for-codespaces/adding-a-dev-container-configuration/introduction-to-dev-containers).
+
+## Coding Style
+
+If you plan to contribute, ensure that all code written in Lua follows the [Roblox Lua Style Guide](https://roblox.github.io/lua-style-guide/) when applicable.
+
+Also, make sure that your code is properly styled and linted using [Selene](https://github.com/Kampfkarren/selene) and [StyLua](https://github.com/JohnnyMorganz/StyLua).
+
+## Pull Requests
+
+We actively welcome your pull requests. When you submit a pull request, we will review your code and respond as soon as possible. We may suggest changes, improvements, or alternatives.
+
+## License
+
+By contributing, you agree that your contributions will be licensed under the [Mozilla Public License 2.0](http://mozilla.org/MPL/2.0/).
diff --git a/docs/favicon.png b/docs/favicon.png
index 6a33149..bf5c89d 100644
Binary files a/docs/favicon.png and b/docs/favicon.png differ
diff --git a/docs/icons/512-maskable.png b/docs/icons/512-maskable.png
new file mode 100644
index 0000000..496f01e
Binary files /dev/null and b/docs/icons/512-maskable.png differ
diff --git a/docs/icons/512.png b/docs/icons/512.png
new file mode 100644
index 0000000..bf5c89d
Binary files /dev/null and b/docs/icons/512.png differ
diff --git a/docs/index.md b/docs/index.md
index 309fbba..808db02 100644
--- a/docs/index.md
+++ b/docs/index.md
@@ -6,16 +6,16 @@ hide:
- feedback
---
-
-
+
+
---
-???+ abstract "Please don't scroll away"
+
Satchel is a modern open-source alternative to Roblox's default backpack.
@@ -23,6 +23,10 @@ Satchel aims to be more customizable and easier to use than the default backpack
This documentation will allow you to install Satchel and learn about how to script using Satchel.
+
+
+
+
---
diff --git a/docs/installation.md b/docs/installation.md
index 8e6acb7..27c147a 100644
--- a/docs/installation.md
+++ b/docs/installation.md
@@ -2,11 +2,6 @@
title: Installation
description: Use of Satchel very easy. Highly customizable using instance attributes and with scripting support.
icon: material/download-outline
-comments: true
-tags:
- - Guide
- - Creator Marketplace
- - GitHub Releases
---
Installing Satchel is easy and painless. Satchel is a drag-and-drop module that works out of the box and with no configuration needed.
@@ -14,37 +9,45 @@ Installing Satchel is easy and painless. Satchel is a drag-and-drop module that
### Creator Marketplace
1. Get the Satchel module from the [Creator Marketplace](https://create.roblox.com/marketplace/asset/13947506401).
-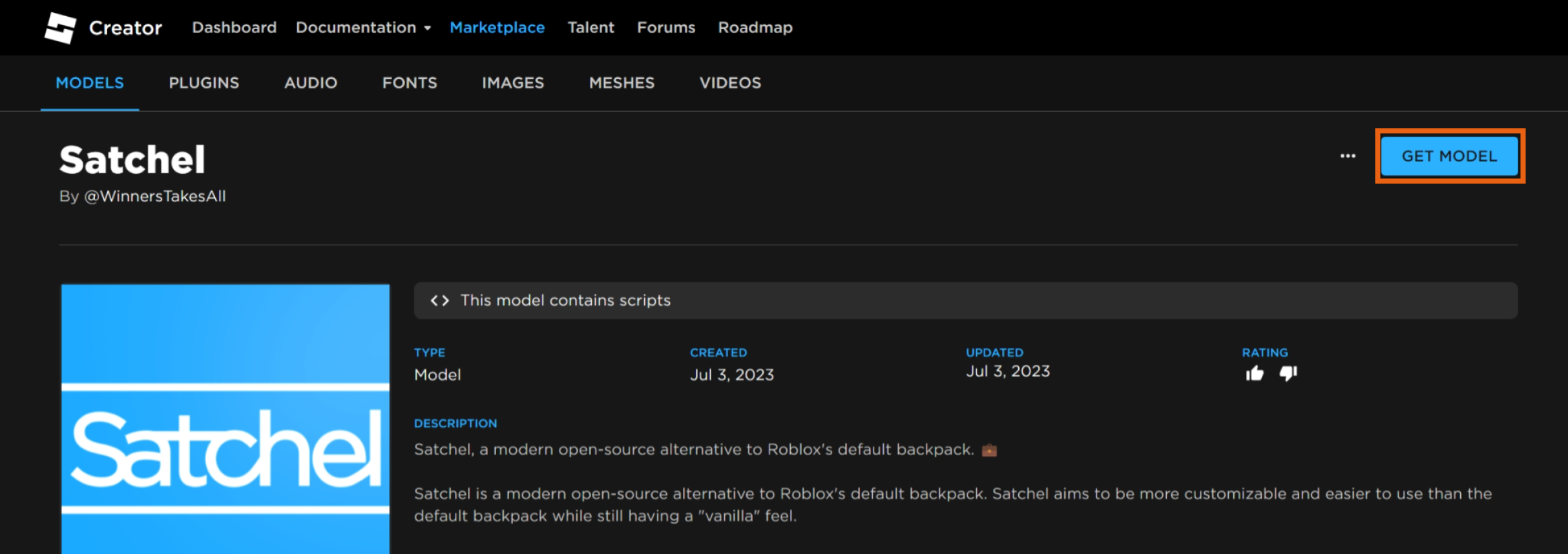
+
+ 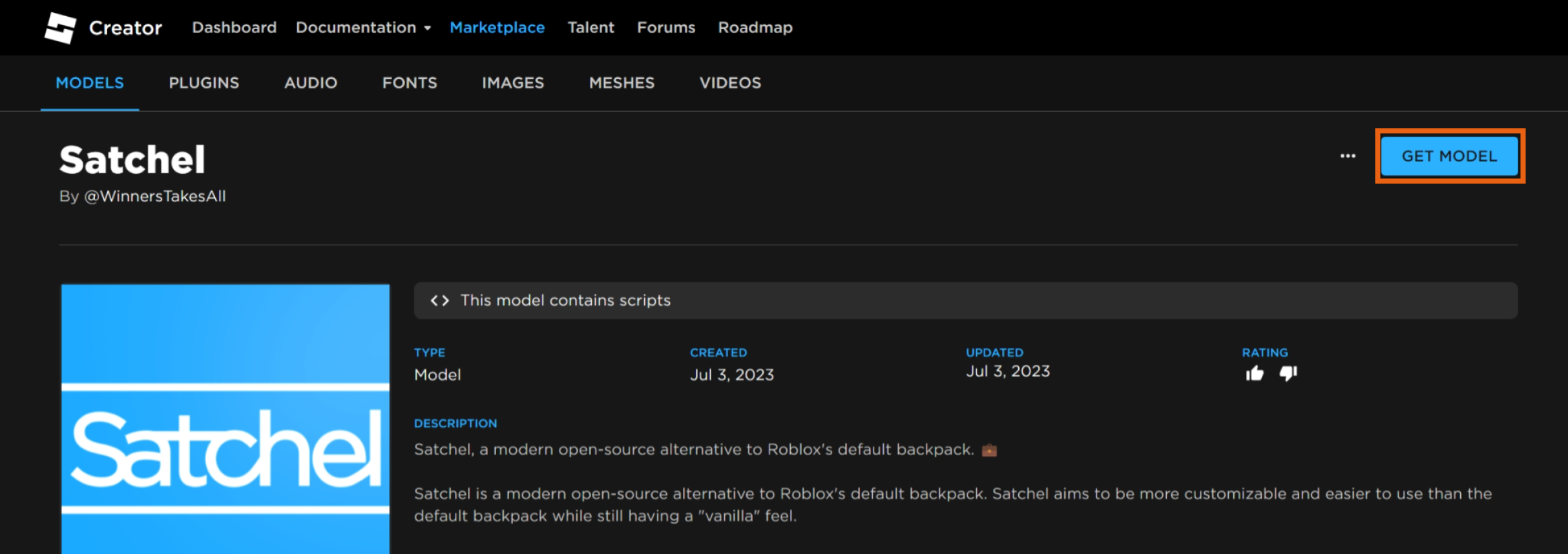{ width="100%" }
2. Open Roblox Studio and create a new place or open an existing place.
3. Open or locate the [Toolbox](https://create.roblox.com/docs/studio/toolbox).
-
+
+ 
4. Open your [Inventory](https://create.roblox.com/docs/studio/toolbox#inventory) from the [Toolbox](https://create.roblox.com/docs/studio/toolbox).
-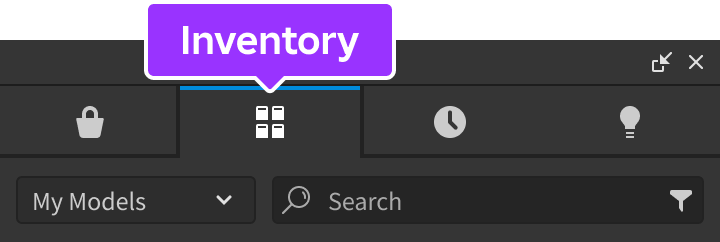
+
+ 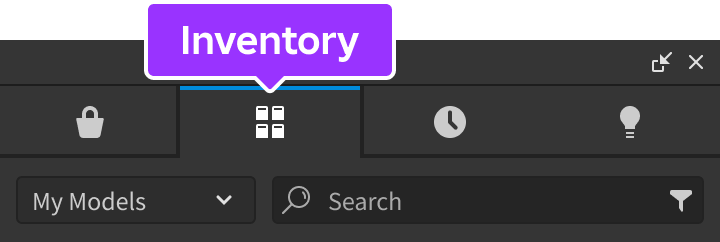{ width="50%" }
5. Search for `Satchel` created by `WinnersTakesAll` and click on it.
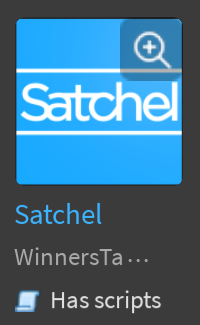
6. Insert `Satchel` into the [Explorer](https://create.roblox.com/docs/studio/explorer) and drag it into [StarterPlayerScripts](https://create.roblox.com/docs/reference/engine/classes/StarterPlayerScripts).
-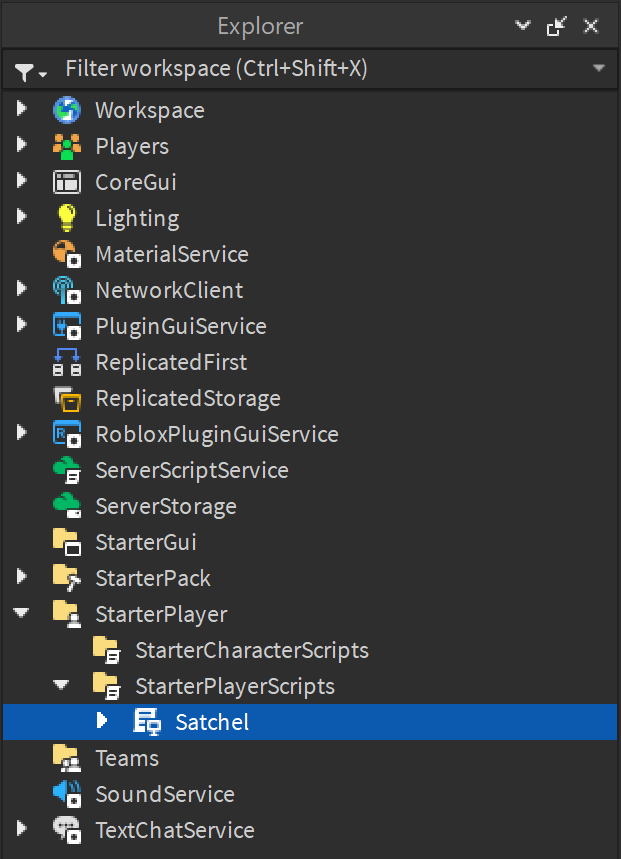
+
+ 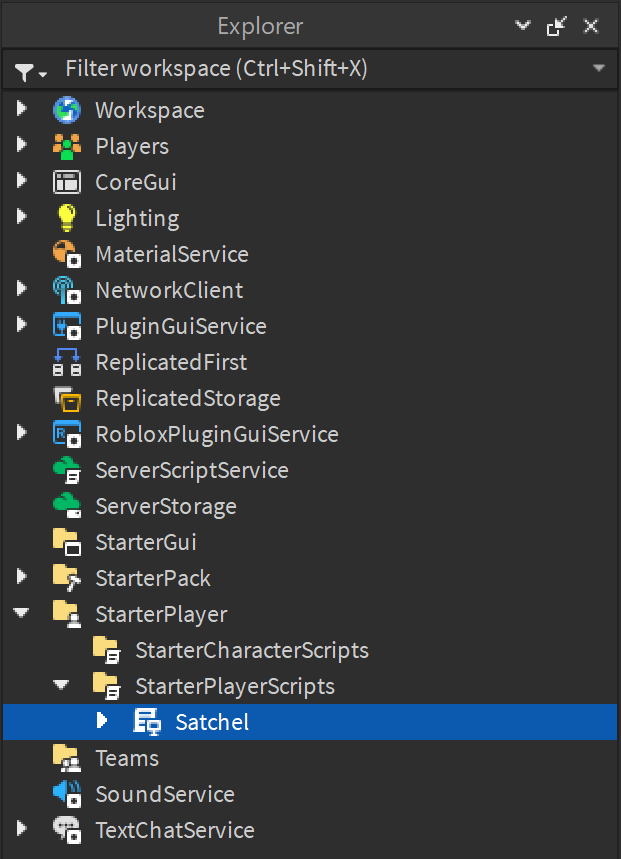{ width="50%" }
### GitHub Releases
1. Download the `Satchel.rbxmx` file from [Releases](https://github.com/RyanLua/Satchel/releases).
-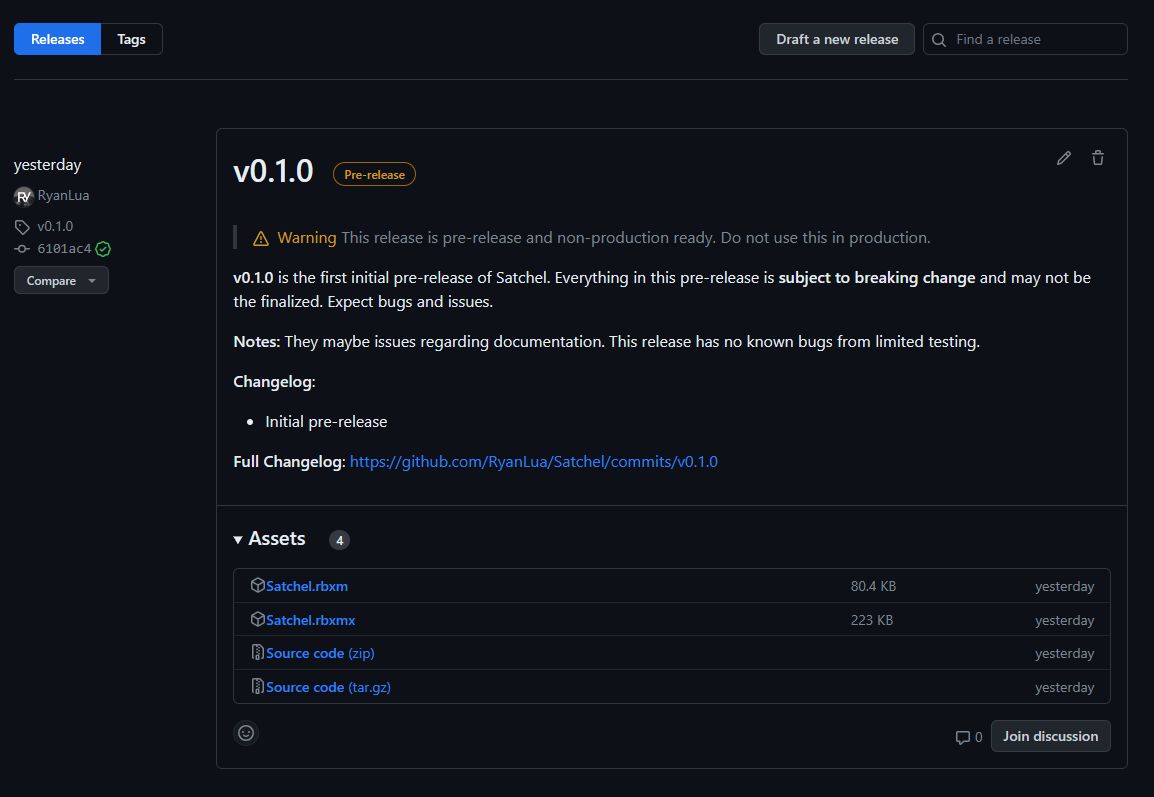
+
+ 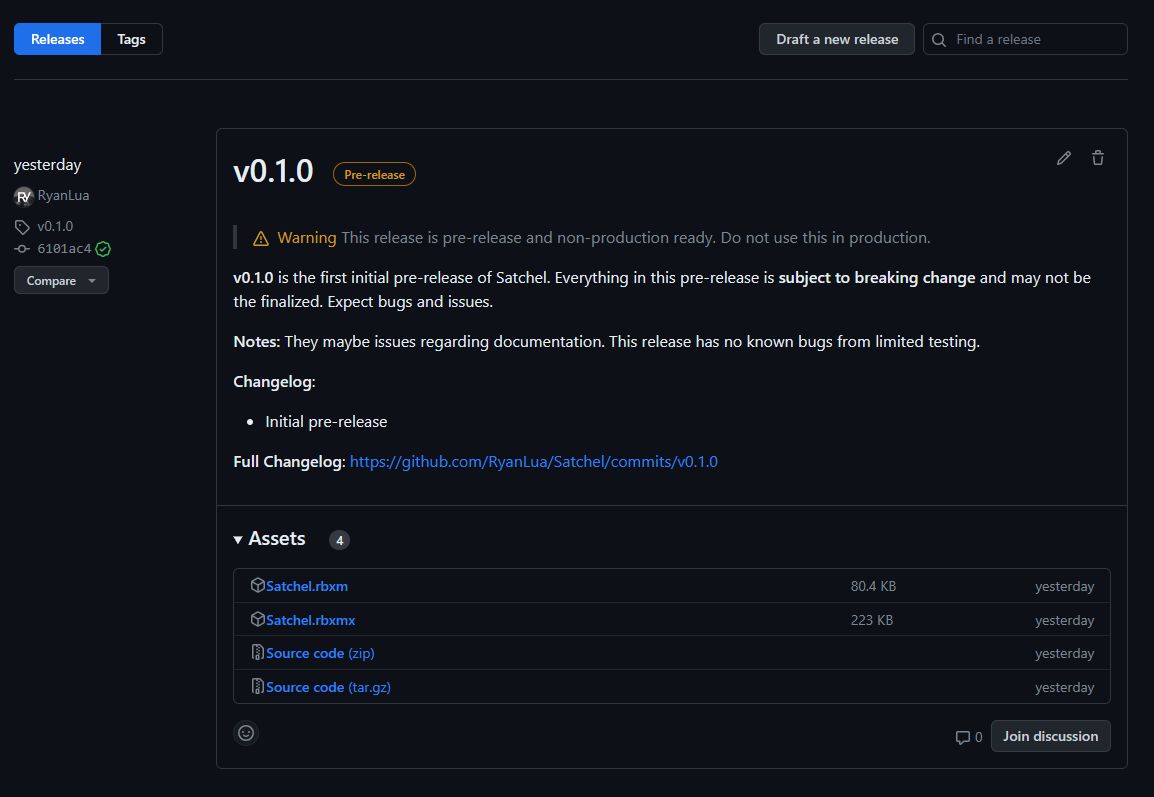{ width="75%" }
2. Open Roblox Studio and create a new place or open an existing place.
3. Go to [Explorer](https://create.roblox.com/docs/studio/explorer) and right-click on [`StarterPlayerScripts`](https://create.roblox.com/docs/reference/engine/classes/StarterPlayerScripts) and click on `Insert from file...`.
-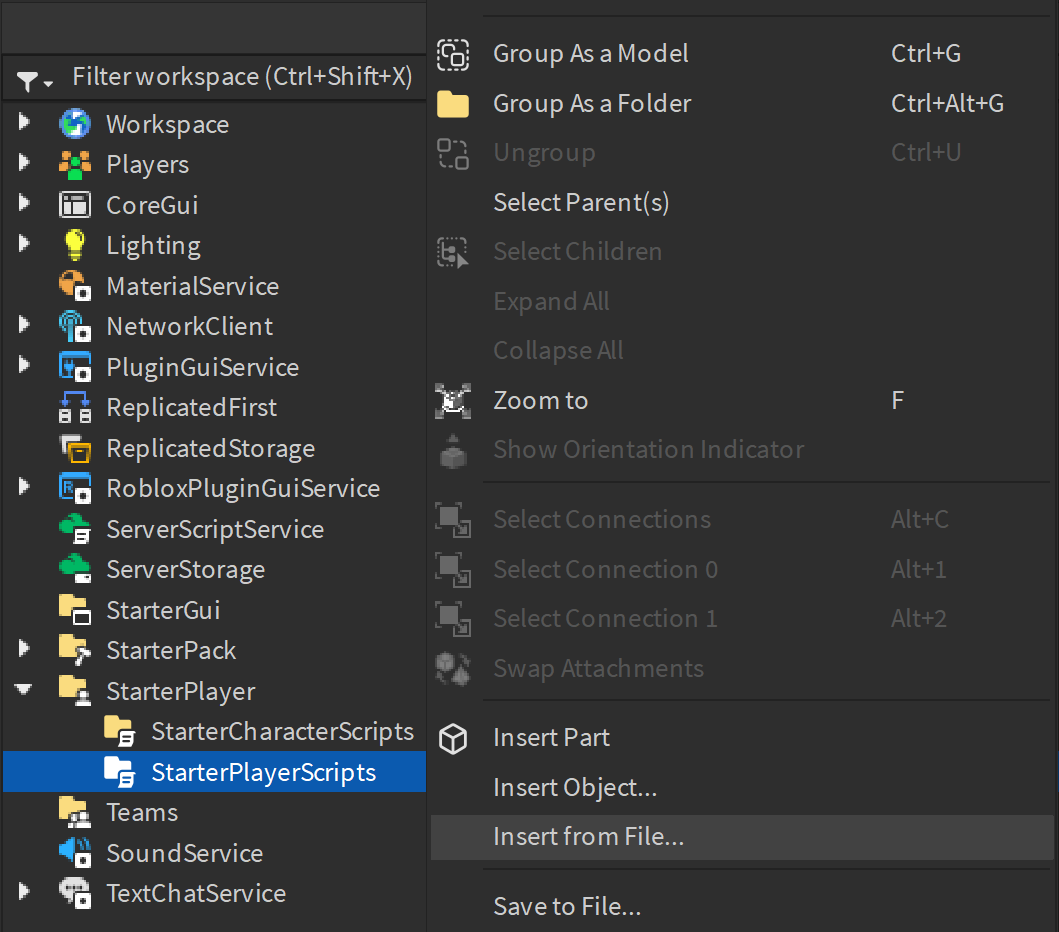
+
+ 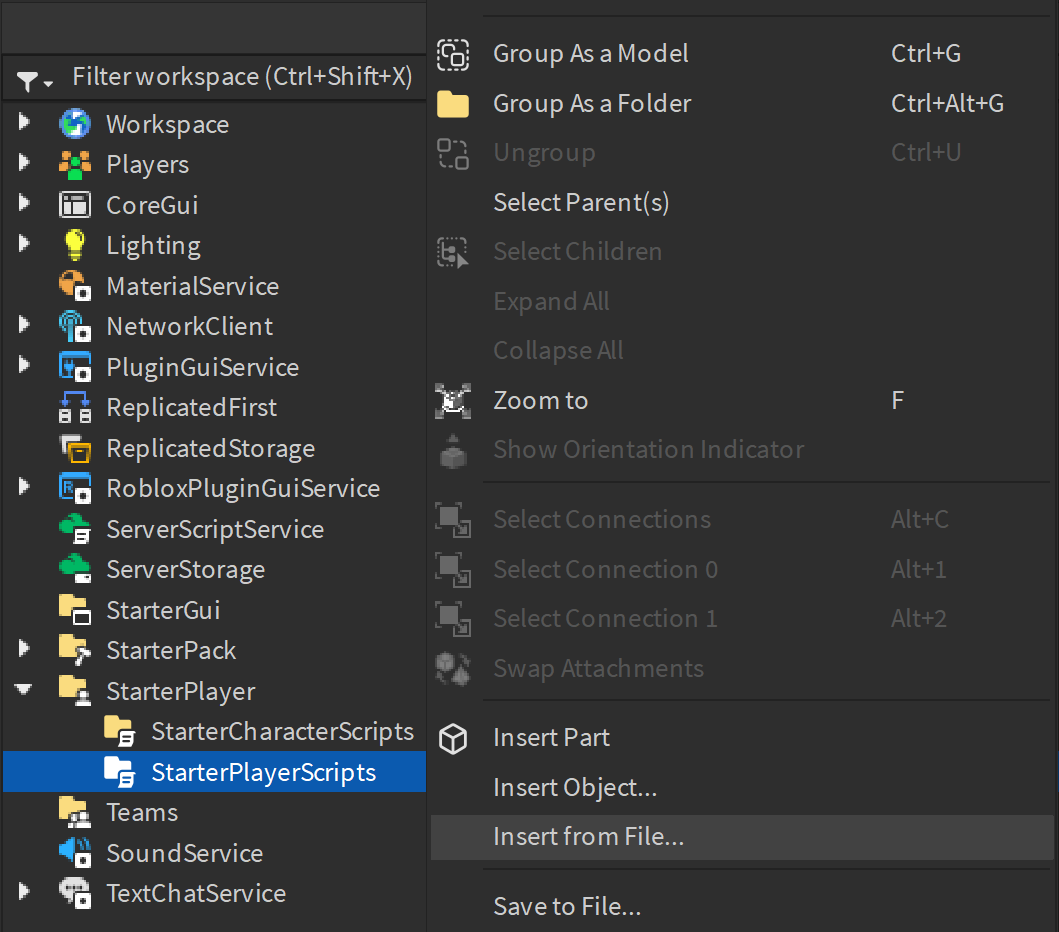{ width="75%" }
4. Select the `Satchel.rbxmx` you downloaded from GitHub and click `Open`.
-
+
+ { width="75%" }
5. Ensure that `Satchel`is in [StarterPlayerScripts](https://create.roblox.com/docs/reference/engine/classes/StarterPlayerScripts).
-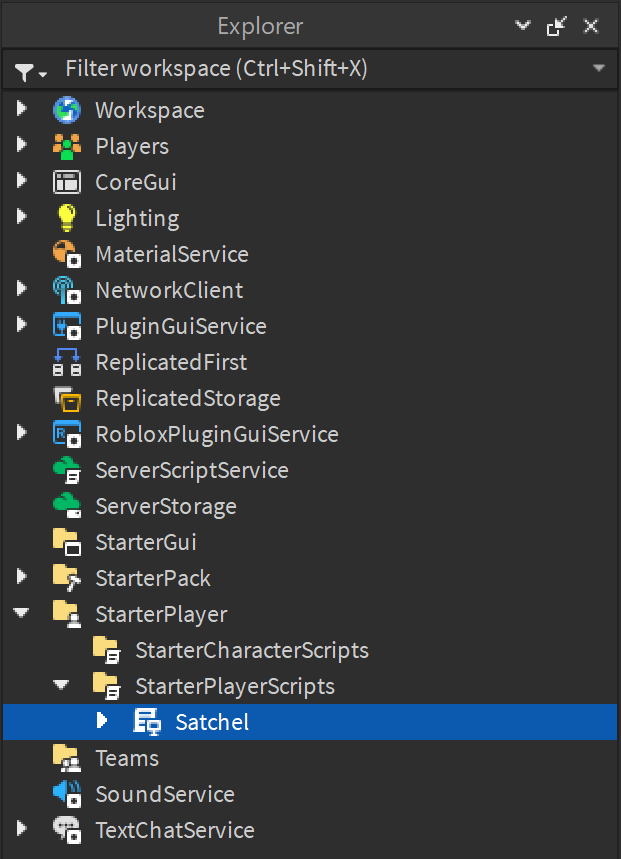
+
+ 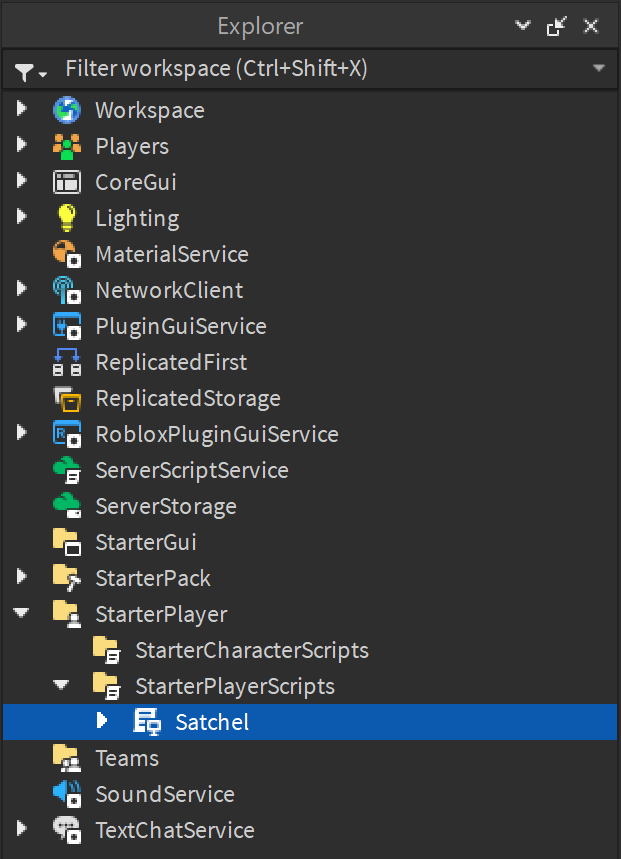{ width="50%" }
### Wally
diff --git a/docs/introduction.md b/docs/introduction.md
index 141549f..0ca1614 100644
--- a/docs/introduction.md
+++ b/docs/introduction.md
@@ -2,11 +2,6 @@
title: Introduction
description: Learn about Satchel and why you should use Satchel over other backpack systems.
icon: material/star-outline
-comments: true
-tags:
- - Getting started
- - First steps
- - About
---
Welcome to Satchel, if you don't know already, Satchel acts as a modern refresh of the default Roblox backpack UI with a lot of improvements in place.
diff --git a/docs/javascripts/tablesort.js b/docs/javascripts/tablesort.js
deleted file mode 100644
index 69c0ff3..0000000
--- a/docs/javascripts/tablesort.js
+++ /dev/null
@@ -1,6 +0,0 @@
-document$.subscribe(function() {
- var tables = document.querySelectorAll("article table:not([class])")
- tables.forEach(function(table) {
- new Tablesort(table)
- })
-})
\ No newline at end of file
diff --git a/docs/logo.png b/docs/logo.png
index 6a33149..fc93cba 100644
Binary files a/docs/logo.png and b/docs/logo.png differ
diff --git a/docs/overrides/main.html b/docs/overrides/main.html
index 4d171c1..e6ebe6b 100644
--- a/docs/overrides/main.html
+++ b/docs/overrides/main.html
@@ -1,11 +1,7 @@
{% extends "base.html" %}
-{% block announce %}
-
-To support Satchel and it's development, please consider becoming a patron. 100% of your donations go towards development and paying for the education of Satchel's maintainers.
-{% endblock %}
-
{% block extrahead %}
+
-
-
-
-{% endif %}
\ No newline at end of file
diff --git a/docs/platforms.md b/docs/platforms.md
index ee8ce1e..3af252d 100644
--- a/docs/platforms.md
+++ b/docs/platforms.md
@@ -2,11 +2,6 @@
title: Platforms
description: Satchel supports all platforms that Roblox supports. Computers, phones, tablets, consoles, and VR are all supported by Satchel right out of the box.
icon: material/devices
-comments: true
-tags:
- - Devices
- - Compatibility
- - Comparison
---
We support all platforms that Roblox supports. Computers, phones, tablets, consoles, and VR are all supported by Satchel right out of the box. Where the default backpack should run, so should Satchel.
diff --git a/docs/support.md b/docs/support.md
new file mode 100644
index 0000000..3515587
--- /dev/null
+++ b/docs/support.md
@@ -0,0 +1,19 @@
+---
+title: Support
+description: Learn how to support Satchel and its development.
+icon: material/heart-outline
+---
+
+Satchel needs your support for its future and development. We distribute Satchel and provide updates for free, for anyone to use or modify. Just know by donating, 100% goes towards the development and paying for the education of the maintainers. So please consider becoming a patron.
+
+By supporting us you will help us to continue to develop and maintain Satchel. We are committed to providing updates and support for Satchel for free, for anyone to use or modify. We are also committed to providing a high-quality product that is easy to use and customizable. Your support will help us to continue to provide updates and support for Satchel.
+
+Support benefits include:
+
+* Early access to new features and updates
+* Access to exclusive content
+* Send us messages for help with Satchel and programming in general
+* Priority support for any issues or questions
+* And more
+
+[Become a Patron](https://patreon.com/RyanLuu){ .md-button }
diff --git a/docs/usage.md b/docs/usage.md
index 5c489e3..f422173 100644
--- a/docs/usage.md
+++ b/docs/usage.md
@@ -2,37 +2,29 @@
title: Usage
description: Use of Satchel very easy. Highly customizable using instance attributes and with scripting support.
icon: material/toolbox-outline
-comments: true
-tags:
- - Overview
- - Customization
- - Scripting
---
Use of Satchel after installation very easy. Just [publish your experience to Roblox](https://create.roblox.com/docs/production/publishing) and see Satchel live in action.
+To learn how to install Satchel, see [Installation](installation.md).
+
!!! note
Please see [API Reference](api-reference.md) for more details on attributes, methods, and events for Satchel and how to use Satchel to it's full potential.
### Customization
-Satchel is highly customizable & adjustable with [instance attributes](https://create.roblox.com/docs/studio/instance-attributes) support allowing you to customize the behavior and appearance of over 10+ attributes. Below see a table containing all the attributes along with a description of what that attribute does.
-
-| Attribute | Description | Default |
-| :--- | :--- | :--- |
-| BackgroundColor3: [`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3) | Determines the background color of the default inventory window and slots. | `[25, 27, 29]` |
-| BackgroundTransparency: [`number`](https://create.roblox.com/docs/scripting/luau/numbers) | Determines the background transparency of the default inventory window and slots. | 0.3 |
-| CornerRadius: [`UDim`](https://create.roblox.com/docs/reference/engine/datatypes/UDim) | Determines the radius, in pixels, of the default inventory window and slots. | `0, 8` |
-| EquipBorderColor3: [`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3) | Determines the color of the equip border when a slot is equipped. | `[255, 255, 255]` |
-| EquipBorderSizePixel: [`number`](https://create.roblox.com/docs/scripting/luau/numbers) | Determines the pixel width of the equip border when a slot is equipped. | `5` |
-| FontFace: [`Font`](https://create.roblox.com/docs/reference/engine/enums/Font) | Determines the font of the default inventory window and slots. | `Gotham SSm` |
-| InsetIconPadding: [`boolean`](https://create.roblox.com/docs/scripting/luau/booleans) | Determines whether or not the tool icon is padded in the default inventory window and slots. | True |
-| OutlineEquipBorder: [`boolean`](https://create.roblox.com/docs/scripting/luau/booleans) | Determines whether or not the equip border is outline or inset when a slot is equipped. | True |
-| TextColor3: [`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3) | Determines the color of the text in default inventory window and slots. | `[255, 255, 255]` |
-| TextSize: [`number`](https://create.roblox.com/docs/scripting/luau/numbers) | Determines the size of the text in the default inventory window and slots. | `14` |
-| TextStrokeColor3: [`Color3`](https://create.roblox.com/docs/reference/engine/datatypes/Color3) | Determines the color of the text stroke of text in default inventory window and slots. | `[0, 0, 0]` |
-| TextStrokeTransparency: [`number`](https://create.roblox.com/docs/scripting/luau/numbers) | Determines the transparency of the text stroke of text in default chat window and slots. | 0.5 |
+Satchel is highly customizable & adjustable with [instance attributes](https://create.roblox.com/docs/studio/instance-attributes) support allowing you to customize the behavior and appearance of over 10+ attributes.
+
+Some of the attributes include:
+
+* Text Color, Size, Stroke Color & Transparency
+* Background Color & Transparency
+* Equip Border Color & Thickness
+* Corner Radius
+* Font
+
+More attributes can be found in the [API Reference](api-reference.md). The list above is not exhaustive and there are may more attributes available for customization.

@@ -41,20 +33,15 @@ Satchel is highly customizable & adjustable with [instance attributes](https://c
### Scripting
-Satchel offers methods and events for scripting purposes. Below see a table with all the methods available.
+Satchel offers methods and events for scripting purposes. In the below code example we will use the `SetBackpackEnabled` method to disable the Satchel. The script expects the Satchel module to be in [`ReplicatedStorage`](https://create.roblox.com/docs/reference/engine/classes/ReplicatedStorage).
-| IsOpened(): [`boolean`](https://create.roblox.com/docs/scripting/luau/booleans) |
-| :--- |
-| Returns whether the inventory is opened or not. |
+``` lua title="LocalScript" linenums="1"
+local ReplicatedStorage = game:GetService("ReplicatedStorage")
+local Satchel = require(ReplicatedStorage:WaitForChild("Satchel"))
-| SetBackpackEnabled(enabled: boolean): `void` |
-| :--- |
-| Sets whether the backpack gui is enabled or disabled. |
+Satchel.SetBackpackEnabled(false) -- (1)!
+```
-| GetBackpackEnabled(): [`boolean`](https://create.roblox.com/docs/scripting/luau/booleans) |
-| :--- |
-| Returns whether the backpack gui is enabled or disabled. |
+1. Disable Satchel using the [SetBackpackEnabled](api-reference.md#setbackpackenabled) method.
-| GetStateChangedEvent(): [`RBXScriptSignal`](https://create.roblox.com/docs/reference/engine/datatypes/RBXScriptSignal) |
-| :--- |
-| Returns a signal that fires when the inventory is opened or closed. |
+For the full API reference, see [API Reference](api-reference.md) for more details on attributes, methods, and events for Satchel and how to use Satchel to it's full potential.
diff --git a/mkdocs.yml b/mkdocs.yml
index 1dc4daf..319895c 100644
--- a/mkdocs.yml
+++ b/mkdocs.yml
@@ -12,60 +12,45 @@ theme:
name: material
custom_dir: docs/overrides
features:
- - announce.dismiss
- content.action.edit
- - content.action.view
- content.code.copy
- content.tooltips
- - header.autohide
- - navigation.instant
- - navigation.instant.progress
- - navigation.prune
+ - content.code.annotate
+ # - navigation.instant
+ # - navigation.instant.progress
- navigation.top
- - navigation.tracking
+ - navigation.tabs
- search.highlight
- - search.share
- search.suggest
- - search.share
- navigation.footer
- toc.follow
palette:
+ - media: "(prefers-color-scheme)"
+ toggle:
+ icon: material/brightness-auto
+ name: Switch to light mode
- media: "(prefers-color-scheme: light)"
- primary: light blue
- accent: light blue
- scheme: default
+ scheme: default
toggle:
icon: material/brightness-7
name: Switch to dark mode
- media: "(prefers-color-scheme: dark)"
- primary: light blue
- accent: light blue
scheme: slate
toggle:
icon: material/brightness-4
- name: Switch to light mode
+ name: Switch to system preference
favicon: favicon.png
logo: logo.png
icon:
repo: fontawesome/brands/github
+ edit: material/pencil
language: en
plugins:
- - glightbox
- search
- - git-authors
- - tags
- - social
- - git-revision-date-localized:
- enable_creation_date: true
- - git-committers:
- repository: RyanLua/Satchel
- branch: main
- token: !!python/object/apply:os.getenv ["MKDOCS_GIT_COMMITTERS_APIKEY"]
-
extra:
- generator: false
+ # generator: false
# version:
# provider: mike
social:
@@ -100,10 +85,6 @@ extra:
Thanks for your feedback! Help us improve this page by
using our feedback form.
-extra_javascript:
- - https://unpkg.com/tablesort@5.3.0/dist/tablesort.min.js
- - javascripts/tablesort.js
-
edit_uri: edit/main/docs/
markdown_extensions:
@@ -122,11 +103,14 @@ markdown_extensions:
emoji_generator: !!python/name:material.extensions.emoji.to_svg
nav:
- - Home: index.md
- - Introduction: introduction.md
- - Installation: installation.md
- - Usage: usage.md
- - Platforms: platforms.md
- - Benchmarks: benchmarks.md
- - Alternatives: alternatives.md
- - API Reference: api-reference.md
+ - Home:
+ - Home: index.md
+ - Introduction: introduction.md
+ - Installation: installation.md
+ - Usage: usage.md
+ - Platforms: platforms.md
+ - Benchmarks: benchmarks.md
+ - Alternatives: alternatives.md
+ - API Reference: api-reference.md
+ - Support: support.md
+ - Contributing: contributing.md