Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #26833 [HttpKernel] Added support for timings in ArgumentValu…
…eResolvers (iltar) This PR was squashed before being merged into the 4.1-dev branch (closes #26833). Discussion ---------- [HttpKernel] Added support for timings in ArgumentValueResolvers | Q | A | ------------- | --- | Branch? | master | Bug fix? | no | New feature? | yes | BC breaks? | no | Deprecations? | no | Tests pass? | yes | Fixed tickets | ~ | License | MIT | Doc PR | ~ This feature provides timings for the supports and resolve methods for each individual argument value resolver. It was already possible to see the `controller.get_arguments` timing, but it was impossible to track a possible performance issue any further without using different tools. I've explicitly added the `supports` method as well, as it might otherwise hide performance issues. The `ServiceValueResolver` for example, does a `container::has`, which in turn might trigger a factory method, which might trigger a query for example. ~~Due to the feature freeze I've already added this to 4.2. If for some reason it's okay to still merge it into 4.1, I can update the upgrade files.~~ - Changed to 4.1 ##### *Without performance issue* 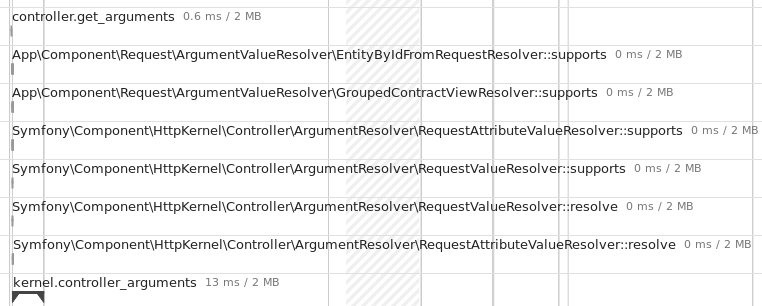 ##### *With performance issue* 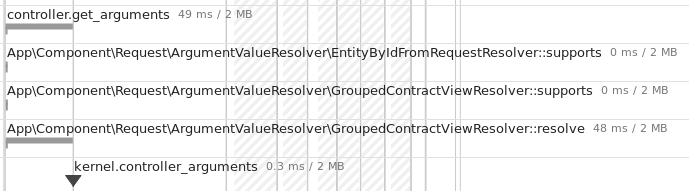 Commits ------- 1c0d892 [HttpKernel] Added support for timings in ArgumentValueResolvers
- Loading branch information
Showing
5 changed files
with
200 additions
and
1 deletion.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
62 changes: 62 additions & 0 deletions
62
src/Symfony/Component/HttpKernel/Controller/ArgumentResolver/TraceableValueResolver.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,62 @@ | ||
<?php | ||
|
||
/* | ||
* This file is part of the Symfony package. | ||
* | ||
* (c) Fabien Potencier <fabien@symfony.com> | ||
* | ||
* For the full copyright and license information, please view the LICENSE | ||
* file that was distributed with this source code. | ||
*/ | ||
|
||
namespace Symfony\Component\HttpKernel\Controller\ArgumentResolver; | ||
|
||
use Symfony\Component\HttpFoundation\Request; | ||
use Symfony\Component\HttpKernel\Controller\ArgumentValueResolverInterface; | ||
use Symfony\Component\HttpKernel\ControllerMetadata\ArgumentMetadata; | ||
use Symfony\Component\Stopwatch\Stopwatch; | ||
|
||
/** | ||
* Provides timing information via the stopwatch. | ||
* | ||
* @author Iltar van der Berg <kjarli@gmail.com> | ||
*/ | ||
final class TraceableValueResolver implements ArgumentValueResolverInterface | ||
{ | ||
private $inner; | ||
private $stopwatch; | ||
|
||
public function __construct(ArgumentValueResolverInterface $inner, ?Stopwatch $stopwatch = null) | ||
{ | ||
$this->inner = $inner; | ||
$this->stopwatch = $stopwatch ?? new Stopwatch(); | ||
} | ||
|
||
/** | ||
* {@inheritdoc} | ||
*/ | ||
public function supports(Request $request, ArgumentMetadata $argument): bool | ||
{ | ||
$method = \get_class($this->inner).'::'.__FUNCTION__; | ||
$this->stopwatch->start($method); | ||
|
||
$return = $this->inner->supports($request, $argument); | ||
|
||
$this->stopwatch->stop($method); | ||
|
||
return $return; | ||
} | ||
|
||
/** | ||
* {@inheritdoc} | ||
*/ | ||
public function resolve(Request $request, ArgumentMetadata $argument): iterable | ||
{ | ||
$method = \get_class($this->inner).'::'.__FUNCTION__; | ||
$this->stopwatch->start($method); | ||
|
||
yield from $this->inner->resolve($request, $argument); | ||
|
||
$this->stopwatch->stop($method); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
76 changes: 76 additions & 0 deletions
76
...ony/Component/HttpKernel/Tests/Controller/ArgumentResolver/TraceableValueResolverTest.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,76 @@ | ||
<?php | ||
|
||
/* | ||
* This file is part of the Symfony package. | ||
* | ||
* (c) Fabien Potencier <fabien@symfony.com> | ||
* | ||
* For the full copyright and license information, please view the LICENSE | ||
* file that was distributed with this source code. | ||
*/ | ||
|
||
namespace Symfony\Component\HttpKernel\Tests\Controller\ArgumentResolver; | ||
|
||
use PHPUnit\Framework\TestCase; | ||
use Symfony\Component\HttpFoundation\Request; | ||
use Symfony\Component\HttpKernel\Controller\ArgumentResolver\TraceableValueResolver; | ||
use Symfony\Component\HttpKernel\Controller\ArgumentValueResolverInterface; | ||
use Symfony\Component\HttpKernel\ControllerMetadata\ArgumentMetadata; | ||
use Symfony\Component\Stopwatch\Stopwatch; | ||
|
||
class TraceableValueResolverTest extends TestCase | ||
{ | ||
public function testTimingsInSupports() | ||
{ | ||
$stopwatch = new Stopwatch(); | ||
$resolver = new TraceableValueResolver(new ResolverStub(), $stopwatch); | ||
$argument = new ArgumentMetadata('dummy', 'string', false, false, null); | ||
$request = new Request(); | ||
|
||
$this->assertTrue($resolver->supports($request, $argument)); | ||
|
||
$event = $stopwatch->getEvent(ResolverStub::class.'::supports'); | ||
$this->assertCount(1, $event->getPeriods()); | ||
} | ||
|
||
public function testTimingsInResolve() | ||
{ | ||
$stopwatch = new Stopwatch(); | ||
$resolver = new TraceableValueResolver(new ResolverStub(), $stopwatch); | ||
$argument = new ArgumentMetadata('dummy', 'string', false, false, null); | ||
$request = new Request(); | ||
|
||
$iterable = $resolver->resolve($request, $argument); | ||
|
||
foreach ($iterable as $index => $resolved) { | ||
$event = $stopwatch->getEvent(ResolverStub::class.'::resolve'); | ||
$this->assertTrue($event->isStarted()); | ||
$this->assertEmpty($event->getPeriods()); | ||
switch ($index) { | ||
case 0: | ||
$this->assertEquals('first', $resolved); | ||
break; | ||
case 1: | ||
$this->assertEquals('second', $resolved); | ||
break; | ||
} | ||
} | ||
|
||
$event = $stopwatch->getEvent(ResolverStub::class.'::resolve'); | ||
$this->assertCount(1, $event->getPeriods()); | ||
} | ||
} | ||
|
||
class ResolverStub implements ArgumentValueResolverInterface | ||
{ | ||
public function supports(Request $request, ArgumentMetadata $argument) | ||
{ | ||
return true; | ||
} | ||
|
||
public function resolve(Request $request, ArgumentMetadata $argument) | ||
{ | ||
yield 'first'; | ||
yield 'second'; | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters