New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
page. setRequestInterception causes network requests to hang/timeout #5003
Comments
I got the same issue on different urls. Reverting to a different puppeteer version solved the issue. |
@dege88 what version was that, out of curiosity? |
Rollback to 1.9.0 is available. |
@joelgriffith did you have any luck with this? Having the same problem... |
Hello, |
I've tried rolling back to previous versions, however the issue still seems present. My guess is that it's something embedded into Chrome and not how puppeteer is interacting with the Chrome Devtools Protocol |
Are y'all still experiencing this with puppeteer 2.0.0 or 2.1.0 ? |
yes! on Mac I have this behaviour |
Seems to be an issue with |
We are using mapbox as map provider this is my interceptor code:
I tried with Puppeteer 1.19.0 and |
Not sure if this will help anybody that's stuck but I had this 'hanging' issue and found I wasn't calling continue. When I call continue, with Found this issue wanted to share in case it helps someone. On Win 10, Chromium Version 80.0.3987.0 (Developer Build) (64-bit) with following dependencies:
This seems to work:
|
Enabling await page.setRequestInterception(true); stops futher execution, and removing await page.setRequestInterception(true); will run smoothly. There is issue with await page.setRequestInterception(true); |
Hey y'all, I found the solution to this. The download script for Chrome was pulling the latest nightly version, which is clearly full of bugs. I downloaded a stable binary of Chrome and symlinked it to the downloaded binary location in the ~/.local folder, and everything went smoothly. |
Facing the issue still. |
Facing the issue in 2021 as well |
This is also happening for me, using puppeteer |
I just noticed that if I |
I recently faceing same issue my target URL is google and youtube, fb, instagram , but it's only working with instagram and facebook, when entering google/ youtube the page not loaded and raise timeout And when i try to downgrade version to 7.1.0 |
Same problem with Google + puppeteer 11.0.0, despite using Reproduction code const browser = await puppeteer.launch({
executablePath: '/Applications/Google Chrome.app/Contents/MacOS/Google Chrome',
headless: false
});
const page = await browser.newPage();
await page.setRequestInterception(true);
page.on('request', async (req) => {
console.log('received a requst!!!');
await req.continue();
console.log('told the request to continue ;)');
});
await page.goto('https://www.google.com'); Edit: hacky solutionconst puppeteer = require('puppeteer');
async function main() {
const browser = await puppeteer.launch({
executablePath: '/Applications/Google Chrome.app/Contents/MacOS/Google Chrome',
headless: false
});
const page = await browser.newPage();
page.on('request', async (req) => { await req.continue(); });
await page.setRequestInterception(true);
await page.goto('https://google.com/' + Date.now())
await page.click(`a[href*=google]`)
}
void main(); |
Just in case you make the same mistake as me Make sure you set up - page.on("request", (request) => ...); Before - await page.goto(...) The docs state - "Once request interception is enabled, every request will stall unless it's continued, responded or aborted." So |
Check that you're not using Google Fonts on the page. Google Fonts (and, I'm assuming, other Google properties?) have an implicit rate limit. I've just debugged it to removing Google Fonts from my tested pages and the network timeout disappeared. They don't reject your request, they time out (at least at the time of this writing). |
We're marking this issue as unconfirmed because it has not had recent activity and we weren't able to confirm it yet. It will be closed if no further activity occurs within the next 30 days. |
I believe there have been many fixes and a whole new cooperative interception mode landed since the issue was reported. If it still happens, please re-open it with a repro using the latest Puppeteer version. |
I'm having the same problem on both Puppeteer 10 and 17. Puppeteer 17 is the newest version that does not cause breaking changes in my application. Adding the following code causes most of my visual regression tests to fail: await page.setRequestInterception(true);
await page.on('request', async request => {
await request.continue();
}); All of the URLs the page requires (which include I'm using storybook.js 6.5.9 (and the storyshots addon) to perform visual regression tests against a Create React App website. See: I have not done further diagnosis to determine what could be causing the failure. I'm using React lazy loading, so that may impact results; not sure. My workaround is to use this code: // do not use setRequestInterception
await page.on('request', request => {
// cause any external requests to fail
if (request.url().startsWith('http')) {
// log the url that attempted to load
console.log('page tried loading: ' + request.url());
// cause the test to fail
throw 'error';
}
}); |
Steps to reproduce
Tell us about your environment:
What steps will reproduce the problem?
Setting request interception causes certain requests to never completely resolve (though the
goto
method doesn't seem to mind).Try the following:
This returns a screenshot of:
Now, turn on request interception:
This produces the following, as mapping tiles don't load (they hang)
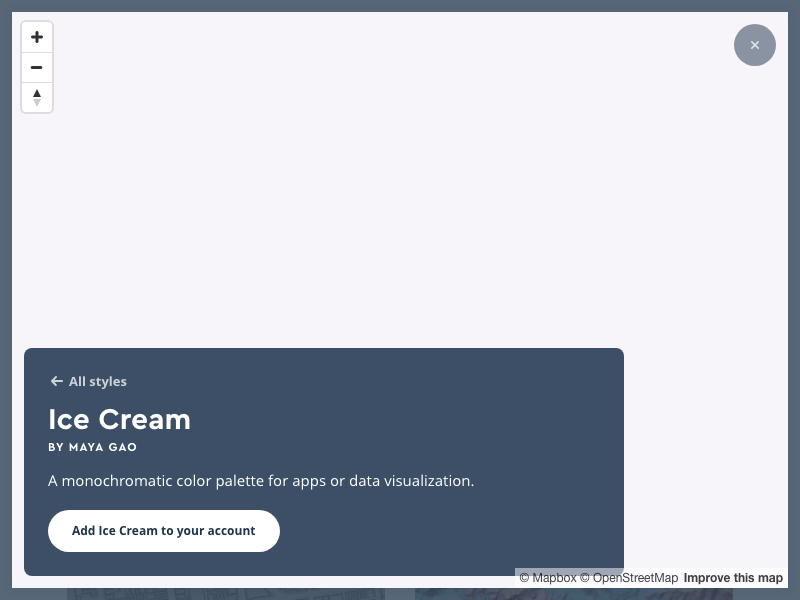
What is the expected result?
All network requests should resolve, when the condition matches
What happens instead?
Network requests never resolve completely.
My suspicion is that this has to do with the version of Chromium bundled with pptr, but I haven't checked prior version just yet.
The text was updated successfully, but these errors were encountered: