Home

Modern Telegram Bot API framework for App Script
A Telegram bot is a program that offers functions and automations that Telegram users can integrate in their chats, channels, or groups
Telesun is a library that makes it simple for you to develop your own Telegram bots using JavaScript and Apps Script
- Full Telegram Bot API 7.0 support
- Simpler 🌟
- easier working across Google products like
Youtube
,Drive
,Gmail...
- per click
Deployment
on google cloud -
Real-Time Database
(Google sheet) already integrated - Develop
100+
of your Bots -
Basic Javascript
is enough - Fully
Typed
Support forAutocompletion
DevHook
-
Long Polling
Support
function initializeTelesunBot() {
const telesun = new Telesun.config({
telegram: '7172550********sLAVw'
});
// Default handler for any incoming messages.
telesun.use((ctx) => ctx.reply("Welcome to our service!"));
// Handler for the `/start` command.
telesun.start((ctx) => {
ctx.reply("Welcome! Ready to explore?");
});
// Specific handler for text messages containing "hello".
telesun.hears("hello", (ctx) =>
ctx.reply("Hello there! How can I assist you today?")
);
// Handler for incoming photos.
telesun.photo((ctx) => ctx.replyWithPhoto("<photo-url|photo-id>"));
// Inline query handler.
telesun.on("inline_query", (ctx) => {
ctx.answerInlineQuery(ctx.inlineQuery.id, []);
});
}
function demonstrateAdvancedFeatures() {
// Advanced setup with spreadsheet connection and memory services.
const telesun = new Telesun.config({
telegram: '7172***********w',
spreadSheetId: "1CWOW*************Xkvhw",
pmemory: PropertiesService.getScriptProperties(),
tmemory: CacheService.getScriptCache()
});
telesun.on("message", (ctx) => {
// Handling message updates.
const update = ctx.update.message; // Correct method to access the message update.
// Clearing a specified sheet.
ctx.sheet("Sheet1").clear();
// Preparing for the next interaction stage.
ctx.setStage("message");
// Sending an email notification.
ctx.sendEmail(
"email@example.com", // Use a placeholder or generic email for examples.
"Telesun V2-beta Released",
"We're excited to announce the release of Telesun V2-beta. Join us in enhancing and refining the documentation."
);
// Setting a temporary session value (with a default lifespan of 10 minutes).
ctx.tSession.setValue("key", "value");
});
}
For additional bot examples see examples folder
To use the Telegram Bot API, you first have to get a bot account by chatting with BotFather.
BotFather will give you a token, something like 123456789:AbCdfGhIJKlmNoQQRsTUVwxyZ
.
Welcome to this exciting tutorial where we'll embark on the journey of creating a Gif Inline Query
bot. This isn't just any bot; it's your gateway to integrating the dynamic and fun world of GIFs right into any chat. Picture this: users type, scroll, and BAM! - an endless stream of GIFs at their fingertips. And guess what? We're tapping into the vast universe of GIPHY API to power our search for those perfect GIFs.
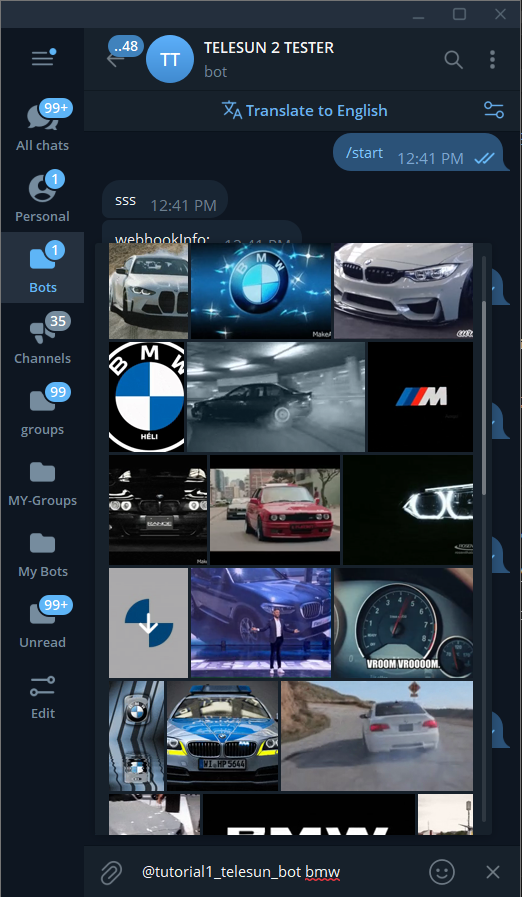
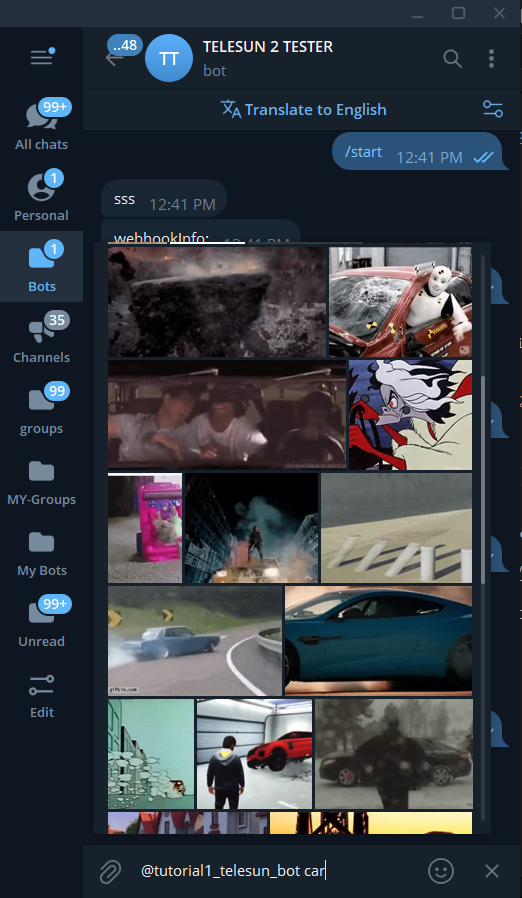
Features to Get Excited About:
- Instant Gratification: Just by mentioning the bot's username in the text area, you'll unleash a world of trending GIFs, loading automatically to greet the user.
- Search on the Fly: As users start typing their queries, our bot springs into action, fetching GIFs that match their search terms in real time.
- Infinite Scrolling Magic: As users delve deeper, scrolling through the GIFs, more and more GIFs magically appear, ensuring the fun never ends.
💪 Ready to dive in? I sure am. Let's get this show on the road and create a bot that brings smiles, laughs, and a whole lot of GIFs to any conversation.
Get Started
- Create an App Script Project: Watch Tutorial
- Import Telesun Libraries: Learn How
- Craft Your Bot: Begin Here
Activate Inline Queries with Bot Father
- Type
/mybots
to Bot Father.- Choose your bot.
- Navigate to
Bot Settings
>Inline Mode
.- Enable it.
create a function, name it anything you like, but for production purposes, the function name must be doPost
.
function doPost() {
// Bot initialization with your token.
const telesun = new Telesun.config({
telegram: '7172550********sLAVw'
});
// handles inline_query updates.
telesun
.on("inline_query", (ctx) => {
// Your code here.
})
.longPolling(); // Maintains open connection for new updates.
}
Now, let's secure our API key from GIPHY API to access trending
and search
endpoints. Below is how we fetch GIFs:
// Fetch GIFs from Giphy, tailored by your query.
function fetchGifs(
apiKey,
query,
offset = 0,
limit = 20,
rating = "g",
lang = "en"
) {
let baseUrl = "https://api.giphy.com/v1/gifs"; // Base endpoint.
const queryParams = `api_key=${apiKey}&limit=${limit}&offset=${offset}&rating=${rating}&lang=${lang}`;
// Adjust endpoint based on query presence.
baseUrl += query
? `/search?q=${encodeURIComponent(query)}&${queryParams}`
: `/trending?${queryParams}`;
const response = UrlFetchApp.fetch(baseUrl); // Fetch the data.
const json = JSON.parse(response.getContentText()); // Parse the JSON.
return json.data; // Return the GIFs.
}
To respond to inline queries, our bot employs the answerInlineQuery
method. This essential method requires parameters such as an array of results
and the inline_query_id
.
Each result
object must include crucial details like type
, id
, gif_url
, and thumbnail_url
.
// Function to format GIFs for Telegram inline query response
function formatGifsForInlineQuery(gifs) {
return gifs.map((gif) => ({
type: "gif",
id: gif.id,
gif_url: gif.images.fixed_height.url,
thumbnail_url: gif.images.fixed_height_small_still.url,
}));
}
function doPost() {
// Initialize the bot with your token.
const telesun = new Telesun.config({
telegram: '7172550********sLAVw'
});
// Handle inline_query updates.
telesun
.on("inline_query", (ctx) => {
const inline_query = ctx.inlineQuery();
const query = inline_query.query;
const offset = parseInt(inline_query.offset, 10) || 0; // Parse the offset, defaulting to 0 if absent.
const apiKey = "<your-giphy-api-key>";
const limit = 20; // Determine the number of results to fetch.
// Fetch GIFs based on the user's query and the current offset.
const gifs = fetchGifs(apiKey, query, offset, limit);
const results = formatGifsForInlineQuery(gifs);
// Reply to the inline query with the fetched results and set the next offset.
const nextOffset = offset + limit;
ctx.answerInlineQuery(inline_query.id, results,{
next_offset: nextOffset.toString(),
});
})
.longPolling(); // Maintain the connection for incoming updates.
}
Integrate the parameter e
into both doPost(e)
and handleWebhook(e)
to elevate our code for production.
function doPost(e) {
// Initialize the bot with your token.
const telesun = new Telesun.config({
telegram: '7172550********sLAVw'
});
// Handle inline_query updates.
telesun
.on("inline_query", (ctx) => {
const inline_query = ctx.inlineQuery();
const query = inline_query.query;
const offset = parseInt(inline_query.offset, 10) || 0; // Parse the offset, defaulting to 0 if absent.
const apiKey = "<your-giphy-api-key>";
const limit = 20; // Determine the number of results to fetch.
// Fetch GIFs based on the user's query and the current offset.
const gifs = fetchGifs(apiKey, query, offset, limit);
const results = formatGifsForInlineQuery(gifs);
// Reply to the inline query with the fetched results and set the next offset.
const nextOffset = offset + limit;
ctx.answerInlineQuery(inline_query.id, results, {
next_offset: nextOffset.toString(),
});
})
.handleWebhook(e);
}
Our GIF inline query bot is now primed for launch. The upcoming section will guide you through deploying
your project to the world.
Ready to bring your bot to life? Just follow these simple steps:
- Ensure your primary script is named
code.gs
.- Verify that
doPost(e)
is set as your main function.
Next, deploy your code to obtain the Web App URL
. Not sure how? Watch this quick guide:
After deploying your code and securing your Web App URL
, execute the following:
function SetWebhook() {
const telesun = new Telesun.config({ telegram: <your-bot-token> });
telesun.setWebhook({ URL: <your-webhook-url> });
}
🌠 That's it! Dive in and enjoy interacting with your bot. 🎉
- Telesun
- Getting Started - Apps script
- Getting Started with the Telesun Ready Template
- Import Telesun
- Telesun-Handlers
- Context Object
- Next Middleware
- Stages
- Manager Classes:
- Mail:
- Google Spreadsheet:
- Best Practice:
- Getting Updates:
- Example Types:
- Example Bots: