struct HomeView: View {
// MARK: - PROPERTY
@State private var showPortfolio: Bool = false
// MARK: - BODY
var body: some View {
ZStack {
// Background Layer
Color.theme.background.ignoresSafeArea()
// Content Layer
VStack {
homeHeader
Spacer(minLength: 0)
} //: VSTACK
} //: ZSTACK
}
}
// MARK: - PREVIEW[
struct HomeView_Previews: PreviewProvider {
static var previews: some View {
NavigationView {
HomeView()
.navigationBarHidden(true)
}
}
}
// MARK: - EXTENTION
extension HomeView {
// Header
private var homeHeader: some View {
HStack {
CircleButtonView(iconName: showPortfolio ? "plus" : "info")
.animation(.none, value: showPortfolio)
.background(
CircleButtonAnimationView(animate: $showPortfolio)
)
Spacer()
Text(showPortfolio ? "Portfolio" : "Live Prices")
.font(.headline)
.fontWeight(.heavy)
.foregroundColor(Color.theme.accent)
.transaction { transaction in
transaction.animation = nil
}
Spacer()
CircleButtonView(iconName: "chevron.right")
.rotationEffect(Angle(degrees: showPortfolio ? 180 : 0))
.onTapGesture {
withAnimation(.spring()) {
showPortfolio.toggle()
}
}
}
.padding(.horizontal)
}
}
struct CircleButtonView: View {
// MARK: - PROPERTY
let iconName: String
// MARK: - BODY
var body: some View {
Image(systemName: iconName)
.font(.headline)
.foregroundColor(Color.theme.accent)
.frame(width: 50, height: 50)
.background(
Circle()
.foregroundColor(Color.theme.background)
)
.shadow(
color: Color.theme.accent.opacity(0.25),
radius: 10, x: 0, y: 0)
}
}
// MARK: - PREVIEW
struct CircleButtonView_Previews: PreviewProvider {
static var previews: some View {
Group {
CircleButtonView(iconName: "info")
.padding()
.previewLayout(.sizeThatFits)
}
Group {
CircleButtonView(iconName: "plus")
.padding()
.previewLayout(.sizeThatFits)
.preferredColorScheme(.dark)
}
}
}
struct CircleButtonAnimationView: View {
// MARK: - PROPERTY
@Binding var animate: Bool
// @State private var animate: Bool = false
// MARK: - BODY
var body: some View {
Circle()
.stroke(lineWidth: 5.0)
.scale(animate ? 1.2 : 0.0)
.opacity(animate ? 0.0 : 1.0)
.animation(animate ? Animation.easeOut(duration: 1.0) : .none, value: animate)
}
}
// MARK: - PREVIEW
struct CircleButtonAnimationView_Previews: PreviewProvider {
static var previews: some View {
CircleButtonAnimationView(animate: .constant(false))
.foregroundColor(.red)
.frame(width: 100, height: 100)
}
}
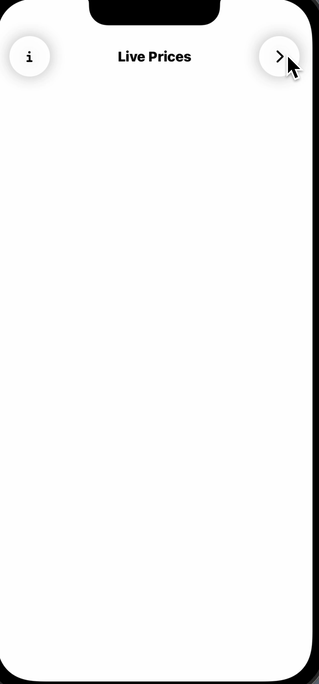