The easiest way to use context less Navigation, Dialog, BottomSheet and many other useful functions such as String, num, context, MediaQuery....
Name | Status |
---|---|
Context Less Navigation Service | âś… |
Context Less Dialog | âś… |
Context Less Bottom Sheet | âś… |
Context Less Snackbar | âś… |
Context Less DatePicker | âś… |
Context Less TimePicker | âś… |
Easy Menu | âś… |
String Useful Function | âś… |
Num Useful Function | âś… |
List Useful Function | âś… |
Map Useful Function | âś… |
Date Useful Function | âś… |
Color Useful Function | âś… |
Responsive Useful Function | âś… |
MediaQuery Useful Function | âś… |
Theme Useful Function | âś… |
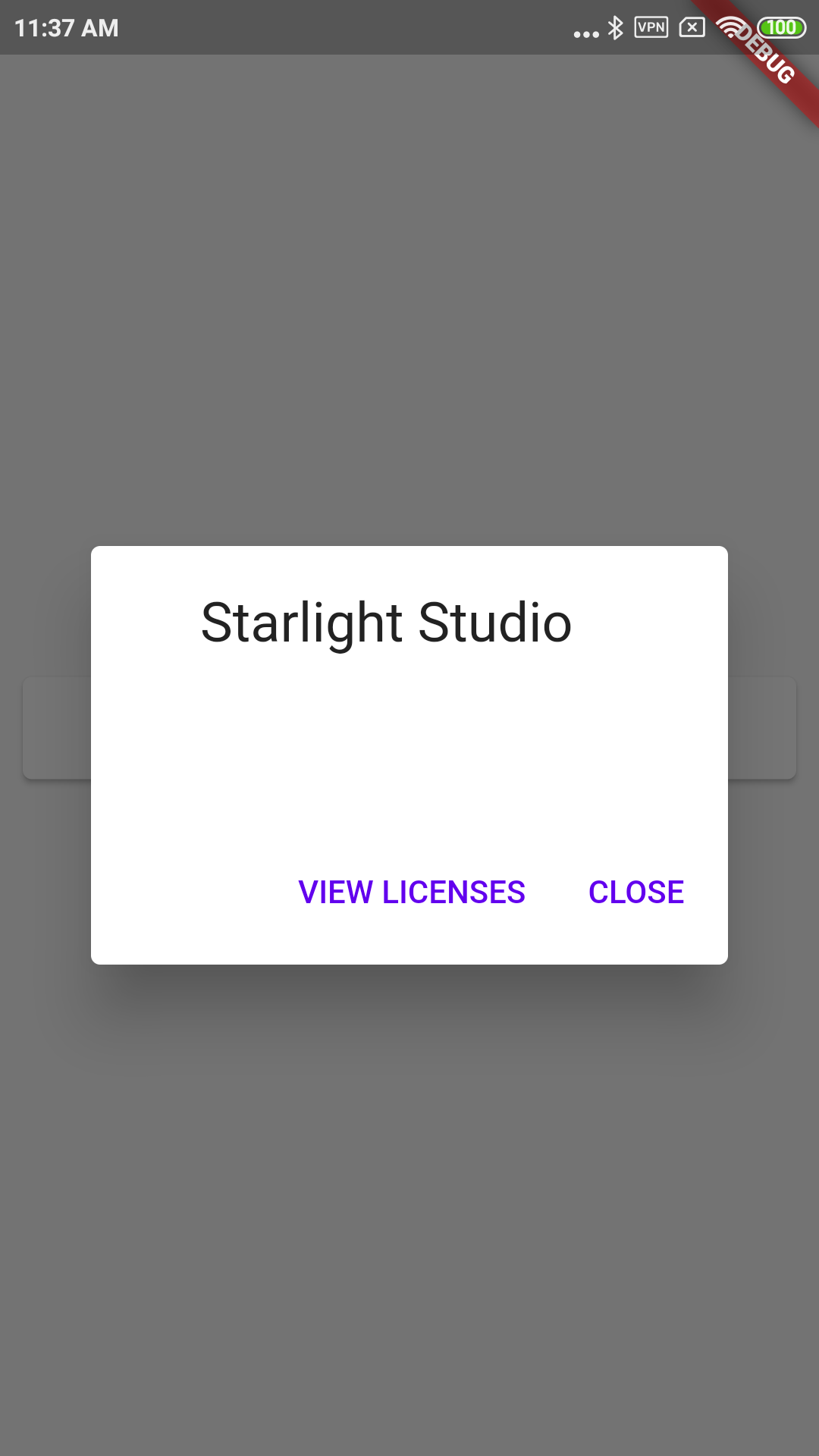
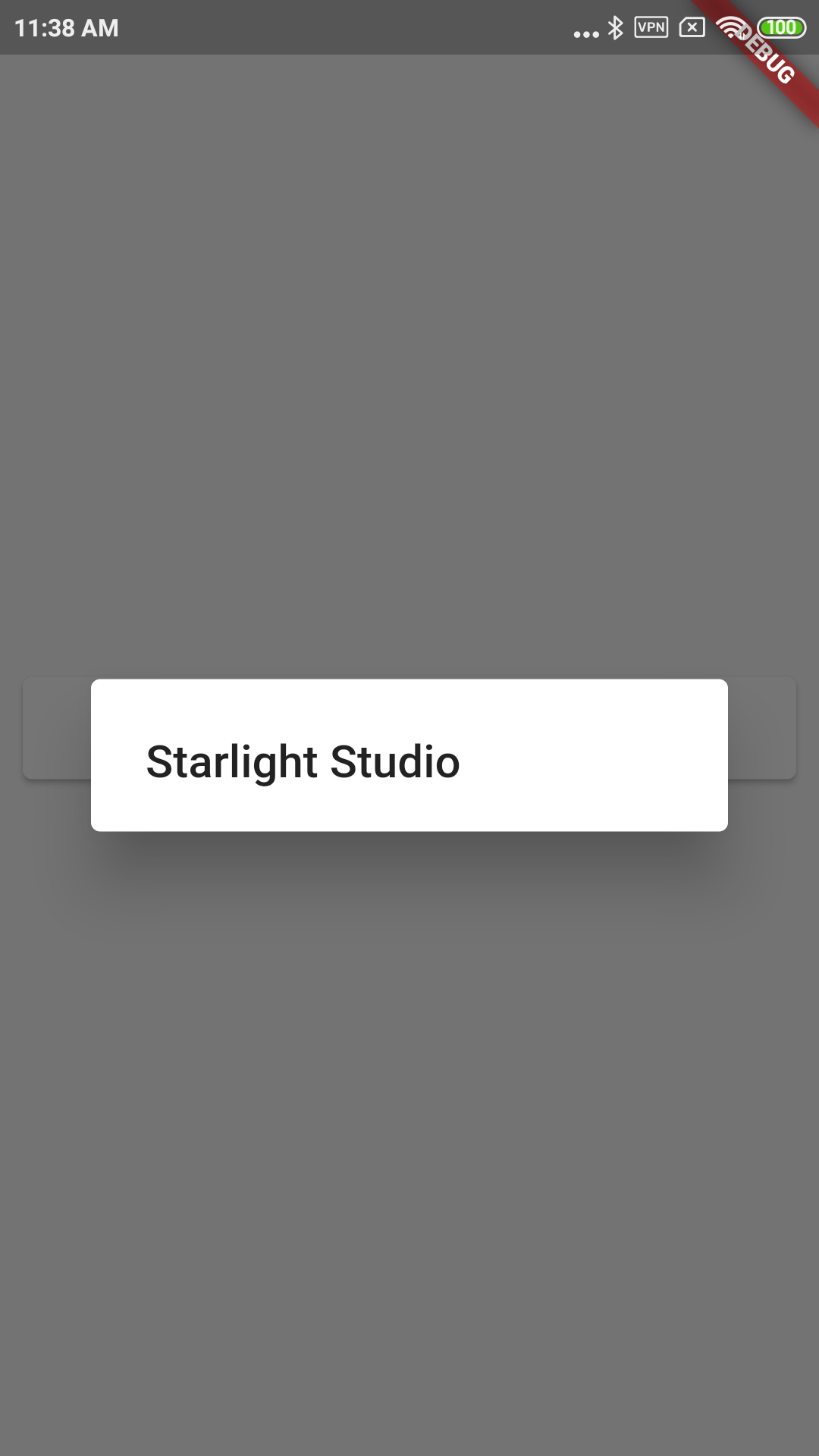
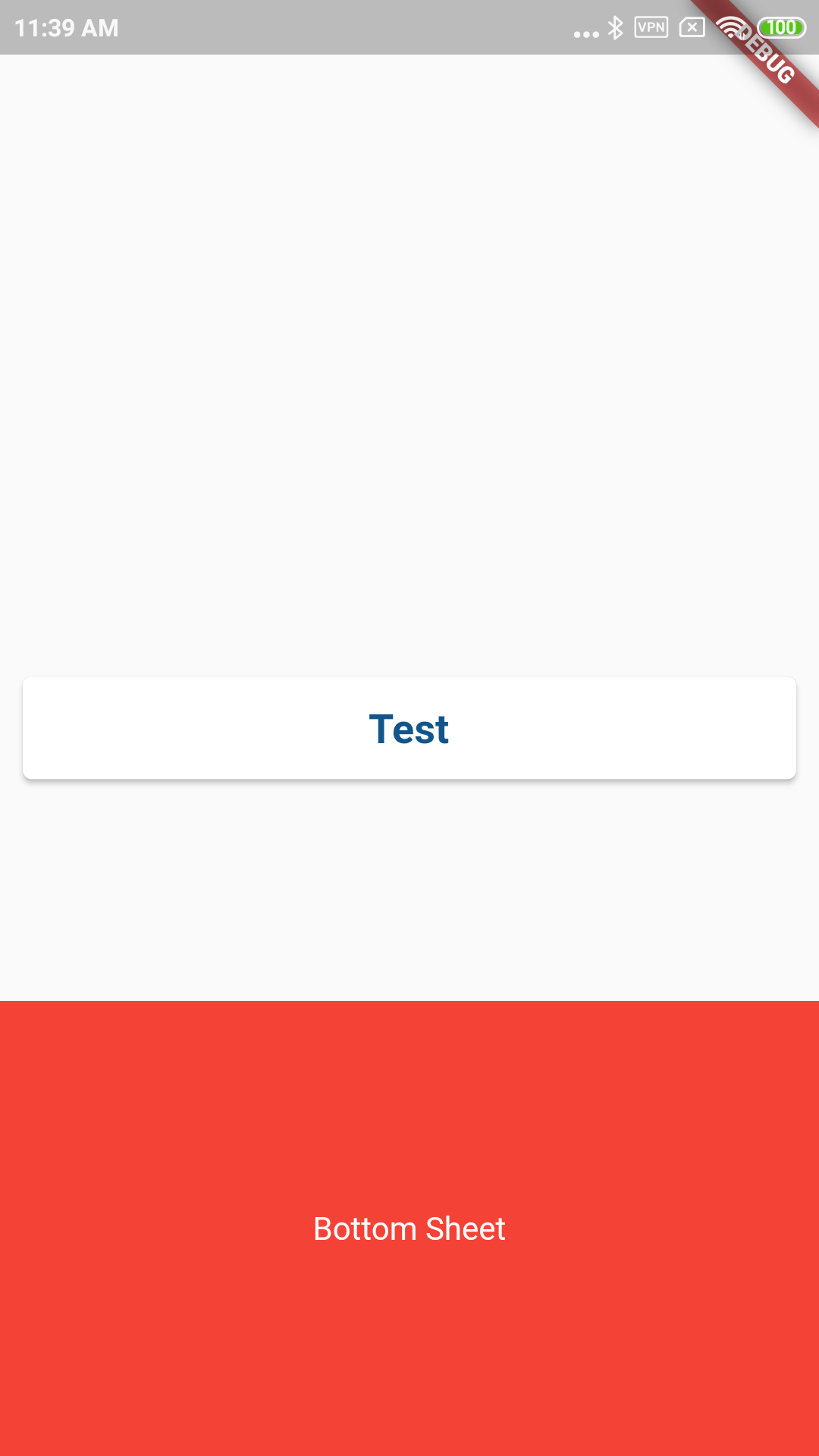
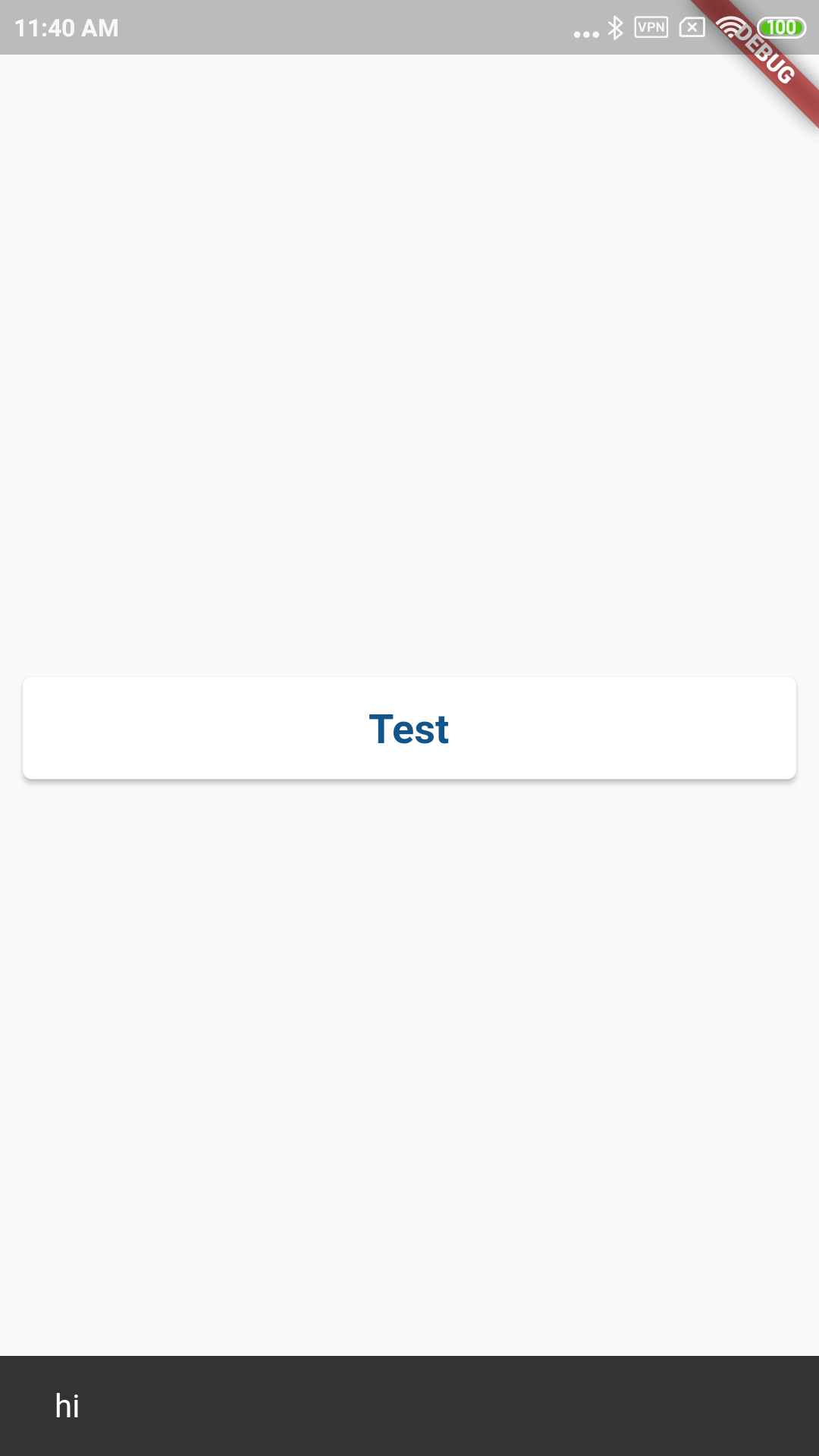
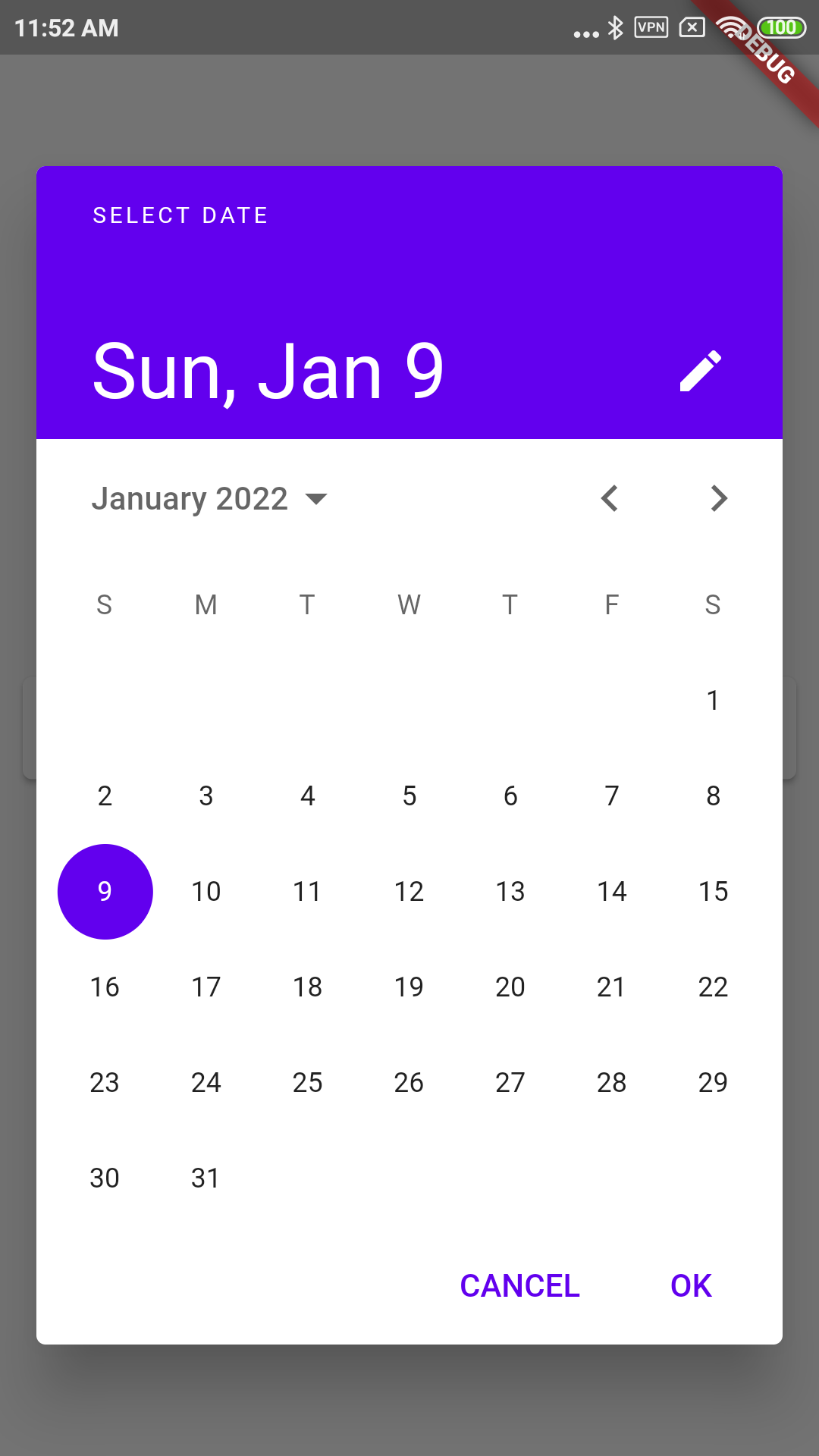
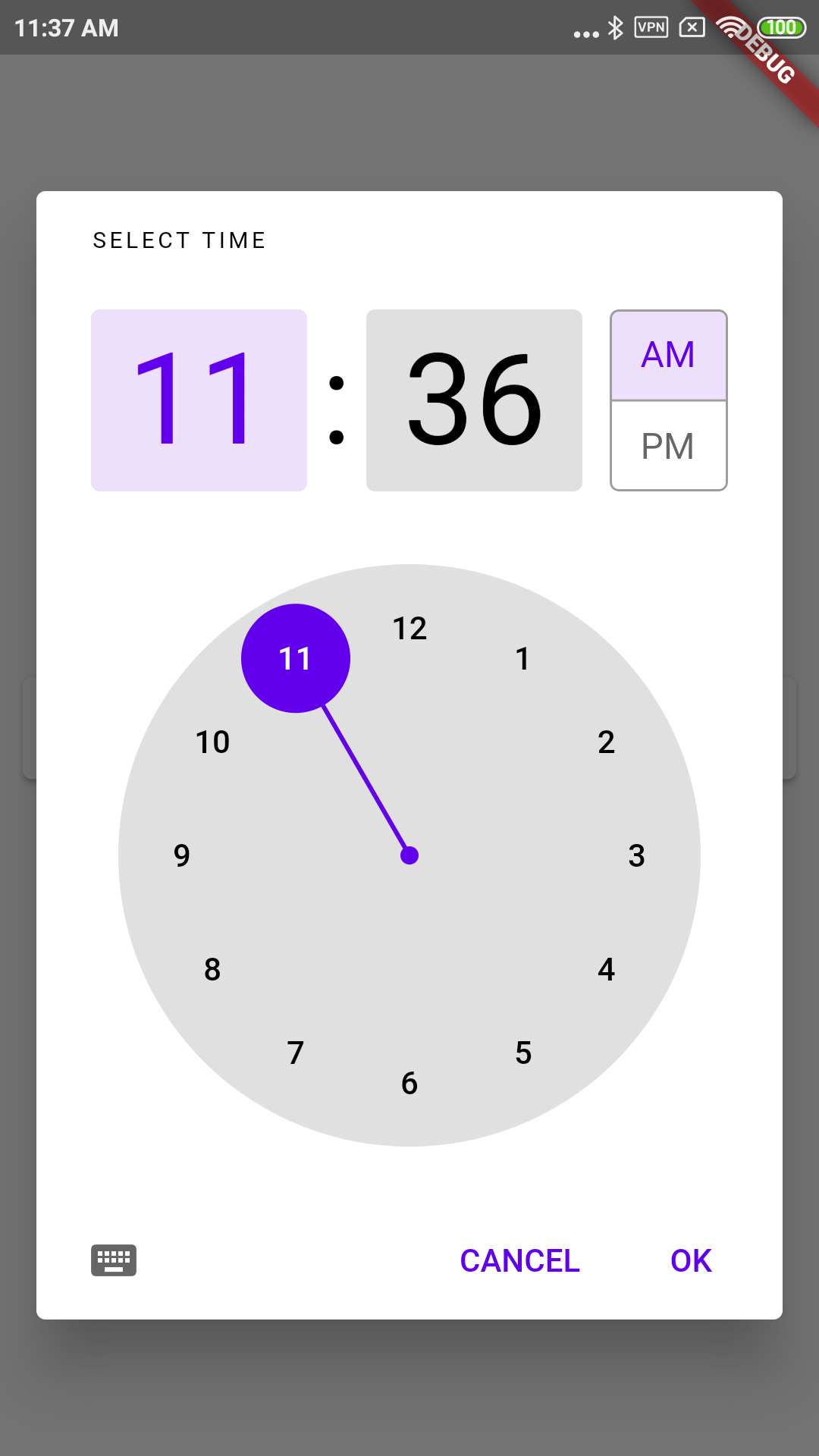
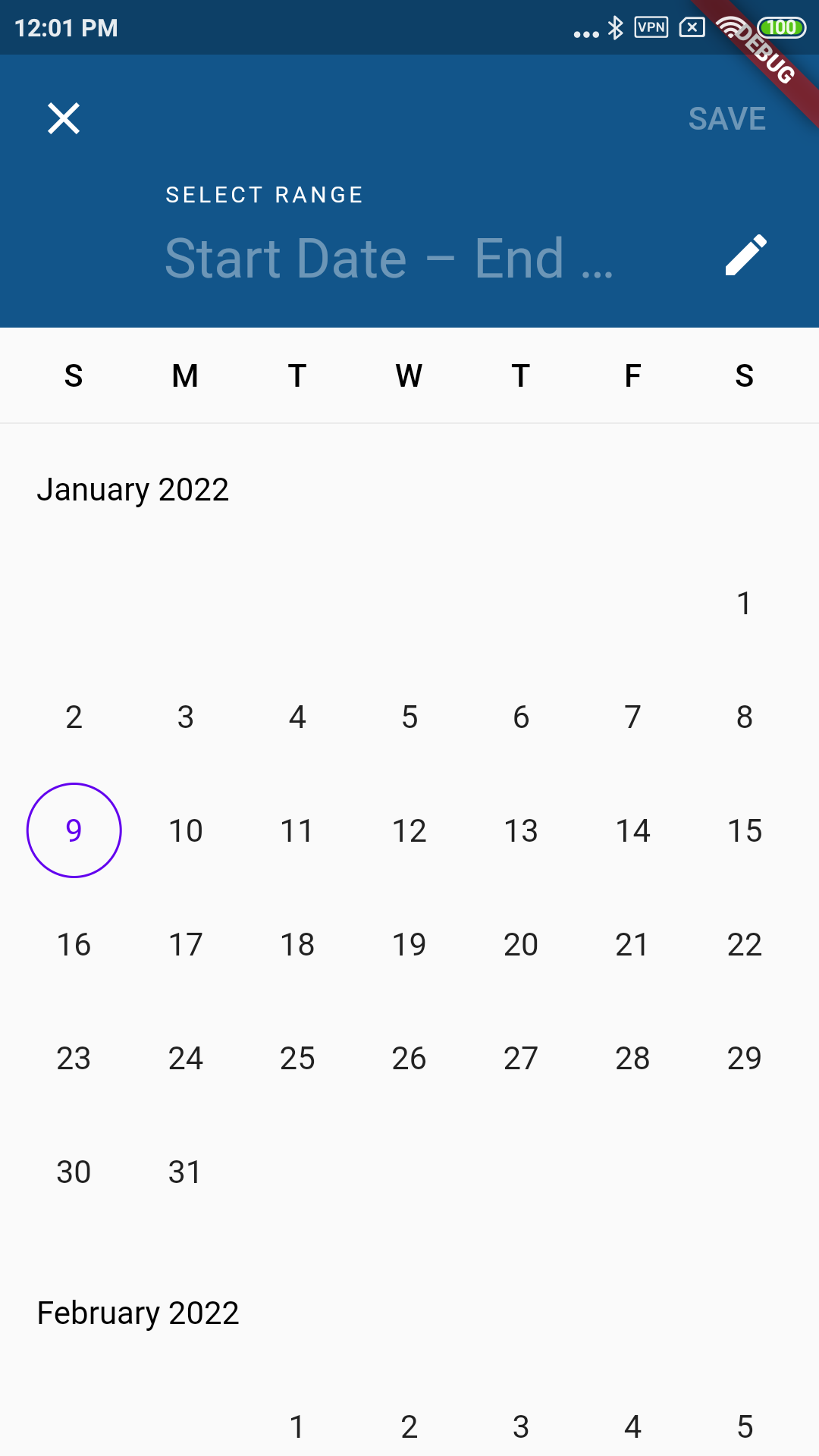
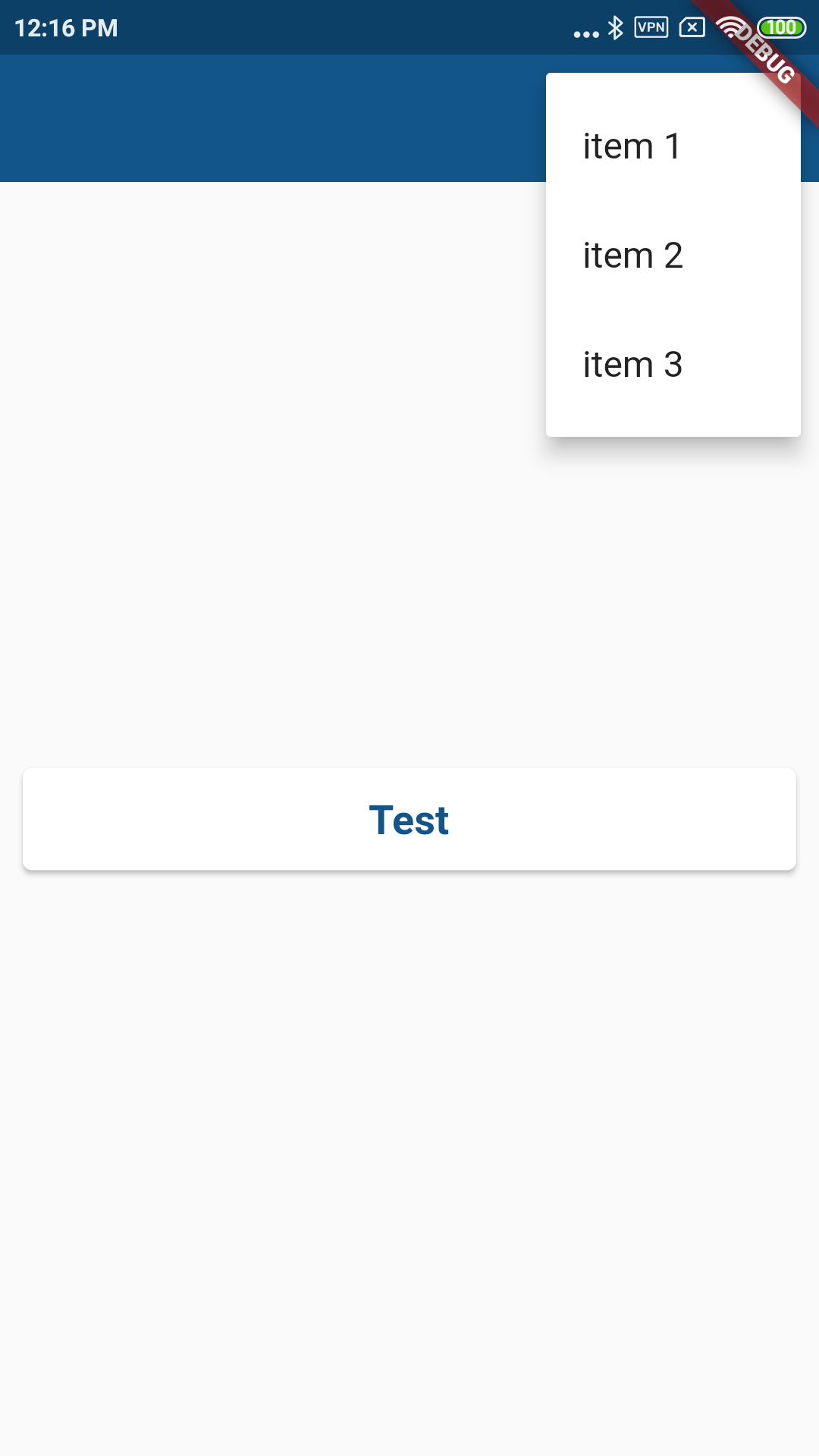
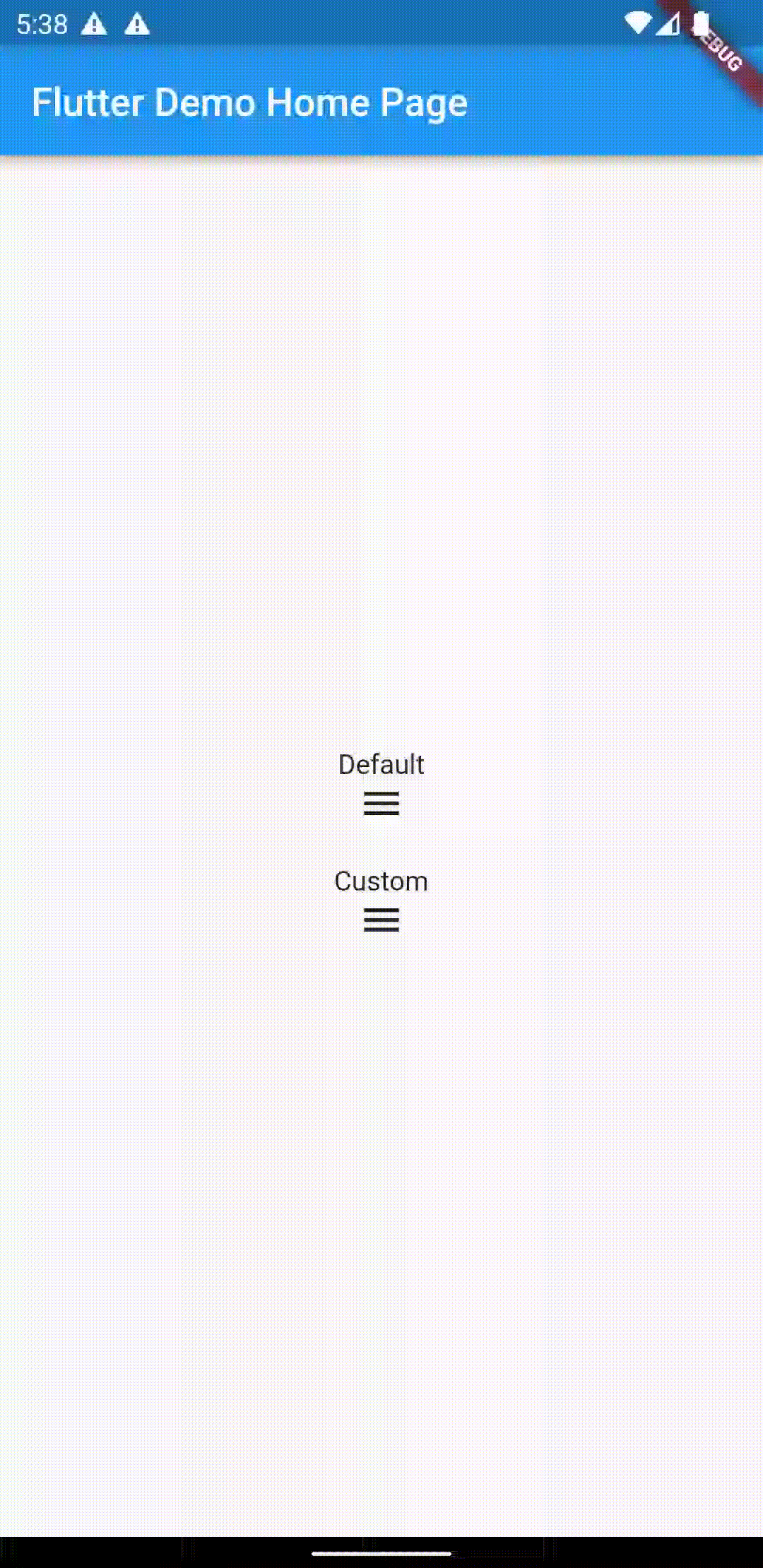
Add starlight_utils as dependency to your pubspec file.
starlight_utils:
git:
url: https://github.com/YeMyoAung/starlight_utils.git
No additional integration steps are required for Android and Ios.
At first, you need to import our package.
import 'package:starlight_utils/starlight_utils.dart';
And then you can use easily.
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
StarlightUtils.push(MyHome());///`Navigation.push()` shortcut.
StarlightUtils.pushNamed('home');///`Navigation.pushNamed()` shortcut.
StarlightUtils.pushNamedAndRemoveUntil('home');///`Navigation.pushNamedAndRemoveUntil()` shortcut.
StarlightUtils.pushAndRemoveUntil(MyHome());///`Navigation.pushAndRemoveUntil()` shortcut.
StarlightUtils.pushReplacement(MyHome());///`Navigation.pushReplacement()` shortcut.
StarlightUtils.pushReplacementNamed('home');///`Navigation.pushReplacement()` shortcut.
StarlightUtils.popAndPushNamed('home');///`Navigation.popAndPushNamed()` shortcut.
///If you used a context(like this(StarlightUtils.of(context))),
///you need to invoke like this StarlightUtils.pop(usedContext:true)
StarlightUtils.pop();///`Navigation.pop()` shortcut.
StarlightUtils.conPop();///`Navigation.conPop()` shortcut.
return MaterialApp(
navigatorKey: StarlightUtils.navigatorKey,///important
);
}
}
class MyApp xtends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: StarlightUtils.aboutDialog,
child: const Text(
"aboutDialog",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.dialog(AlertDialog(
title: Text("hello"),
));
},
child: const Text(
"dialog",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.bottomSheet(Container(
width: context.width,
height: 100,
child: Text("bottom sheet"),
));
},
child: const Text(
"bottomSheet",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.snackbar(SnackBar(content: Text('hello')));
},
child: const Text(
"snackbar",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
StarlightUtils.of(context);///important
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.datePicker(
initialDate: DateTime.now(),
firstDate: DateTime(2000),
lastDate: DateTime(2100),
);
},
child: const Text(
"datePicker",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
StarlightUtils.of(context);///important
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.timePicker(
initialTime: TimeOfDay.fromDateTime(
DateTime.now(),
),
);
},
child: const Text(
"timePicker",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
StarlightUtils.of(context);///important
return SizedBox(
width: context.width - 20,
height: 45,
child: ElevatedButton(
onPressed: () {
StarlightUtils.dateRangePicker(
firstDate: DateTime(2000),
lastDate: DateTime(2100),
);
},
child: const Text(
"dateRangePicker",
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
StarlightUtils.of(context);///important
return Scaffold(
appBar: AppBar(
actions: [
IconButton(
onPressed: () {
StarlightUtils.menu(
position: RelativeRect.fromLTRB(10, 0, 0, 0),
items: [
PopupMenuItem(child: Text('item 1')),
PopupMenuItem(child: Text('item 2')),
PopupMenuItem(child: Text('item 3')),
],
);
},
icon: Icon(Icons.more_vert),
)
],
),
);
}
}
class TestSocketScreen extends StatelessWidget {
const TestSocketScreen({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return StarlightEasyMenu(
childBuilder: (context, controller) => StreamBuilder<bool>(
initialData: false,
stream: stream,
builder: (_, snapshot) {
return AnimatedIcon(
icon: snapshot.data == true
? AnimatedIcons.menu_close
: AnimatedIcons.arrow_menu,
progress: kAlwaysCompleteAnimation,
);
},),
menuBuilder:(context, controller) => Container(
width: 200,
height: 120,
color: Colors.white,
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
ListTile(
onTap: controller.hideMenu,
title: Row(
children: const [
Icon(Icons.settings),
SizedBox(
width: 10,
),
Text("Setting"),
],
),
),
ListTile(
onTap: controller.hideMenu,
title: Row(
children: const [
Icon(Icons.help),
SizedBox(
width: 10,
),
Text("Help"),
],
),
),
],
),
),
);
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
print("Hello World".toValidate);///helloworld
print("a b c".withoutWhiteSpace);///abc
print("a@".isEmail);///false
print("password".isStrongPassword(
checkUpperCase:true,
checkLowerCase:true,
checkDigit:true,
checkSpecailChar:true,
minLength:6
));///null or requirement
final File file = "file_path".file;///File Instance
final Uri uri = "http://url.com".uri;///Uri Instance
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
print(1.ordinal);///1st (String)
print(2.ordinal);///2nd (String)
print(100000.currencyFormat);///100,000 (String)
print(1000.humanReadAble(useLowerCase:true));///1k (String)
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
print(["Starlight Studio",{"name":"Starlight Studio"}].equal(["Starlight Studio",{"name":"Starlight Studio"}]));///true
print([1,2,3,4,5,6].chunk(2));///[[1,2],[3,4],[5,6]]
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
print({"name":"Starlight Studio",
"in_list":[
"Starlight Studio"]
}.equal({"name":"Starlight Studio",
"in_list":[
"Starlight Studio"]
}));///true
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
10.w(context);/// Returns a calculated width based on the device
10.h(context);/// Returns a calculated height based on the device
3.sp(context);///Returns a calculated sp based on the device
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
context.with;///device width
context.height;///device height
context.devicePixelRatio;///device pixel ratio
context.textScaleFactor;///text scale factor
context.topSafe;///Appbar height
context.bottomSafe;///bottom navigationbar height
context.orientation;///Device orientation
context.invertColors;///is inverting Colors or not
context.highContrast;///is highContrast or not
context.gestureSettings;///gesture settings
context.boldText;///is bold text or not
context.alwaysUse24HourFormat;///is using 24 hour format or not
context.accessibleNavigation;///is accessible navigation or not
context.viewInsets;//ToHandle Keyboard is show or hide
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
print(DateTime.now().monthformat(MonthFormat.long));///February
print(DateTime.now().monthformat(MonthFormat.short));///Feb
print(DateTime.now().monthformat(MonthFormat.ordinal));///2nd
print(DateTime.now().monthformat(MonthFormat.none));///2
print(DateTime.now().isLeapYear);///false
print(DateTime.now().maxDay);///28
print(DateTime(2022, 2, 2).differenceTimeInString(DateTime(2022, 2, 1)));//24h
return Container();
}
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
context.theme;///ThemeData
return Container();
}
}