This repository has been archived by the owner on Feb 8, 2020. It is now read-only.
generated from satya164/typescript-template
-
Notifications
You must be signed in to change notification settings - Fork 41
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: lazy initialized MaterialTopTabNavigator routes (#9)
Routes in `MaterialTopTabNavigator` are now lazy initialized like in `MaterialBottomTabNavigator`. A scene visibility is computed from multiple states and props: To handle the pan between tabs, we check if you're currently swiping between tabs and the prop `lazyOnSwipe` is true (default value) or if the tab have been already loaded, we'll check if this tab is a sibling of the focused tab. Then, we'll display the tab if it's a sibling. ~With the prop `animationEnabled` to true, we shouldn't hide a tab before the transition is done. So we're waiting `COMPLETE_TRANSITION` action to hide it. Also, if the prop `sceneAlwaysVisible` is true (default value), we won't hide scenes between A and D while transitioning.~ If the current tab has not been loaded and must not be visible, we do not render it. I'll update the docs accordingly to this PR. 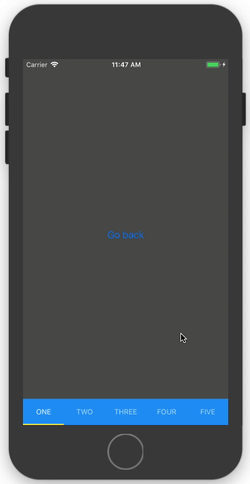 <!-- #### Default behavior Tabs are lazy initialized on swipe or focus and are always visible while transitioning. 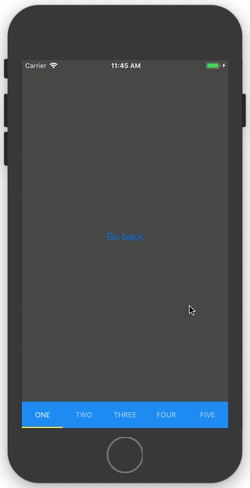 #### Hide tabs between while transitioning ```js { sceneAlwaysVisible: false, } ``` 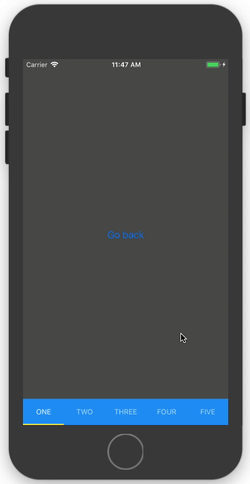 #### Fallback to only lazy initialized tabs on focus ```js { lazyOnSwipe: false, } ``` 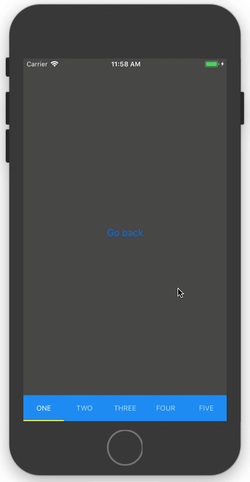 -->
- Loading branch information
Showing
4 changed files
with
143 additions
and
17 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters