You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
the problem seems to stem from the fact that in the FillCircle method the individual lines sometimes get draw multiple times:
here is my modified example code to generate the above image:
#define OLC_PGE_APPLICATION
#include "olcPixelGameEngine.h"
class Example : public olc::PixelGameEngine
{
public:
Example()
{
sAppName = "Example";
}
public:
bool OnUserCreate() override
{
// Called once at the start, so create things here
olc::PixelGameEngine::SetPixelMode(olc::Pixel::ALPHA);
this->Clear(olc::BLACK);
FillCircle(100, 100, 60, olc::Pixel(255,0,0,200));
return true;
}
bool OnUserUpdate(float fElapsedTime) override
{
// called once per frame
return true;
}
};
int main()
{
Example demo;
if (demo.Construct(256, 256, 2, 2))
demo.Start();
return 0;
}
and here the modified FillCircle to show that is has overdrawn:
void PixelGameEngine::FillCircle(int32_t x, int32_t y, int32_t radius, Pixel p)
{
// Taken from wikipedia
int x0 = 0;
int y0 = radius;
int d = 3 - 2 * radius;
if (!radius) return;
static std::vector<std::vector<int>> hits(260, std::vector<int>(260,0));
auto drawline = [&](int sx, int ex, int ny)
{
for (int i = sx; i <= ex; i++)
{
hits[i][ny]++;
Draw(i, ny, p);
}
};
while (y0 >= x0)
{
// Modified to draw scan-lines instead of edges
drawline(x - x0, x + x0, y - y0);
drawline(x - y0, x + y0, y - x0);
drawline(x - x0, x + x0, y + y0);
drawline(x - y0, x + y0, y + x0);
if (d < 0) d += 4 * x0++ + 6;
else d += 4 * (x0++ - y0--) + 10;
}
for(auto v : hits)
{
for(int i : v)
std::cout << i;
std::cout << std::endl;
}
}
on the console (background in the image) one can clearly see multiple calls to draw for the same position, because alphablending is enabled this leads to brighter reds than at other positions, hence the stripes.
The text was updated successfully, but these errors were encountered:
the problem seems to stem from the fact that in the FillCircle method the individual lines sometimes get draw multiple times:
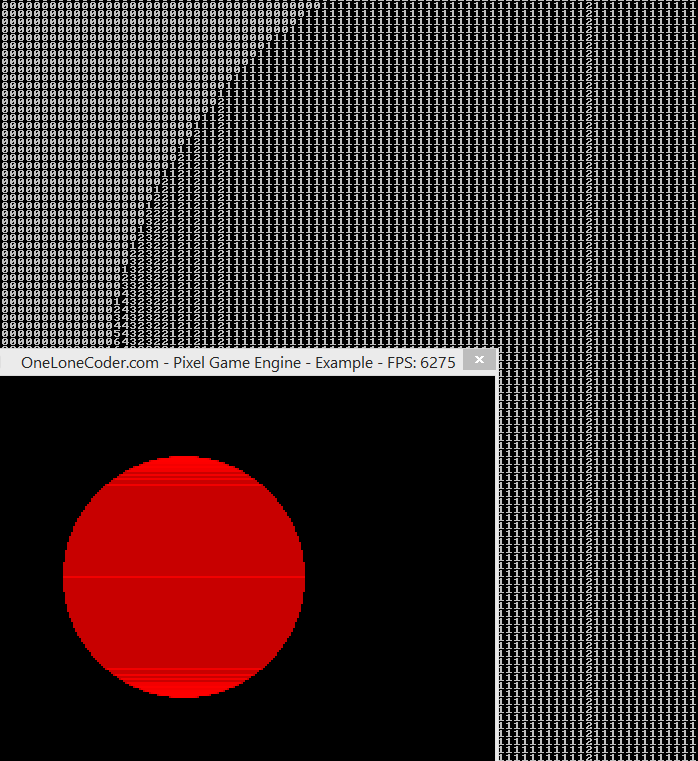
here is my modified example code to generate the above image:
and here the modified FillCircle to show that is has overdrawn:
on the console (background in the image) one can clearly see multiple calls to draw for the same position, because alphablending is enabled this leads to brighter reds than at other positions, hence the stripes.
The text was updated successfully, but these errors were encountered: