diff --git a/packages/react/function/README.md b/packages/react/function/README.md
index 4f16ada..02b409d 100644
--- a/packages/react/function/README.md
+++ b/packages/react/function/README.md
@@ -1,8 +1,15 @@
-# @ibrahimstudio/function
+
+
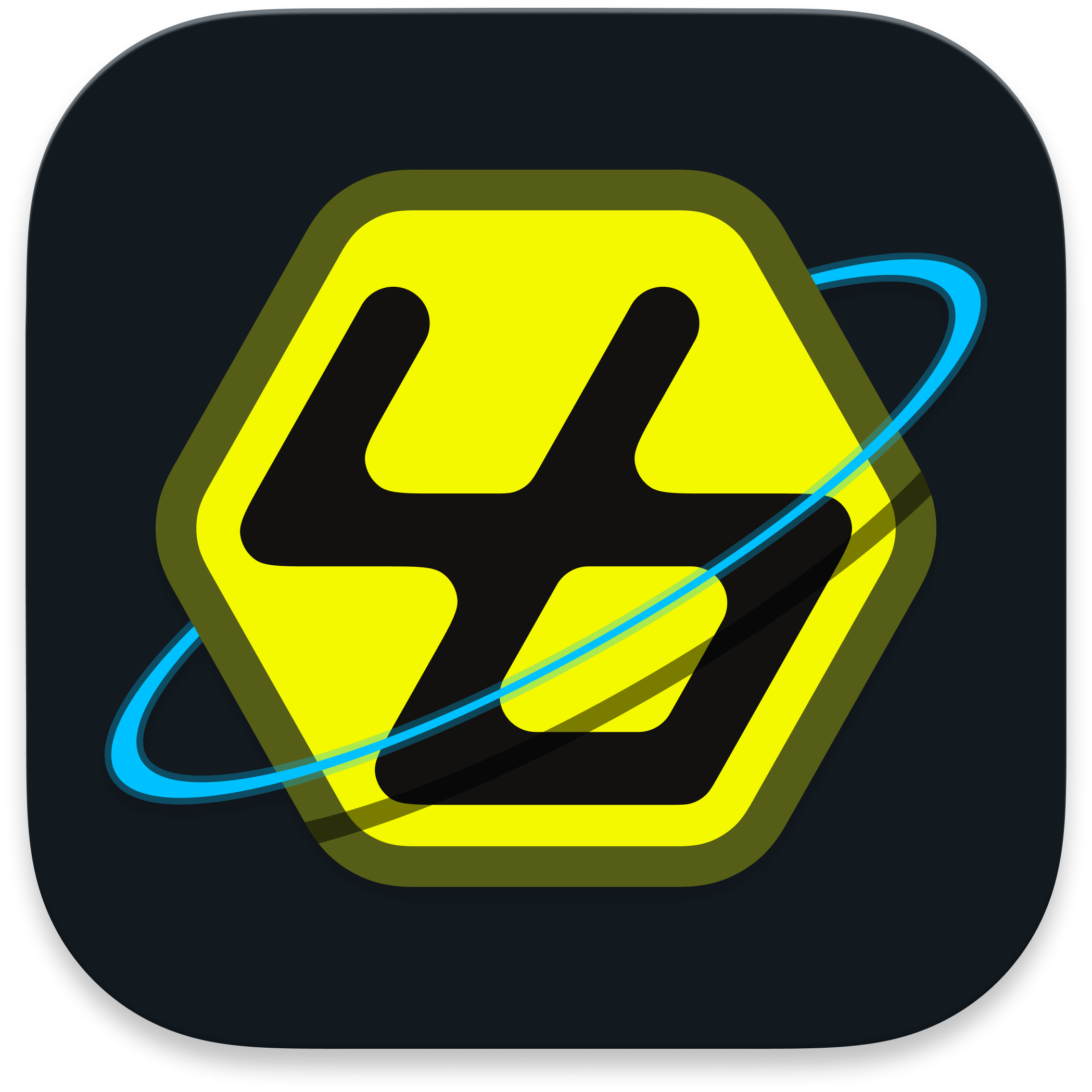
+
+
@ibrahimstudio/function
+
by: Ibrahim Space Studio
+
This package provides useful JavaScript functions for handling events and string manipulations.
+
+
-This package provides useful JavaScript functions for handling events and string manipulations.
+---
-## Installation
+## 1. Installation
You can install this package via npm:
@@ -12,11 +19,11 @@ npm i @ibrahimstudio/function
yarn add @ibrahimstudio/function
```
-## Usage
+## 2. Usage
-### 1. `scrollView`
+### 2.1. `scrollView` function
-The `scrollView` function allows you to smoothly scroll to a specific element on the page.
+A lightweight utility function to smoothly scroll to a specified element on the page.
```javascript
import { scrollView } from "@ibrahimstudio/function";
@@ -36,20 +43,11 @@ const Homepage = () => {
};
```
-### scrollView Props
-
-| Attribute | Type | Description | Default |
-| --------- | ------------------- | ---------------------------------------------------- | ------- |
-| `offset` | _number_ (required) | The offset from the top of the element to scroll to. | - |
-| `id` | _string_ (required) | The ID of the element to scroll to. | - |
-
-> Note: If the `offset` has no value, fill it with `0`
-
---
-### 2. `toTitleCase`
+### 2.2. `toTitleCase` function
-The `toTitleCase` function converts a string to title case.
+A simple npm package that converts a string to title case.
```javascript
import { toTitleCase } from "@ibrahimstudio/function";
@@ -59,14 +57,49 @@ const title = toTitleCase("hello world");
console.log(title); // Output: "Hello World"
```
-### toTitleCase Props
+---
+
+### 2.3. `formatDate` function
+
+A simple npm package for formatting dates using the Intl.DateTimeFormat API.
-| Attribute | Type | Description | Default |
-| --------- | ------------------- | ------------------------------------ | ------- |
-| `str` | _string_ (required) | The string to convert to title Case. | - |
+```javascript
+import { formatDate } from "@ibrahimstudio/function";
+
+// Format a date string
+const formattedDate = formatDate("2024-04-20T12:00:00", "en-US");
+console.log(formattedDate);
+// Output: April 20, 2024, 12:00 PM
+```
+
+## 3. API
+
+### 3.1. `scrollView` Props
+
+| Attribute | Type | Description | Default |
+| --------- | ------------------- | -------------------------------------------------------- | ------- |
+| `offset` | _number_ (required) | The offset in pixels from the top of the target element. | - |
+| `id` | _string_ (required) | The id of the target element to scroll to. | - |
+
+> Note: If the `offset` has no value, fill it with `0`
---
+### 3.2. `toTitleCase` Props
+
+| Attribute | Type | Description | Default |
+| --------- | ------------------- | ----------------------------------------------- | ------- |
+| `str` | _string_ (required) | The input string to be converted to title case. | - |
+
+---
+
+### 3.3. `formatDate` Props
+
+| Attribute | Type | Description | Default |
+| ------------ | ------------------- | --------------------------------- | ------- |
+| `dateString` | _string_ (required) | A string representing the date. | - |
+| `locale` | _string_ | The locale to use for formatting. | _en-US_ |
+
## Contributing
Contributions are welcome! If you have any improvements, bug fixes, or features, feel free to open an issue or create a pull request on GitHub.
diff --git a/packages/react/function/__tests__/function.test.ts b/packages/react/function/__tests__/function.test.ts
index ef2c87d..1c99e3e 100644
--- a/packages/react/function/__tests__/function.test.ts
+++ b/packages/react/function/__tests__/function.test.ts
@@ -1,4 +1,5 @@
import { scrollView, toTitleCase, formatDate } from "../src/functions";
+import "@testing-library/jest-dom";
describe("scrollView", () => {
// Mocking document and window for testing
diff --git a/packages/react/function/package.json b/packages/react/function/package.json
index ad76ae6..da6ad87 100644
--- a/packages/react/function/package.json
+++ b/packages/react/function/package.json
@@ -1,34 +1,15 @@
{
"name": "@ibrahimstudio/function",
- "version": "1.0.4",
+ "version": "1.0.6",
"description": "This function package provides useful functions for handling common tasks in React applications.",
"main": "dist/index.js",
"module": "dist/index.es.js",
"types": "dist/index.d.ts",
"sideEffects": false,
"scripts": {
- "build": "npm run clean && tsc",
+ "build": "npm run clean && rollup -c",
"clean": "rimraf dist",
- "prepublishOnly": "npm run build",
- "test": "jest"
- },
- "jest": {
- "moduleFileExtensions": [
- "ts",
- "tsx",
- "js",
- "jsx",
- "json",
- "node"
- ],
- "transform": {
- "^.+\\.(ts|tsx)$": "ts-jest",
- "^.+\\.css$": "jest-css-modules-transform"
- },
- "testMatch": [
- "**/__tests__/**/*.+(ts|tsx|js|jsx)",
- "**/?(*.)+(spec|test).+(ts|tsx|js|jsx)"
- ]
+ "prepublishOnly": "npm run build"
},
"keywords": [
"function",
diff --git a/packages/react/function/src/functions.ts b/packages/react/function/src/functions.ts
index 8ea929d..1cbb524 100644
--- a/packages/react/function/src/functions.ts
+++ b/packages/react/function/src/functions.ts
@@ -1,4 +1,4 @@
-export function scrollView(offset: number, id: string): void {
+function scrollView(offset: number, id: string): void {
const element = document.querySelector(`[id="${id}"]`) as HTMLElement | null;
if (element) {
const yOffset: number = offset;
@@ -10,16 +10,13 @@ export function scrollView(offset: number, id: string): void {
}
}
-export function toTitleCase(str: string): string {
+function toTitleCase(str: string): string {
return str.replace(/\w\S*/g, function (txt: string): string {
return txt.charAt(0).toUpperCase() + txt.substr(1).toLowerCase();
});
}
-export function formatDate(
- dateString: string,
- locale: string = "en-US"
-): string {
+function formatDate(dateString: string, locale: string = "en-US"): string {
const date = new Date(dateString);
const options: Intl.DateTimeFormatOptions = {
day: "numeric",
@@ -30,3 +27,5 @@ export function formatDate(
};
return date.toLocaleDateString(locale, options);
}
+
+export { scrollView, toTitleCase, formatDate };