- Tiny with 0 dependency and simple (639B gzip)
- Persist state by default (
sessionStorage
orlocalStorage
) - Build with React Hooks
$ npm install little-state-machine
This is a Provider Component to wrapper around your entire app in order to create context.
<StateMachineProvider>
<App />
</StateMachineProvider>
Function to initialize the global store, invoked at your app root (where <StateMachineProvider />
lives).
function log(store) {
console.log(store);
return store;
}
createStore(
{
yourDetail: { firstName: '', lastName: '' } // it's an object of your state
},
{
name?: string; // rename the store
middleWares?: [ log ]; // function to invoke each action
storageType?: Storage; // session/local storage (default to session)
},
);
This hook function will return action/actions and state of the app.
const { actions, state } = useStateMachine<T>({
updateYourDetail,
});
Quick video tutorial on little state machine.
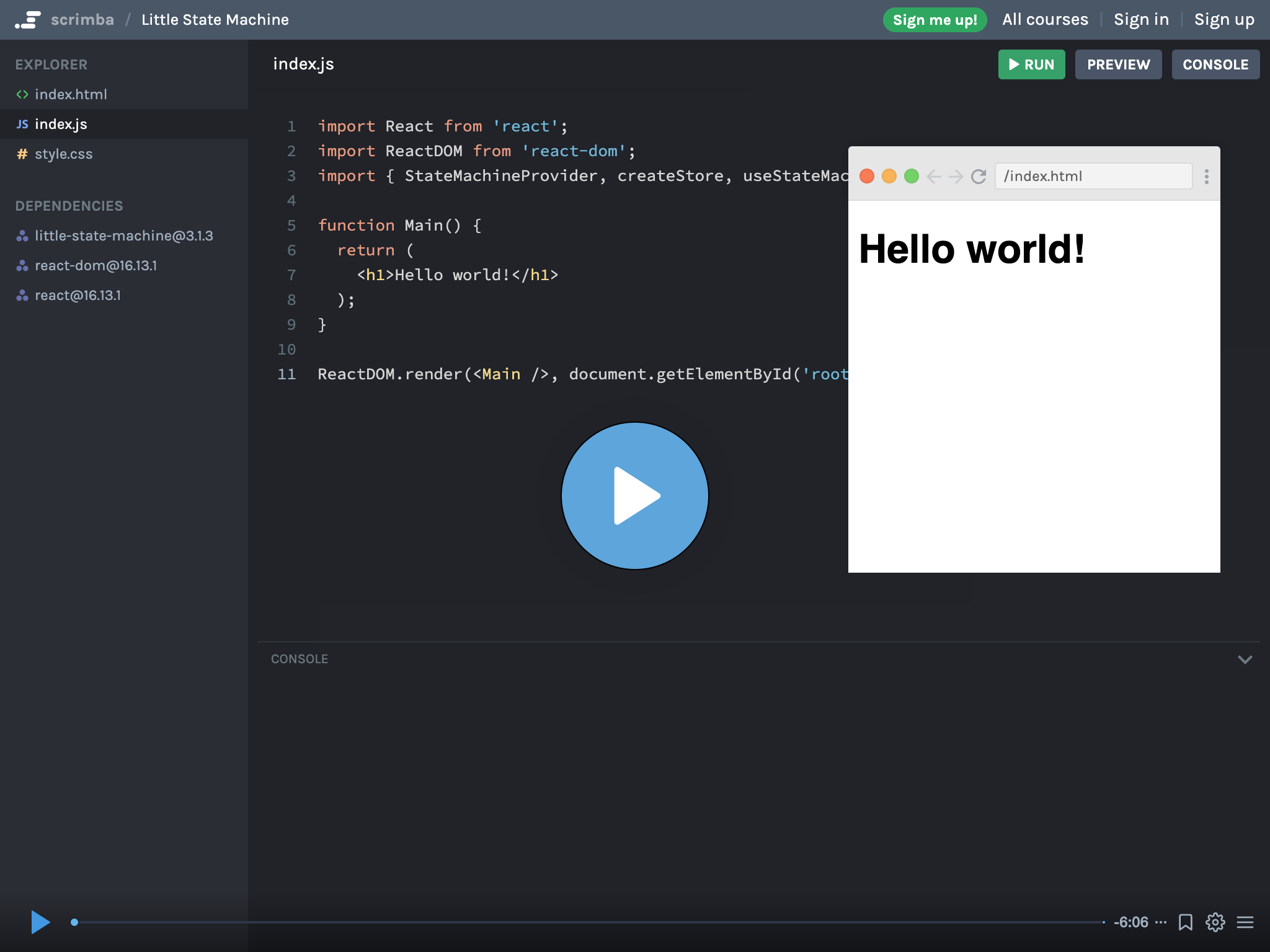
Check out the Demo.
import React from 'react';
import {
StateMachineProvider,
createStore,
useStateMachine,
} from 'little-state-machine';
createStore({
yourDetail: { name: '' },
});
function updateName(state, payload) {
return {
...state,
yourDetail: {
...state.yourDetail,
...payload,
},
};
}
function YourComponent() {
const { actions, state } = useStateMachine(updateName);
return (
<div onClick={() => actions.updateName({ name: 'bill' })}>
{state.yourDetail.name}
</div>
);
}
export default () => (
<StateMachineProvider>
<YourComponent />
</StateMachineProvider>
);
DevTool component to track your state change and action.
import { DevTool } from 'little-state-machine-devtools';
<StateMachineProvider>
<DevTool />
</StateMachineProvider>;
If you run into issues, feel free to open an issue.
Consider adding Object.entries()
polyfill if you're wondering to have support for old browsers.
You can weather consider adding snippet below into your code, ideally before your App.js file:
utils.[js|ts]
if (!Object.entries) {
Object.entries = function (obj) {
var ownProps = Object.keys(obj),
i = ownProps.length,
resArray = new Array(i); // preallocate the Array
while (i--) resArray[i] = [ownProps[i], obj[ownProps[i]]];
return resArray;
};
}
Or you can add core-js polyfill into your project and add core-js/es/object/entries
in your polyfills.[js|ts]
file.
Thank you very much for those kind people with their sponsorship to this project.