-
Notifications
You must be signed in to change notification settings - Fork 3.2k
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Property 'type' does not exist on type 'Node' when trying to apply custom formatting #4915
Comments
I think you should find the answer here. |
You need to check if node is block like this: match: n => Editor.isBlock(editor, n) && n.type === 'code' |
The type error still happens on the latest version, 0.77.2, even after using the TypeScript playground example: import { BaseElement, Editor, Element, Node } from "slate";
declare const editor: Editor;
declare const node: Node;
if (Editor.isBlock(editor, node)) {
node.type;
// ~~~~
// Property 'type' does not exist on type 'BaseEditor | BaseElement'.
// Property 'type' does not exist on type 'BaseEditor'.
}
if (Element.isElement(node)) {
node.type;
// ~~~~
// Property 'type' does not exist on type 'BaseEditor | BaseElement'.
// Property 'type' does not exist on type 'BaseEditor'.
}
declare const baseElement: BaseElement;
baseElement.type;
// ~~~~
// Property 'type' does not exist on type 'BaseElement'. |
Hi! Are there any workarounds for this? |
Hey @JoshuaKGoldberg did you find any solution to this? |
Nope |
Quick workaround:
to
|
The next recommendation in the official documentation is not fully working You may occur with an error after narrowing types using
To solve this you need to extend slate types like that // custom.d.ts
import { BaseElement } from 'slate';
declare module 'slate' {
export interface BaseElement {
type: string;
}
} |
Mine was actually for bold. I found a workaround for this by first extending the BaseEditor and then creating a new type for Text and Element.
|
Thanks for the help. |
For this problem, the solution is simple and actually documented in official docs
|
my solution: export type LinkifyElement = {
type: 'link';
url: string;
children: Text[];
};
declare module 'slate' {
interface CustomTypes {
Editor: LinkifyEditor;
Element: LinkifyElement | BaseElement;
Text: LinkifyElement | BaseText;
}
} later: const [link] = Editor.nodes(editor, {
match: (n) => 'type' in n && n.type === 'link',
});
...
const Element = ({ attributes, children, element }: RenderElementProps) => {
const editor = useSlateStatic();
if ('type' in element) {
if (element.type === 'link') {
return editor.linkElementType({ attributes, children, element });
}
}
return <p {...attributes}>{children}</p>;
}; |
None of the answers solves the original problem.
And yet, any way I fetch a node using Editor.next, Editor.parent, Editor.above, Editor.node, etc. I always get the same type error:
I am tempted to use Any suggestions? |
@rahulnyk you need to narrow down the type of the Node to Element. You can do that by applying a condition, either an 'type' in node && node.type === 'someType'
// -> TS now knows `type` property exists or Element.isElement(node) && node.type === 'someType' The second example might fail if your custom |
Thanks @maral We need better documentation here. Also, if I haven't said it already... thank you to all the contributors for Slate :) |
this worked for me.
|
Description
From the example (which isn't in TS, but I am doing my best to translate as I follow along), using match seemed to work with custom types. This is my code for toggling Custom Formatting:
However, I get the warning:
Recording
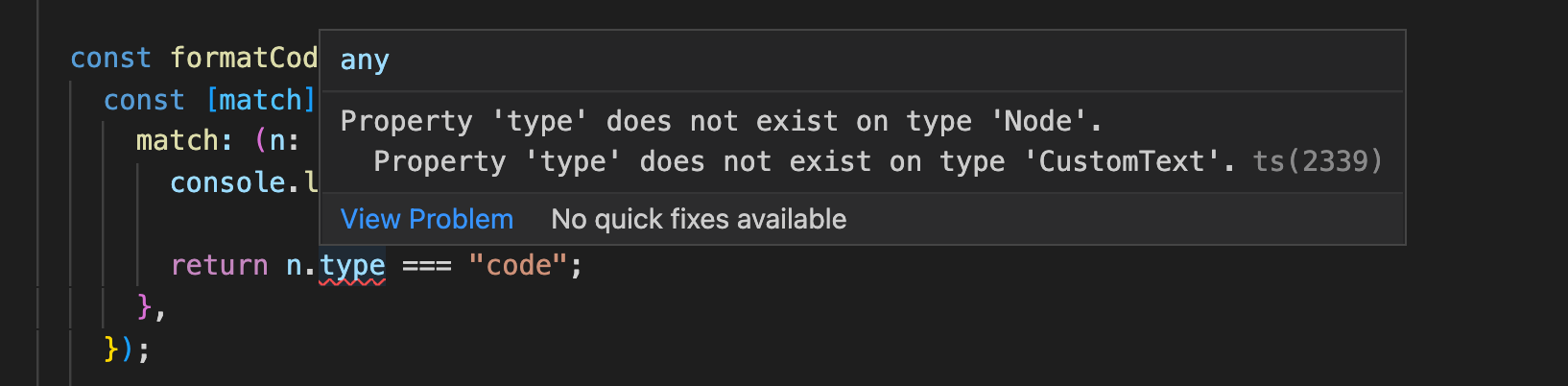
Environment
Operating System: macOS
TypeScript Version:
"typescript": "^4.6.2"
Context
I'll add the relevant code below
The text was updated successfully, but these errors were encountered: