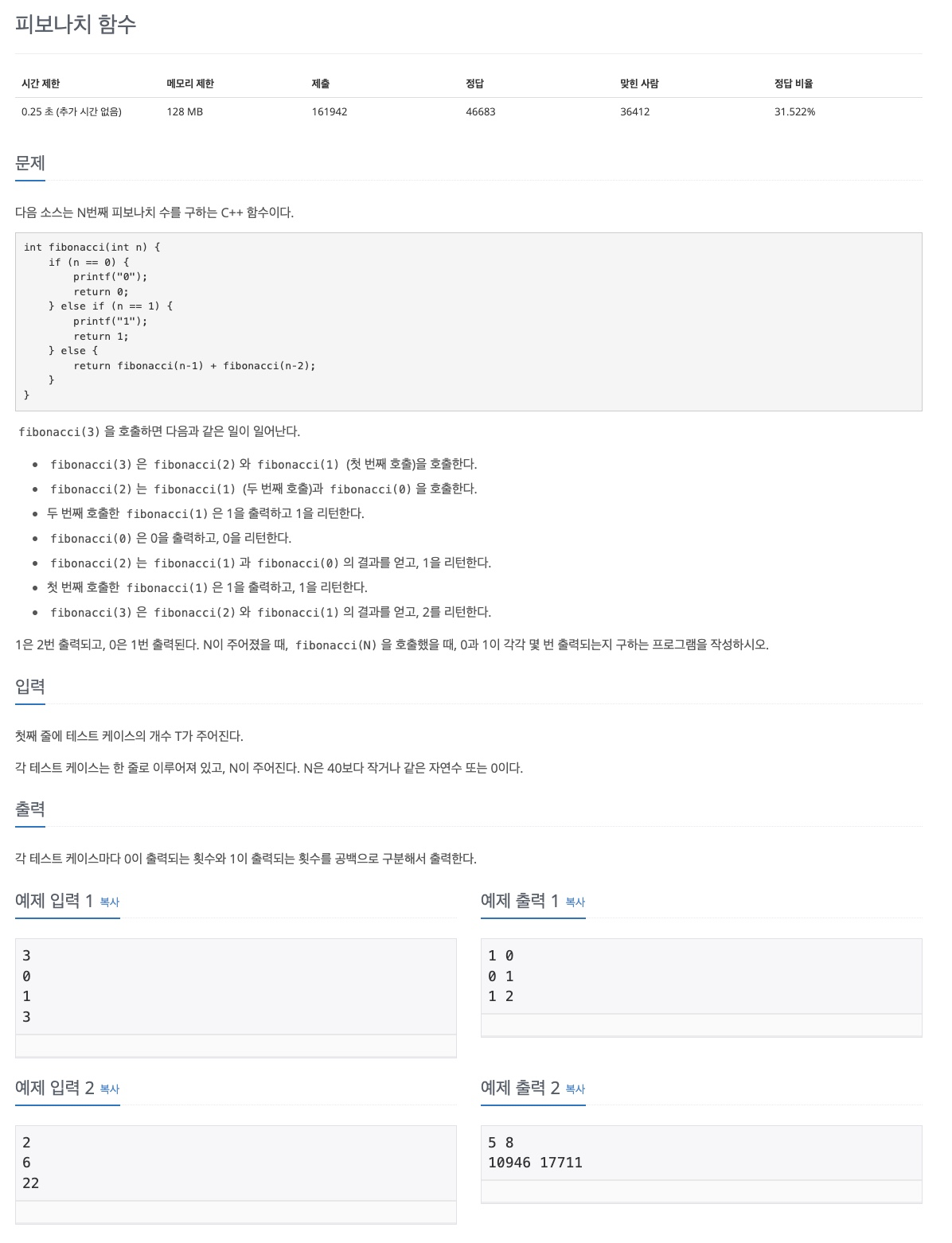
let T: Int = Int(readLine()!)!
var tc: Int
var result: [Int]
for _ in 0..<T {
tc = Int(readLine()!)!
result = [1, 0]
if tc != 0 {
for _ in 0..<tc {
result[1] = result[0] + result[1]
result[0] = result[1] - result[0]
}
}
print("\(result[0]) \(result[1])")
}
package BOJ_study;
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class Main_BOJ_1003_피보나치함수_0628 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int[] fibo0 = new int[41];
int[] fibo1 = new int[41];
fibo0[0] = 1;
fibo0[1] = 0;
fibo1[0] = 0;
fibo1[1] = 1;
for(int i=2; i<=40; i++) {
fibo0[i] = fibo0[i-1] + fibo0[i-2];
fibo1[i] = fibo1[i-1] + fibo1[i-2];
}
int TC = Integer.parseInt(br.readLine());
for(int tc=0; tc<TC; tc++) {
int N = Integer.parseInt(br.readLine());
System.out.println(fibo0[N] + " " + fibo1[N]);
}
}
}
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
public class BOJ_1003 {
public static void main(String[] args) throws IOException {
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
int[][] fibo = new int[41][2];
fibo[0][0] = 1;
fibo[0][1] = 0;
fibo[1][0] = 0;
fibo[1][1] = 1;
for(int i=2; i<=40; i++){
fibo[i][0] = fibo[i-1][0] + fibo[i-2][0];
fibo[i][1] = fibo[i-1][1] + fibo[i-2][1];
}
int T = Integer.parseInt(br.readLine());
for(int t=0; t<T; t++){
int N = Integer.parseInt(br.readLine());
System.out.println(fibo[N][0] + " " + fibo[N][1]);
}
}
}
import java.io.BufferedReader;
import java.io.BufferedWriter;
import java.io.IOException;
import java.io.InputStreamReader;
import java.io.OutputStreamWriter;
import java.util.StringTokenizer;
//BOJ_1003_피보나치 함수
class Main{
/*
0 | 1 0
1 | 0 1
2 | 1 1
3 | 1 2
4 | 2 3
5 | 3 5
6 | 5 8
7 | 8 13
*/
public static void main(String[] args) throws IOException{
BufferedReader br = new BufferedReader(new InputStreamReader(System.in));
BufferedWriter bw = new BufferedWriter(new OutputStreamWriter(System.out));
StringTokenizer st = new StringTokenizer(br.readLine(), " ");
int T = Integer.parseInt(st.nextToken());
int fibo[] = fibo(41); //41까지의 피보나치 구하기
for (int tc = 1; tc<=T; tc++){
int N = Integer.parseInt(br.readLine());
if (N == 0) System.out.println(1 + " " + 0); //0인 경우 0 1번 출력
else if(N==1) System.out.println( 0 + " " + 1); //1인 경우 1 한번 출력
else System.out.println(fibo[N-1] + " " + fibo[N]); //나머지는 피보나치와 동일
}
bw.flush();
bw.close();
br.close();
}
public static int[] fibo(int targetNum){ //41까지의 피보나치
int fibo[] = new int[targetNum+1];
for (int i = 0; i<targetNum; i++){
if (i==0) {
fibo[i] = 0;
}else if(i==1){
fibo[i] = 1;
}
else fibo[i] = fibo[i-1] + fibo[i-2];
}
return fibo;
}
}