-
Notifications
You must be signed in to change notification settings - Fork 76
Add support for inlining multiple text and passing an array via the lineHeight prop. Features for #893, #902, and #904. #328
Add support for inlining multiple text and passing an array via the lineHeight prop. Features for #893, #902, and #904. #328
Conversation
@parkerziegler this looks good to me. Can you add your example to the demo page? |
@boygirl Added the examples to the bottom of |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Nice! 👏
@boygirl @david-davidson Just updated the main comment for the PR. Should fix #893 and #902 together. Let me know if there are any issues. Both features have examples in the demo app. |
src/victory-label/victory-label.js
Outdated
@@ -264,10 +267,14 @@ export default class VictoryLabel extends React.Component { | |||
const style = this.style[i] || this.style[0]; | |||
const lastStyle = this.style[i - 1] || this.style[0]; | |||
const fontSize = (style.fontSize + lastStyle.fontSize) / 2; | |||
const lineHeight = Array.isArray(this.lineHeight) |
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
Do we need to detect/handle empty arrays? (Same question for existing style
var too)
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
I suppose for completeness sake we should, but in both of those cases, that would only happen if a user explicitly added the prop like lineHeight={[]}
. That would technically be allowed by the prop definition, but doesn't make any intuitive sense. I think it's a highly unlikely edge case to hit, but I agree that it's bad practice to trust the user to not break things.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@parkerziegler can you add a fallback for empty arrays for lineHeight
in this PR. I'll create a new issue for styles
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@boygirl @ryan-roemer Sure thing, I'll do it first thing when I get in tomorrow.
There was a problem hiding this comment.
Choose a reason for hiding this comment
The reason will be displayed to describe this comment to others. Learn more.
@ryan-roemer I think I'm pretty much all set to push the changes. We fallback to default values for both style
and lineHeight
if empty arrays are passed for those props. Should I add a console.warn
just to let users know this is not a recommended practice?
@parkerziegler code looks good, but please lint the changes you added to the demo |
@boygirl This should be updated. I added 4 test cases to cover the issues in |
This PR accomplishes three tasks, related to issues #893, #902, and #904.
Issue #893: Allow VictoryLabel to render multiple text inline
The first portion of this PR adds support for a
boolean inline
prop, which specifies that labels passed as an array inVictoryLabel
'stext
prop
should be rendered inline. To do this, we simply return undefined for thex
anddy
attributes ifinline
istrue
. This allows thedx
prop
to specify the relative spacing of inlined<tspan />
elements. For example, this code:will render:
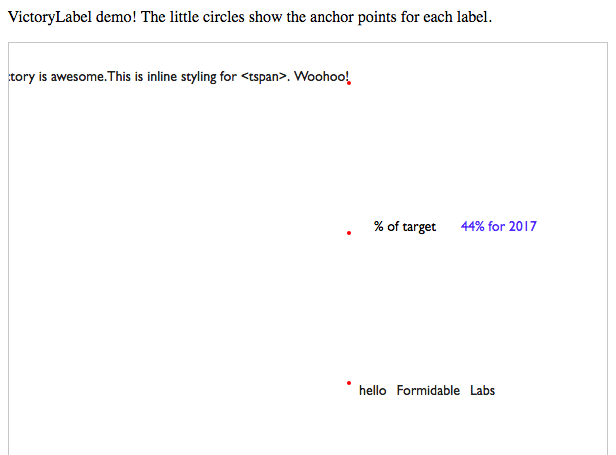
We could also consider doing some more explicit styling for inlined labels – this solution just takes advantage of
svg
's default inline display for<tspan />
s inside of<text />
.Issue #902: For a multiline VictoryLabel, I would like to have different lineHeights per line.
The second portion of this PR adds support for passing a
number[]
orstring[]
as a validlineHeight prop
. To do this, we check iflineHeight
is passed as an array and, if so, average thelineHeight
of the current and previous index (similar to how we averagefontSize
) in order to calculate the properdy
attribute for each<tspan />
. For example, the following code:will render:
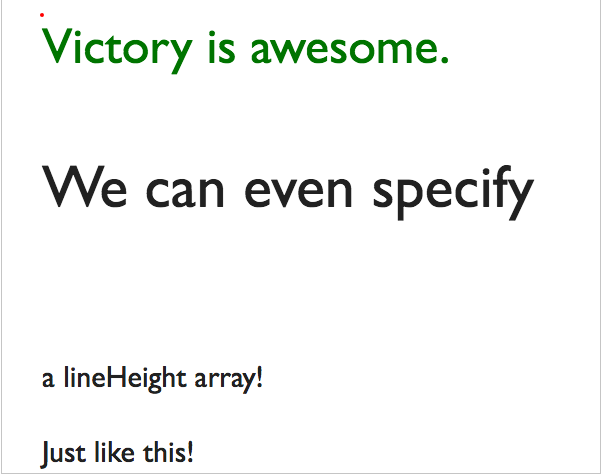
We may want to revisit how this interacts with
verticalTextAnchor
– we use the firstlineHeight
, similar to how we use the firstfontSize
, to determine thedy
attribute of the<text />
container itself.Issue #904 Empty arrays for VictoryLabel's style prop cause an error
There are now checks for a user passing empty arrays into both the
style
andlineHeight
props. If an empty array gets passed intostyle
, we default todefaultStyles
. If an empty array gets passed intolineHeight
, we default to using 1 as thelineHeight
.