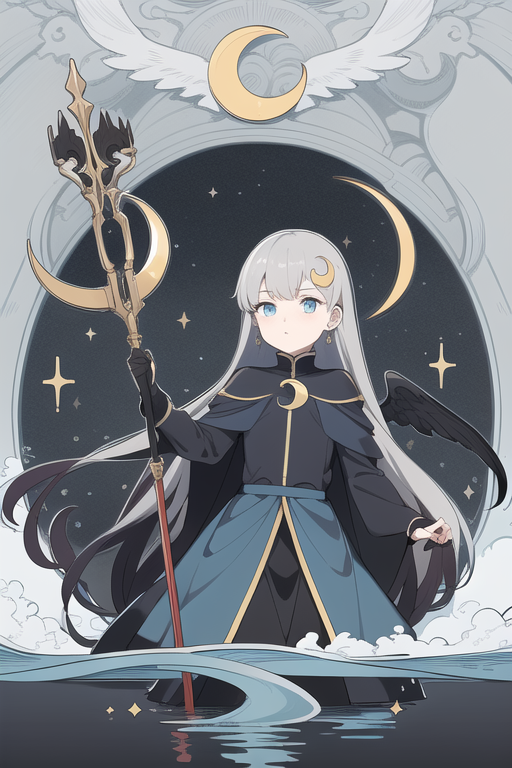
🌙 A command handler for Hikari that keeps your project neat and tidy.
- Simple and intuitive API.
- Slash, user, and message commands.
- Command localization.
- Error handling for commands, events, and autocomplete.
- Hooks to run function before or after a command (or any command from a group!)
- Plugin system to easily split bot into different modules.
- Easily use a custom context class.
- Makes typehinting easy.
- RESTBot and GatewayBot support.
📖 | User Guide
🗃️ | Docs
📦 | Pypi
Crescent is supported in python3.8+.
pip install hikari-crescent
- mCodingBot - The bot for the mCoding Discord server.
- Starboard 3 - A starbord bot by @CircuitSacul in over 17k servers.
Crescent uses class commands to simplify creating commands. Class commands allow you to create a command similar to how you declare a dataclass. The option function takes a type followed by the description, then optional information.
import crescent
import hikari
bot = hikari.GatewayBot("YOUR_TOKEN")
client = crescent.Client(bot)
# Include the command in your client - don't forget this
@client.include
# Create a slash command
@crescent.command(name="say")
class Say:
word = crescent.option(str, "The word to say")
async def callback(self, ctx: crescent.Context) -> None:
await ctx.respond(self.word)
bot.run()
Simple commands can use functions instead of classes. It is recommended to use a function when your command does not have any options.
@client.include
@crescent.command
async def ping(ctx: crescent.Context):
await ctx.respond("Pong!")
Adding arguments to the function adds more options. Information for arguments can be provided using the Annotated
type hint.
See this example for more information.
# python 3.9 +
from typing import Annotated as Atd
# python 3.8
from typing_extensions import Annotated as Atd
@client.include
@crescent.command
async def say(ctx: crescent.Context, word: str):
await ctx.respond(word)
# The same command but with a description for `word`
@client.include
@crescent.command
async def say(ctx: crescent.Context, word: Atd[str, "The word to say"]) -> None:
await ctx.respond(word)
Type | Option Type |
---|---|
str |
Text |
int |
Integer |
bool |
Boolean |
float |
Number |
hikari.User |
User |
hikari.Role |
Role |
crescent.Mentionable |
Role or User |
Any Hikari channel type. | Channel. The options will be the channel type and its subclasses. |
List[Channel Types] (classes only) |
Channel. ^ |
Union[Channel Types] (functions only) |
Channel. ^ |
hikari.Attachment |
Attachment |
Autocomplete is supported by using a callback function. This function returns a list of tuples where the first
value is the option name and the second value is the option value. str
, int
, and float
value types
can be used.
async def autocomplete(
ctx: crescent.AutocompleteContext, option: hikari.AutocompleteInteractionOption
) -> list[tuple[str, str]]:
return [("Option 1", "Option value 1"), ("Option 2", "Option value 2")]
@client.include
@crescent.command(name="class-command")
class ClassCommand:
option = crescent.option(str, autocomplete=autocomplete)
async def callback(self) -> None:
await ctx.respond(self.option)
@client.include
@crescent.command
async def function_command(
ctx: crescent.Context,
option: Annotated[str, crescent.Autocomplete(autocomplete)]
) -> None:
await ctx.respond(option)
Errors that are raised by a command can be handled by crescent.catch_command
.
class MyError(Exception):
...
@client.include
@crescent.catch_command(MyError)
async def on_err(exc: MyError, ctx: crescent.Context) -> None:
await ctx.respond("An error occurred while running the command.")
@client.include
@crescent.command
async def my_command(ctx: crescent.Context):
raise MyError()
There is also a crescent.catch_event
and crescent.catch_autocomplete
function for
events and autocomplete respectively.
import hikari
@client.include
@crescent.event
async def on_message_create(event: hikari.MessageCreateEvent):
if event.message.author.is_bot:
return
await event.message.respond("Hello!")
Using crescent's event decorator lets you use crescent's event error handling system.
Crescent has 3 builtin extensions.
- crescent-ext-cooldowns - Allows you to add sliding window rate limits to your commands.
- crescent-ext-locales - Contains classes that cover common use cases for localization.
- crescent-ext-tasks - Schedules background tasks using loops or cronjobs.
These extensions can be installed with pip.
- crescent-ext-docstrings - Lets you use docstrings to write descriptions for commands and options.
- crescent-ext-kebabify - Turns your command names into kebabs!
You can ask questions in the #crescent
channel in the Hikari Discord server. My Discord username is lunarmagpie
.
Create an issue for your feature. There aren't any guidelines right now so just don't be rude.