-
Notifications
You must be signed in to change notification settings - Fork 135
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
feat!: add modals and text input #253
feat!: add modals and text input #253
Conversation
Since Discord doesn't dispatch this event, it wouldn't make sense to dispatch it, as it isn't accurate with the actual close event.
Good stuff! There're still things that should be slightly changed but I can't explain them in a review. I'll make a PR to your repo later. |
I didn't do this before because of a misunderstanding I had in `ui.action_row.py`.
This is only used when someone tries to respond a modal with another modal, which isn't allowed.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Neat, some pretty cool changes!
I've got just a few comments, some of which aren't about modals themselves but about WrappedComponent
and handling of actionrows, and a few things will probably require further discussion
this time as discussed
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Almost every collaborator worked on this. We can finally call it a complete implementation of modals and text inputs.
mistake :(
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
🎉
Summary
Bored of using
wait_for("message")
to get user input? I present you Modals, a new way to asking for user input, along withTextInput
, a component to write text (These can only be used in modals).Examples
Final result
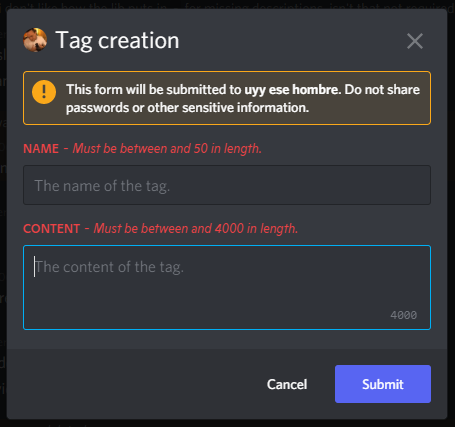
TODO list
disnake.ui.Modal
)disnake.ui.TextInput
anddisnake.components.TextInput
)ModalInteraction
(AndModalInteractionData
).InteractionRespone.send_modal
.ModalChaingNotSupported
errorAt the moment only
TextInput
is supported in modals, with a maximum of 5 action rows; 1 component per row.And to finish I want to thanks zhu for helping me to test this when we didn't have access to the modals beta.
Closes #212
Checklist
pre-commit run --all-files