You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
UITableViewDelegate&UITableViewDelegate
通过右侧边栏点-> Show the connections inspector可以看到IDE已经帮我们设置好了UITableViewDelegate和UITableViewDelegate
还有一个prefetchDataSource不知道是干啥的,这个我后面慢慢捋。
// this protocol can provide information about cells before they are displayed on screen.
public protocol UITableViewDataSourcePrefetching : NSObjectProtocol {
// indexPaths are ordered ascending by geometric distance from the table view
@available(iOS 2.0, *)
func tableView(_ tableView: UITableView, prefetchRowsAt indexPaths: [IndexPath])
// indexPaths that previously were considered as candidates for pre-fetching, but were not actually used; may be a subset of the previous call to -tableView:prefetchRowsAtIndexPaths:
@available(iOS 2.0, *)
optional func tableView(_ tableView: UITableView, cancelPrefetchingForRowsAt indexPaths: [IndexPath])
}
这个接口是预加载接口
第一个接口是返回要预加载数据的索引,第二个接口是取消预加载的数据索引
说的好像那么一回事,但是怎么都不“直观”
直接上代码加Log来看看两个api的效果
首先把prefetchDataSource关联上ViewController
func tableView(_ tableView:UITableView, prefetchRowsAt indexPaths:[IndexPath]){print("prefetchRowsAt rows:\(productIndexStr( indexPaths))")}func tableView(_ tableView:UITableView, cancelPrefetchingForRowsAt indexPaths:[IndexPath]){print("cancelPrefetchingForRowsAt rows:\(productIndexStr( indexPaths))")}func productIndexStr( _ indexPaths:[IndexPath])->String{varindexStr=""
for index in indexPaths{
indexStr +="\(index.row)"}return indexStr.trimmingCharacters(in:.whitespaces)}
前言
UITableViewController虽然在前面的练习中有接触,但是并没做过系统性总结。
这篇的blog是怼UITableViewController基本用法的归纳。以及后面我想要做一个相对难一点的动态加载数据的一个预热,算是一个过渡章节吧
UITableView
首先UITableViewController和UITableView是配对一起使用的,和前面的控件有点不一样。而且是直接是以ViewController这个相对更高阶一点的层次。和它同级的都是Navigation Controller 和 Tab Controller这种。
这是一个真正意义上的数据集合控件,和数据紧密联系在一起,可以创建动态视图
和下面的控件类似
WPF:ItemSource、ListView
Andriod:RecyclerView
最简实现
我们还是通过Storyboard的接口来创建
删掉默认的UIViewController
直接+ UITableViewController,拖动到预览图里面
设置好 “Is Intial View Controller” 设为 true
修改ViewController的基类
修改继承 “UIViewController” 改为 UITableViewController
在Storyboard里面修改UITableViewController
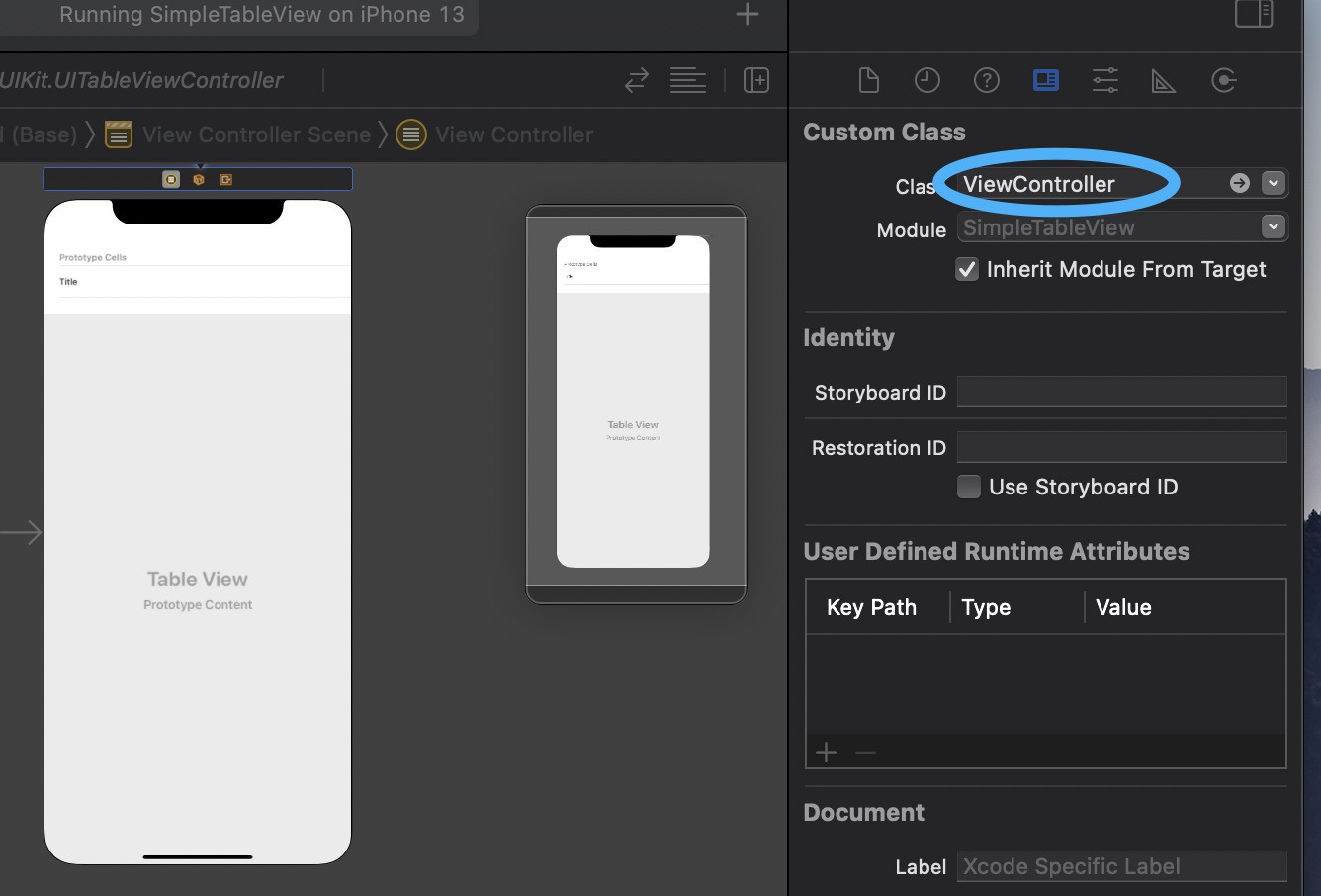
再回到Main.storyboard 的IDE
把关联的Class 从UITableViewController改到我们前面改的“ViewContoller”
注:ViewController基类没改之前是上不去的
修改Table Cell
通过IDE,Style改为“Basic”,Identifier加上“Item”为了以后Code的时候关联
UITableViewDelegate&UITableViewDelegate
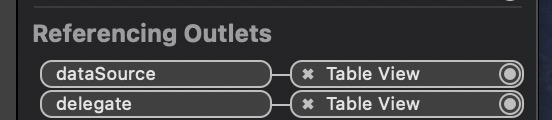
通过右侧边栏点-> Show the connections inspector可以看到IDE已经帮我们设置好了UITableViewDelegate和UITableViewDelegate
还有一个prefetchDataSource不知道是干啥的,这个我后面慢慢捋。
UITableViewDelegate都到ViewContoller上
再转到UITableViewController定义
由此可见UITableViewController是UIViewController的派生类,同时已经实现了UITableViewDelegate,UITableViewDataSource。
这里如果你看一些相对“老”一点的教程,UITableViewDataSource和UITableViewDataSource都要自己定义的。新版Swift帮我们整合一块了方便很多。
UITableViewController虽然实现了这两个接口,但是只是空载实现。真正和业务数据的绑定还需要我们进行override掉
为了省事,随便弄点数据
完整的签名是 tableView(_ tableView: UITableView, numberOfRowsInSection section: Int) -> Int ,swift的函数命名有点意思,表意不再函数名而是参数名,这是要告诉UI,一共要显示多少行
其中numberOfRowsInSection是章节,我们这里做single section,所以我们这里不care当前section的值(永远0)
这个函数是实现怎么呈现一个单元格
这里要说明下,tableView默认已经实现“虚拟化UI”的,每个cell都是复用的,所以我们需要调用dequeueReusableCell方法从单元格池里面去拿,有点类似线程池一样的概念。
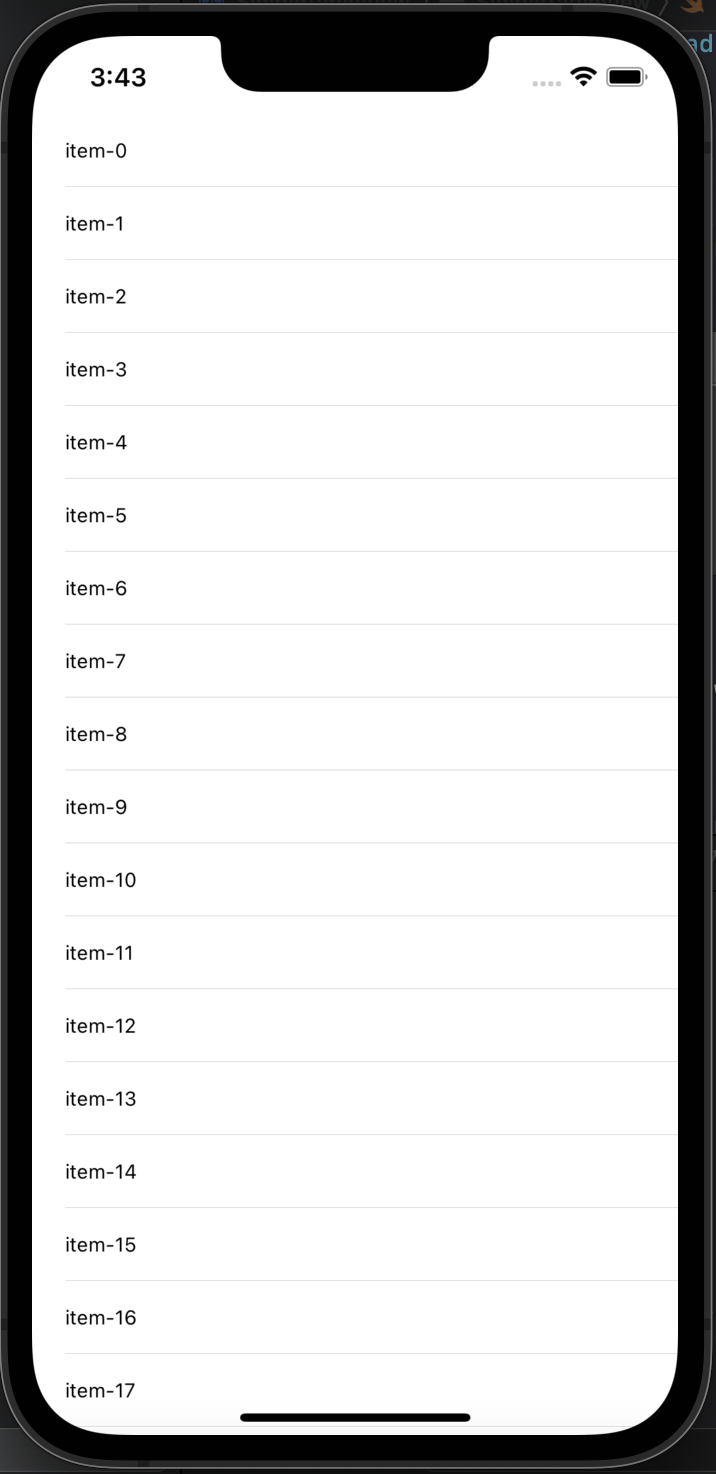
直接显示下文字结束
加餐 UITableViewDataSourcePrefetching
前面说了prefetchDataSource不知道干啥的
不如直接看代码
这个接口是预加载接口
第一个接口是返回要预加载数据的索引,第二个接口是取消预加载的数据索引
说的好像那么一回事,但是怎么都不“直观”
直接上代码加Log来看看两个api的效果
首先把prefetchDataSource关联上ViewController
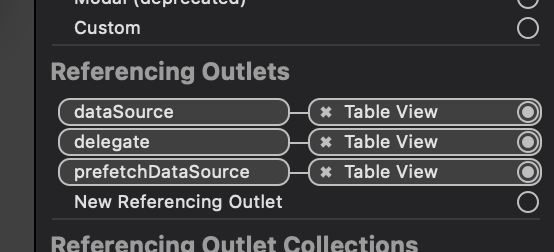
swif的collection好像没有Linq的Aggregate函数这种写法的,只能for了接下来看看效果
日志空空如也,说明初始化的那批数据并不会触发预加载。界面上显示了17条数据
往下拉
再拉到top
基本上可以看出来,他是对加载数据进行了一个“预测”
这个对静态数据没用,对动态加载数据有用
The text was updated successfully, but these errors were encountered: