New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Deserialization of Map<Enum, Int> fails with generic implementation, works with concrete? (Kotlin) #412
Comments
As a test I created a function out of these 2 lines:
into the
But when using that function in the test which worked fine (ie, replacing the 2 lines above, with the function), it fails ?!
This fails at the assertion because of the same reason. Reverting back to the 2 lines in the test , makes the test pass again? |
I narrowed it down to a Kotlin feature, but I don't know why it happens: The following function fails:
But removing the Ie, the function:
works! This is unfortunate, because my actual goal is to have a generics like function like so:
But - as I would expect now - still fails due the same reason. I am not sufficient enough in Kotlin to explain this behavior. Or perhaps it is a Kotlin/Jackson mixture that plays? |
I found it. I should have known, but somehow I overlooked. The whole reason this won't work how it is written now is because of Type Erasure. This answer at stackoverflow explains it. So the whole The reason the code worked without However Kotlin to the rescue! It is possible to make a generic function to read a Map with generic types. Using inline and reified from Kotlin. With that, I made the function as following (which works):
( This simply will inline the function everywhere where it is used, which can be a downside (makes the code a bit bigger). But now it will work with any kind of map you pass in it and it is statically typed 🥳 I guess I answered my own question here. Final test now looks like:
|
Although the unit test works, when further working with the data, directly in to a
Sigh. |
Are you able to recreate that failure in a test? If so, would you share a branch of j-m-k (branch off of 2.13) with your test case? |
I use:
I try to deserialize a map which has keys which should be an
enum
type (LuggageType
).When writing a test, it works as expected:
However, we use a util class (called
SwissKnife
) with some generics stuff in it. Which does:The only real difference is using a
K,V
for theTypeReference
. Now using that in another test, it seems to fail:This test basically is a copy of the first, but also calls the SwissKnife versions and compares them. At the end, it simply checks if the map will have 2 enum keys. However, it doesn't, it fails because of:
So it is as if the keys are still String.
For reference, the
LuggageType
enum is:Even uncommenting the
@JsonCreator
will not make a difference (hence I don't even see it being called!?).Your question
Can anyone elaborate/explain what is going on? Is this a bug? Or is there something I'm doing wrong?
I get confused because the only real difference is changing explicit types to generics, then again the calls are the same and the resulting objects seem to be the same. Calling
toString
on theswissKnifeMap
gives the impression it works, but since the keys are String you wont be able to tell the difference.As you can see, the keys are truely strings:
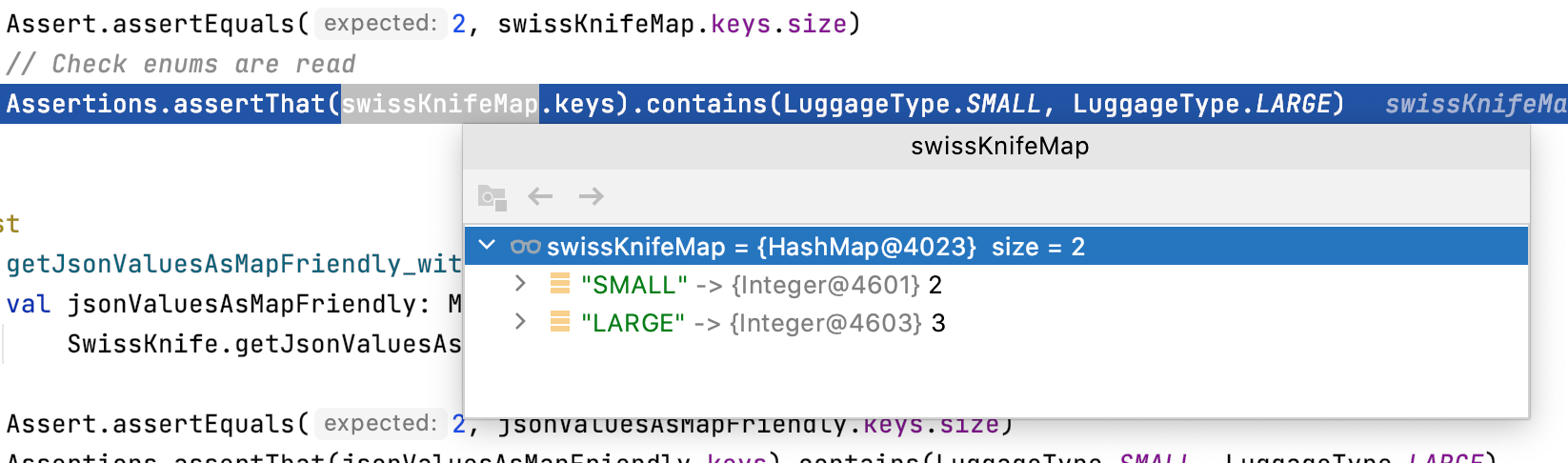
The text was updated successfully, but these errors were encountered: