-
Notifications
You must be signed in to change notification settings - Fork 59
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Constructor Injected Class Instance Variable Loses Mocking doThrow or doReturn Doesn't work #91
Comments
@gursahibsahni Hi there, 2 things
Thanks |
@bbonanno sorry about the confusion. I have replaced The exception has changed and its a missing dependency
|
@gursahibsahni There are quite a few things wrong with this, let's separate them in 2
<dependency>
<groupId>org.mockito</groupId>
<artifactId>mockito-core</artifactId>
<version>2.25.1</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>net.bytebuddy</groupId>
<artifactId>byte-buddy</artifactId>
<version>1.9.7</version>
</dependency>
<dependency>
<groupId>net.bytebuddy</groupId>
<artifactId>byte-buddy-agent</artifactId>
<version>1.9.7</version>
</dependency>
Mockito.verify(headerParamsResolverMock).resolve(any[NativeWebRequest])
doReturn("string", Nil: _*).when(headerParamsResolverMock).resolve(any[NativeWebRequest])
"resolverArgument" should {
"invoke resolve method if header parameter is not resolved" in {
val parameter = mock[Parameter]
doReturn(parameter).when(methodParameterMock).getParameter
doReturn("string").when(parameter).getName
doReturn(false).when(headerParamsResolverMock).isResolved
doReturn("string").when(headerParamsResolverMock).resolve(*)
irsArgumentResolver.resolveArgument(methodParameterMock, mock[ModelAndViewContainer], mock[NativeWebRequest], mock[WebDataBinderFactory])
verify(headerParamsResolverMock).resolve(*)
}
} |
@bbonanno big thanks for addressing all the points. Especially the incorrect maven resolver. All looks good and it feels so good writing verbose but less code for unit tests. |
@gursahibsahni No problem You mean the examples in the tests that use |
@bbonanno I tried to raise a PR against release/1.x but I was denied permission to push. I couldn't find the contribution guidelines I can refer to. |
@gursahibsahni Is the standard flow, you have to fork the repo, push into that fork and raise a pull request from there to this repo. |
thanks for the instructions |
I have a spring boot application and am using MockitoSugar to write tests.
Spring boot version: 2.0.5.RELEASE
Scala version: 2.11.8
mockito-scala_2.11: 1.2.4
Injecting mock in constructor:
Test Case:
Class under test
If I see the debugger, the headerParamsResolver isn't shown as a mock object like other method parameters show up. Like
Mock for Method Paramater
ORMock for ModelAndViewContainer
etc. Moreover, only the isResolved class instance variable is set but other mock features like doThrow or doReturn doesn't work. PFA debugger screen.Moreover, this test case throws exception:
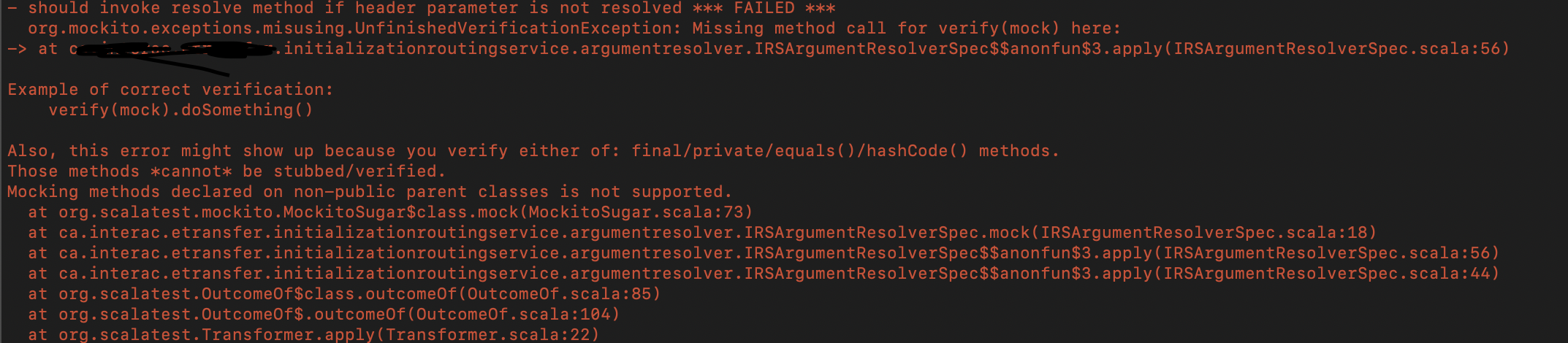
The text was updated successfully, but these errors were encountered: