-
Notifications
You must be signed in to change notification settings - Fork 283
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Help with creating simulation box and locating stl in simulation box #57
Comments
Hi @VijayN10, apologies for the delayed response! This is a very interesting setup and I wanted to give a very detailed response, but my schedule was too busy until now. I hope this helps you, and please let me know if there is still something unclear. The You want to place the geometry such that it is sitting flush on the bottom (-z) and right (+y) walls of the simulation box, also taking into account the one grid layer for the simulation box walls.. Here it is easiest to split the stl voxelization into 2 parts:
The loading step puts it in the center of the simulation box, and then we need to translate it from the center by Mesh* mesh = read_stl(get_exe_path()+"../stl/kyoto.stl", lbm.size(), lbm.center(), 30.0f);
const float3 mesh_size = mesh->pmax-mesh->pmin;
const float3 mesh_translation = float3(0.0f, -1.0f+0.5f*lbm.size().y-0.5f*mesh_size.y, 1.0f-0.5f*lbm.size().z+0.5f*mesh_size.z); // "+-1.0f" to account for simulation box boundary
mesh->translate(mesh_translation);
lbm.voxelize_mesh_on_device(mesh); To your specific questions:
Note that you also need to set a volume force in the LBM cionstructor such that the water stays at the bottom and does not start to float around in zero-g. Have a look at the "raindrop" sample setup, and also how the unit conversion is done there with the aid of the Thank you for using FluidX3D, have fun with it! |
@ProjectPhysX , thank you so much for the detailed explanation! I will definitely try this out and will update you sooner. |
Hello!
I want to simulate fluid - structure interactions for coastal waves. But have few queries regarding creation of simulation box (domain) and locating stl file in the simulation box. The desired set up would look like as in figure below with a domain size of 6m x 30m x 4m. It is having fluid below 0.75m.
The stl file would look like following image, where blue coordinate symbol represents origin -
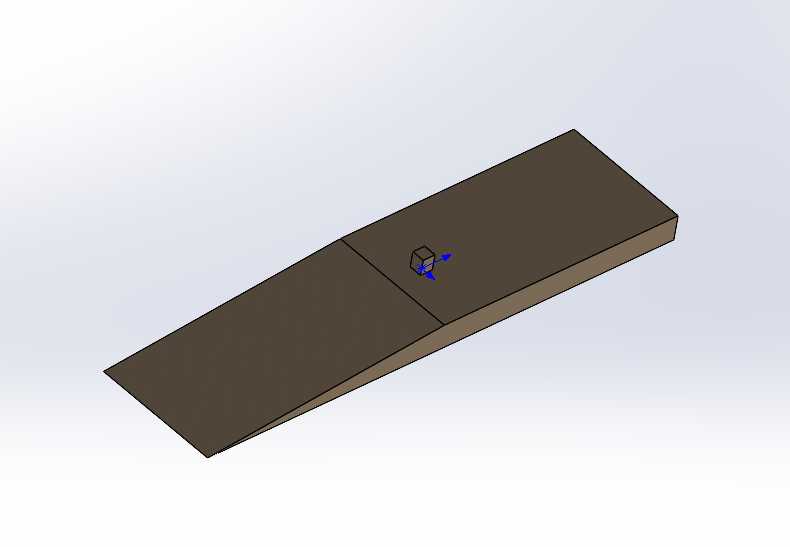
I have used following code -
But, when I run the case. The set up appears as below image -
Here, the stl gets scaled up uneven. And also the water level seems not correct.
I have written my doubts in the respective code lines
Thank you for your time and assistance and look forward to hearing back from you!
The text was updated successfully, but these errors were encountered: