You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
I have a few issues using the keyboard actions. I have created a simple startup project that extends from your main sample - my app is much more complex and has a deep nesting of scrollable widgets but I have tried to abstract that functionality away by creating a small app that shows the issue. Hopefully I am missing something very basic.
Note
If autoscroll is turned off then for the simple use case below, keyboard actions functions - however - within our own live app which has many scrollable widgets nested deeply - we need to enable autoscroll on a per case basis.
Additionally - you will see that we have code such as :
showBody() {
List<Widget> list = [];
// Run with keyboard actions causes issues (Uncomment)
list.add(
FormKeyboardActions(
child: TextField2(),
),
);
Which adds one or more widgets encased within a FormKeyboardActions - in our app, the user can create as many TextField widgets as they desire - each textfield are multilined and can contain any number of lines. Scrolling between these textFields is important.
Here is the app running without using Keyboard Actions
From the gif above, we can see that the on screen keyboard allows newline entries and that we have a multiline textfield. Everything works perfectly except there is no close button (Hence the desire to use keyboard Action)
We are wrapping a TextField in a custom widget :
TextField2 (Which uses KeyboardAction)
TextField3 (which doesnt use KeyboardAction)
Build method showing how these widgets are used
Widget build(BuildContext context) {
return Scaffold(
resizeToAvoidBottomInset: false,
appBar: AppBar(title: Text('keyboardActions')),
body: showBody());
}
showBody() {
List<Widget> list = [];
// Run with keyboard actions causes issues (Uncomment)
// list.add(
// FormKeyboardActions(
// child: TextField2(
// ),
// ),
// );
// Run without keyboard actions shows the text field
list.add(
TextField3(),
);
return Column(
children: list,
);
}
Running the app with keyboard Actions causes exception
I was thinking I could use this in normal composition terms and simply decorate the Widget at the place of use with a FormKeyboardAction ... but the approach to place the FormkeyboardAction in a higher container widget works in my use case ....
I have a few issues using the keyboard actions. I have created a simple startup project that extends from your main sample - my app is much more complex and has a deep nesting of scrollable widgets but I have tried to abstract that functionality away by creating a small app that shows the issue. Hopefully I am missing something very basic.
Note
If autoscroll is turned off then for the simple use case below, keyboard actions functions - however - within our own live app which has many scrollable widgets nested deeply - we need to enable autoscroll on a per case basis.
Additionally - you will see that we have code such as :
Which adds one or more widgets encased within a FormKeyboardActions - in our app, the user can create as many TextField widgets as they desire - each textfield are multilined and can contain any number of lines. Scrolling between these textFields is important.
Here is the app running without using Keyboard Actions
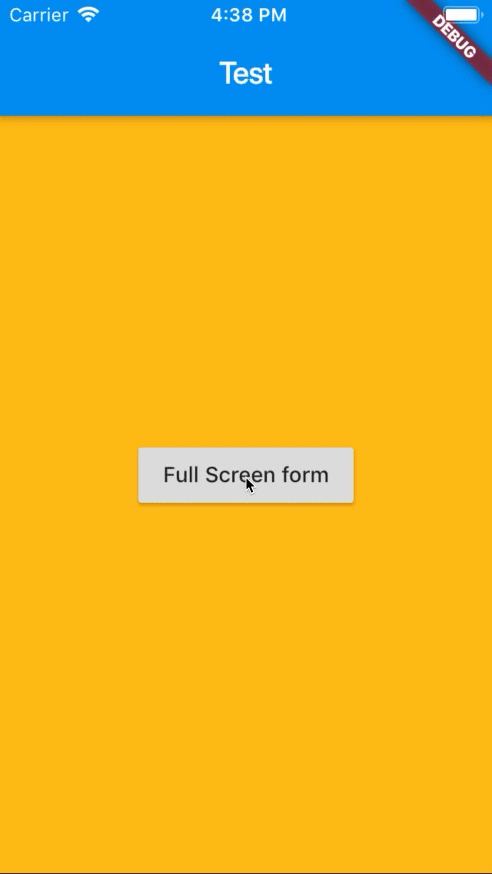
From the gif above, we can see that the on screen keyboard allows newline entries and that we have a multiline textfield. Everything works perfectly except there is no close button (Hence the desire to use keyboard Action)
We are wrapping a TextField in a custom widget :
TextField2 (Which uses KeyboardAction)
TextField3 (which doesnt use KeyboardAction)
Build method showing how these widgets are used
Running the app with keyboard Actions causes exception
Source
Two small simple files :
Main.dart
TextField2
The text was updated successfully, but these errors were encountered: