You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
Expected behavior
Both comment and search fields should be validated with yup if we click on submit without selecting any options then error message should be shown same as comment field. If we enter comment and select one option and then if we click on submit we should get values object on formik onsubmit.
Screenshots
Additional context
Add any other context about the problem here.
The text was updated successfully, but these errors were encountered:
I want to use ReactSearchAutocomplete with formik and yup how to validate with yup and how to render the component as children of form field.
Package.json
Codesandbox example
(https://codesandbox.io/s/amazing-diffie-o4hbod)
Visit this codesandbox example for reference
https://codesandbox.io/s/amazing-diffie-o4hbod
To Reproduce
SearchComponent.js
Expected behavior
Both comment and search fields should be validated with yup if we click on submit without selecting any options then error message should be shown same as comment field. If we enter comment and select one option and then if we click on submit we should get values object on formik onsubmit.
Screenshots
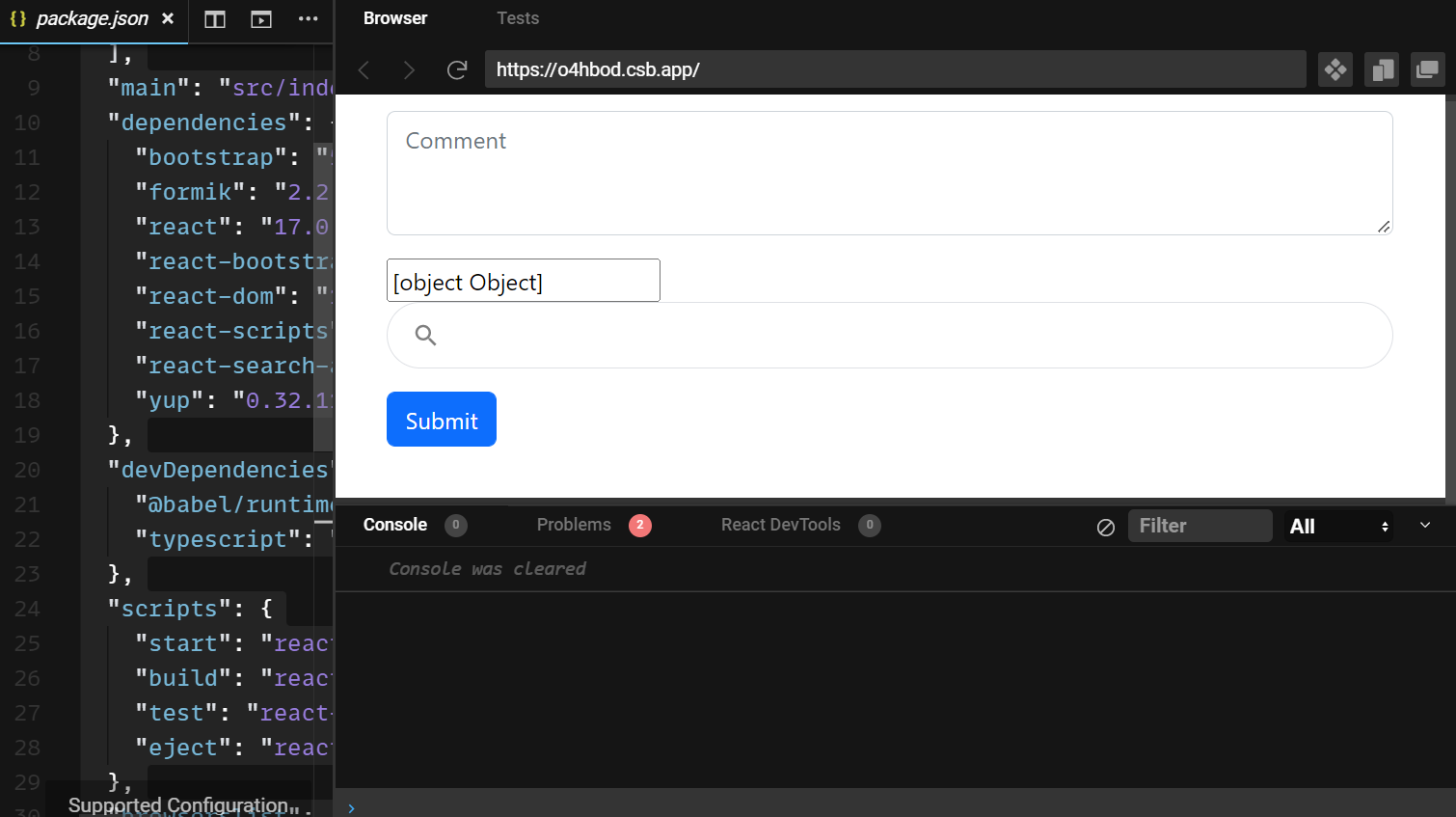
Additional context
Add any other context about the problem here.
The text was updated successfully, but these errors were encountered: