Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Added CHANGELOG and READMEs for DevTools v4 NPM packages (#16404)
- Loading branch information
Brian Vaughn
committed
Aug 15, 2019
1 parent
868d02d
commit 6edff8f
Showing
4 changed files
with
430 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,44 @@ | ||
# `react-devtools-core` | ||
|
||
A standalone React DevTools implementation. | ||
|
||
This is a low-level package. If you're looking for the Electron app you can run, **use `react-devtools` package instead.** | ||
|
||
## API | ||
|
||
### `react-devtools-core` | ||
|
||
This is similar requiring the `react-devtools` package, but provides several configurable options. Unlike `react-devtools`, requiring `react-devtools-core` doesn't connect immediately but instead exports a function: | ||
|
||
```js | ||
const { connectToDevTools } = require("react-devtools-core"); | ||
connectToDevTools({ | ||
// Config options | ||
}); | ||
|
||
``` | ||
|
||
Run `connectToDevTools()` in the same context as React to set up a connection to DevTools. | ||
Be sure to run this function *before* importing e.g. `react`, `react-dom`, `react-native`. | ||
|
||
The `options` object may contain: | ||
* `host: string` (defaults to "localhost") - Websocket will connect to this host. | ||
* `port: number` (defaults to `8097`) - Websocket will connect to this port. | ||
* `websocket: Websocket` - Custom websocked to use. Overrides `host` and `port` settings if provided. | ||
* `resolveNativeStyle: (style: number) => ?Object` - Used by the React Native style plug-in. | ||
* `isAppActive: () => boolean` - If provided, DevTools will poll this method and wait until it returns true before connecting to React. | ||
|
||
## `react-devtools-core/standalone` | ||
|
||
Renders the DevTools interface into a DOM node. | ||
|
||
```js | ||
require("react-devtools-core/standalone") | ||
.setContentDOMNode(document.getElementById("container")) | ||
.setStatusListener(status => { | ||
// This callback is optional... | ||
}) | ||
.startServer(port); | ||
``` | ||
|
||
Reference the `react-devtools` package for a complete integration example. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,146 @@ | ||
# `react-devtools-inline` | ||
|
||
React DevTools implementation for embedding within a browser-based IDE (e.g. [CodeSandbox](https://codesandbox.io/), [StackBlitz](https://stackblitz.com/)). | ||
|
||
This is a low-level package. If you're looking for the standalone DevTools app, **use the `react-devtools` package instead.** | ||
|
||
## Usage | ||
|
||
This package exports two entry points: a frontend (to be run in the main `window`) and a backend (to be installed and run within an `iframe`<sup>1</sup>). | ||
|
||
The frontend and backend can be initialized in any order, but **the backend must not be activated until after the frontend has been initialized**. Because of this, the simplest sequence is: | ||
|
||
1. Frontend (DevTools interface) initialized in the main `window`. | ||
1. Backend initialized in an `iframe`. | ||
1. Backend activated. | ||
|
||
<sup>1</sup> Sandboxed iframes are supported. | ||
|
||
## API | ||
|
||
### `react-devtools-inline/backend` | ||
|
||
* **`initialize(contentWindow)`** - | ||
Installs the global hook on the window. This hook is how React and DevTools communicate. **This method must be called before React is loaded.** (This means before any `import` or `require` statements!) | ||
* **`activate(contentWindow)`** - | ||
Lets the backend know when the frontend is ready. It should not be called until after the frontend has been initialized, else the frontend might miss important tree-initialization events. | ||
|
||
```js | ||
import { activate, initialize } from 'react-devtools-inline/backend'; | ||
|
||
// Call this before importing React (or any other packages that might import React). | ||
initialize(); | ||
|
||
// Call this only once the frontend has been initialized. | ||
activate(); | ||
``` | ||
|
||
### `react-devtools-inline/frontend` | ||
|
||
* **`initialize(contentWindow)`** - | ||
Configures the DevTools interface to listen to the `window` the backend was injected into. This method returns a React component that can be rendered directly<sup>2</sup>. | ||
|
||
```js | ||
import { initialize } from 'react-devtools-inline/frontend'; | ||
|
||
// This should be the iframe the backend hook has been installed in. | ||
const iframe = document.getElementById(frameID); | ||
const contentWindow = iframe.contentWindow; | ||
|
||
// This returns a React component that can be rendered into your app. | ||
// <DevTools {...props} /> | ||
const DevTools = initialize(contentWindow); | ||
``` | ||
|
||
<sup>2</sup> Because the DevTools interface makes use of several new React APIs (e.g. suspense, concurrent mode) it should be rendered using either `ReactDOM.unstable_createRoot` or `ReactDOM.unstable_createSyncRoot`. It should not be rendered with `ReactDOM.render`. | ||
|
||
## Examples | ||
|
||
### Configuring a same-origin `iframe` | ||
|
||
The simplest way to use this package is to install the hook from the parent `window`. This is possible if the `iframe` is not sandboxed and there are no cross-origin restrictions. | ||
|
||
```js | ||
import { | ||
activate as activateBackend, | ||
initialize as initializeBackend | ||
} from 'react-devtools-inline/backend'; | ||
import { initialize as initializeFrontend } from 'react-devtools-inline/frontend'; | ||
|
||
// The React app you want to inspect with DevTools is running within this iframe: | ||
const iframe = document.getElementById('target'); | ||
const { contentWindow } = iframe; | ||
|
||
// Installs the global hook into the iframe. | ||
// This must be called before React is loaded into that frame. | ||
initializeBackend(contentWindow); | ||
|
||
// React application can be injected into <iframe> at any time now... | ||
// Note that this would need to be done via <script> tag injection, | ||
// as setting the src of the <iframe> would load a new page (withou the injected backend). | ||
|
||
// Initialize DevTools UI to listen to the hook we just installed. | ||
// This returns a React component we can render anywhere in the parent window. | ||
const DevTools = initializeFrontend(contentWindow); | ||
|
||
// <DevTools /> interface can be rendered in the parent window at any time now... | ||
// Be sure to use either ReactDOM.unstable_createRoot() | ||
// or ReactDOM.unstable_createSyncRoot() to render this component. | ||
|
||
// Let the backend know the frontend is ready and listening. | ||
activateBackend(contentWindow); | ||
``` | ||
|
||
### Configuring a sandboxed `iframe` | ||
|
||
Sandboxed `iframe`s are also supported but require more complex initialization. | ||
|
||
**`iframe.html`** | ||
```js | ||
import { activate, initialize } from "react-devtools-inline/backend"; | ||
|
||
// The DevTooks hook needs to be installed before React is even required! | ||
// The safest way to do this is probably to install it in a separate script tag. | ||
initialize(window); | ||
|
||
// Wait for the frontend to let us know that it's ready. | ||
function onMessage({ data }) { | ||
switch (data.type) { | ||
case "activate-backend": | ||
window.removeEventListener("message", onMessage); | ||
|
||
activate(window); | ||
break; | ||
default: | ||
break; | ||
} | ||
} | ||
|
||
window.addEventListener("message", onMessage); | ||
``` | ||
|
||
**`main-window.html`** | ||
```js | ||
import { initialize } from "react-devtools-inline/frontend"; | ||
|
||
const iframe = document.getElementById("target"); | ||
const { contentWindow } = iframe; | ||
|
||
// Initialize DevTools UI to listen to the iframe. | ||
// This returns a React component we can render anywhere in the main window. | ||
// Be sure to use either ReactDOM.unstable_createRoot() | ||
// or ReactDOM.unstable_createSyncRoot() to render this component. | ||
const DevTools = initialize(contentWindow); | ||
|
||
// Let the backend know to initialize itself. | ||
// We can't do this directly because the iframe is sandboxed. | ||
// Only initialize the backend once the DevTools frontend has been initialized. | ||
iframe.onload = () => { | ||
contentWindow.postMessage( | ||
{ | ||
type: "activate-backend" | ||
}, | ||
"*" | ||
); | ||
}; | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,142 @@ | ||
# React DevTools changelog | ||
|
||
<!-- ## [Unreleased] | ||
<details> | ||
<summary> | ||
Changes that have landed in master but are not yet released. | ||
Click to see more. | ||
</summary> | ||
<!-- Upcoming changes go here | ||
</details> --> | ||
|
||
## 4.0.0 (release date TBD) | ||
|
||
### General changes | ||
|
||
#### Improved performance | ||
The legacy DevTools extension used to add significant performance overhead, making it unusable for some larger React applications. That overhead has been effectively eliminated in version 4. | ||
|
||
[Learn more](https://github.com/bvaughn/react-devtools-experimental/blob/master/OVERVIEW.md) about the performance optimizations that made this possible. | ||
|
||
#### Component stacks | ||
|
||
React component authors have often requested a way to log warnings that include the React ["component stack"](https://reactjs.org/docs/error-boundaries.html#component-stack-traces). DevTools now provides an option to automatically append this information to warnings (`console.warn`) and errors (`console.error`). | ||
|
||
 | ||
|
||
It can be disabled in the general settings panel: | ||
|
||
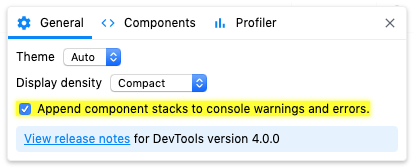 | ||
|
||
### Components tree changes | ||
|
||
#### Component filters | ||
|
||
Large component trees can sometimes be hard to navigate. DevTools now provides a way to filter components so that you can hide ones you're not interested in seeing. | ||
|
||
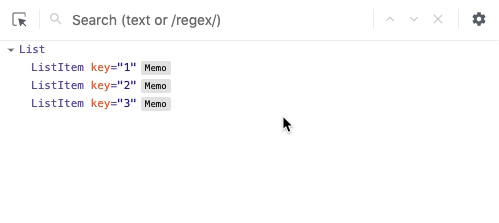 | ||
|
||
Host nodes (e.g. HTML `<div>`, React Native `View`) are now hidden by default, but you can see them by disabling that filter. | ||
|
||
Filter preferences are remembered between sessions. | ||
|
||
#### No more in-line props | ||
|
||
Components in the tree no longer show in-line props. This was done to [make DevTools faster](https://github.com/bvaughn/react-devtools-experimental/blob/master/OVERVIEW.md) and to make it easier to browse larger component trees. | ||
|
||
You can view a component's props, state, and hooks by selecting it: | ||
|
||
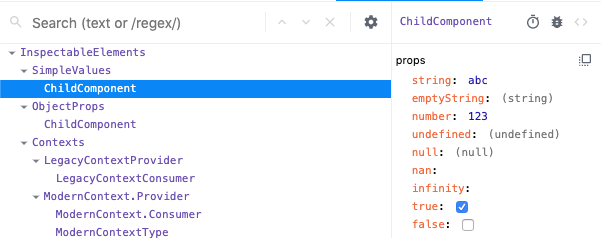 | ||
|
||
#### "Rendered by" list | ||
|
||
In React, an element's "owner" refers the thing that rendered it. Sometimes an element's parent is also its owner, but usually they're different. This distinction is important because props come from owners. | ||
|
||
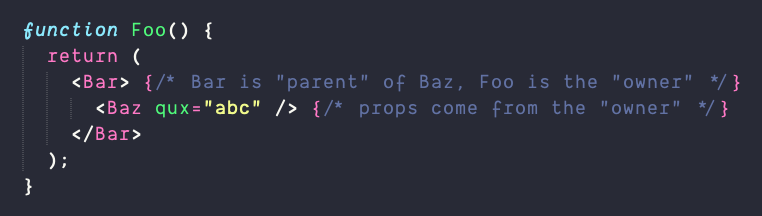 | ||
|
||
When you are debugging an unexpected prop value, you can save time if you skip over the parents. | ||
|
||
DevTools v4 adds a new "rendered by" list in the right hand pane that allows you to quickly step through the list of owners to speed up your debugging. | ||
|
||
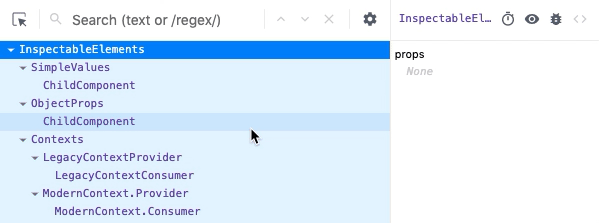 | ||
|
||
#### Owners tree | ||
|
||
The inverse of the "rendered by" list is called the "owners tree". It is the list of things rendered by a particular component- (the things it "owns"). This view is kind of like looking at the source of the component's render method, and can be a helpful way to explore large, unfamiliar React applications. | ||
|
||
Double click a component to view the owners tree and click the "x" button to return to the full component tree: | ||
|
||
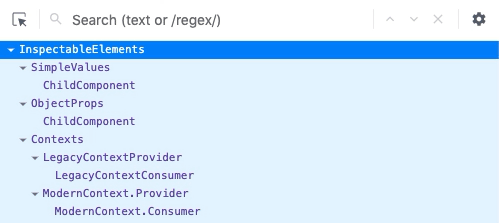 | ||
|
||
#### No more horizontal scrolling | ||
|
||
Deeply nested components used to require both vertical and horizontal scrolling to see, making it easy to "get lost" within large component trees. DevTools now dynamically adjusts nesting indentation to eliminate horizontal scrolling. | ||
|
||
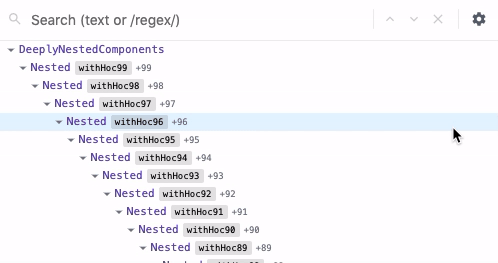 | ||
|
||
#### Improved hooks support | ||
|
||
Hooks now have the same level of support as props and state: values can be edited, arrays and objects can be drilled into, etc. | ||
|
||
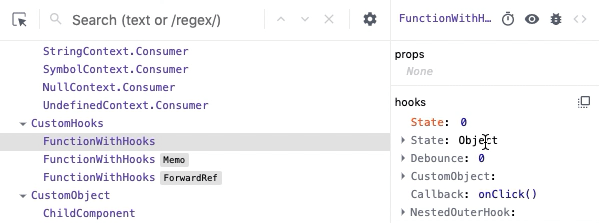 | ||
|
||
#### Improved search UX | ||
|
||
Legacy DevTools search filtered the components tree to show matching nodes as roots. This made the overall structure of the application harder to reason about, because it displayed ancestors as siblings. | ||
|
||
Search results are now shown inline similar to the browser's find-in-page search. | ||
|
||
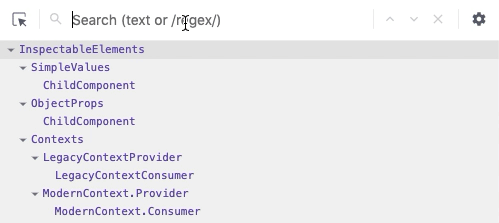 | ||
|
||
#### Higher order components | ||
|
||
[Higher order components](https://reactjs.org/docs/higher-order-components.html) (or HOCs) often provide a [custom `displayName`](https://reactjs.org/docs/higher-order-components.html#convention-wrap-the-display-name-for-easy-debugging) following a convention of `withHOC(InnerComponentName)` in order to make it easier to identify components in React warnings and in DevTools. | ||
|
||
The new Components tree formats these HOC names (along with several built-in utilities like `React.memo` and `React.forwardRef`) as a special badge to the right of the decorated component name. | ||
|
||
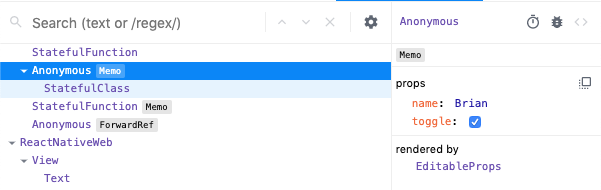 | ||
|
||
Components decorated with multiple HOCs show the topmost badge and a count. Selecting the component shows all of the HOCs badges in the properties panel. | ||
|
||
 | ||
|
||
#### Suspense toggle | ||
|
||
React's experimental [Suspense API](https://reactjs.org/docs/react-api.html#suspense) lets components "wait" for something before rendering. `<Suspense>` components can be used to specify loading states when components deeper in the tree are waiting to render. | ||
|
||
DevTools lets you test these loading states with a new toggle: | ||
|
||
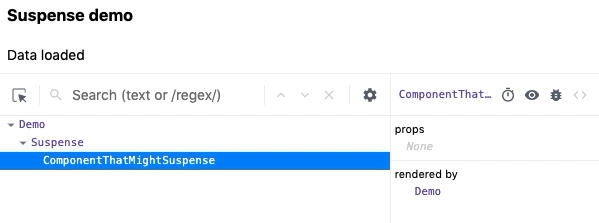 | ||
|
||
### Profiler changes | ||
|
||
#### Reload and profile | ||
|
||
The profiler is a powerful tool for performance tuning React components. Legacy DevTools supported profiling, but only after it detected a profiling-capable version of React. Because of this there was no way to profile the initial _mount_ (one of the most performance sensitive parts) of an application. | ||
|
||
This feature is now supported with a "reload and profile" action: | ||
|
||
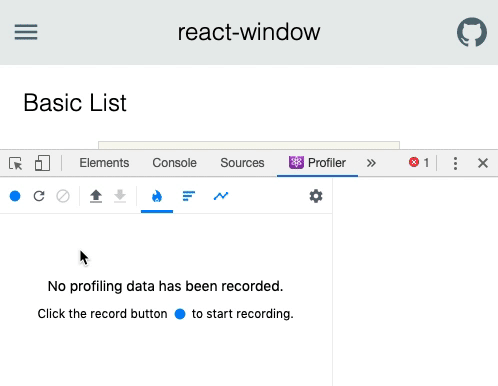 | ||
|
||
#### Import/export | ||
|
||
Profiler data can now be exported and shared with other developers to enable easier collaboration. | ||
|
||
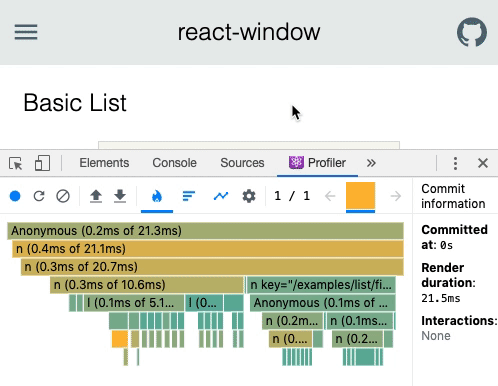 | ||
|
||
Exports include all commits, timings, interactions, etc. | ||
|
||
#### "Why did this render?" | ||
|
||
"Why did this render?" is a common question when profiling. The profiler now helps answer this question by recording which props and state change between renders. | ||
|
||
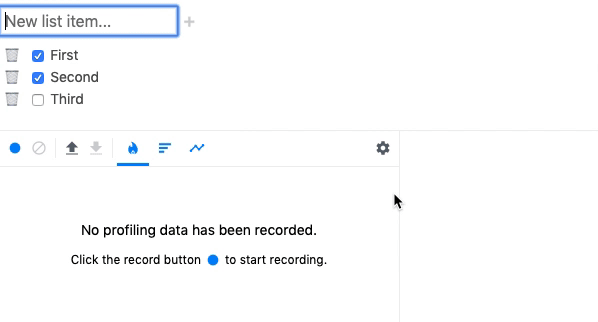 | ||
|
||
Because this feature adds a small amount of overhead, it can be disabled in the profiler settings panel. | ||
|
||
#### Component renders list | ||
|
||
The profiler now displays a list of each time the selected component rendered during a profiling session, along with the duration of each render. This list can be used to quickly jump between commits when analyzing the performance of a specific component. | ||
|
||
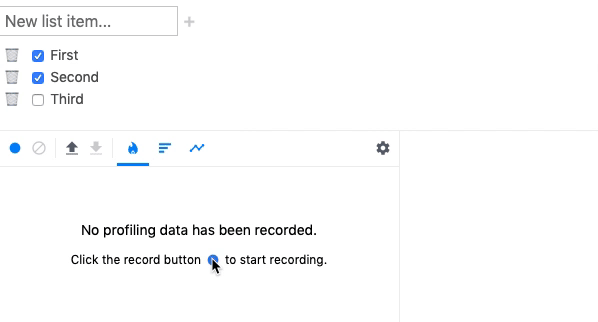 |
Oops, something went wrong.