Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Auto merge of #85292 - wesleywiser:enum_debuginfo, r=michaelwoerister
Improve debugging experience for enums on windows-msvc This PR makes significant improvements over the status quo of debugging enums on the windows-msvc platform with either WinDbg or Visual Studio in three ways: 1. Improves the debugger experience for directly tagged enums. 2. Fixes a bug which caused the debugger to sometimes show the wrong debug info for niche layout enums. For example, `Option<&u32>` could sometimes use the debug info for `Option<&f64>` instead leading to nonsensical variable values in the debugger. 3. Significantly improves the debugger experience for niche-layout enums. Let's look at a few examples: ```rust pub enum CStyleEnum { Base = 2, Exponent = 16, } pub enum NicheLayoutEnum { Tag1, Data { my_data: CStyleEnum }, Tag2, Tag3, Tag4, } pub enum OtherEnum<T> { Case1(T), Case2(T), } fn main() { let a = Some(CStyleEnum::Base); let b = Option::<CStyleEnum>::None; let c = NicheLayoutEnum::Tag1; let d = NicheLayoutEnum::Data { my_data: CStyleEnum::Exponent }; let e = NicheLayoutEnum::Tag2; let f = Some(&1u32); let g = Option::<&'static u32>::None; let h = Some(&2u64); let i = Option::<&'static u64>::None; let j = Some(12u32); let k = Option::<u32>::None; let l = Some(12.34f64); let m = Option::<f64>::None; let n = CStyleEnum::Base; let o = CStyleEnum::Exponent; let p = Some("IAMA optional string!".to_string()); let q = OtherEnum::Case1(42u32); } ``` This is what WinDbg Preview shows using the latest rustc nightly: 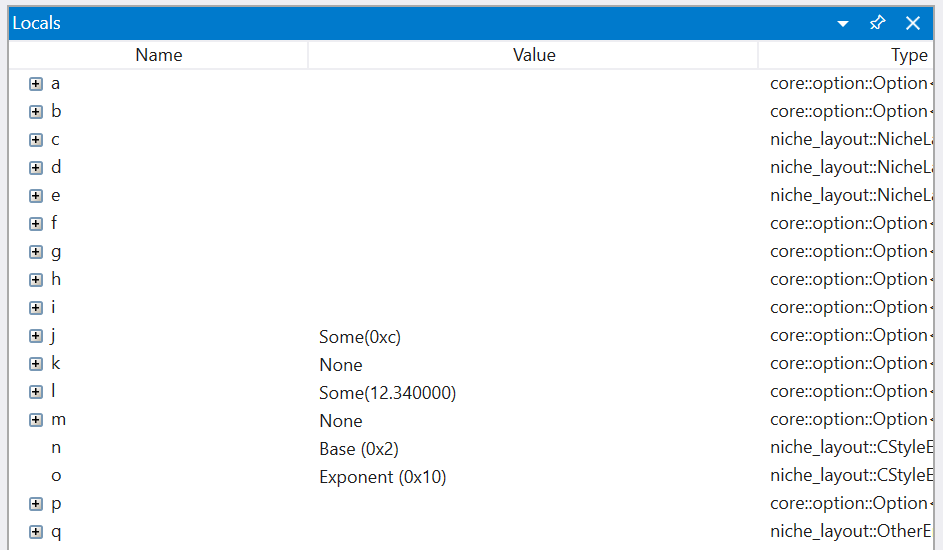 Most of the variables don't show a meaningful value expect for a few cases that we have targeted natvis definitions covering. Even worse, drilling into many of these variables shows information that can be difficult to interpret without an understanding of the layout of Rust types: 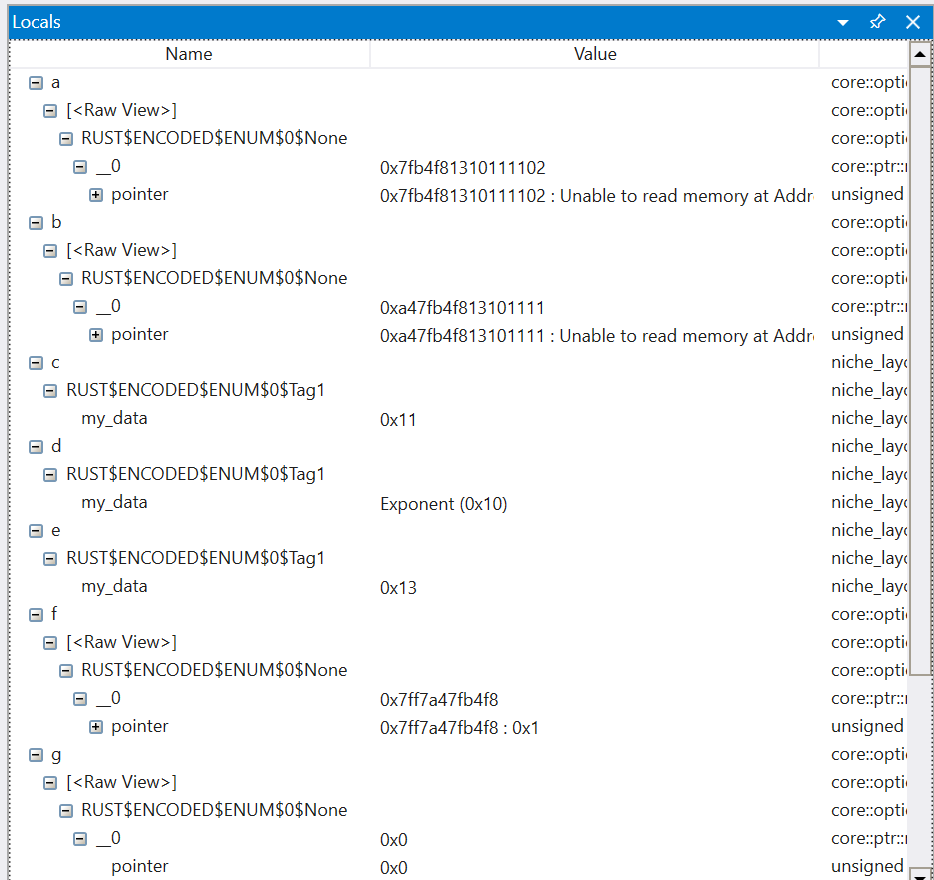 With the changes in this PR, we're able to write two natvis definitions that cover all enum cases generally. After building with these changes, WinDbg now shows this instead: 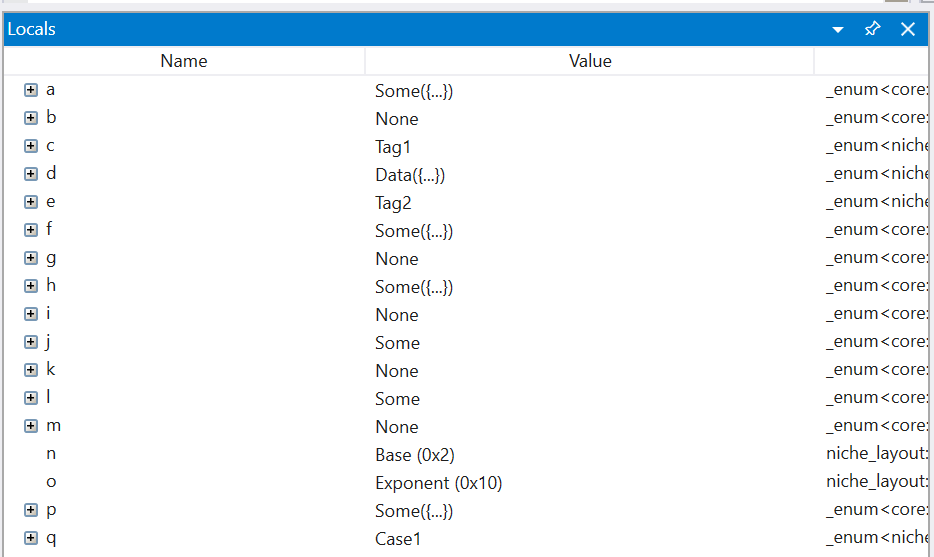 Drilling into the same variables, we can see much more useful information: 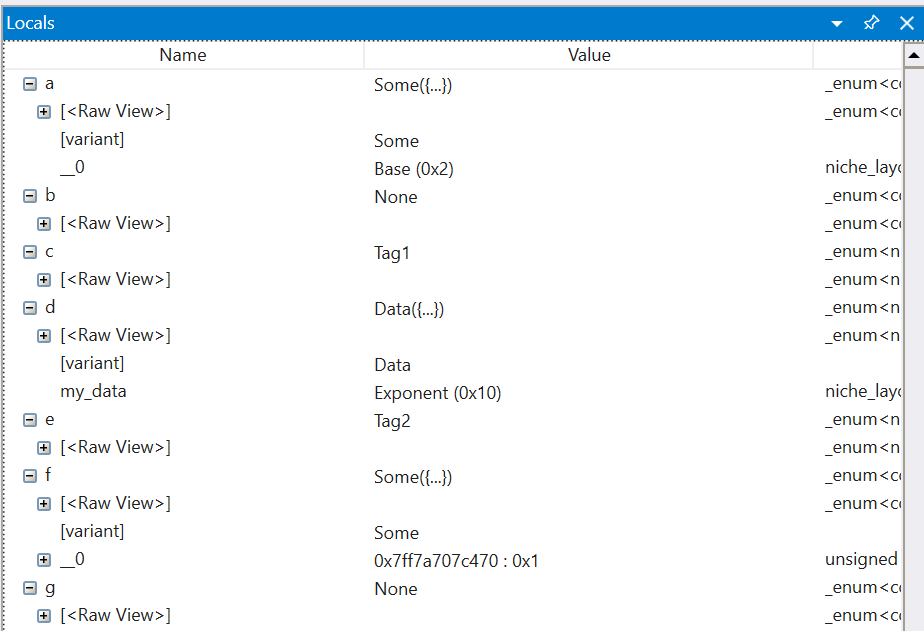 Fixes #84670 Fixes #84671
- Loading branch information
Showing
9 changed files
with
376 additions
and
142 deletions.
There are no files selected for viewing
251 changes: 149 additions & 102 deletions
251
compiler/rustc_codegen_llvm/src/debuginfo/metadata.rs
Large diffs are not rendered by default.
Oops, something went wrong.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,97 @@ | ||
// only-cdb | ||
// ignore-tidy-linelength | ||
// compile-flags:-g | ||
|
||
// cdb-command: g | ||
|
||
// Note: The natvis used to visualize niche-layout enums don't work correctly in cdb | ||
// so the best we can do is to make sure we are generating the right debuginfo | ||
|
||
// cdb-command: dx -r2 a,! | ||
// cdb-check:a,! [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>::Some] | ||
// cdb-check: [+0x000] __0 : Low (0x2) [Type: msvc_pretty_enums::CStyleEnum] | ||
// cdb-check: [+0x000] discriminant : 0x2 [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 b,! | ||
// cdb-check:b,! [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>::Some] | ||
// cdb-check: [+0x000] __0 : 0x11 [Type: msvc_pretty_enums::CStyleEnum] | ||
// cdb-check: [+0x000] discriminant : None (0x11) [Type: enum$<core::option::Option<enum$<msvc_pretty_enums::CStyleEnum>>, 2, 16, Some>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 c,! | ||
// cdb-check:c,! [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Data] | ||
// cdb-check: [+0x000] my_data : 0x11 [Type: msvc_pretty_enums::CStyleEnum] | ||
// cdb-check: [+0x000] discriminant : Tag1 (0x11) [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 d,! | ||
// cdb-check:d,! [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Data] | ||
// cdb-check: [+0x000] my_data : High (0x10) [Type: msvc_pretty_enums::CStyleEnum] | ||
// cdb-check: [+0x000] discriminant : 0x10 [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 e,! | ||
// cdb-check:e,! [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Data] | ||
// cdb-check: [+0x000] my_data : 0x13 [Type: msvc_pretty_enums::CStyleEnum] | ||
// cdb-check: [+0x000] discriminant : Tag2 (0x13) [Type: enum$<msvc_pretty_enums::NicheLayoutEnum, 2, 16, Data>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 f,! | ||
// cdb-check:f,! [Type: enum$<core::option::Option<u32*>, 1, [...], Some>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<core::option::Option<u32*>, 1, [...], Some>::Some] | ||
// cdb-check: [+0x000] __0 : 0x[...] : 0x1 [Type: unsigned int *] | ||
// cdb-check: [+0x000] discriminant : 0x[...] [Type: enum$<core::option::Option<u32*>, 1, [...], Some>::Discriminant$] | ||
|
||
// cdb-command: dx -r2 g,! | ||
// cdb-check:g,! [Type: enum$<core::option::Option<u32*>, 1, [...], Some>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<core::option::Option<u32*>, 1, [...], Some>::Some] | ||
// cdb-check: [+0x000] __0 : 0x0 [Type: unsigned int *] | ||
// cdb-check: [+0x000] discriminant : None (0x0) [Type: enum$<core::option::Option<u32*>, 1, [...], Some>::Discriminant$] | ||
|
||
// cdb-command: dx h | ||
// cdb-check:h : Some [Type: enum$<core::option::Option<u32>>] | ||
// cdb-check: [+0x000] variant$ : Some (0x1) [Type: core::option::Option] | ||
// cdb-check: [+0x004] __0 : 0xc [Type: unsigned int] | ||
|
||
// cdb-command: dx i | ||
// cdb-check:i : None [Type: enum$<core::option::Option<u32>>] | ||
// cdb-check: [+0x000] variant$ : None (0x0) [Type: core::option::Option] | ||
|
||
// cdb-command: dx j | ||
// cdb-check:j : High (0x10) [Type: msvc_pretty_enums::CStyleEnum] | ||
|
||
// cdb-command: dx -r2 k,! | ||
// cdb-check:k,! [Type: enum$<core::option::Option<alloc::string::String>, 1, [...], Some>] | ||
// cdb-check: [+0x000] dataful_variant [Type: enum$<core::option::Option<alloc::string::String>, 1, [...], Some>::Some] | ||
// cdb-check: [+0x000] __0 [Type: alloc::string::String] | ||
// cdb-check: [+0x000] discriminant : 0x[...] [Type: enum$<core::option::Option<alloc::string::String>, 1, [...], Some>::Discriminant$] | ||
|
||
pub enum CStyleEnum { | ||
Low = 2, | ||
High = 16, | ||
} | ||
|
||
pub enum NicheLayoutEnum { | ||
Tag1, | ||
Data { my_data: CStyleEnum }, | ||
Tag2, | ||
} | ||
|
||
fn main() { | ||
let a = Some(CStyleEnum::Low); | ||
let b = Option::<CStyleEnum>::None; | ||
let c = NicheLayoutEnum::Tag1; | ||
let d = NicheLayoutEnum::Data { my_data: CStyleEnum::High }; | ||
let e = NicheLayoutEnum::Tag2; | ||
let f = Some(&1u32); | ||
let g = Option::<&'static u32>::None; | ||
let h = Some(12u32); | ||
let i = Option::<u32>::None; | ||
let j = CStyleEnum::High; | ||
let k = Some("IAMA optional string!".to_string()); | ||
|
||
zzz(); // #break | ||
} | ||
|
||
fn zzz() { () } |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters