You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
int res = 0;
Stack<Integer> stack = new Stack<Integer>();
int index = 0;
while (index < height.length) {
while (stack.size() > 0 && height[index] > height[stack.peek()]) {
//数组当前index下值>数组中栈顶元素对应值----》 确定一组边界
int top = stack.pop(); //保存并弹出栈顶元素
if (stack.empty()) {
break;
}
int h = Math.min(height[index], height[stack.peek()]) - height[top];
//长度差=min(数组中index下值,数组中当前栈顶下值)-数组中当旧栈顶(top)下值
//旧栈顶对应的一组边界的面积已经在旧栈顶入栈时计算,所以去和原栈顶的长度差??
int d = index - stack.peek() - 1; // 宽度=数组坐标差
res = res + h * d;
}
stack.push(index++);
}
return res;
Week01总结:
数组
随机访问的时间复杂度为 O(1)
如果在数组的末尾插入元素,复杂度为 O(1)
如果在开头 ,复杂度为 O(n)
删除同插入
旋转数组
//挂起本次目标位元素
//降前一轮挂起元素 放入目标位
//目标位 = (起始位+ 移动位数) 同 长度 取模
梳理逻辑的草图@_@
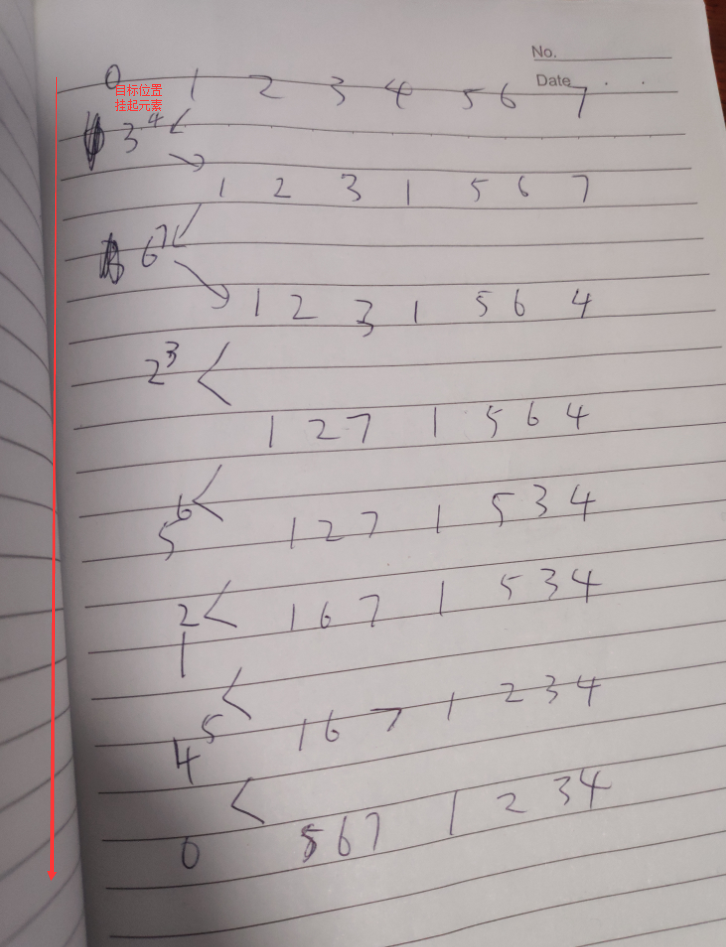
后进先出,简称LIFO结构
peek() 取得栈顶
pop() 取得栈顶并从栈中移除栈顶
push(E item) 入栈
接雨水
`public int trap(int[] height) {
}`
草图@_@
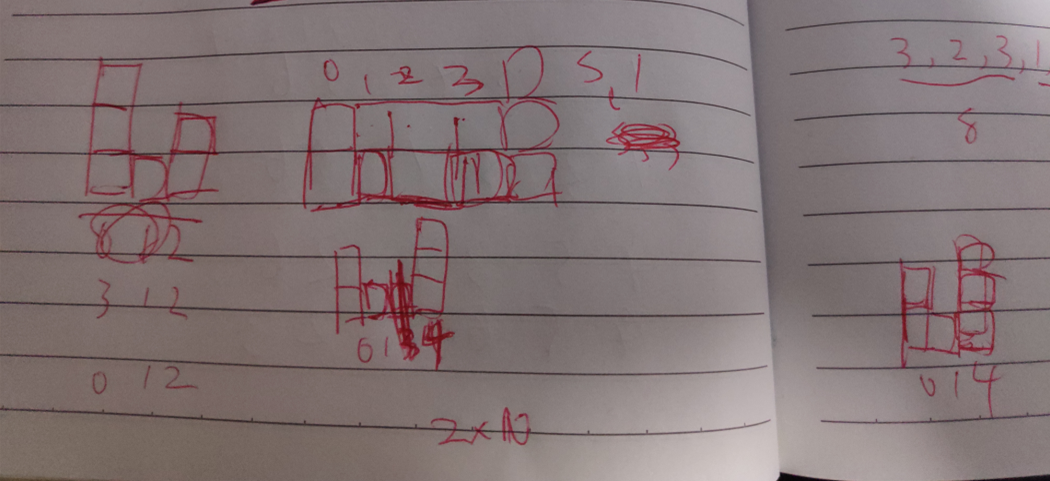
PriorityQueue 优先队列 有优先顺序的queue
poll() 取得并移除队列头部元素
peek() 取得但不移除队列头部元素
add() ,offer()
语义相同,都是向优先队列中插入元素 ,并按自然顺序或比较器Comparator的顺序排序
offer 插入失败时返回false
add插入失败时返回异常
插入或移除元素时 会对队列进行扩容 或减小size 并调用siftUp,siftDown重新调整排序
The text was updated successfully, but these errors were encountered: