diff --git a/extensions/gitlab/README.md b/extensions/gitlab/README.md
index a5588ace81c6d..7194bc48c6438 100644
--- a/extensions/gitlab/README.md
+++ b/extensions/gitlab/README.md
@@ -1,10 +1,102 @@
-# GitLab integration for Raycast
+
+

-## Prerequisites
+
+ GitLab
+
-call `npm ci`
+Raycast extension to create, search and modify issues, manage merge requests, projects and more.
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+
+## Features
+
+- Manage your issue
+- Manage your assigned issues
+- Manage your GitLab todos
+- Manage your projects
+- Manage your epics
+- Manage your merge requests
+- Manage your reviews
+- Search other users
+
+and many more
+
+## How to get the access token for the GitLab API
+
+- Goto to your GitLab instance e.g. https://gitlab.com
+- Click on your avatar image in the right upper corner
+- Click on `Edit profile`
+- Click on `Access Tokens` on the left sidebar
+- Give your token a name e.g. `raycast` and set an expiration date (highly recommended)
+- Select your scope of choice
+
+ You need at least `read_api`. When you want to make write operation via raycast, you should use `api`
+
+- Store the given access token in a secret box because GitLab want show you the key again
+- Go to the preferences in Raycast (or start any command of GitLab extension)
+- Set your GitLab instance url
+
+ For gitlab.com this would be `https://gitlab.com`.
+ Your own instance could be `https://mygitlab.org`.
+
+- Set the token from the previous step into the `API Token` field
+
+Now you should be able to manage your GitLab instance with Raycast 🚀.
## API Token/Personal Access Token scope
For all read only commands the `read_api` scope is enough. If you want to create/modify e.g. an issue you need
the `api` scope.
+
+## Showcases
+
+### Todos
+
+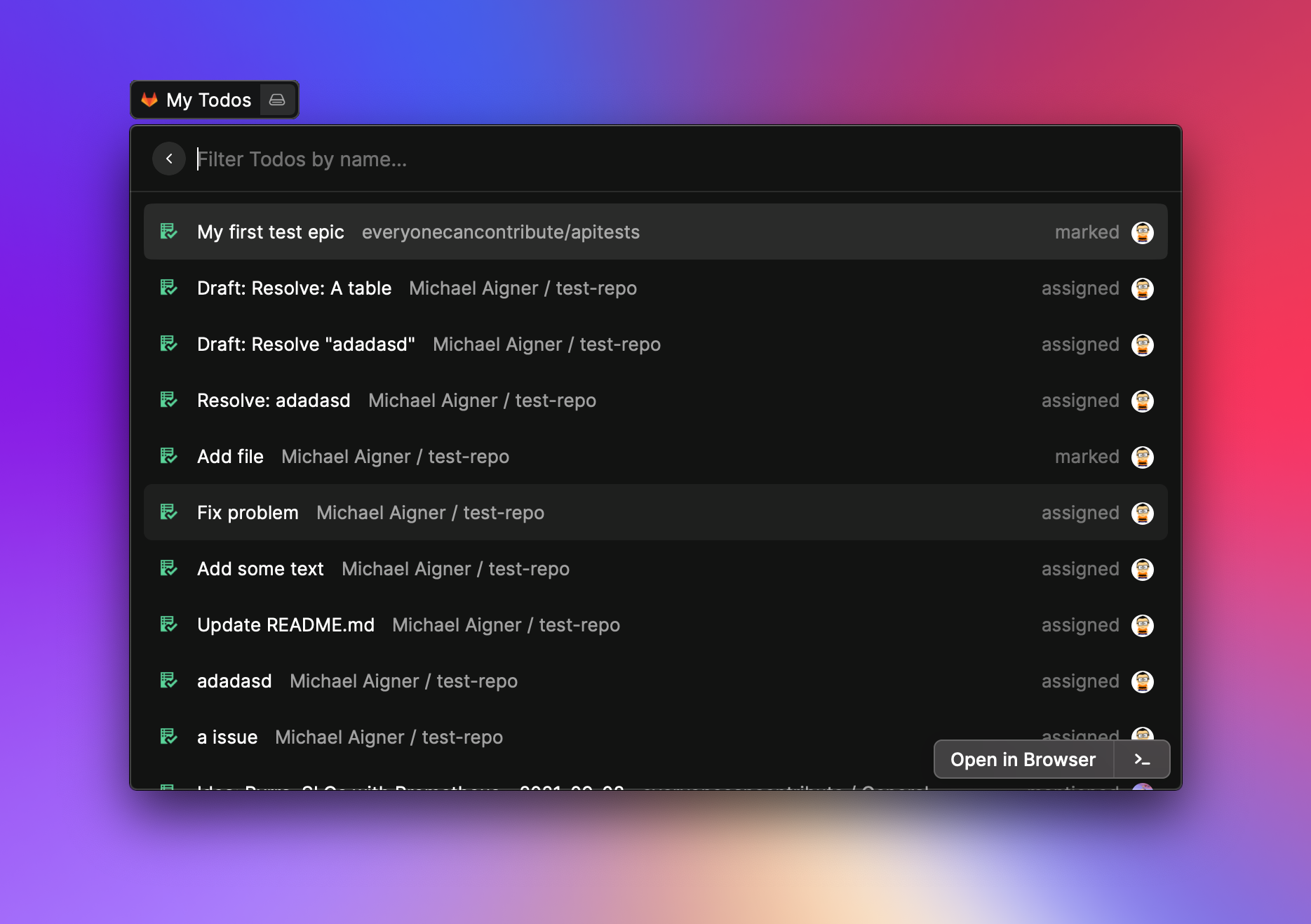
+
+### My Open Issues
+
+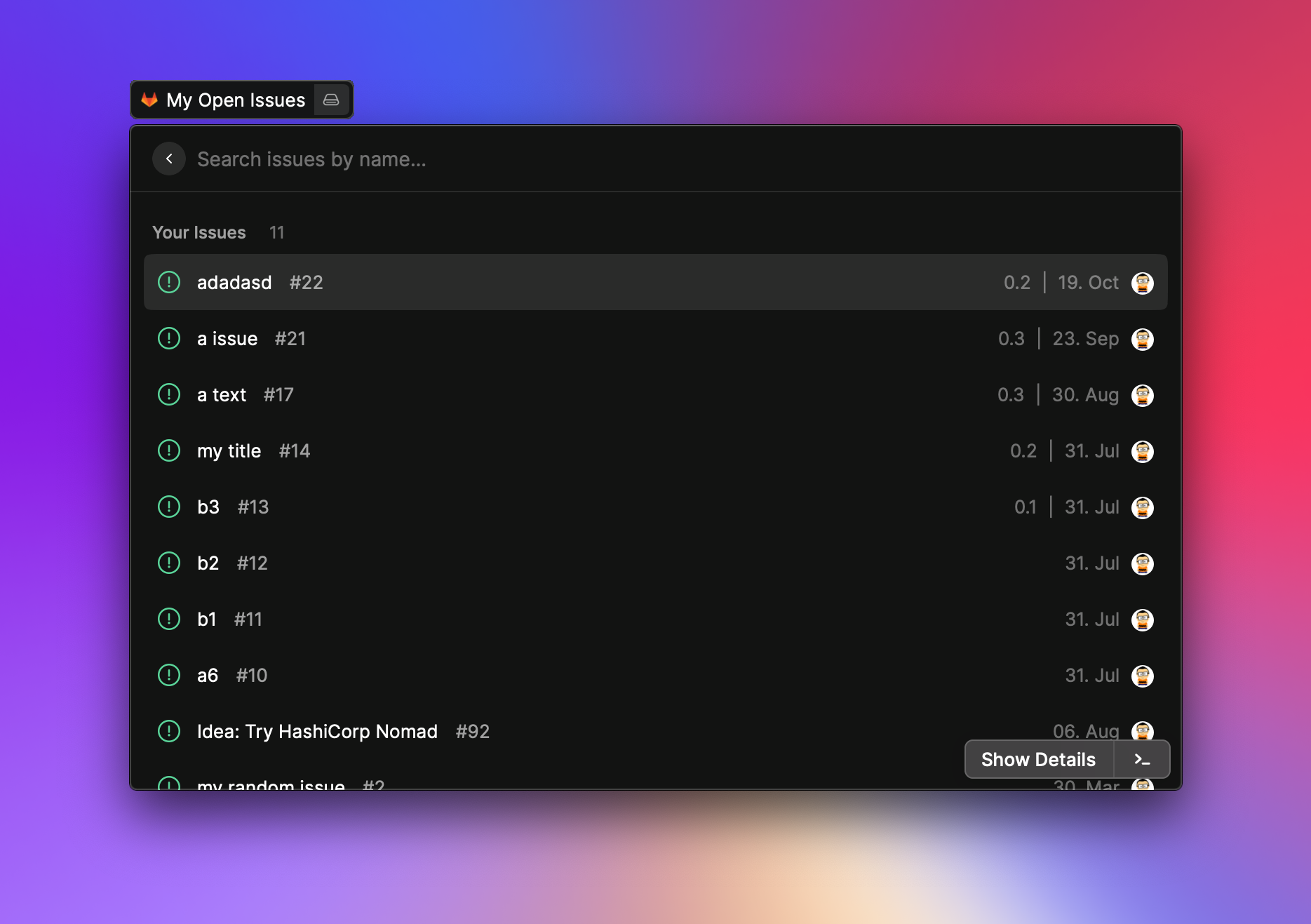
+
+### My Groups
+
+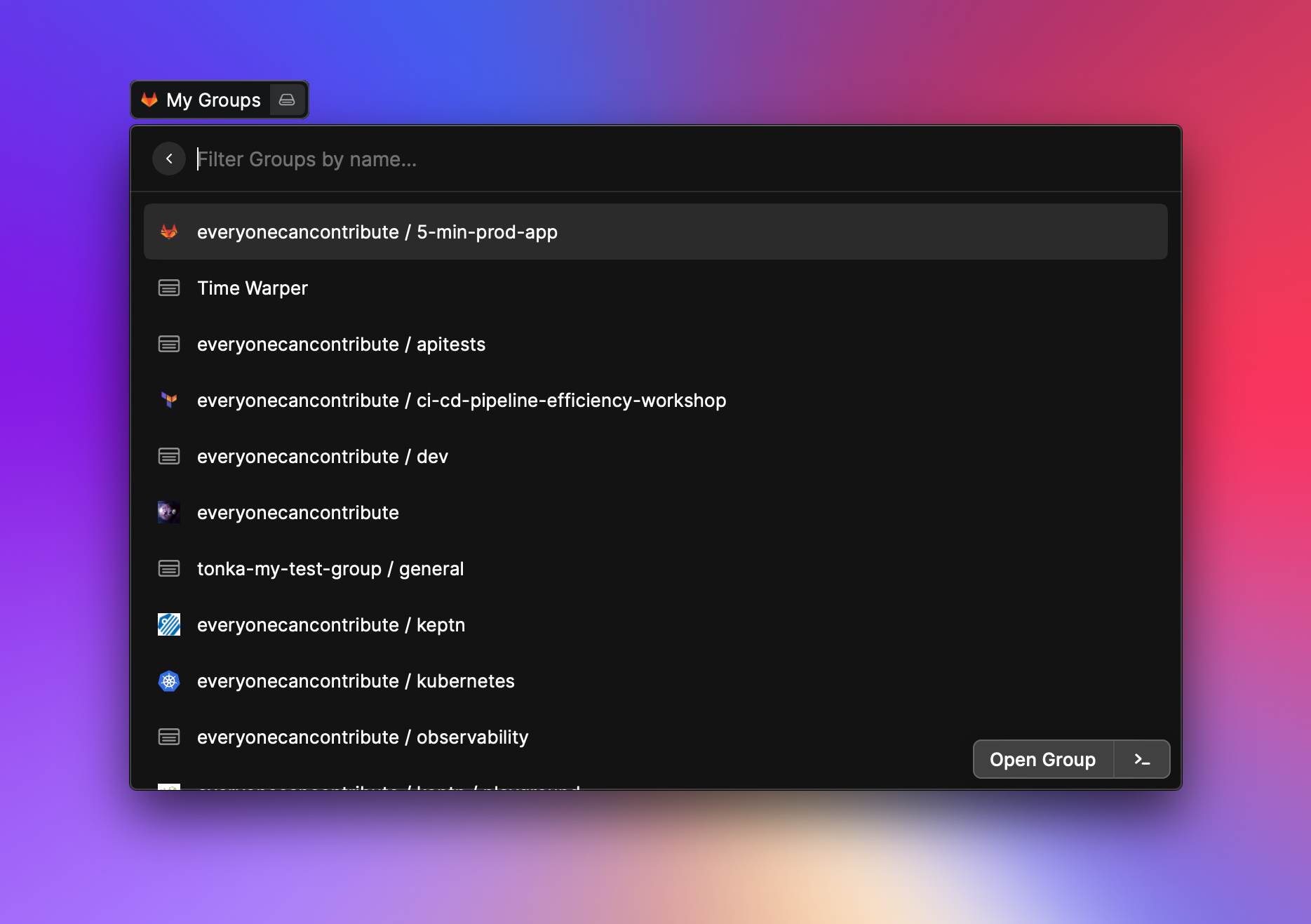
+
+### Epics
+
+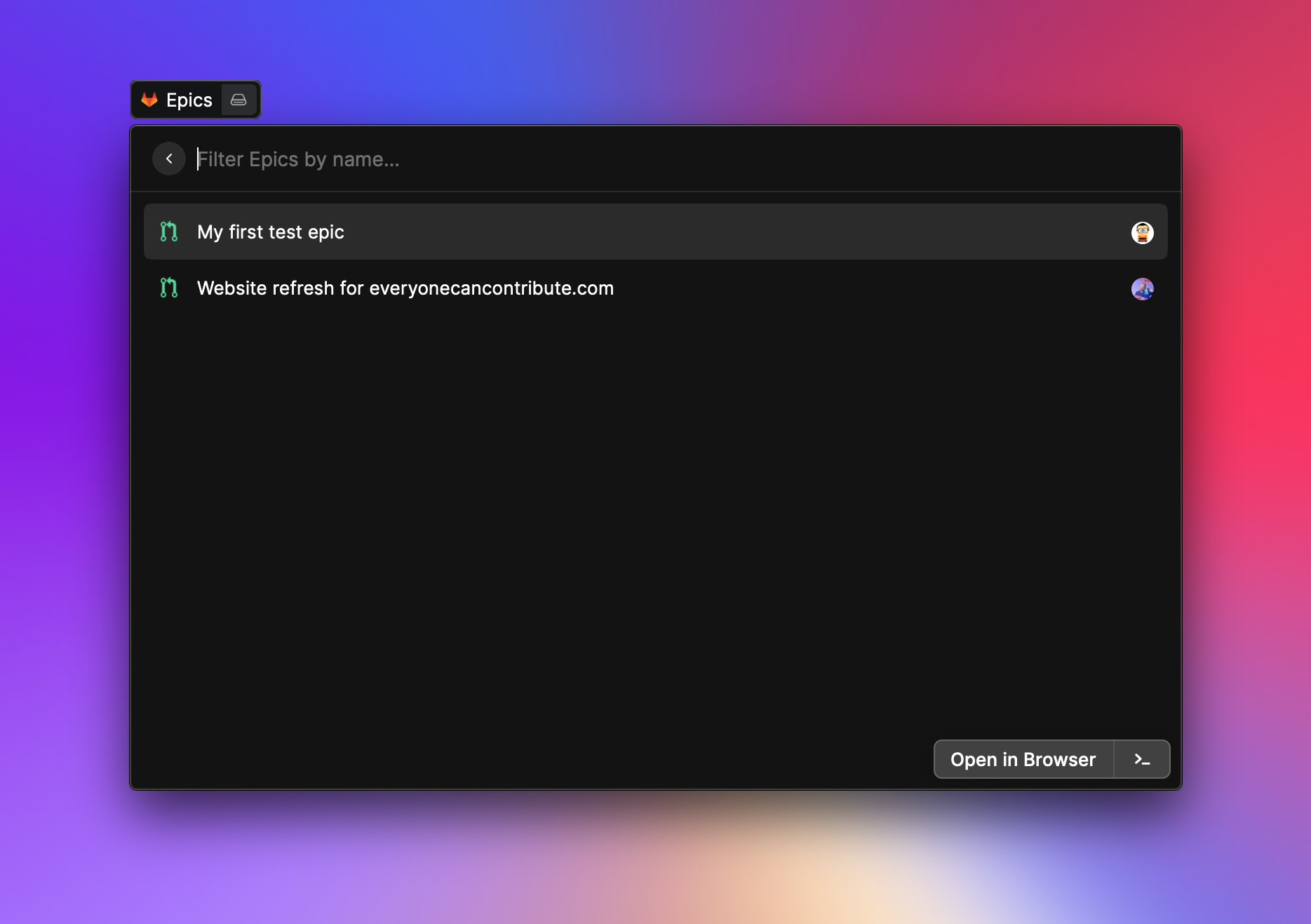
diff --git a/extensions/gitlab/src/components/epic_actions.tsx b/extensions/gitlab/src/components/epic_actions.tsx
new file mode 100644
index 0000000000000..b5ef6001c67b6
--- /dev/null
+++ b/extensions/gitlab/src/components/epic_actions.tsx
@@ -0,0 +1,32 @@
+import { ActionPanel, Color, KeyboardShortcut, showToast, ToastStyle } from "@raycast/api";
+import { gitlab } from "../common";
+import { Epic } from "../gitlabapi";
+import { GitLabIcons } from "../icons";
+
+export function CreateEpicTodoAction(props: { epic: Epic; shortcut?: KeyboardShortcut }) {
+ const epic = props.epic;
+ async function handleAction() {
+ try {
+ await gitlab.post(`groups/${epic.group_id}/epics/${epic.iid}/todo`);
+ showToast(ToastStyle.Success, "To do created");
+ } catch (error: any) {
+ showToast(
+ ToastStyle.Failure,
+ "Failed to add as to do",
+ error instanceof Error ? error.message : error.toString()
+ );
+ }
+ }
+ if (epic.state === "opened") {
+ return (
+
+ );
+ } else {
+ return null;
+ }
+}
diff --git a/extensions/gitlab/src/components/epics.tsx b/extensions/gitlab/src/components/epics.tsx
index 407e08e022886..7fcc5a6974dde 100644
--- a/extensions/gitlab/src/components/epics.tsx
+++ b/extensions/gitlab/src/components/epics.tsx
@@ -15,6 +15,7 @@ import { gitlab } from "../common";
import { Epic, Group, searchData } from "../gitlabapi";
import { GitLabIcons } from "../icons";
import { ClearLocalCacheAction } from "./cache_actions";
+import { CreateEpicTodoAction } from "./epic_actions";
function getIcon(state: string): Image {
if (state == "opened") {
@@ -36,6 +37,7 @@ export function EpicListItem(props: { epic: any }) {
actions={
+
diff --git a/extensions/gitlab/src/components/issue_actions.tsx b/extensions/gitlab/src/components/issue_actions.tsx
index 5f224691a0292..606b5dc0bb1de 100644
--- a/extensions/gitlab/src/components/issue_actions.tsx
+++ b/extensions/gitlab/src/components/issue_actions.tsx
@@ -4,6 +4,7 @@ import {
Color,
CopyToClipboardAction,
Icon,
+ KeyboardShortcut,
PushAction,
showToast,
ToastStyle,
@@ -76,10 +77,39 @@ function ShowIssueLabelsAction(props: { labels: Label[] }) {
);
}
+export function CreateIssueTodoAction(props: { issue: Issue; shortcut?: KeyboardShortcut }) {
+ const issue = props.issue;
+ async function handleAction() {
+ try {
+ await gitlab.post(`projects/${issue.project_id}/issues/${issue.iid}/todo`);
+ showToast(ToastStyle.Success, "To do created");
+ } catch (error: any) {
+ showToast(
+ ToastStyle.Failure,
+ "Failed to add as to do",
+ error instanceof Error ? error.message : error.toString()
+ );
+ }
+ }
+ if (issue.state === "opened") {
+ return (
+
+ );
+ } else {
+ return null;
+ }
+}
+
export function IssueItemActions(props: { issue: Issue }) {
const issue = props.issue;
return (
+
{issue.state == "opened" && }
{issue.state == "opened" && }
diff --git a/extensions/gitlab/src/components/todo.tsx b/extensions/gitlab/src/components/todo.tsx
index 6cd0cb50c5189..efb887630832e 100644
--- a/extensions/gitlab/src/components/todo.tsx
+++ b/extensions/gitlab/src/components/todo.tsx
@@ -53,11 +53,12 @@ export function TodoList() {
export function TodoListItem(props: { todo: Todo }) {
const todo = props.todo;
+ const subtitle = todo.group ? todo.group.full_path : todo.project_with_namespace || "";
return (