Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feature #24363 [Console] Modify console output and print multiple mod…
…ifyable sections (pierredup) This PR was squashed before being merged into the 4.1-dev branch (closes #24363). Discussion ---------- [Console] Modify console output and print multiple modifyable sections | Q | A | ------------- | --- | Branch? | 4.1 | Bug fix? | no | New feature? | yes | BC breaks? | no | Deprecations? | no | Tests pass? | TBD | Fixed tickets | | License | MIT | Doc PR | symfony/symfony-docs#9304 Add support to create different output sections for the console output. Each section is it's own 'stream' of output, where the output can be modified (even if there are other output after it). This allows you to modify previous output in the console, either by appending new lines, modifying previous lines or clearing the output. Modifying a sections output doesn't affect the output after that or in other sections. Some examples of what can be done: **Overwriting content in a previous section:** Code: ```php $section1 = $output->section(); $section2 = $output->section(); $section1->writeln("<comment>Doing something</comment>\n"); usleep(500000); $section2->writeln('<info>Result of first operation</info>'); usleep(500000); $section1->overwrite("<comment>Doing something else</comment>\n"); usleep(500000); $section2->writeln('<info>Result of second operation</info>'); usleep(500000); $section1->overwrite("<comment>Finishing</comment>\n"); usleep(500000); $section2->writeln('<info>Last Result</info>'); ``` Result: 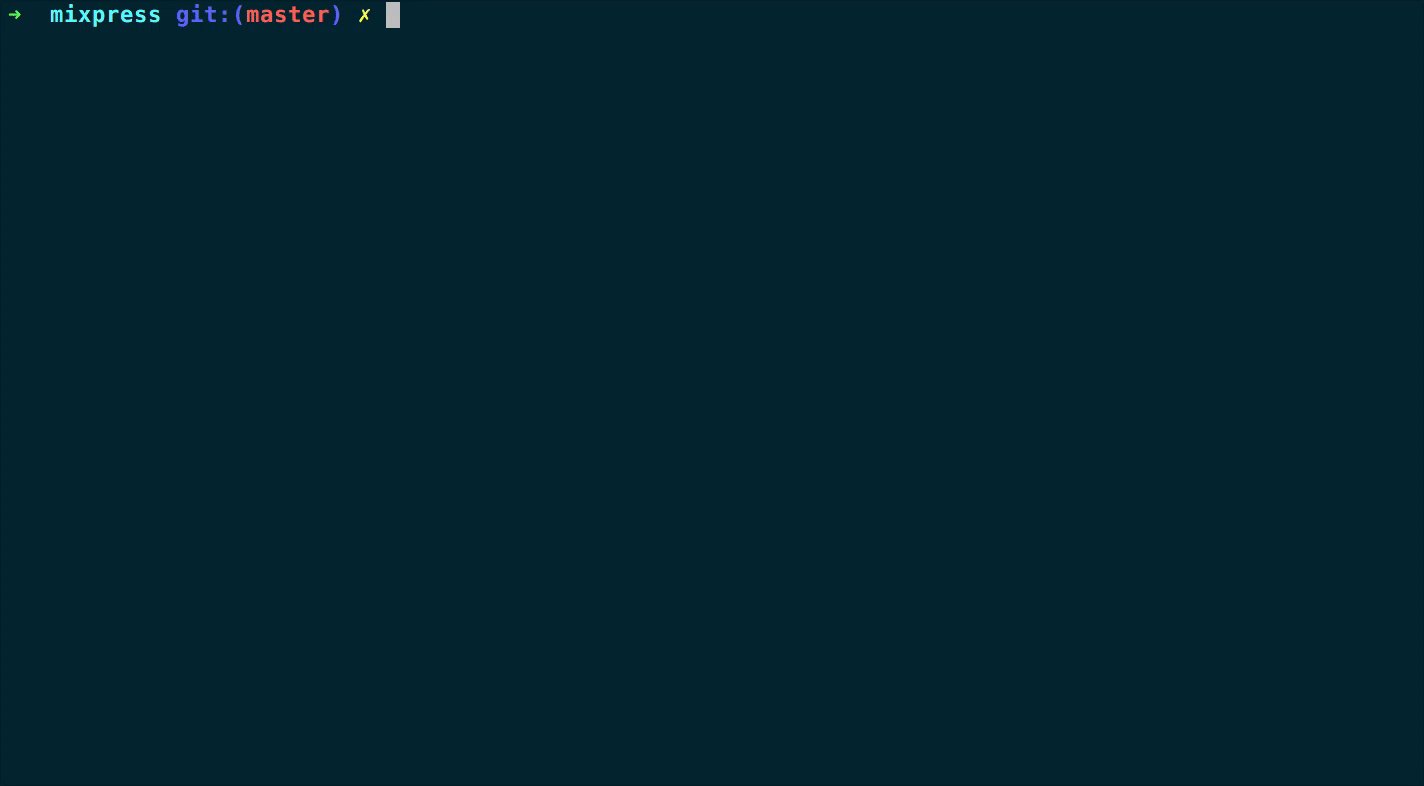 **Multiple Progress Bars:** Code: ```php $section1 = $output->section(); $section2 = $output->section(); $progress = new ProgressBar($section1); $progress2 = new ProgressBar($section2); $progress->start(100); $progress2->start(100); $c = 0; while (++$c < 100) { $progress->advance(); if ($c % 2 === 0) { $progress2->advance(4); } usleep(500000); } ``` Result: 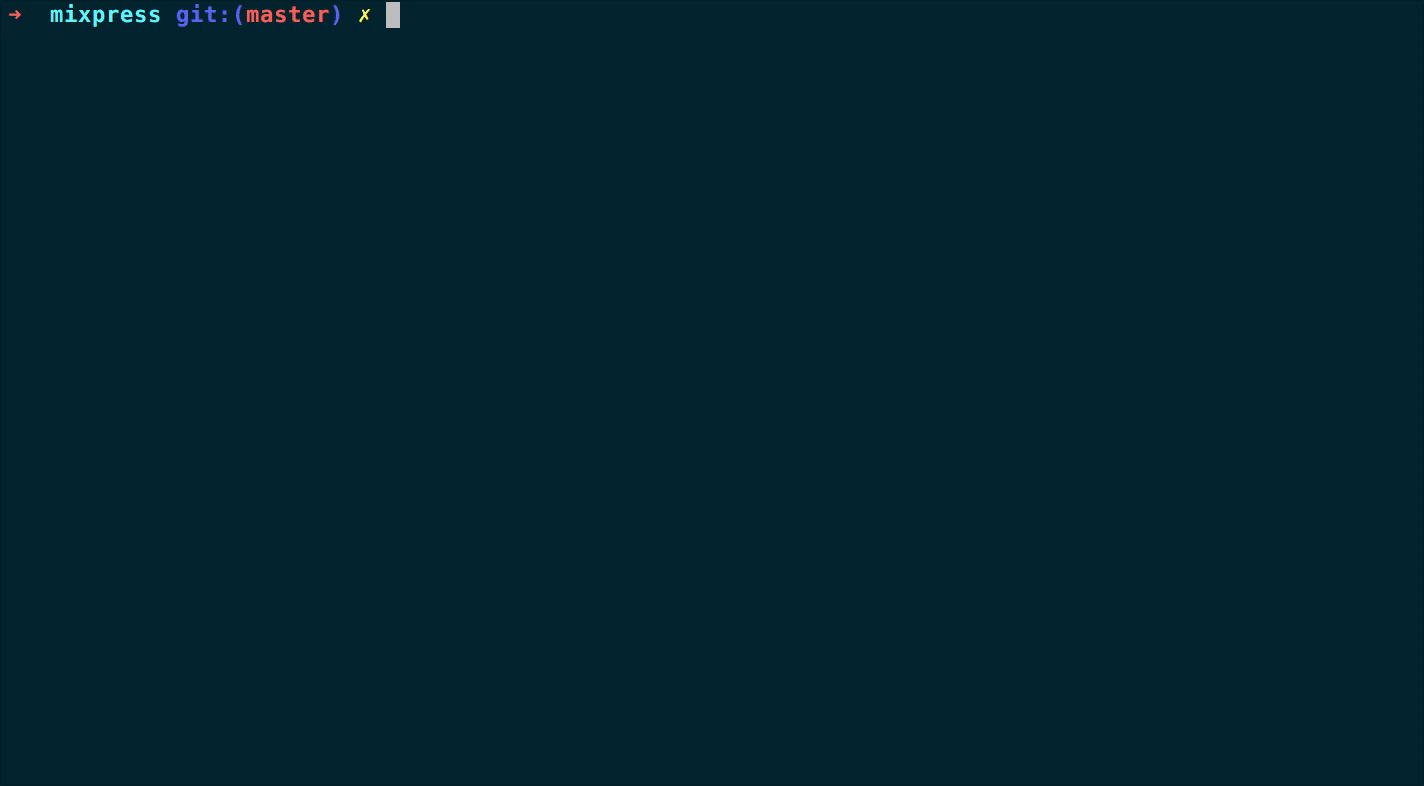 **Modifying content of a table & updating a progress bar:** Code: ```php $section1 = $output->section(); $section2 = $output->section(); $progress = new ProgressBar($section1); $table = new Table($section2); $table->addRow(['Row 1']); $table->render(); $progress->start(5); $c = 0; while (++$c < 5) { $table->appendRow(['Row '.($c + 1)]); $progress->advance(); usleep(500000); } $progress->finish(); $section1->clear(); ``` Result: 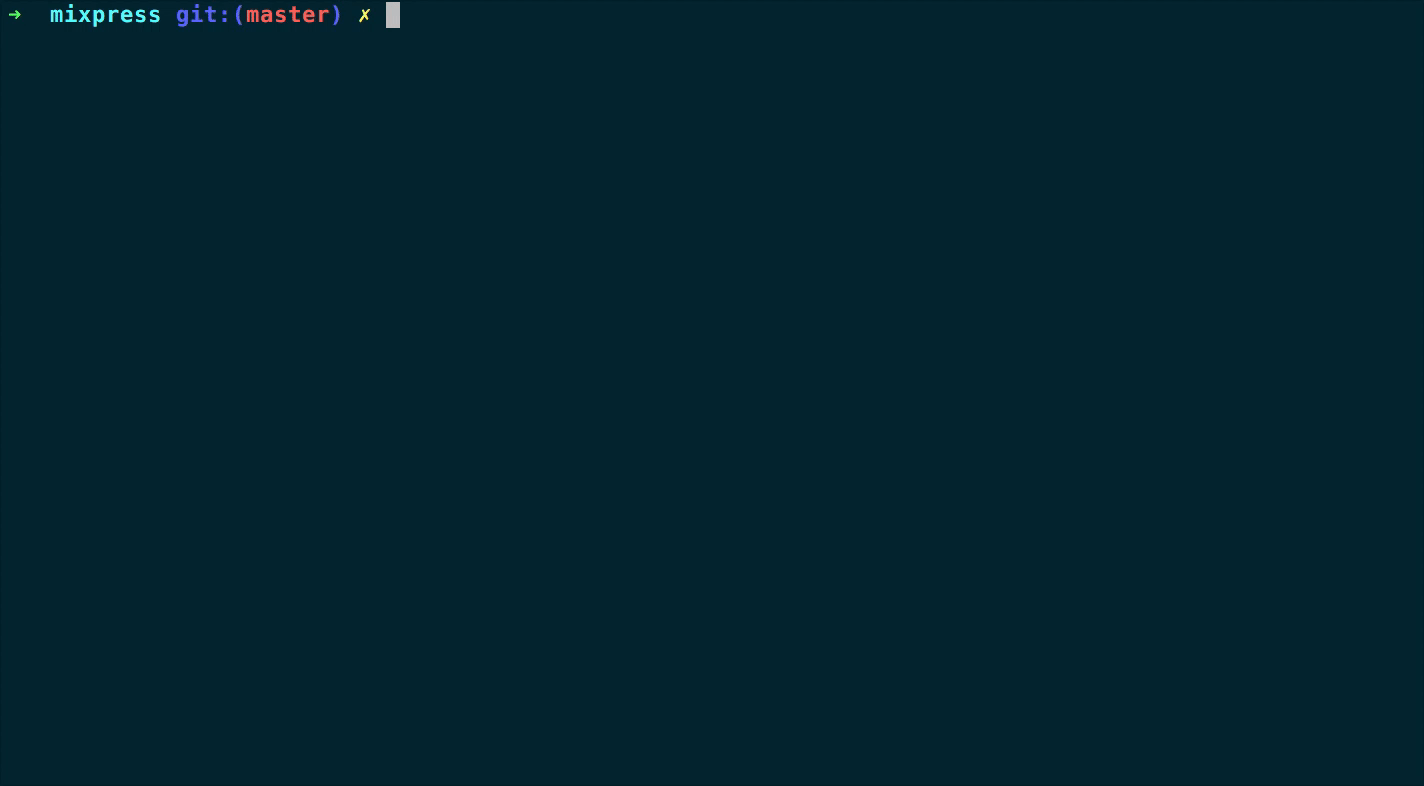 **Example with Symfony Installer:*** Before: 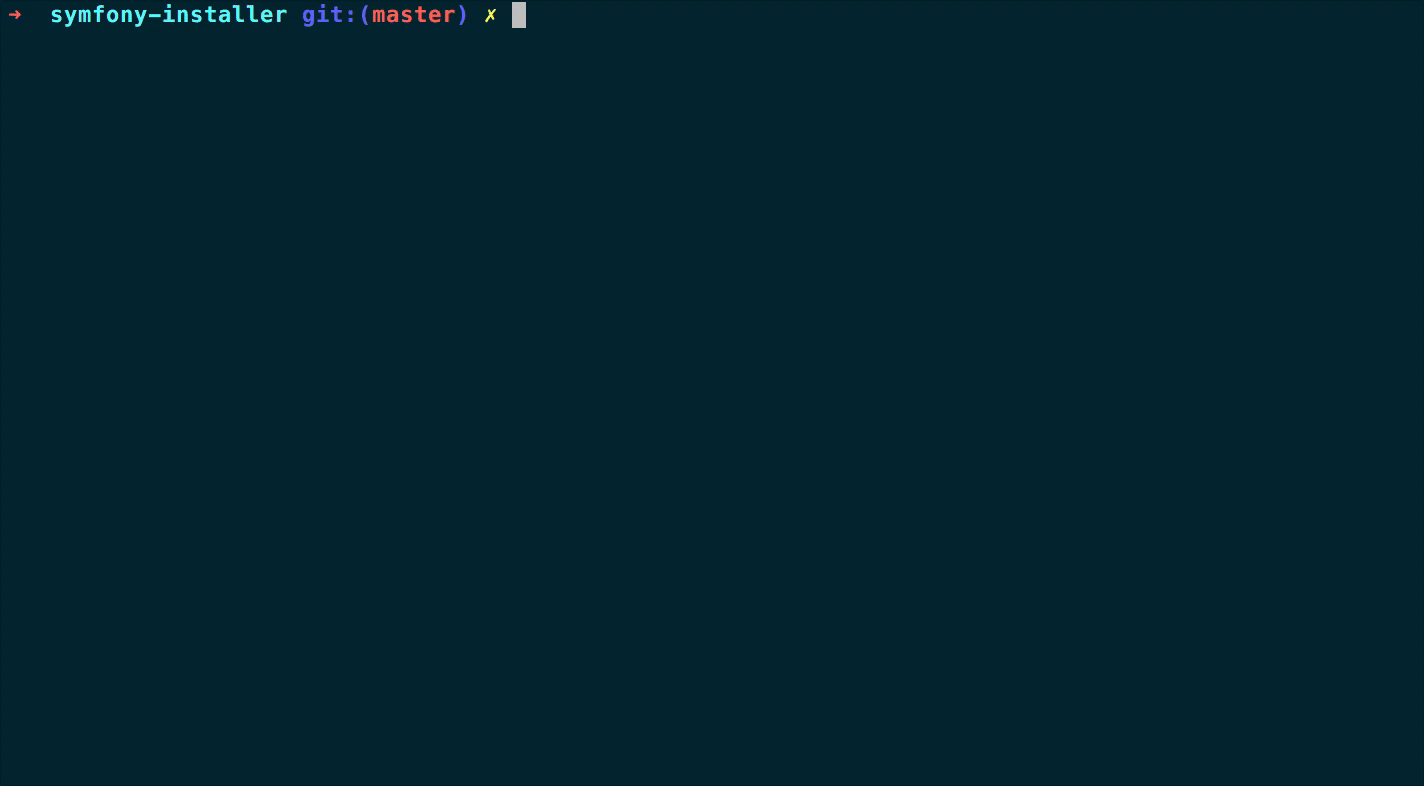 After: 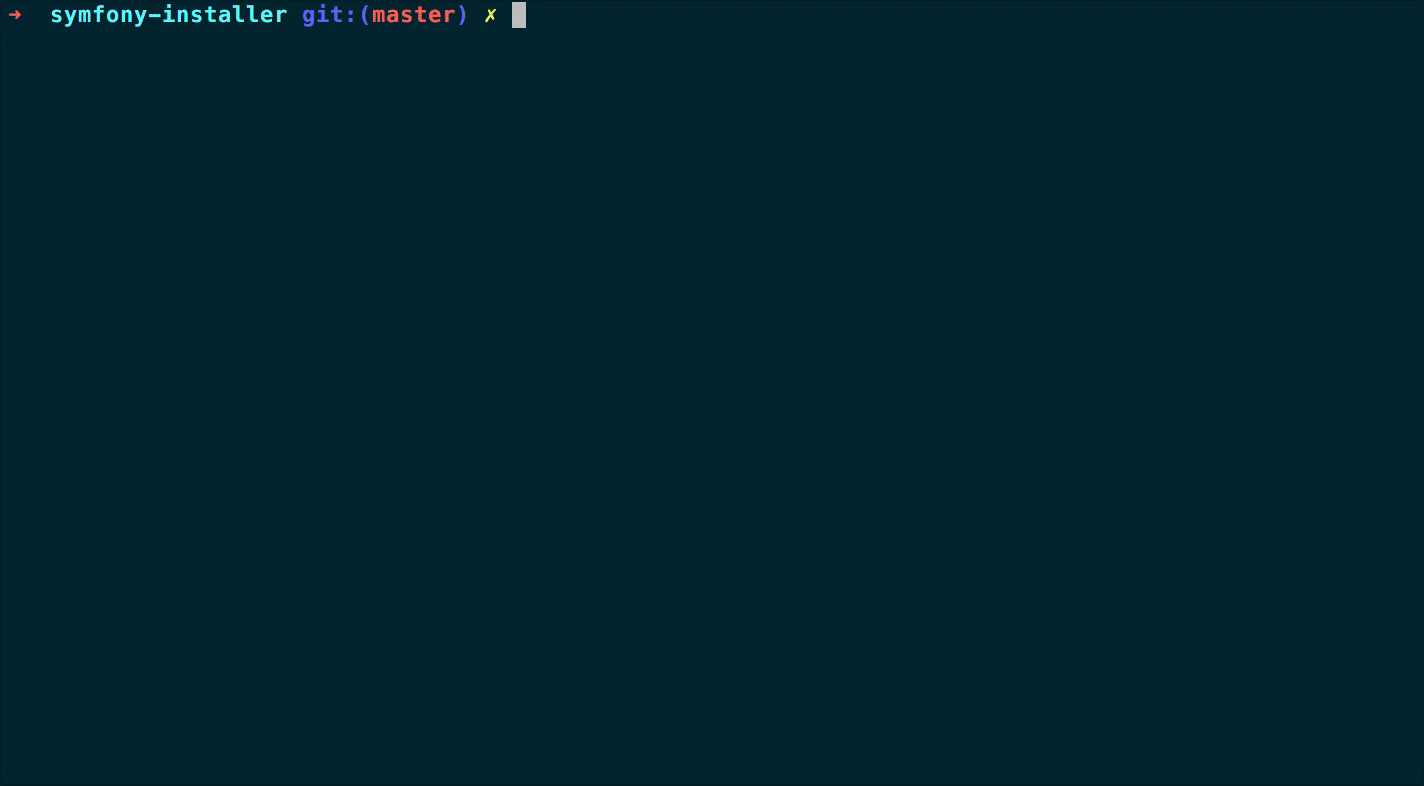 TODO: - [x] Add unit tests Commits ------- 9ec51a1 [Console] Modify console output and print multiple modifyable sections
- Loading branch information
Showing
9 changed files
with
531 additions
and
8 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
133 changes: 133 additions & 0 deletions
133
src/Symfony/Component/Console/Output/ConsoleSectionOutput.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,133 @@ | ||
<?php | ||
|
||
/* | ||
* This file is part of the Symfony package. | ||
* | ||
* (c) Fabien Potencier <fabien@symfony.com> | ||
* | ||
* For the full copyright and license information, please view the LICENSE | ||
* file that was distributed with this source code. | ||
*/ | ||
|
||
namespace Symfony\Component\Console\Output; | ||
|
||
use Symfony\Component\Console\Formatter\OutputFormatterInterface; | ||
use Symfony\Component\Console\Helper\Helper; | ||
use Symfony\Component\Console\Terminal; | ||
|
||
/** | ||
* @author Pierre du Plessis <pdples@gmail.com> | ||
* @author Gabriel Ostrolucký <gabriel.ostrolucky@gmail.com> | ||
*/ | ||
class ConsoleSectionOutput extends StreamOutput | ||
{ | ||
private $content = array(); | ||
private $lines = 0; | ||
private $sections; | ||
private $terminal; | ||
|
||
/** | ||
* @param resource $stream | ||
* @param ConsoleSectionOutput[] $sections | ||
*/ | ||
public function __construct($stream, array &$sections, int $verbosity, bool $decorated, OutputFormatterInterface $formatter) | ||
{ | ||
parent::__construct($stream, $verbosity, $decorated, $formatter); | ||
array_unshift($sections, $this); | ||
$this->sections = &$sections; | ||
$this->terminal = new Terminal(); | ||
} | ||
|
||
/** | ||
* Clears previous output for this section. | ||
* | ||
* @param int $lines Number of lines to clear. If null, then the entire output of this section is cleared | ||
*/ | ||
public function clear(int $lines = null) | ||
{ | ||
if (empty($this->content) || !$this->isDecorated()) { | ||
return; | ||
} | ||
|
||
if ($lines) { | ||
\array_splice($this->content, -($lines * 2)); // Multiply lines by 2 to cater for each new line added between content | ||
} else { | ||
$lines = $this->lines; | ||
$this->content = array(); | ||
} | ||
|
||
$this->lines -= $lines; | ||
|
||
parent::doWrite($this->popStreamContentUntilCurrentSection($lines), false); | ||
} | ||
|
||
/** | ||
* Overwrites the previous output with a new message. | ||
* | ||
* @param array|string $message | ||
*/ | ||
public function overwrite($message) | ||
{ | ||
$this->clear(); | ||
$this->writeln($message); | ||
} | ||
|
||
public function getContent(): string | ||
{ | ||
return implode('', $this->content); | ||
} | ||
|
||
/** | ||
* {@inheritdoc} | ||
*/ | ||
protected function doWrite($message, $newline) | ||
{ | ||
if (!$this->isDecorated()) { | ||
return parent::doWrite($message, $newline); | ||
} | ||
|
||
$erasedContent = $this->popStreamContentUntilCurrentSection(); | ||
|
||
foreach (explode(PHP_EOL, $message) as $lineContent) { | ||
$this->lines += ceil($this->getDisplayLength($lineContent) / $this->terminal->getWidth()) ?: 1; | ||
$this->content[] = $lineContent; | ||
$this->content[] = PHP_EOL; | ||
} | ||
|
||
parent::doWrite($message, true); | ||
parent::doWrite($erasedContent, false); | ||
} | ||
|
||
/** | ||
* At initial stage, cursor is at the end of stream output. This method makes cursor crawl upwards until it hits | ||
* current section. Then it erases content it crawled through. Optionally, it erases part of current section too. | ||
*/ | ||
private function popStreamContentUntilCurrentSection(int $numberOfLinesToClearFromCurrentSection = 0): string | ||
{ | ||
$numberOfLinesToClear = $numberOfLinesToClearFromCurrentSection; | ||
$erasedContent = array(); | ||
|
||
foreach ($this->sections as $section) { | ||
if ($section === $this) { | ||
break; | ||
} | ||
|
||
$numberOfLinesToClear += $section->lines; | ||
$erasedContent[] = $section->getContent(); | ||
} | ||
|
||
if ($numberOfLinesToClear > 0) { | ||
// move cursor up n lines | ||
parent::doWrite(sprintf("\x1b[%dA", $numberOfLinesToClear), false); | ||
// erase to end of screen | ||
parent::doWrite("\x1b[0J", false); | ||
} | ||
|
||
return implode('', array_reverse($erasedContent)); | ||
} | ||
|
||
private function getDisplayLength(string $text): string | ||
{ | ||
return Helper::strlenWithoutDecoration($this->getFormatter(), str_replace("\t", ' ', $text)); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.