-
-
Notifications
You must be signed in to change notification settings - Fork 1.1k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
ThemeContext missing for external components #1107
Comments
Maybe your library is trying to use other Provider? Can't know for sure though without runnable reproduction. |
Unfortunately I'm not sure of a way to do it on Codesandbox . I would have to have the ability to create multiple projects and link them. Is uploading source code with instructions frowned upon? I'm aware it's certainly not ideal, but I don't know how to reproduce with the online tools I'm familiar with. As for the the library using another Provider -- wouldn't a nested ThemeProvider simply overwrite whatever theme was from the context(s) above it? I'm positive I'm not wrapping a ThemeProvider when exporting the component and only once in the consuming application. |
While codesandbox is preferred because it's just so easy to use you can also prepare sample repositories and list steps to setup them.
Not necessarily. If they have different identities then they won't shadow each other. |
Thanks for the response, I have added a reproduction of the issue as well as instructions to a git repository. |
https://github.com/btoles/emotion-issue/pull/1 The problem is that when you install in You'd have to figure out how to work around this the best way in your case, you could ie:
|
I appreciate the help in diagnosing the problem, unfortunately I was unable to get it working even with 2 of your 3 suggestions. We are using create-react-app at the moment and don't plan to eject so the third option isn't viable for us quite yet. I'm not sure what changed with version 10 but this was a non-issue in version 9 and we'll probably stick with that. |
Hm, peerDep and removing as postlink step were just ideas and it seems they dont work because the symlink gets resolved and it doesnt want to traverse up to the "host" app node_modules. U dont have to eject - u could use i.e. https://github.com/timarney/react-app-rewired . I understand that you might be hesitant to that though.
Emotion@9 was using legacy context which has used strings to identify which context it should use, emotion@10 is using new context API which is identity based, basically what happens is similar to this: const noop1 = () => {}
const noop2 = () => {}
noop1 === noop2 // false React just cant match those contexts because they are created by 2 "different" copies of emotion in your setup. The same thing would happen with any other context-based library (i.e. react-router). |
Hey @Andarist could you elaborate on the webpack alias approach? Currently we have a project structure similar to the one above
In
I use
Everything builds fine, but at runtime I get this error
I have not created a git repository to replicate as it seems similar to the issue above, but can try to in case it's required. |
Hey @HiranmayaGundu, I finally figured out my issue, which may be yours as well. Going back to what @Andarist said, the newer Context API is identity based. The solution was to export the Use the |
Hey @Btoles! Thanks, will try that out! 😀 So from my understanding, we can have only one version of |
Follow up, learned more. The problem was indeed two versions of the theming library. Required: // package.json
// preferred if publishing library
{
"peerDependencies": {
"emotion-theming": "x.x.x"
}
} Using rollup: // rollup.config.js
export default {
external: ['emotion-theming']
} Using webpack for bundling a library: // webpack.config.js
module.exports = {
externals: {
'emotion-theming': 'commonjs2 emotion-theming'
}
} Using webpack for bundling an application: // webpack.config.js
const path = require('path');
module.exports = {
//...
resolve: {
alias: {
// point to the common version of the theming library
'emotion-theming': path.resolve(__dirname, 'node_modules/emotion-theming')
}
}
}; |
External Component
emotion
version: 10.0.2react
version: 16.4.2Consuming Application
emotion
version: 10.0.2react
version: 16.6.3What happened:
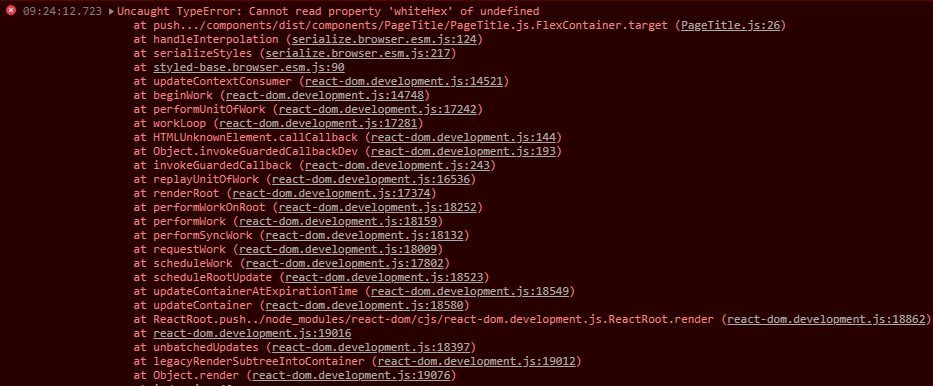
Reproduction:
At this point the local component of your application should pick up the ThemeContext while the imported component will have an empty object for theme.
Problem description:
The component from our external library does not get the ThemeContext from the ThemeProvider and falls back to the default empty object. This was working prior to upgrading to emotion 10.x.x and worked sporadically using 10.x.x
The theme is also imported from our component library and can be used normally with
styled
components that are creating within the consuming application but it will not apply to the components that are imported en tandem with the theme.The text was updated successfully, but these errors were encountered: