-
Notifications
You must be signed in to change notification settings - Fork 239
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[RFC]: DataStore category for Amplify Flutter #160
Comments
Amplify DataStore for flutter is now available as a developer preview. Get started here |
I started integrating Amplify DataStore to my flutter project but there is a mismatch between what version of CLI to use for codegen Flutter preview suggests to use But
As |
@NimaSoroush you do not need the preview tag any more. You can go ahead and use
I'll make a PR to update the docs. Thanks for catching this! |
@NimaSoroush Can you please create a separate bug report for that issue, and remove it from this RFC? This is more discussing roadmap. |
This issue is a Request For Comments (RFC). It is intended to elicit community feedback regarding support for Amplify library in Flutter platform. Please feel free to post comments or questions here.
Purpose
This RFC is in continuation of this and expands on DataStore category and discusses few approaches being proposed.
Proposed Solution
High Level Overview
Of all the Amplify categories, Datastore is the trickiest due to the use of auto-generated (codegen) models that reside in the client’s application. The models are used when making Datastore calls such as
query(Model.Type)
,save(Model())
, anddelete(Model())
, where Model is a codegen type.As mentioned in the overview RFC, one goal of Amplify Flutter is to reuse as much of the native Amplify libraries as possible, and to write only the minimum high-level API code in Dart, that Flutter developers can call from their Dart code. Hence in the Datastore category, our goal is to codegen user models in Dart, such that developers can write queries and mutations against it from Dart.
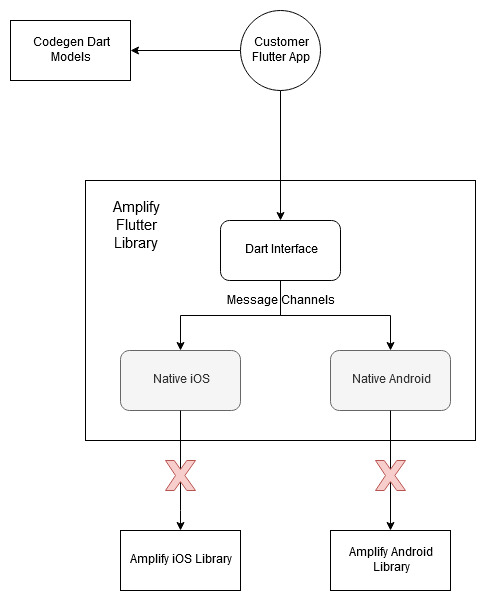
The problem arises when the request crosses over from Flutter/Dart to Native (iOS and android). The request is serialized at the flutter end and sent as a binary message to the native end of the Amplify flutter library. This means that there are no static types available on the native end of the Amplify flutter library to call Amplify Native library APIs iOS and android
The proposal here is that we will add support for calling native libraries' DataStore APIs that will accept
ModelSchema
as input rather than concrete Model Types. Find the supporting android RFC here.DataStore APIs
Query
It’s complicated to mimic the exact behavior of query API of iOS and Android in flutter because of Dart language limitations. For instance, Dart Type objects are not generic (i.e. Type<? extends Model>) and it cannot be used to instantiate objects. To workaround this, we will create another generic “type” class ModelType to encapsulate the model types.
API Proposed
DX
Save
API Proposed
DX
delete
delete API is tricky since we have two distinct uses cases to support in this API but dart doesn't support method overloading.
Instead of cramming everything in one
delete()
API we are proposing two APIs for each use case.API Proposed
DX
clear
API Proposed
DX
observe
Currently there is no way to dynamically create an eventChannel (https://api.flutter.dev/flutter/services/EventChannel-class.html) between dart and native platform plugins so it’s not possible to create a new event channel for each subscription or even for every model (unless we codegen the bridge code)
Rather we will create only one data streaming channel for communicating all the datastore subscriptions. Both android and iOS flutter platform plugins will subscribe to all Models and transmit over a single EventChannel() i.e. all subscriptions for all models will be transmitted over this event channel. The filtering byId and by modelType will happen in Dart side of flutter (ok, some pun intended).
API Proposed
DX
Appendix 1
Sample model that will be codegen by Amplify based on user's graphql schema
DX
The text was updated successfully, but these errors were encountered: