-
Notifications
You must be signed in to change notification settings - Fork 102
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Removing false branches #11
Comments
Ok so i've made this: git diffdiff --git a/src/helpers/traversalHelper.ts b/src/helpers/traversalHelper.ts
index 135dc29..a441744 100644
--- a/src/helpers/traversalHelper.ts
+++ b/src/helpers/traversalHelper.ts
@@ -9,7 +9,7 @@ export default class TraversalHelper {
* @param node The node to replace.
* @param replacement The replacement node.
*/
- static replaceNode(root: Shift.Node, node: Shift.Node, replacement: Shift.Node | null): void {
+ static replaceNode(root: Shift.Node, node: Shift.Node, replacement: Shift.Node | null | Array<Shift.Node>): void {
traverse(root, {
enter(n: Shift.Node, parent: Shift.Node) {
if (n == node) {
@@ -22,7 +22,11 @@ export default class TraversalHelper {
const index = array.indexOf(node);
if (index != -1) {
if (replacement) {
- array[index] = replacement;
+ if (Array.isArray(replacement)){
+ array.splice(index, 1, ...replacement);
+ } else {
+ array[index] = replacement;
+ }
} else {
array.splice(index, 1);
}
diff --git a/src/index.ts b/src/index.ts
index c4fbf64..a0d4ca7 100644
--- a/src/index.ts
+++ b/src/index.ts
@@ -10,6 +10,7 @@ import CleanupHelper from './helpers/cleanupHelper';
import Config from './config';
import VariableRenamer from './modifications/renaming/variableRenamer';
import FunctionExecutor from './modifications/execution/functionExecutor';
+import BlockRemover from './modifications/blockRemover';
export function deobfuscate(source: string, config: Config): string {
const ast = parseScript(source) as Shift.Script;
@@ -42,6 +43,8 @@ export function deobfuscate(source: string, config: Config): string {
if (config.miscellaneous.renameHexIdentifiers) {
modifications.push(new VariableRenamer(ast));
}
+ modifications.push(new BlockRemover(ast));
+
for (const modification of modifications) {
console.log(`[${(new Date()).toISOString()}]: Executing ${modification.constructor.name}`);
diff --git a/src/modifications/blockRemover.ts b/src/modifications/blockRemover.ts
new file mode 100644
index 0000000..29dd7d4
--- /dev/null
+++ b/src/modifications/blockRemover.ts
@@ -0,0 +1,64 @@
+import Modification from "../modification";
+import * as Shift from 'shift-ast';
+import isValid from 'shift-validator';
+import { traverse } from '../helpers/traverse';
+import TraversalHelper from "../helpers/traversalHelper";
+
+export default class BlockRemover extends Modification {
+ /**
+ * Creates a new modification.
+ * @param ast The AST.
+ */
+ constructor(ast: Shift.Script) {
+ super('Remove false blocks', ast);
+ }
+
+ /**
+ * Executes the modification.
+ */
+ execute(): void {
+ this.removeBlocks(this.ast);
+ }
+
+
+ private removeBlocks(node: Shift.Node): void {
+ const self = this;
+
+ traverse(node, {
+ enter(node: Shift.Node, parent: Shift.Node) {
+ if (node.type != "IfStatement" && node.type != "ConditionalExpression"){
+ return;
+ }
+ if ((node as any).test.type != "LiteralBooleanExpression"){
+ return;
+ }
+
+ let replacement = self.getTrueBranch(node);
+ if (replacement){
+ self.removeBlocks(replacement);
+ TraversalHelper.replaceNode(parent, node, self.extractStatements(replacement));
+ } else {
+ TraversalHelper.replaceNode(parent, node, replacement);
+ }
+ }
+ })
+ }
+ private getTrueBranch(node: Shift.IfStatement | Shift.ConditionalExpression): Shift.Node | null {
+ let branch;
+ if ((node.test as Shift.LiteralBooleanExpression).value) {
+ return node.consequent;
+ } else {
+ return node.alternate;
+ }
+ return null;
+ }
+
+ private extractStatements(node: Shift.Node): Shift.Node | Array<Shift.Node> {
+ switch (node.type) {
+ case 'BlockStatement':
+ return node.block.statements;
+ default:
+ return node;
+ }
+ }
+} it's my first time coding typescript and second time seeing it at all. it seems to work fine but it needs a couple of runs sample code: if (true) {
console.log("hello there")
true ? console.log(1) : console.log(2)
if (true) {
if (false) {
console.log(44)
}
console.log(3)
} else {
console.log(6)
}
} else {
console.log(2+2)
console["log"](2+2)
}
console.log(99) deobfuscated: console.log("hello there");
console.log(1);
console.log(3);
console.log(99); |
Have implemented this in a similar approach in 3bb12a3 Your code was pretty spot on, especially for someone who doesn't know TypeScript, thanks for contributing :) |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Hello! Thank you for making this cool tool.
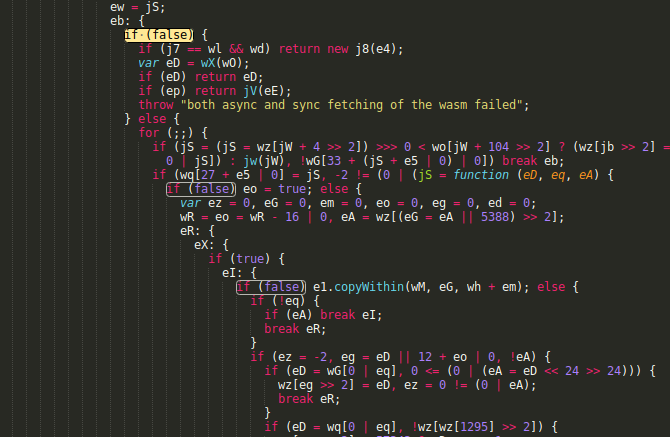

i'm using https://deobfuscate.io/
after deobfuscating other parts of an obfuscator i'm left with a lot of true and false branches like this:
don't forget ternary operators too!
sample code:
deobfuscated with https://deobfuscate.io/
The text was updated successfully, but these errors were encountered: