You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
The arithmetic on extensions with the same degree is the same, i.e. proc on Fp2 and Fp12 are the same. And if we built Fp36 on top of Fp12 it would have the same arithmetic as Fp6.
However I noticed that my generated codesize was quite huge with lots of useless procedures that were generated for the exact same input types over 15 times:
(Another side issue is that compile-time is quite long, about ~10 on my very beefy machine.)
Furthermore, inline procedures defined on Base can somehow be duplicated in the Fp6 file even though nothing in Fp2 is not tagged inline and so there is no reason for those to appear in the Fp6 file.
Use-case
There are actually not many proc defined per types: addition, substraction, squaring, doubling, setZero, setOne, inversion, multiplication but this generates ~20k lines with a lot of duplicate code.
This is problematic because those towers are used to implement pairing-based cryptography which is particularly attractive in embedded and resource-restricted devices for the small key sizes required compared to the security provided (i.e. for 128 bits of security, a 256-bit and 512-bit secret/public keypair is needed compared to RSA 2x3000+ bits) and obviously code size should be small as well.
As bad as it might look, we need to investigate if the linker can remove the duplicated code or not. In theory duplicate function elimination is trivial to do...
I'm not sure if the linker can handle the sheer size of duplicates, compilers have artificial limits on code analysis (like ~300 statements even if they can be optimized away in later passes).
I didn't realize how bad it is but I have 457 instances of duplicated "prod_complex" if I understood properly the mangling scheme:
Line 13k: 220 instances of prod complex
Line 19k: 458 instances
And notice how the last prod_generic is calling different (but duplicates) proc with the same name with just an incremented counter at the end.
Context
I need to build a "tower" of mathematical objects of the following representation:
complex
from 2real
coordinates)Example
The arithmetic on extensions with the same degree is the same, i.e. proc on Fp2 and Fp12 are the same. And if we built Fp36 on top of Fp12 it would have the same arithmetic as Fp6.
In short, this is a great place to use concepts, which I did: https://github.com/mratsim/constantine/blob/8a9cb928/constantine/tower_field_extensions/tower_common.nim
However I noticed that my generated codesize was quite huge with lots of useless procedures that were generated for the exact same input types over 15 times:
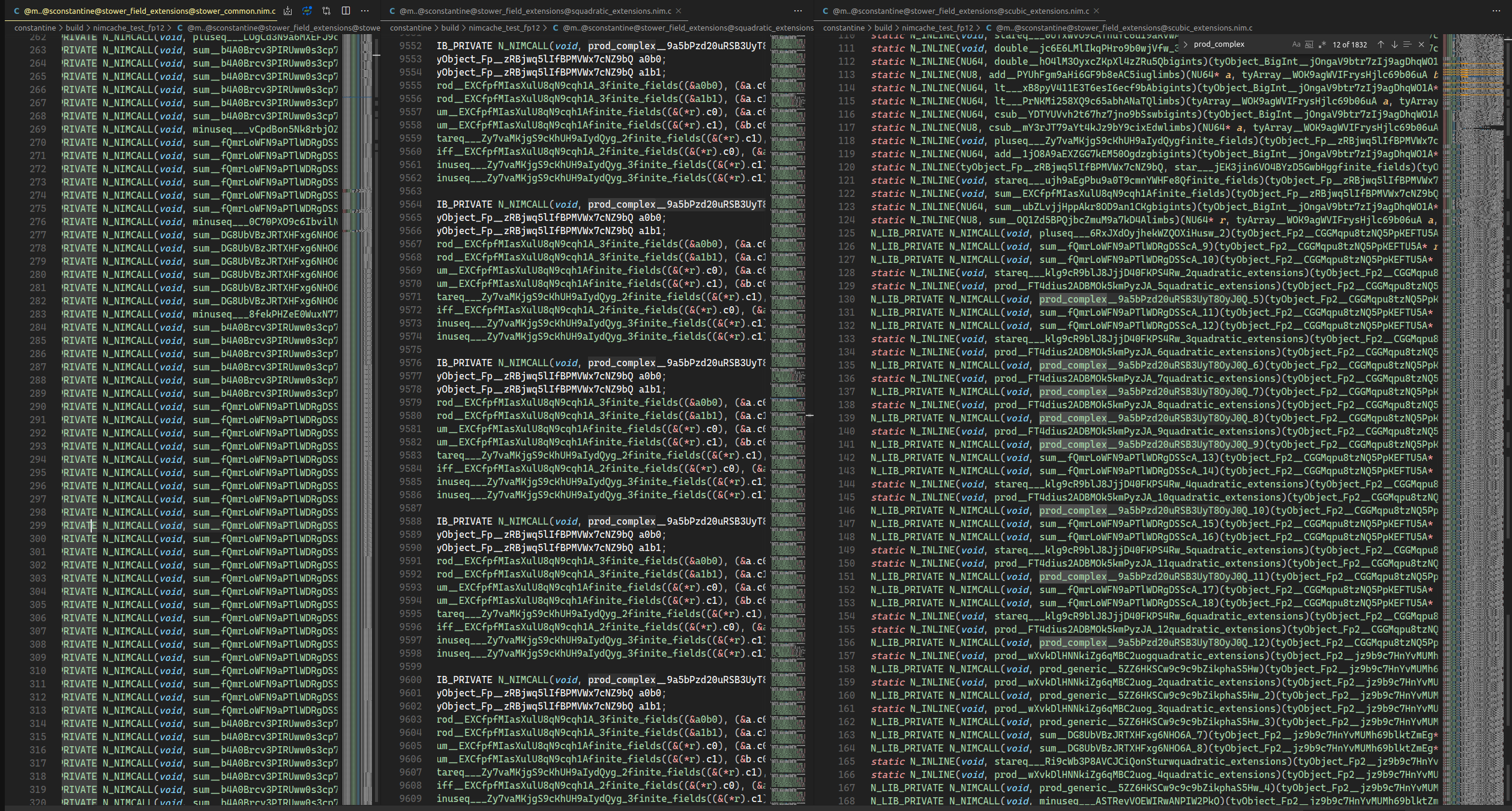
(Another side issue is that compile-time is quite long, about ~10 on my very beefy machine.)
Furthermore, inline procedures defined on
Base
can somehow be duplicated in theFp6
file even though nothing inFp2
is not tagged inline and so there is no reason for those to appear in theFp6
file.Use-case
There are actually not many proc defined per types: addition, substraction, squaring, doubling, setZero, setOne, inversion, multiplication but this generates ~20k lines with a lot of duplicate code.
This is problematic because those towers are used to implement pairing-based cryptography which is particularly attractive in embedded and resource-restricted devices for the small key sizes required compared to the security provided (i.e. for 128 bits of security, a 256-bit and 512-bit secret/public keypair is needed compared to RSA 2x3000+ bits) and obviously code size should be small as well.
Reproducing
Minimal example
Here is a minimal example, 4 files are needed
Compile to a local nimcache, for example (release because the linetrace are quite verbose in the codegen)
The
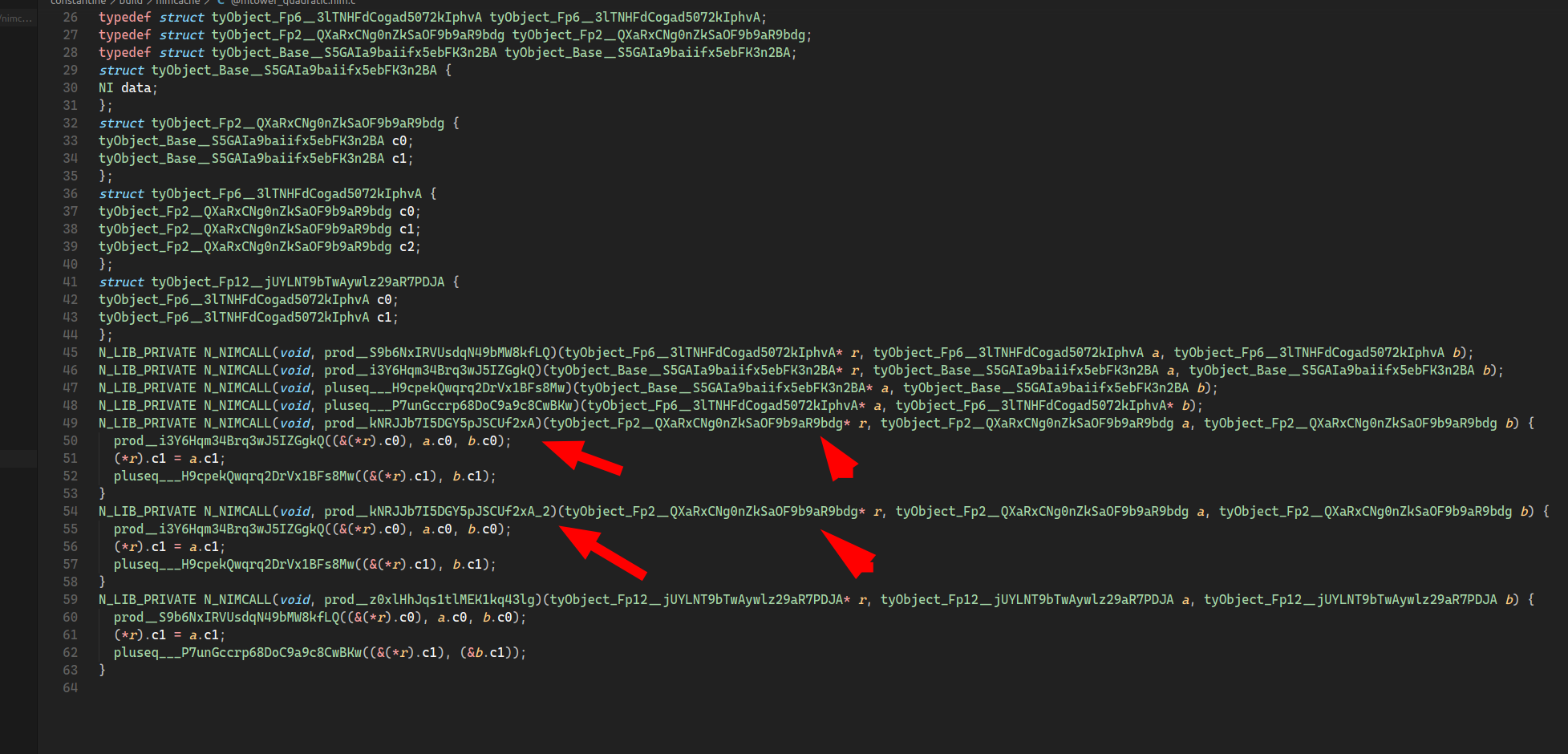
prod
procedure in quadratic.nim is duplicated:In the actual codebase
To reproduce in the actual codebase:
And look for the files over 3 MB. Compilation will be long.
The file involved int he tower are in this folder https://github.com/mratsim/constantine/tree/8a9cb928/constantine/tower_field_extensions
The text was updated successfully, but these errors were encountered: