
Creational Design Patterns | Structural Design Patterns | Behavioral Design Patterns
In software engineering, a software design pattern is a general reusable solution to a commonly occurring problem within a given context in software design. It is not a finished design that can be transformed directly into source or machine code. It is a description or template for how to solve a problem that can be used in many different situations.
This article assumes you are reasonably proficient in at least one object-oriented programming language, and you should have some experience in object-oriented design as well. You definitely shouldn't have to rush to the nearest dictionary the moment we mention "types" and "polymorphism," or "interface" as opposed to "implementation / inheritance”.
But I'll try to keep the literature as simple as possible.
Don't worry if you don’t understand this article completely on the first reading. We didn’t understand it all on the first writing! Remember that this isn't an article to read once and forget it. We hope you'll find yourself referring to it again and again for design insights and for inspiration.
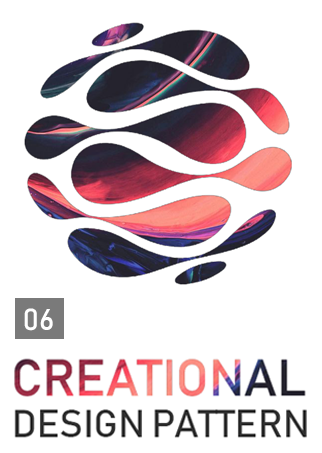
Creational Design Patterns are concerned with the way in which objects are created.
Creational design patterns abstract the instantiation process, meaning construction of objects is decoupled from their implementation logic.
They help make a system independent of how its objects are created,composed, and represented.
Encapsulate knowledge about which concreate classes the system uses.
Hide how instances of these classes are created and put together.
Note : A class creational pattern uses inheritance to vary the class that's instantiated, where as an object creational pattern will delegate instantiation to another object.
Creational Design patterns Table
Thumbnail | Design Pattern |
---|---|
Factory Method | |
Abstract Factory | |
Builder | |
Prototype | |
Singleton | |
Object Pool Design Pattern |
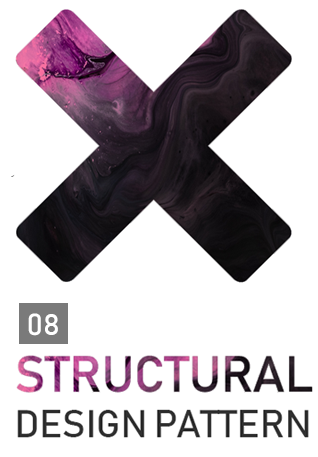
Structural patterns are mostly concerned with object composition or in other words how the entities can use each other. Or yet another explanation would be, they help in answering "How to build a software component?"
Structural patterns explain how to assemble objects and classes into larger structures while keeping these structures flexible and efficient.
A structural design pattern serves as a blueprint for how different classes and objects are combined to form larger structures. Unlike creational patterns, which are mostly different ways to fulfill the same fundamental purpose, each structural pattern has a different purpose.
Structural Design patterns Table
Thumbnail | Design Pattern |
---|---|
Adapter | |
Composite | |
Proxy | |
Flyweight | |
Facade | |
Bridge | |
Decorator |
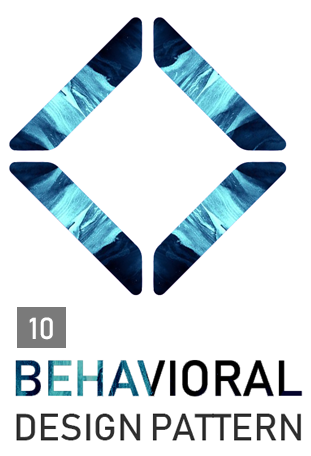
Behavioral design patterns are concerned with algorithms and the assignment of responsibilities between objects.
Behavioral Design Patterns identify common communication patterns between objects and realize these patterns. By doing so, these patterns increase flexibility in carrying out this communication.
What makes them different from structural patterns is they don't just specify the structure but also outline the patterns for message passing/communication between them. Or in other words, they assist in answering "How to run a behavior in software component?"
Behavioral Design patterns Table
Thumbnail | Design Pattern |
---|---|
Template Method | |
Mediator | |
Chain of Responsibility | |
Observer | |
Strategy | |
Command | |
State | |
Visitor | |
Interpreter | |
Iterator | |
Memento |
Once you understand the design patterns and have had an "Aha!" (and not just a "Huh?") experience with them, you won't ever think about object-oriented design in the same way.
The intent of this Article is provide you with insights that can make your own designs more flexible, modular, reusable, and understandable.
Inspiration -> Ahmed Kamran