Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat(instrumenter): add excludedMutations support (#2513)
Add support to configure `mutator.excludedMutations`. This was already supported in Stryker@<3, but not yet in Stryker4 beta. Instead of not generating the mutants, it generates them without placing them in the code. They get reported as `ignored` in your mutation testing report. 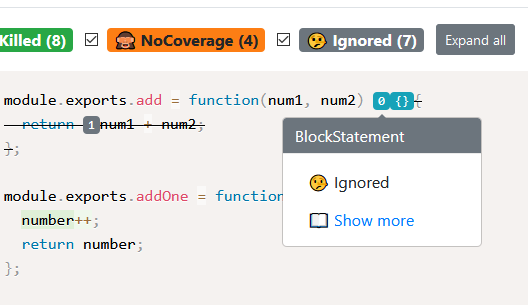 Also, add error message, ignore reason, and "killed by" test name in the "show more" modal dialog. 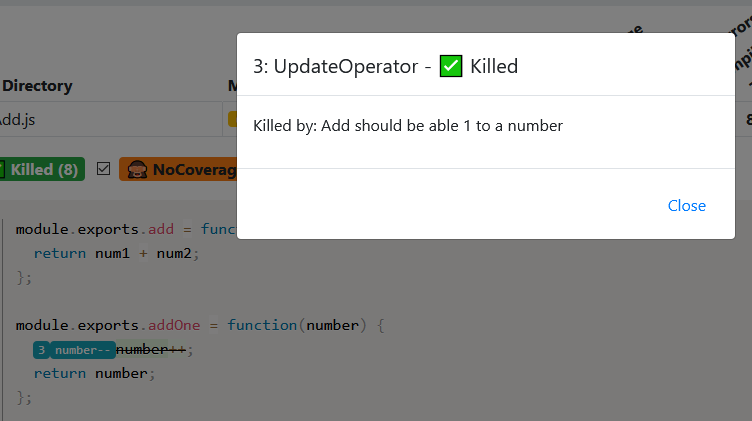
- Loading branch information
Showing
48 changed files
with
637 additions
and
164 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,4 @@ | ||
{ | ||
"require": "./test/helpers/testSetup.js", | ||
"spec": ["test/unit/*.js"] | ||
} |
Some generated files are not rendered by default. Learn more about how customized files appear on GitHub.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,11 @@ | ||
{ | ||
"name": "ignore-project", | ||
"description": "e2e test for different ignored mutants", | ||
"version": "0.0.0", | ||
"private": true, | ||
"scripts": { | ||
"test:unit": "mocha", | ||
"test": "stryker run", | ||
"posttest": "mocha --no-config --require ../../tasks/ts-node-register.js verify/*.ts" | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
module.exports.add = function(num1, num2) { | ||
return num1 + num2; | ||
}; | ||
|
||
module.exports.addOne = function(number) { | ||
number++; | ||
return number; | ||
}; | ||
|
||
module.exports.negate = function(number) { | ||
return -number; | ||
}; | ||
|
||
module.exports.notCovered = function(number) { | ||
return number > 10; | ||
}; | ||
|
||
module.exports.isNegativeNumber = function(number) { | ||
var isNegative = false; | ||
if(number < 0){ | ||
isNegative = true; | ||
} | ||
return isNegative; | ||
}; | ||
|
||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
module.exports.getCircumference = function(radius) { | ||
//Function to test multiple math mutations in a single function. | ||
return 2 * Math.PI * radius; | ||
}; | ||
|
||
module.exports.untestedFunction = function() { | ||
var i = 5 / 2 * 3; | ||
}; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,18 @@ | ||
{ | ||
"$schema": "../../node_modules/@stryker-mutator/core/schema/stryker-schema.json", | ||
"testRunner": "mocha", | ||
"concurrency": 2, | ||
"coverageAnalysis": "perTest", | ||
"mutator": { | ||
"excludedMutations": ["ArithmeticOperator", "BlockStatement"] | ||
}, | ||
"reporters": [ | ||
"clear-text", | ||
"html", | ||
"event-recorder" | ||
], | ||
"plugins": [ | ||
"@stryker-mutator/mocha-runner" | ||
], | ||
"allowConsoleColors": false | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
exports.mochaHooks = { | ||
beforeAll() { | ||
global.expect = require('chai').expect; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
const { expect } = require('chai'); | ||
const { add, addOne, isNegativeNumber, negate, notCovered } = require('../../src/Add'); | ||
|
||
describe('Add', function () { | ||
it('should be able to add two numbers', function () { | ||
var num1 = 2; | ||
var num2 = 5; | ||
var expected = num1 + num2; | ||
|
||
var actual = add(num1, num2); | ||
|
||
expect(actual).to.be.equal(expected); | ||
}); | ||
|
||
it('should be able 1 to a number', function () { | ||
var number = 2; | ||
var expected = 3; | ||
|
||
var actual = addOne(number); | ||
|
||
expect(actual).to.be.equal(expected); | ||
}); | ||
|
||
it('should be able negate a number', function () { | ||
var number = 2; | ||
var expected = -2; | ||
|
||
var actual = negate(number); | ||
|
||
expect(actual).to.be.equal(expected); | ||
}); | ||
|
||
it('should be able to recognize a negative number', function () { | ||
var number = -2; | ||
|
||
var isNegative = isNegativeNumber(number); | ||
|
||
expect(isNegative).to.be.true; | ||
}); | ||
|
||
it('should be able to recognize that 0 is not a negative number', function () { | ||
var number = 0; | ||
|
||
var isNegative = isNegativeNumber(number); | ||
|
||
expect(isNegative).to.be.false; | ||
}); | ||
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,13 @@ | ||
const { expect } = require('chai'); | ||
const { getCircumference } = require('../../src/Circle'); | ||
|
||
describe('Circle', function () { | ||
it('should have a circumference of 2PI when the radius is 1', function () { | ||
var radius = 1; | ||
var expectedCircumference = 2 * Math.PI; | ||
|
||
var circumference = getCircumference(radius); | ||
|
||
expect(circumference).to.be.equal(expectedCircumference); | ||
}); | ||
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
import { expectMetrics } from '../../../helpers'; | ||
|
||
describe('After running stryker on jest-react project', () => { | ||
it('should report expected scores', async () => { | ||
await expectMetrics({ | ||
killed: 8, | ||
ignored: 13, | ||
mutationScore: 66.67, | ||
}); | ||
/* | ||
-----------|---------|----------|-----------|------------|----------|---------| | ||
File | % score | # killed | # timeout | # survived | # no cov | # error | | ||
-----------|---------|----------|-----------|------------|----------|---------| | ||
All files | 66.67 | 8 | 0 | 0 | 4 | 0 |*/ | ||
}); | ||
}); |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,13 +1,38 @@ | ||
import Range from './Range'; | ||
import Location from './Location'; | ||
|
||
/** | ||
* Represents a mutant | ||
*/ | ||
interface Mutant { | ||
/** | ||
* The id of the mutant. Unique within a run. | ||
*/ | ||
id: number; | ||
/** | ||
* The name of the mutator that generated this mutant. | ||
*/ | ||
mutatorName: string; | ||
/** | ||
* The file name from which this mutant originated | ||
*/ | ||
fileName: string; | ||
/** | ||
* The range of this mutant (from/to within the file) | ||
*/ | ||
range: Range; | ||
/** | ||
* The line number/column location of this mutant | ||
*/ | ||
location: Location; | ||
/** | ||
* The replacement (actual mutated code) | ||
*/ | ||
replacement: string; | ||
/** | ||
* If the mutant was ignored during generation, the reason for ignoring it, otherwise `undefined` | ||
*/ | ||
ignoreReason?: string; | ||
} | ||
|
||
export default Mutant; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,6 +1,6 @@ | ||
/** | ||
* Represents a location in a file by range [fromInclusive, toExclusive] | ||
* Represents a location in a file by range. | ||
*/ | ||
type Range = [number, number]; | ||
type Range = [fromInclusive: number, toExclusive: number]; | ||
|
||
export default Range; |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.