diff --git a/.gitignore b/.gitignore index a1b8abe..725d7c0 100644 --- a/.gitignore +++ b/.gitignore @@ -1,3 +1,4 @@ -.DS_Store -*/.DS_Store -**/node_modules \ No newline at end of file +.DS_Store +*/.DS_Store +**/node_modules +.idea \ No newline at end of file diff --git a/1/contract_structure.md b/1/contract_structure.md index 90984a7..5236004 100644 --- a/1/contract_structure.md +++ b/1/contract_structure.md @@ -11,7 +11,7 @@ one contract. Vyper supports a version pragma to ensure that a contract is only compiled by the intended compiler version, or range of versions. Version -strings use [NPM](https://docs.npmjs.com/misc/semver) style syntax. +strings use [NPM](https://docs.npmjs.com/about-semantic-versioning) style syntax. For the scope of this tutorial, we'll want to compile our smart contracts with any compiler version in the range of `0.2.0` (inclusive) to `0.3.0` (exclusive). It looks like this: diff --git a/1/state_vars_and_ints.md b/1/state_vars_and_ints.md index 44ce8fa..a97e7a9 100644 --- a/1/state_vars_and_ints.md +++ b/1/state_vars_and_ints.md @@ -13,7 +13,7 @@ State variables are permanently stored in contract storage. This means they're w storedData: int128 ``` -In this example contract, we created a [`int128`](https://vyper.readthedocs.io/en/stable/types.html#signed-integer-128-bit) called `storedData` which holds a _default_ value of `1`. +In this example contract, we created a [`int128`](https://docs.vyperlang.org/en/stable/types.html#signed-integer-n-bit) called `storedData` which holds a _default_ value of `1`. ## Unsigned Integers: `uint256` diff --git a/2/assert.md b/2/assert.md index a73e7bd..c539b0b 100644 --- a/2/assert.md +++ b/2/assert.md @@ -38,7 +38,7 @@ To select a specific `Pokemon` of a trainer we need 2 things: - A `uint256` index that will choose a specific pokemon of the trainer. -You may recall from [Chapter 2](/#/2/msg-sender) that you can get the address of the contract caller using `msg.sender`. +You may recall from [Chapter 2](/2/msg-sender) that you can get the address of the contract caller using `msg.sender`. 1. Create an `external` function named `battleWildPokemon` which takes a single parameter: `pokemonIndex` of `uint256` type. diff --git a/2/calling_a_contract.md b/2/calling_a_contract.md index 989ab87..e9a66c2 100644 --- a/2/calling_a_contract.md +++ b/2/calling_a_contract.md @@ -1,14 +1,14 @@ # Chapter 10: Calling a Contract -In [Chapter 8](/#/2/interfaces), we added `WildPokemons` interface in the trainer contract. In this chapter, we will call the `battle` function of the `WildPokemon` interface. +In [Chapter 8](/2/interfaces), we added `WildPokemons` interface in the trainer contract. In this chapter, we will call the `battle` function of the `WildPokemon` interface. -If you recall from [Chapter 8](/#/2/interfaces), we need a contract address to interact with `WildPokemons`. +If you recall from [Chapter 8](/2/interfaces), we need a contract address to interact with `WildPokemons`. We will learn how to deploy a contract to Ethereum blockchain and get the contract address in future lessons. For the purpose of this chapter, I have deployed the [`WildPokemons` contract to the Rinkeby Testnet](https://rinkeby.etherscan.io/address/0x66f4804E06007630e1aF0a7B0b279e6F27A3FdE5) Here is the contract address: [0x66f4804E06007630e1aF0a7B0b279e6F27A3FdE5](https://rinkeby.etherscan.io/address/0x66f4804E06007630e1aF0a7B0b279e6F27A3FdE5) -Using the contract address and the interface, you can make external calls to the interface functions (which was discussed in depth in [Chapter 8](/#/2/interfaces)): +Using the contract address and the interface, you can make external calls to the interface functions (which was discussed in depth in [Chapter 8](/2/interfaces)): ```vyper interface Car: diff --git a/2/interfaces.md b/2/interfaces.md index 97b20a8..7b92afd 100644 --- a/2/interfaces.md +++ b/2/interfaces.md @@ -12,7 +12,7 @@ So, for our use-case, we need to create an interface for the pokemon battle cont ### Using Interfaces -Interfaces can be added to contracts either through inline definition or by [importing them from a separate file](https://vyper.readthedocs.io/en/stable/interfaces.html?highlight=import#imports-via-import). +Interfaces can be added to contracts either through inline definition or by [importing them from a separate file](https://docs.vyperlang.org/en/stable/interfaces.html?highlight=import#imports-via-import). The `interface` keyword is used to define an inline external interface: @@ -65,7 +65,7 @@ def test(some_address: address): ## Put it to the test -As the coding area can only have one file at a time, we have removed the pokemon battle contract and added the trainer contract. You can check out the pokemon battle contract [here](https://github.com/vyperlang/vyper.fun/blob/chapter1/assets/2/2.7-finished-code.vy). +As the coding area can only have one file at a time, we have removed the pokemon battle contract and added the trainer contract. You can check out the pokemon battle contract [here](https://github.com/vyperlang/learn/blob/chapter1/assets/2/2.7-finished-code.vy). 1. The pokemon battle contract only has 1 external function: `battle` diff --git a/2/msg-sender.md b/2/msg-sender.md index 7490701..2a84b3b 100644 --- a/2/msg-sender.md +++ b/2/msg-sender.md @@ -6,7 +6,7 @@ In order to do this, we need to use something called `msg.sender`. ## msg.sender -In Vyper, there are certain global variables that are available to all functions. One of these is `msg.sender`, which refers to the address of the person (or smart contract) who called the current function. +In Vyper, there are certain [global variables](https://docs.vyperlang.org/en/stable/constants-and-vars.html#environment-variables) that are available to all functions. One of these is `msg.sender`, which refers to the address of the person (or smart contract) who called the current function. > Note: In Vyper, function execution always needs to start with an external caller. A contract will just sit on the blockchain doing nothing until someone calls one of its functions. So there will always be a `msg.sender`. diff --git a/2/wild_pokemons.md b/2/wild_pokemons.md index a2987b9..442883e 100644 --- a/2/wild_pokemons.md +++ b/2/wild_pokemons.md @@ -17,8 +17,8 @@ We will re-use some parts of our previous contract. Now, let's add some state va 4. Make following changes to the `_generateRandomDNA` function: - Remove the `_name` input parameter so that `_generateRandomDNA` accepts no parameter. - - In the `_generateRandomDNA` body, replace `_name` with `battleCount`. Now, you may remember from [Lesson 1, Chapter 11](/#/1/keccak256-and-typecasting), that `keccak256` expects a single parameter of type `bytes32`, `Bytes` or `String`. So, we need to use `convert` to typecast `battleCount` to `bytes32`. - - You may remember from [Lesson 1, Chapter 10](/#/1/more_on_functions) that we add `@pure` decorator to a function which does not read contract state or environment variables. But now as `_generateRandomDNA` is accessing `battleCount` (a state variable), it is no longer a `@pure` function. So, remove the `@pure` function decorator. + - In the `_generateRandomDNA` body, replace `_name` with `battleCount`. Now, you may remember from [Lesson 1, Chapter 11](/1/keccak256-and-typecasting), that `keccak256` expects a single parameter of type `bytes32`, `Bytes` or `String`. So, we need to use `convert` to typecast `battleCount` to `bytes32`. + - You may remember from [Lesson 1, Chapter 10](/1/more_on_functions) that we add `@pure` decorator to a function which does not read contract state or environment variables. But now as `_generateRandomDNA` is accessing `battleCount` (a state variable), it is no longer a `@pure` function. So, remove the `@pure` function decorator. diff --git a/CONTRIBUTION.md b/CONTRIBUTION.md index 1b6be5e..1f22072 100644 --- a/CONTRIBUTION.md +++ b/CONTRIBUTION.md @@ -40,7 +40,7 @@ Click the "pencil" icon on the top right to edit the file. 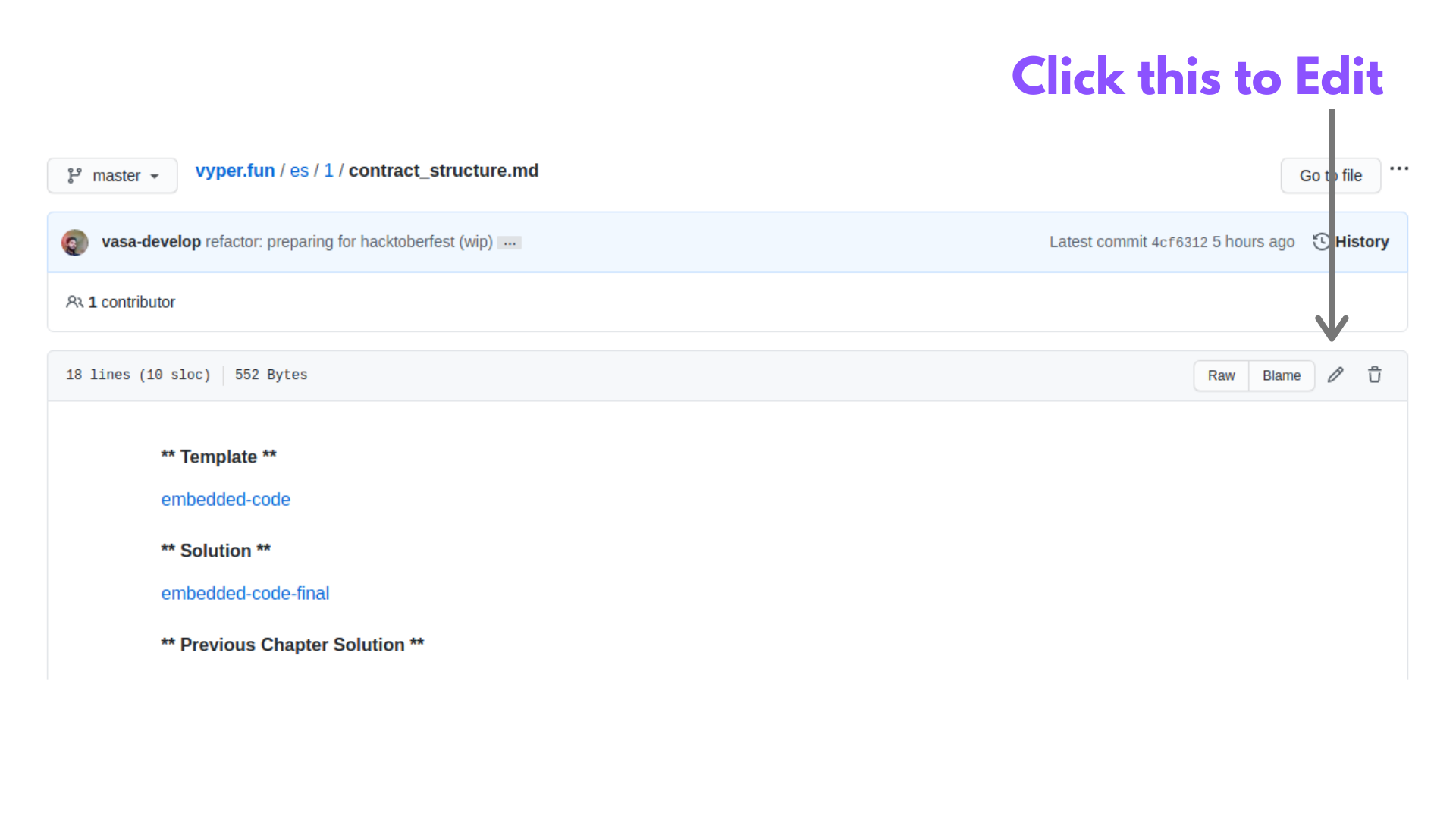 -In the first line of the file, you will find a link. In this case you will find the following link: https://vyper.fun/#/1/contract_structure +In the first line of the file, you will find a link. In this case you will find the following link: https://learn.vyperlang.org/#/1/contract_structure 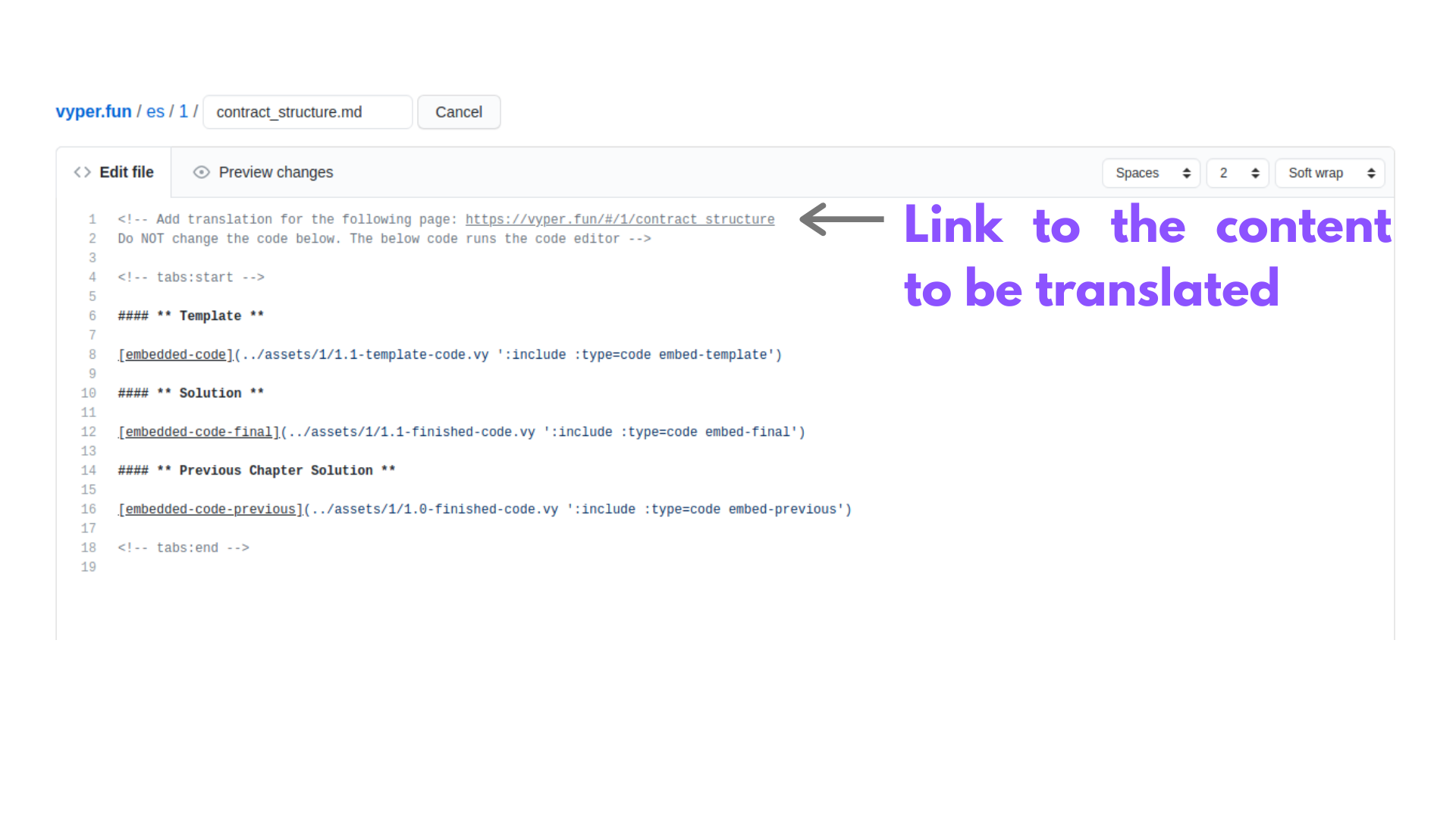 @@ -66,6 +66,6 @@ In the next page, you can add a description about the chapter and the translatio Keep an on this page. I will review your edits and if there is something wrong, I will let you know in the comments section of the page. -If everything is ok, then your pull request will be merged! You will be listed as a contributor on the [contributors wall](https://github.com/vyperfun/vyper.fun#contributors)! +If everything is ok, then your pull request will be merged! You will be listed as a contributor on the [contributors wall](https://github.com/vyperlang/learn#contributors)! 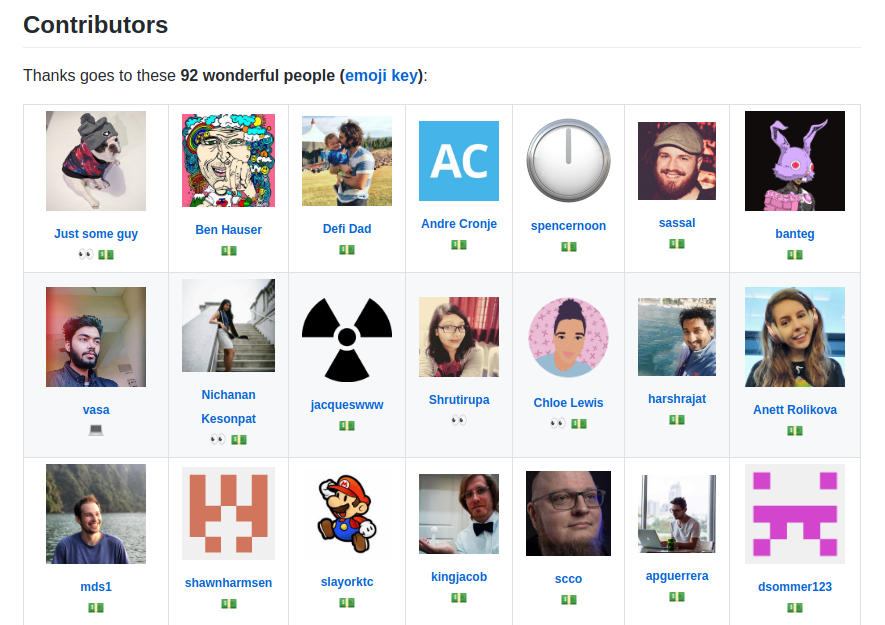 diff --git a/README.md b/README.md index 2b29c08..9e59fcc 100644 --- a/README.md +++ b/README.md @@ -4,29 +4,22 @@ ## What is this? -This is an [interactive tutorial website]( https://learn.vyperlang.org/#/) for learning Vyper while building games using smart contracts, similar to Cryptozombies. +This is an [interactive tutorial website](https://learn.vyperlang.org/) for learning Vyper while building games using smart contracts, similar to Cryptozombies. ## Running tutorials locally 1. Clone the repo: ```bash -git clone https://github.com/vyperlang/vyper.fun +git clone https://github.com/vyperlang/learn ``` 2. Download and Install [Node.js](https://nodejs.org/). -3. Install `docsify` globally. +3. Run the tutorial locally. ```bash -// you may need to use sudo -npm i docsify-cli -g -``` - -4. Run the tutorial locally. - -```bash -docsify serve . +npx docsify-cli serve . ``` ## Contribution Guide @@ -37,7 +30,7 @@ Given that Vyper.fun is a global project, we believe it's critical that Vyper.fu ### How to get involved -Check out [contribution guide](https://github.com/vyperlang/vyper.fun/blob/master/CONTRIBUTION.md) for instructions on how to get started as a translator. Don't see your language listed? Please comment [here](https://github.com/vyperlang/vyper.fun/issues/6) & we'll help get you set up. +Check out [contribution guide](https://github.com/vyperlang/learn/blob/master/CONTRIBUTION.md) for instructions on how to get started as a translator. Don't see your language listed? Please comment [here](https://github.com/vyperlang/learn/issues/6) & we'll help get you set up. Join our [Discord server](https://discord.gg/Svaav43) for collaboration & support. diff --git a/WELCOME.md b/WELCOME.md index 4e4a4b5..07bc79a 100644 --- a/WELCOME.md +++ b/WELCOME.md @@ -4,4 +4,4 @@ ## What is this? -This is an [interactive tutorial website](https://vyper.fun) for learning Vyper while building games using smart contracts, similar to Cryptozombies. +This is an [interactive tutorial website](https://learn.vyperlang.org) for learning Vyper while building games using smart contracts, similar to Cryptozombies. diff --git a/_coverpage.md b/_coverpage.md index 320e5ac..1e687ed 100644 --- a/_coverpage.md +++ b/_coverpage.md @@ -5,8 +5,8 @@ > Learn Vyper by building a Pokémon Game! [Get Started](/lessons.html) -[Empezar](https://vyper.fun/en-lessons.html) -[GitHub](https://github.com/vyperlang/vyper.fun) +[Empezar](/es-lessons.html) +[GitHub](https://github.com/vyperlang/learn) diff --git a/ar-sa/1/events.md b/ar-sa/1/events.md index 4898387..b7cc3f8 100644 --- a/ar-sa/1/events.md +++ b/ar-sa/1/events.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/external_internal_functions.md b/ar-sa/1/external_internal_functions.md index f3a1a6a..e979dac 100644 --- a/ar-sa/1/external_internal_functions.md +++ b/ar-sa/1/external_internal_functions.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/function_declarations.md b/ar-sa/1/function_declarations.md index 480bb06..c944da3 100644 --- a/ar-sa/1/function_declarations.md +++ b/ar-sa/1/function_declarations.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/introduction.md b/ar-sa/1/introduction.md index a97bd30..e9d2da1 100644 --- a/ar-sa/1/introduction.md +++ b/ar-sa/1/introduction.md @@ -1,2 +1,2 @@ - diff --git a/ar-sa/1/keccak256-and-typecasting.md b/ar-sa/1/keccak256-and-typecasting.md index c2f12c5..98e4495 100644 --- a/ar-sa/1/keccak256-and-typecasting.md +++ b/ar-sa/1/keccak256-and-typecasting.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/mappings.md b/ar-sa/1/mappings.md index 340fb00..d3ffe90 100644 --- a/ar-sa/1/mappings.md +++ b/ar-sa/1/mappings.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/math_operations.md b/ar-sa/1/math_operations.md index 5852e6b..1e77aae 100644 --- a/ar-sa/1/math_operations.md +++ b/ar-sa/1/math_operations.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/more_on_functions.md b/ar-sa/1/more_on_functions.md index 39527d4..8cb8d94 100644 --- a/ar-sa/1/more_on_functions.md +++ b/ar-sa/1/more_on_functions.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/public_vars.md b/ar-sa/1/public_vars.md index 18b984d..f5181f5 100644 --- a/ar-sa/1/public_vars.md +++ b/ar-sa/1/public_vars.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/putting_it_together.md b/ar-sa/1/putting_it_together.md index 6acc642..78f6fdd 100644 --- a/ar-sa/1/putting_it_together.md +++ b/ar-sa/1/putting_it_together.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/state_vars_and_ints.md b/ar-sa/1/state_vars_and_ints.md index 85d0080..c382678 100644 --- a/ar-sa/1/state_vars_and_ints.md +++ b/ar-sa/1/state_vars_and_ints.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/1/structs.md b/ar-sa/1/structs.md index 228bd30..8b7a733 100644 --- a/ar-sa/1/structs.md +++ b/ar-sa/1/structs.md @@ -1,4 +1,4 @@ - #### ** Template ** diff --git a/ar-sa/1/working_with_structs.md b/ar-sa/1/working_with_structs.md index ce655f0..f8356bd 100644 --- a/ar-sa/1/working_with_structs.md +++ b/ar-sa/1/working_with_structs.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/addresses.md b/ar-sa/2/addresses.md index ae8d9b0..b7beaa5 100644 --- a/ar-sa/2/addresses.md +++ b/ar-sa/2/addresses.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/assert.md b/ar-sa/2/assert.md index 7df5fca..e6f3689 100644 --- a/ar-sa/2/assert.md +++ b/ar-sa/2/assert.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/calling_a_contract.md b/ar-sa/2/calling_a_contract.md index 2766189..d339b6a 100644 --- a/ar-sa/2/calling_a_contract.md +++ b/ar-sa/2/calling_a_contract.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/comparison_operator.md b/ar-sa/2/comparison_operator.md index ebab2d1..bffbbbb 100644 --- a/ar-sa/2/comparison_operator.md +++ b/ar-sa/2/comparison_operator.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/empty.md b/ar-sa/2/empty.md index 90ddc57..2850ba7 100644 --- a/ar-sa/2/empty.md +++ b/ar-sa/2/empty.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/init.md b/ar-sa/2/init.md index b3c001c..c1b5beb 100644 --- a/ar-sa/2/init.md +++ b/ar-sa/2/init.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/interfaces.md b/ar-sa/2/interfaces.md index 16fad51..a9f0c69 100644 --- a/ar-sa/2/interfaces.md +++ b/ar-sa/2/interfaces.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/introduction.md b/ar-sa/2/introduction.md index 1cf915c..ff9b282 100644 --- a/ar-sa/2/introduction.md +++ b/ar-sa/2/introduction.md @@ -1,2 +1,2 @@ - diff --git a/ar-sa/2/msg-sender.md b/ar-sa/2/msg-sender.md index 091d861..8813b56 100644 --- a/ar-sa/2/msg-sender.md +++ b/ar-sa/2/msg-sender.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/putting_it_together.md b/ar-sa/2/putting_it_together.md index 6b63fd4..014cdf8 100644 --- a/ar-sa/2/putting_it_together.md +++ b/ar-sa/2/putting_it_together.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/random_wild_pokemon.md b/ar-sa/2/random_wild_pokemon.md index 640ded5..51b0e02 100644 --- a/ar-sa/2/random_wild_pokemon.md +++ b/ar-sa/2/random_wild_pokemon.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/2/wild_pokemons.md b/ar-sa/2/wild_pokemons.md index 079323b..9fb25ad 100644 --- a/ar-sa/2/wild_pokemons.md +++ b/ar-sa/2/wild_pokemons.md @@ -1,4 +1,4 @@ - diff --git a/ar-sa/WELCOME.md b/ar-sa/WELCOME.md index 4e4a4b5..07bc79a 100644 --- a/ar-sa/WELCOME.md +++ b/ar-sa/WELCOME.md @@ -4,4 +4,4 @@ ## What is this? -This is an [interactive tutorial website](https://vyper.fun) for learning Vyper while building games using smart contracts, similar to Cryptozombies. +This is an [interactive tutorial website](https://learn.vyperlang.org) for learning Vyper while building games using smart contracts, similar to Cryptozombies. diff --git a/ar-sa/_sidebar.md b/ar-sa/_sidebar.md index 71dd49b..1672143 100644 --- a/ar-sa/_sidebar.md +++ b/ar-sa/_sidebar.md @@ -31,4 +31,4 @@ - [Putting It Together](2/putting_it_together.md) - [Gitcoin Grant](https://gitcoin.co/grants/1122/vyperfun) -- [Report an Issue](https://github.com/vyperlang/vyper.fun/issues) +- [Report an Issue](https://github.com/vyperlang/learn/issues) diff --git a/assets/1/wildPokemons.vy b/assets/1/wildPokemons.vy index f98ab5d..7d5d41c 100644 --- a/assets/1/wildPokemons.vy +++ b/assets/1/wildPokemons.vy @@ -55,7 +55,7 @@ def battle(pokemon: Pokemon) -> (bool, String[32], uint256, uint256): self.battleCount += 1 - if(pokemon.HP > randomHP): + if pokemon.HP > randomHP: return True, randomName, randomDNA, randomHP else: return False, empty(String[32]), empty(uint256), empty(uint256) \ No newline at end of file diff --git a/assets/2/2.11-finished-code.vy b/assets/2/2.11-finished-code.vy index 5af025c..0e35a5e 100644 --- a/assets/2/2.11-finished-code.vy +++ b/assets/2/2.11-finished-code.vy @@ -44,9 +44,9 @@ def battleWildPokemon(pokemonIndex: uint256): battleResult, newPokemonName, newPokemonDNA, newPokemonHP = WildPokemons(WILD_POKEMON).battle(self.trainerToPokemon[msg.sender][pokemonIndex]) - if(battleResult): - self.trainerToPokemon[msg.sender][pokemonIndex].matches +=1 - self.trainerToPokemon[msg.sender][pokemonIndex].wins +=1 + if battleResult: + self.trainerToPokemon[msg.sender][pokemonIndex].matches += 1 + self.trainerToPokemon[msg.sender][pokemonIndex].wins += 1 newPokemon: Pokemon = Pokemon({ name: newPokemonName, @@ -65,7 +65,7 @@ def battleWildPokemon(pokemonIndex: uint256): log NewPokemonCreated(newPokemonName, newPokemonDNA, newPokemonHP) else: - self.trainerToPokemon[msg.sender][pokemonIndex].matches +=1 + self.trainerToPokemon[msg.sender][pokemonIndex].matches += 1 @pure @internal diff --git a/assets/2/2.6-finished-code.vy b/assets/2/2.6-finished-code.vy index 53ac549..1a8864a 100644 --- a/assets/2/2.6-finished-code.vy +++ b/assets/2/2.6-finished-code.vy @@ -55,5 +55,5 @@ def battle(pokemon: Pokemon) -> (bool, String[32], uint256, uint256): self.battleCount += 1 - if(pokemon.HP > randomHP): + if pokemon.HP > randomHP: return True, randomName, randomDNA, randomHP \ No newline at end of file diff --git a/assets/2/2.7-finished-code.vy b/assets/2/2.7-finished-code.vy index 2aa103e..8b4693e 100644 --- a/assets/2/2.7-finished-code.vy +++ b/assets/2/2.7-finished-code.vy @@ -55,7 +55,7 @@ def battle(pokemon: Pokemon) -> (bool, String[32], uint256, uint256): self.battleCount += 1 - if(pokemon.HP > randomHP): + if pokemon.HP > randomHP: return True, randomName, randomDNA, randomHP else: return False, empty(String[32]), empty(uint256), empty(uint256) \ No newline at end of file diff --git a/assets/2/2.7-template-code.vy b/assets/2/2.7-template-code.vy index a23d81e..2d181e7 100644 --- a/assets/2/2.7-template-code.vy +++ b/assets/2/2.7-template-code.vy @@ -55,6 +55,6 @@ def battle(pokemon: Pokemon) -> (bool, String[32], uint256, uint256): self.battleCount += 1 - if(pokemon.HP > randomHP): + if pokemon.HP > randomHP: return True, randomName, randomDNA, randomHP # add else statement here \ No newline at end of file diff --git a/assets/final/pokemon-battle.vy b/assets/final/pokemon-battle.vy index 2aa103e..8b4693e 100644 --- a/assets/final/pokemon-battle.vy +++ b/assets/final/pokemon-battle.vy @@ -55,7 +55,7 @@ def battle(pokemon: Pokemon) -> (bool, String[32], uint256, uint256): self.battleCount += 1 - if(pokemon.HP > randomHP): + if pokemon.HP > randomHP: return True, randomName, randomDNA, randomHP else: return False, empty(String[32]), empty(uint256), empty(uint256) \ No newline at end of file diff --git a/assets/final/pokemon-trainer.vy b/assets/final/pokemon-trainer.vy index 22bedda..baa717e 100644 --- a/assets/final/pokemon-trainer.vy +++ b/assets/final/pokemon-trainer.vy @@ -46,10 +46,10 @@ def battleWildPokemon(pokemonIndex: uint256): battleResult, newPokemonName, newPokemonDNA, newPokemonHP = WildPokemons(WILD_POKEMON).battle(self.trainerToPokemon[msg.sender][pokemonIndex]) - if(battleResult): + if battleResult: - self.trainerToPokemon[msg.sender][pokemonIndex].matches +=1 - self.trainerToPokemon[msg.sender][pokemonIndex].wins +=1 + self.trainerToPokemon[msg.sender][pokemonIndex].matches += 1 + self.trainerToPokemon[msg.sender][pokemonIndex].wins += 1 newPokemon: Pokemon = Pokemon({ name: newPokemonName, @@ -68,7 +68,7 @@ def battleWildPokemon(pokemonIndex: uint256): log NewPokemonCreated(newPokemonName, newPokemonDNA, newPokemonHP) else: - self.trainerToPokemon[msg.sender][pokemonIndex].matches +=1 + self.trainerToPokemon[msg.sender][pokemonIndex].matches += 1 @pure @internal diff --git a/custom/custom.css b/custom/custom.css index c291f93..30dddaa 100644 --- a/custom/custom.css +++ b/custom/custom.css @@ -212,6 +212,7 @@ section.cover .cover-main > p:last-child a:last-child { .markdown-section a { font-weight: unset; + color: #dcdca9; } /* End custom markdown styling */ diff --git a/de/1/contract_structure.md b/de/1/contract_structure.md index d208bbb..3914b0c 100644 --- a/de/1/contract_structure.md +++ b/de/1/contract_structure.md @@ -1,4 +1,4 @@ - # Kapitel 1: Contracts @@ -13,7 +13,7 @@ Vyper Contracts sind innerhalb von Dateien. Jede Datei enthält genau einen Cont Vyper unterstützt Versions-Compiler-Anweisungen um sicherzustellen, dass ein Contract nur mit einer festgelegten Compilerversion bzw. Versionsspanne kompiliert wird. -Dazu wird die [NPM](https://docs.npmjs.com/misc/semver) Syntax verwendet. +Dazu wird die [NPM](https://docs.npmjs.com/about-semantic-versioning) Syntax verwendet. Für dieses Tutorial verwenden wir eine Compilerversion zwischen `0.2.0` (inklusive) und `0.3.0` (exklusive). Da sieht wie folgt aus: diff --git a/de/1/events.md b/de/1/events.md index 4898387..b7cc3f8 100644 --- a/de/1/events.md +++ b/de/1/events.md @@ -1,4 +1,4 @@ - diff --git a/de/1/external_internal_functions.md b/de/1/external_internal_functions.md index f3a1a6a..e979dac 100644 --- a/de/1/external_internal_functions.md +++ b/de/1/external_internal_functions.md @@ -1,4 +1,4 @@ - diff --git a/de/1/function_declarations.md b/de/1/function_declarations.md index 480bb06..c944da3 100644 --- a/de/1/function_declarations.md +++ b/de/1/function_declarations.md @@ -1,4 +1,4 @@ - diff --git a/de/1/introduction.md b/de/1/introduction.md index 46e6960..4e9fad4 100644 --- a/de/1/introduction.md +++ b/de/1/introduction.md @@ -1,4 +1,4 @@ - # Erstelle dein erstes Pokemon diff --git a/de/1/keccak256-and-typecasting.md b/de/1/keccak256-and-typecasting.md index c2f12c5..98e4495 100644 --- a/de/1/keccak256-and-typecasting.md +++ b/de/1/keccak256-and-typecasting.md @@ -1,4 +1,4 @@ - diff --git a/de/1/mappings.md b/de/1/mappings.md index 340fb00..d3ffe90 100644 --- a/de/1/mappings.md +++ b/de/1/mappings.md @@ -1,4 +1,4 @@ - diff --git a/de/1/math_operations.md b/de/1/math_operations.md index 0d2f697..3e137fa 100644 --- a/de/1/math_operations.md +++ b/de/1/math_operations.md @@ -1,4 +1,4 @@ - # Chapter 3: Mathematische Operationen diff --git a/de/1/more_on_functions.md b/de/1/more_on_functions.md index 39527d4..8cb8d94 100644 --- a/de/1/more_on_functions.md +++ b/de/1/more_on_functions.md @@ -1,4 +1,4 @@ - diff --git a/de/1/public_vars.md b/de/1/public_vars.md index 18b984d..f5181f5 100644 --- a/de/1/public_vars.md +++ b/de/1/public_vars.md @@ -1,4 +1,4 @@ - diff --git a/de/1/putting_it_together.md b/de/1/putting_it_together.md index 6acc642..78f6fdd 100644 --- a/de/1/putting_it_together.md +++ b/de/1/putting_it_together.md @@ -1,4 +1,4 @@ - diff --git a/de/1/state_vars_and_ints.md b/de/1/state_vars_and_ints.md index c954658..ff4b176 100644 --- a/de/1/state_vars_and_ints.md +++ b/de/1/state_vars_and_ints.md @@ -1,4 +1,4 @@ - # Kapitel 2: Zustandsvariablen, Zahlen & Konstanten @@ -16,7 +16,7 @@ Zustandsvariablen sind permanent im Contract-Speicher gespeichert. Das bedeutet, storedData: int128 ``` -In diesem Beispielcontract speicherten wir ein [`int128`](https://vyper.readthedocs.io/en/stable/types.html#signed-integer-128-bit) genannt `storedData`, welche einen _Standardwert_ von `1` besitzt. +In diesem Beispielcontract speicherten wir ein [`int128`](https://docs.vyperlang.org/en/stable/types.html#signed-integer-n-bit) genannt `storedData`, welche einen _Standardwert_ von `1` besitzt. ## Positive Zahlen: `uint256` diff --git a/de/1/structs.md b/de/1/structs.md index 228bd30..8b7a733 100644 --- a/de/1/structs.md +++ b/de/1/structs.md @@ -1,4 +1,4 @@ - #### ** Template ** diff --git a/de/1/working_with_structs.md b/de/1/working_with_structs.md index ce655f0..f8356bd 100644 --- a/de/1/working_with_structs.md +++ b/de/1/working_with_structs.md @@ -1,4 +1,4 @@ - diff --git a/de/2/addresses.md b/de/2/addresses.md index ceca33d..568ae6d 100644 --- a/de/2/addresses.md +++ b/de/2/addresses.md @@ -1,4 +1,4 @@ - # Kapitel 1: Adressen diff --git a/de/2/assert.md b/de/2/assert.md index 7df5fca..e6f3689 100644 --- a/de/2/assert.md +++ b/de/2/assert.md @@ -1,4 +1,4 @@ - diff --git a/de/2/calling_a_contract.md b/de/2/calling_a_contract.md index 2766189..d339b6a 100644 --- a/de/2/calling_a_contract.md +++ b/de/2/calling_a_contract.md @@ -1,4 +1,4 @@ - diff --git a/de/2/comparison_operator.md b/de/2/comparison_operator.md index ebab2d1..bffbbbb 100644 --- a/de/2/comparison_operator.md +++ b/de/2/comparison_operator.md @@ -1,4 +1,4 @@ - diff --git a/de/2/empty.md b/de/2/empty.md index 90ddc57..2850ba7 100644 --- a/de/2/empty.md +++ b/de/2/empty.md @@ -1,4 +1,4 @@ - diff --git a/de/2/init.md b/de/2/init.md index b3c001c..c1b5beb 100644 --- a/de/2/init.md +++ b/de/2/init.md @@ -1,4 +1,4 @@ - diff --git a/de/2/interfaces.md b/de/2/interfaces.md index 16fad51..a9f0c69 100644 --- a/de/2/interfaces.md +++ b/de/2/interfaces.md @@ -1,4 +1,4 @@ - diff --git a/de/2/introduction.md b/de/2/introduction.md index fd410d9..3a85a14 100644 --- a/de/2/introduction.md +++ b/de/2/introduction.md @@ -1,4 +1,4 @@ - # Fang 'sie alle diff --git a/de/2/msg-sender.md b/de/2/msg-sender.md index 091d861..8813b56 100644 --- a/de/2/msg-sender.md +++ b/de/2/msg-sender.md @@ -1,4 +1,4 @@ - diff --git a/de/2/putting_it_together.md b/de/2/putting_it_together.md index 6b63fd4..014cdf8 100644 --- a/de/2/putting_it_together.md +++ b/de/2/putting_it_together.md @@ -1,4 +1,4 @@ - diff --git a/de/2/random_wild_pokemon.md b/de/2/random_wild_pokemon.md index 640ded5..51b0e02 100644 --- a/de/2/random_wild_pokemon.md +++ b/de/2/random_wild_pokemon.md @@ -1,4 +1,4 @@ - diff --git a/de/2/wild_pokemons.md b/de/2/wild_pokemons.md index 079323b..9fb25ad 100644 --- a/de/2/wild_pokemons.md +++ b/de/2/wild_pokemons.md @@ -1,4 +1,4 @@ - diff --git a/de/WELCOME.md b/de/WELCOME.md index 4e4a4b5..07bc79a 100644 --- a/de/WELCOME.md +++ b/de/WELCOME.md @@ -4,4 +4,4 @@ ## What is this? -This is an [interactive tutorial website](https://vyper.fun) for learning Vyper while building games using smart contracts, similar to Cryptozombies. +This is an [interactive tutorial website](https://learn.vyperlang.org) for learning Vyper while building games using smart contracts, similar to Cryptozombies. diff --git a/de/_sidebar.md b/de/_sidebar.md index 71dd49b..1672143 100644 --- a/de/_sidebar.md +++ b/de/_sidebar.md @@ -31,4 +31,4 @@ - [Putting It Together](2/putting_it_together.md) - [Gitcoin Grant](https://gitcoin.co/grants/1122/vyperfun) -- [Report an Issue](https://github.com/vyperlang/vyper.fun/issues) +- [Report an Issue](https://github.com/vyperlang/learn/issues) diff --git a/en-lessons.html b/es-lessons.html similarity index 94% rename from en-lessons.html rename to es-lessons.html index 9a4d16c..fa4bcd7 100644 --- a/en-lessons.html +++ b/es-lessons.html @@ -35,8 +35,8 @@ property="og:description" content="Learn Vyper by building a Pokemon Game. Study structure of a contract, types, variables and constants, statements, control structures, scoping and declarations, built-in functions, interfaces, event logging, natspec metadata" /> - - + + @@ -113,7 +113,7 @@