01. Getting started
To get started, download the library to your computer: navigate to the library main page on GitHub, press the green Code button and Download ZIP. Extract the contents of the zip file somewhere in your computer. Now you can run UIAViewer.ahk or any of the example files.
If you run UIAViewer and click the "Start capturing" button, you should see something like this:
Here we have a Notepad window open, we see that the element under the cursor is highlighted with a blue box ("Edit" menu item), and there is some information displayed about it:
- In the Properties listbox we have most of the properties that the item has: for example the name of the button is "Edit", the type is "MenuItem" and so on.
- In the Pattern listbox we see different ways of interacting with the item: ExpandCollapse pattern contains ways of expanding (opening the Edit menu item menu) and collapsing (closing the menu), also getting information about its state like is it collapsed or not. LegacyIAccessible pattern contains information and methods from MSAA/Acc, which is the predecessor to UIA, and can also be used to get some info about it and interact with it.
- In the TreeView on the right we see part of the UIA tree: the Edit menu items sibling elements, its parents, and if it had any children then those as well. This gives us information about how to get to other elements relative to this one.
If we stop capturing and then press the "Construct tree for whole Window" button, the whole tree for the Notepad window is shown:
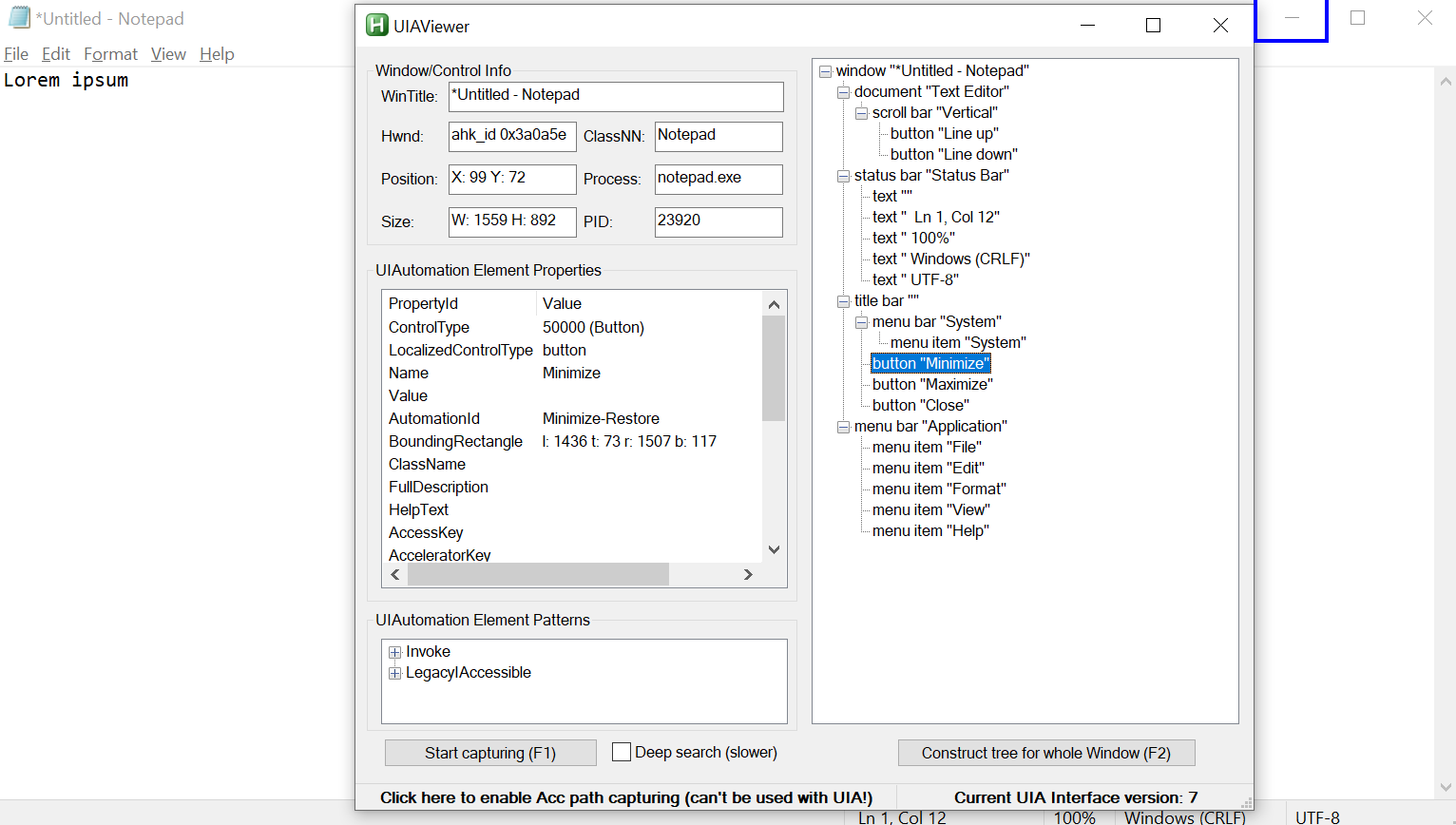
Now we have selected the "Minimize" button, and we see its parent is a title bar element, and its parent the Notepad window element. The root element "Desktop 1" is not shown in the tree, but it is indeed the parent of the window.
To see a simple example about interacting with the Notepad window, go to the Examples folder in the librarys main folder and open Example1_Notepad.ahk:
Run, notepad.exe
UIA := UIA_Interface() ; Initialize UIA interface
WinWaitActive, ahk_exe notepad.exe
npEl := UIA.ElementFromHandle(WinExist("ahk_exe notepad.exe")) ; Get the element for the Notepad window
documentEl := npEl.FindFirstByType("Document") ; Find the first Document control (in Notepad there is only one). This assumes the user is running a relatively recent Windows and UIA interface version 2+ is available. In UIA interface v1 this control was Edit, so an alternative option instead of "Document" would be "UIA.__Version > 1 ? "Document" : "Edit""
documentEl.SetValue("Lorem ipsum") ; Set the value for the document control.
; documentEl.CurrentValue := "Lorem ipsum"
; documentEl.Value := "Lorem ipsum"
Some irrelevant lines have been discarded here.
Lets go through this example:
-
#include ..\Lib\UIA_Interface.ahk
includes the main UIA library file. If you're not sure how #include works, take a look how to use #include here. Usually its easiest to put your script in the same folder where the Lib folder is located at, then you can just use#include <UIA_Interface>
to include it. -
UIA := UIA_Interface()
initiates the connection to the UIA interface and returns a new UIA_Interface object. This is the starting point which we can use to get starting point elements (eg the Notepad window), create conditions to filter elements and more. To learn more, take a look at the "UIA_Interface class" section of this Wiki. -
npEl := UIA.ElementFromHandle(WinExist("ahk_exe notepad.exe"))
gets us the starting point element, the Notepad window element (in UIAViewer this was the topmost element at the top of the tree), by using the ElementFromHandle method inside the UIA_Interface class.npEl
is a new UIA_Element object, which can be used to access all of this elements properties (Name, ControlType etc), get access to Patterns to interact with it, and more. To learn more about elements, take a look at the "UIA_Element class" section of this Wiki. -
documentEl := npEl.FindFirstByType("Document")
Here we look for the first element in the Notepad window tree that matches the type of "Document". In UIAViewer we see that there is adocument "Text Editor"
just below the window element, and that is the element we will get. Like for the Notepad window element, this is also a UIA_Element object. -
documentEl.SetValue("Lorem ipsum")
SetValue is a custom function in the UIA_Element class, that automatically gets the ValuePattern for our document element, and then uses the SetValue method of ValuePattern to change the value. This is the only pattern where we don't have to access the pattern ourselves, because it is such a common pattern. Alternatively we can usedocumentEl.Value := "Lorem ipsum"
which does the exact same thing, again by interacting with the ValuePattern for us.