jdbc
GProM also features a JDBC driver that uses libgprom through JNA to rewrite queries. This JDBC driver acts as a wrapper for a backend database's JDBC driver.
If GProM is compiled with Java bindings (--disable-java
is not passed to configure
), then make
will build a JDBC driver jar in build/gprom-jdbc.jar
. This jar file contains a native version of libgprom that was build as part of the standard gprom build process. The additional dependencies (jar files) need to use this driver are automatically downloaded to build/buildlib
as part of the build process.
If you prefer to use a fat jar (one jar file containing the JDBC driver and all dependencies) then run:
ant jar-fat
This will build a file build/gprom-jdbc-all.jar
using GProM's docker based cross-compilation build system to build versions of gprom-jdbc.jar
and gprom-jdbc-all.jar
that contains native versions of libgprom for several operating systems:
- Windows (32 and 64 bit versions)
- Linux (32 and 64 bit versions)
- Mac OS X (64 bit version)
Alternatively, you can use the shell script travis/build.sh
to build gprom including the fat jar using docker for all steps fo the build process. This only requires docker to be available on your system.
Examples for how to use the driver to connect to SQLite and Postgres can be found in the javatest/org/gprom/jdbc/test/
folder (GProMJDBCTest.java
and SQLiteJDBCTest.java
).
To use the driver to connect to a backend database, you need to load both GProM's driver as well as backend database's driver. For instance, if you are using Postgres then you need to load the Postgres driver (org.postgresql.Driver
):
Class.forName("org.gprom.jdbc.driver.GProMDriver");
Class.forName("org.postgresql.Driver");
After loading a driver, a JDBC connection is created by prefixing the JDBC URL for the backend database with gprom
. For instance, to connect to a local postgres database (127.0.0.1
) called test
running on port 5432
use:
String url = "jdbc:gprom:postgresql://localhost:5432/test"
Properties info = new Properties();
info.setProperty("user", "postgres");
info.setProperty("password", "mypassword");
Connection con = (GProMConnection) DriverManager.getConnection(url,info);
instead of
String url = "jdbc:postgresql://localhost:5432/test"
Properties info = new Properties();
info.setProperty("user", "postgres");
info.setProperty("password", "mypassword");
Connection con = (GProMConnection) DriverManager.getConnection(url,info);
Note that GProM's driver will forward properties set when opening a connection to the backend driver except for options prefixed with gpromOption.
. These operations are passed to GProM.
There are two ways of how to set options. Either by setting connection properties with gpromOption.option
when creating a connection. Alternatively, by retrieving the libgprom's Java wrapper from the connection and using it to set options. For example to activate GProM's heuristic relational algebra optimization:
...
Properties info = new Properties();
info.setProperty("user", "postgres");
info.setProperty("password", "mypassword");
info.setProperty("gpromOptions.optimize_operator_model", "true");
Connection con = (GProMConnection) DriverManager.getConnection(url,info);
...
Properties info = new Properties();
info.setProperty("user", "postgres");
info.setProperty("password", "mypassword");
Connection con = (GProMConnection) DriverManager.getConnection(url,info);
con.getW().setBoolOption("optimize_operator_model", true);
GProM's JDBC driver uses log4j version 2 for logging. Log output of libgprom is also forwarded to log4j. The verbosity of GProM logging can be controlled by setting the log.level
option:
0 = no logging
1 = error
2 = info
3 = debug
4 = trace
All GProM log output is routed to a logger named LIBGPROM
.
To see a list of options supported by GProM with descriptions you can call optionsHelp
from the GProM wrapper. Assume that you have opened connection con
:
System.out.println(con.getW().optionsHelp());
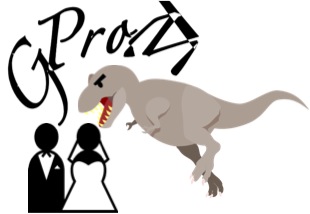