Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
0 parents
commit a908f05
Showing
149 changed files
with
227,238 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,51 @@ | ||
APP_NAME=Laravel | ||
APP_ENV=local | ||
APP_KEY= | ||
APP_DEBUG=true | ||
APP_URL=http://localhost | ||
|
||
LOG_CHANNEL=stack | ||
LOG_LEVEL=debug | ||
|
||
DB_CONNECTION=mysql | ||
DB_HOST=127.0.0.1 | ||
DB_PORT=3306 | ||
DB_DATABASE=starters | ||
DB_USERNAME=root | ||
DB_PASSWORD= | ||
|
||
BROADCAST_DRIVER=log | ||
CACHE_DRIVER=file | ||
FILESYSTEM_DRIVER=local | ||
QUEUE_CONNECTION=sync | ||
SESSION_DRIVER=file | ||
SESSION_LIFETIME=120 | ||
|
||
MEMCACHED_HOST=127.0.0.1 | ||
|
||
REDIS_HOST=127.0.0.1 | ||
REDIS_PASSWORD=null | ||
REDIS_PORT=6379 | ||
|
||
MAIL_MAILER=smtp | ||
MAIL_HOST=mailhog | ||
MAIL_PORT=1025 | ||
MAIL_USERNAME=null | ||
MAIL_PASSWORD=null | ||
MAIL_ENCRYPTION=null | ||
MAIL_FROM_ADDRESS=null | ||
MAIL_FROM_NAME="${APP_NAME}" | ||
|
||
AWS_ACCESS_KEY_ID= | ||
AWS_SECRET_ACCESS_KEY= | ||
AWS_DEFAULT_REGION=us-east-1 | ||
AWS_BUCKET= | ||
AWS_USE_PATH_STYLE_ENDPOINT=false | ||
|
||
PUSHER_APP_ID= | ||
PUSHER_APP_KEY= | ||
PUSHER_APP_SECRET= | ||
PUSHER_APP_CLUSTER=mt1 | ||
|
||
VITE_PUSHER_APP_KEY="${PUSHER_APP_KEY}" | ||
VITE_PUSHER_APP_CLUSTER="${PUSHER_APP_CLUSTER}" |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,5 @@ | ||
* text=auto | ||
*.css linguist-vendored | ||
*.scss linguist-vendored | ||
*.js linguist-vendored | ||
CHANGELOG.md export-ignore |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
/node_modules | ||
/public/hot | ||
/public/build | ||
/public/storage | ||
/storage/*.key | ||
/vendor | ||
.env | ||
.env.backup | ||
.phpunit.result.cache | ||
docker-compose.override.yml | ||
Homestead.json | ||
Homestead.yaml | ||
npm-debug.log | ||
yarn-error.log | ||
/.idea | ||
/.vscode |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,14 @@ | ||
php: | ||
preset: laravel | ||
version: 8 | ||
disabled: | ||
- no_unused_imports | ||
finder: | ||
not-name: | ||
- index.php | ||
- server.php | ||
js: | ||
finder: | ||
not-name: | ||
- webpack.mix.js | ||
css: true |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
## Laravel Breeze: Tailwind Pages Skeleton | ||
|
||
Laravel boilerplate repository to create simple demo-projects. It allows to quickly add new routes/pages, and has examples of a table page, and a form page. | ||
|
||
It uses the Starter Kit [Laravel Breeze](https://github.com/laravel/breeze) based on Tailwind framework. | ||
|
||
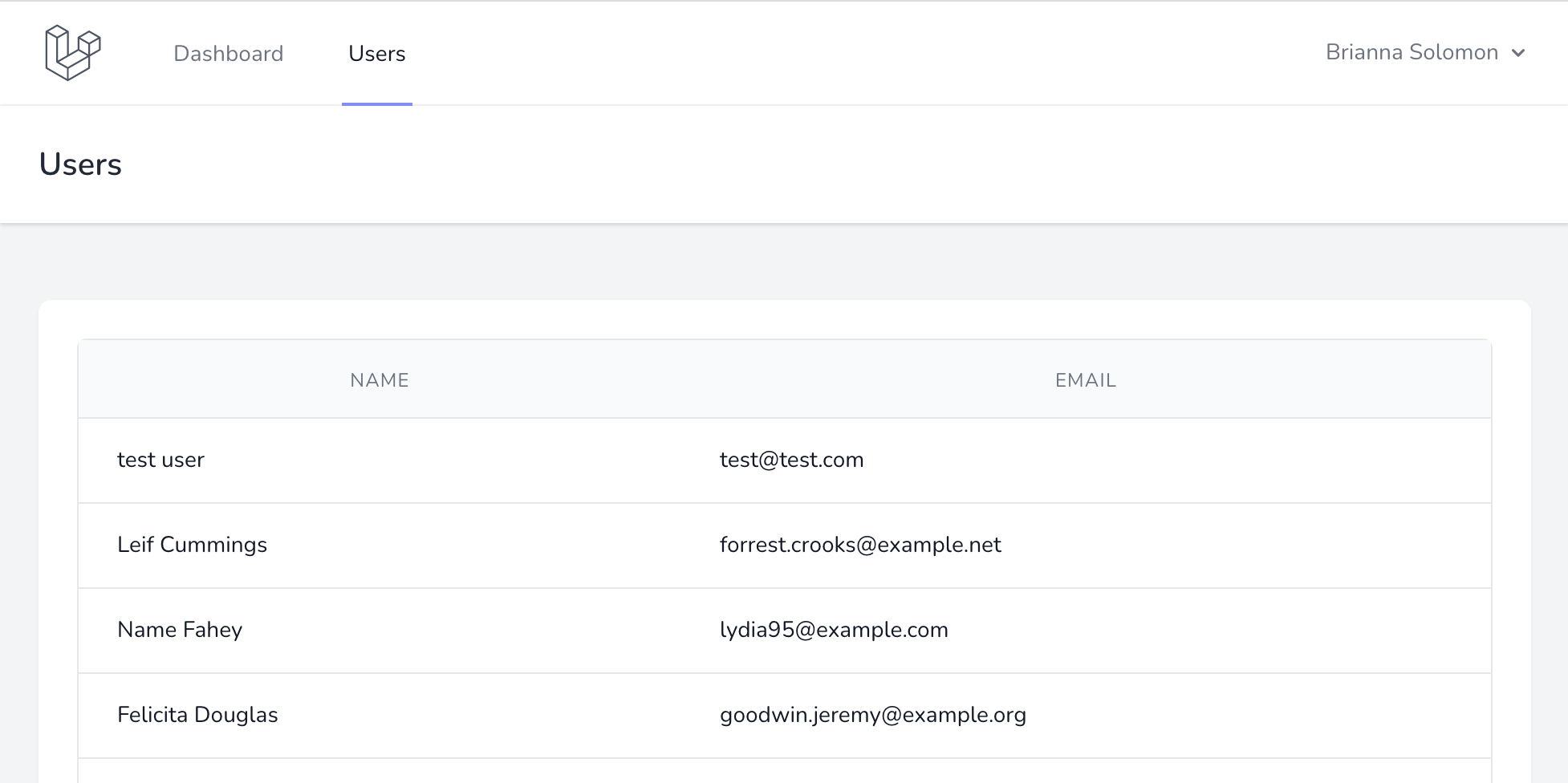 | ||
|
||
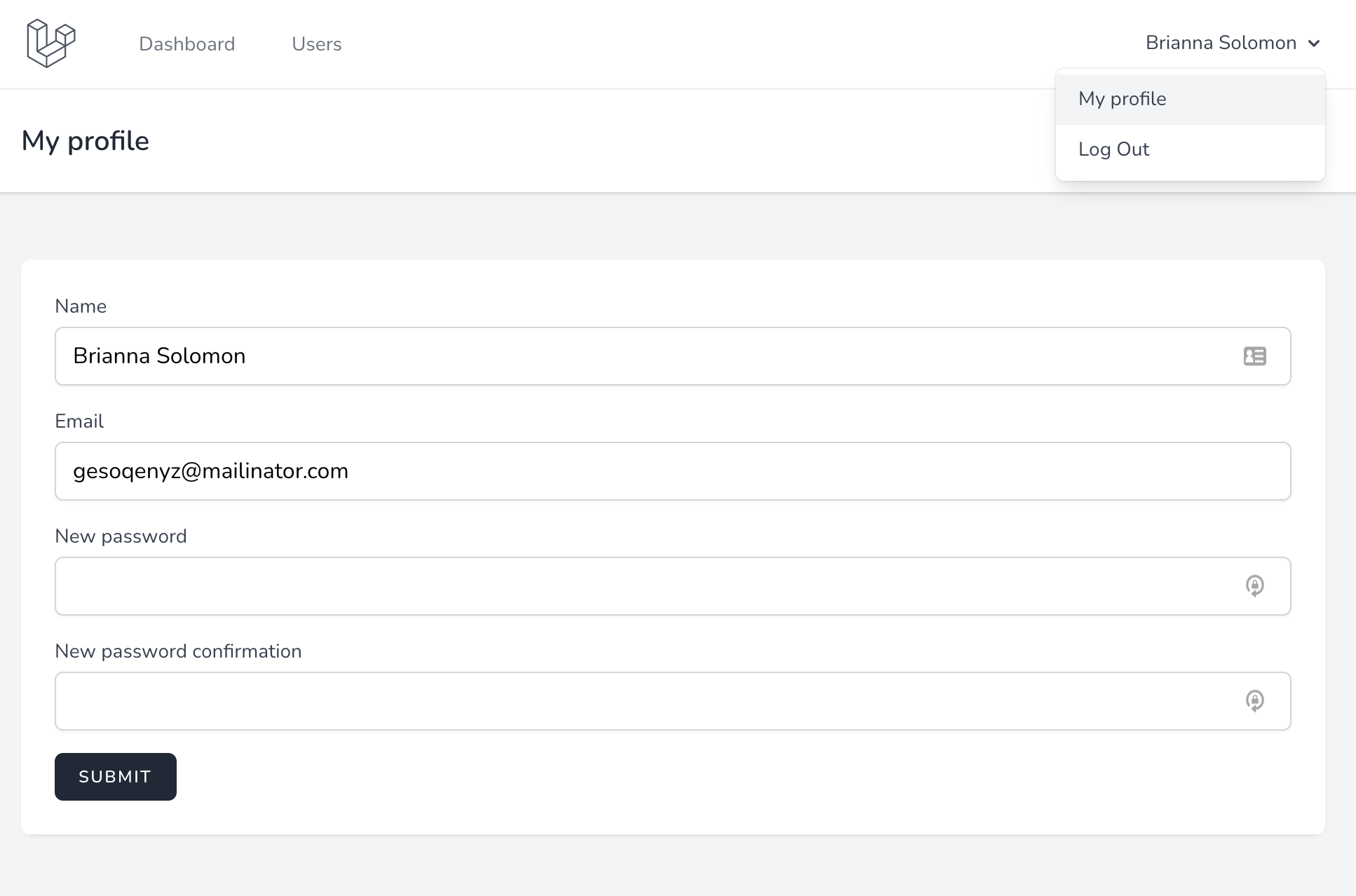 | ||
|
||
----- | ||
|
||
### How to use | ||
|
||
- Clone the project with `git clone` | ||
- Copy `.env.example` file to `.env` and edit database credentials there | ||
- Run `composer install` | ||
- Run `php artisan key:generate` | ||
- Run `php artisan migrate --seed` (it has some seeded data for your testing) | ||
- That's it: launch the main URL | ||
|
||
|
||
--- | ||
|
||
## More from our LaravelDaily Team | ||
|
||
- Enroll in our [Laravel Daily Courses](https://laraveldaily.com/) | ||
- Check out our adminpanel generator [QuickAdminPanel](https://quickadminpanel.com) | ||
- Purchase our [Livewire Kit](https://livewirekit.com) | ||
- Subscribe to our [YouTube channel Laravel Daily](https://www.youtube.com/channel/UCTuplgOBi6tJIlesIboymGA) |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,27 @@ | ||
<?php | ||
|
||
namespace App\Console; | ||
|
||
use Illuminate\Console\Scheduling\Schedule; | ||
use Illuminate\Foundation\Console\Kernel as ConsoleKernel; | ||
|
||
class Kernel extends ConsoleKernel | ||
{ | ||
/** | ||
* Define the application's command schedule. | ||
*/ | ||
protected function schedule(Schedule $schedule): void | ||
{ | ||
// $schedule->command('inspire')->hourly(); | ||
} | ||
|
||
/** | ||
* Register the commands for the application. | ||
*/ | ||
protected function commands(): void | ||
{ | ||
$this->load(__DIR__.'/Commands'); | ||
|
||
require base_path('routes/console.php'); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
<?php | ||
|
||
namespace App\Exceptions; | ||
|
||
use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler; | ||
use Throwable; | ||
|
||
class Handler extends ExceptionHandler | ||
{ | ||
/** | ||
* A list of exception types with their corresponding custom log levels. | ||
* | ||
* @var array<class-string<\Throwable>, \Psr\Log\LogLevel::*> | ||
*/ | ||
protected $levels = [ | ||
// | ||
]; | ||
|
||
/** | ||
* A list of the exception types that are not reported. | ||
* | ||
* @var array<int, class-string<\Throwable>> | ||
*/ | ||
protected $dontReport = [ | ||
// | ||
]; | ||
|
||
/** | ||
* A list of the inputs that are never flashed to the session on validation exceptions. | ||
* | ||
* @var array<int, string> | ||
*/ | ||
protected $dontFlash = [ | ||
'current_password', | ||
'password', | ||
'password_confirmation', | ||
]; | ||
|
||
/** | ||
* Register the exception handling callbacks for the application. | ||
*/ | ||
public function register(): void | ||
{ | ||
$this->reportable(function (Throwable $e) { | ||
// | ||
}); | ||
} | ||
} |
48 changes: 48 additions & 0 deletions
48
app/Http/Controllers/Auth/AuthenticatedSessionController.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
<?php | ||
|
||
namespace App\Http\Controllers\Auth; | ||
|
||
use App\Http\Controllers\Controller; | ||
use App\Http\Requests\Auth\LoginRequest; | ||
use App\Providers\RouteServiceProvider; | ||
use Illuminate\Http\RedirectResponse; | ||
use Illuminate\Http\Request; | ||
use Illuminate\Support\Facades\Auth; | ||
use Illuminate\View\View; | ||
|
||
class AuthenticatedSessionController extends Controller | ||
{ | ||
/** | ||
* Display the login view. | ||
*/ | ||
public function create(): View | ||
{ | ||
return view('auth.login'); | ||
} | ||
|
||
/** | ||
* Handle an incoming authentication request. | ||
*/ | ||
public function store(LoginRequest $request): RedirectResponse | ||
{ | ||
$request->authenticate(); | ||
|
||
$request->session()->regenerate(); | ||
|
||
return redirect()->intended(RouteServiceProvider::HOME); | ||
} | ||
|
||
/** | ||
* Destroy an authenticated session. | ||
*/ | ||
public function destroy(Request $request): RedirectResponse | ||
{ | ||
Auth::guard('web')->logout(); | ||
|
||
$request->session()->invalidate(); | ||
|
||
$request->session()->regenerateToken(); | ||
|
||
return redirect('/'); | ||
} | ||
} |
41 changes: 41 additions & 0 deletions
41
app/Http/Controllers/Auth/ConfirmablePasswordController.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
<?php | ||
|
||
namespace App\Http\Controllers\Auth; | ||
|
||
use App\Http\Controllers\Controller; | ||
use App\Providers\RouteServiceProvider; | ||
use Illuminate\Http\RedirectResponse; | ||
use Illuminate\Http\Request; | ||
use Illuminate\Support\Facades\Auth; | ||
use Illuminate\Validation\ValidationException; | ||
use Illuminate\View\View; | ||
|
||
class ConfirmablePasswordController extends Controller | ||
{ | ||
/** | ||
* Show the confirm password view. | ||
*/ | ||
public function show(): View | ||
{ | ||
return view('auth.confirm-password'); | ||
} | ||
|
||
/** | ||
* Confirm the user's password. | ||
*/ | ||
public function store(Request $request): RedirectResponse | ||
{ | ||
if (! Auth::guard('web')->validate([ | ||
'email' => $request->user()->email, | ||
'password' => $request->password, | ||
])) { | ||
throw ValidationException::withMessages([ | ||
'password' => __('auth.password'), | ||
]); | ||
} | ||
|
||
$request->session()->put('auth.password_confirmed_at', time()); | ||
|
||
return redirect()->intended(RouteServiceProvider::HOME); | ||
} | ||
} |
25 changes: 25 additions & 0 deletions
25
app/Http/Controllers/Auth/EmailVerificationNotificationController.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,25 @@ | ||
<?php | ||
|
||
namespace App\Http\Controllers\Auth; | ||
|
||
use App\Http\Controllers\Controller; | ||
use App\Providers\RouteServiceProvider; | ||
use Illuminate\Http\RedirectResponse; | ||
use Illuminate\Http\Request; | ||
|
||
class EmailVerificationNotificationController extends Controller | ||
{ | ||
/** | ||
* Send a new email verification notification. | ||
*/ | ||
public function store(Request $request): RedirectResponse | ||
{ | ||
if ($request->user()->hasVerifiedEmail()) { | ||
return redirect()->intended(RouteServiceProvider::HOME); | ||
} | ||
|
||
$request->user()->sendEmailVerificationNotification(); | ||
|
||
return back()->with('status', 'verification-link-sent'); | ||
} | ||
} |
22 changes: 22 additions & 0 deletions
22
app/Http/Controllers/Auth/EmailVerificationPromptController.php
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
<?php | ||
|
||
namespace App\Http\Controllers\Auth; | ||
|
||
use App\Http\Controllers\Controller; | ||
use App\Providers\RouteServiceProvider; | ||
use Illuminate\Http\RedirectResponse; | ||
use Illuminate\Http\Request; | ||
use Illuminate\View\View; | ||
|
||
class EmailVerificationPromptController extends Controller | ||
{ | ||
/** | ||
* Display the email verification prompt. | ||
*/ | ||
public function __invoke(Request $request): RedirectResponse|View | ||
{ | ||
return $request->user()->hasVerifiedEmail() | ||
? redirect()->intended(RouteServiceProvider::HOME) | ||
: view('auth.verify-email'); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,61 @@ | ||
<?php | ||
|
||
namespace App\Http\Controllers\Auth; | ||
|
||
use App\Http\Controllers\Controller; | ||
use Illuminate\Auth\Events\PasswordReset; | ||
use Illuminate\Http\RedirectResponse; | ||
use Illuminate\Http\Request; | ||
use Illuminate\Support\Facades\Hash; | ||
use Illuminate\Support\Facades\Password; | ||
use Illuminate\Support\Str; | ||
use Illuminate\Validation\Rules; | ||
use Illuminate\View\View; | ||
|
||
class NewPasswordController extends Controller | ||
{ | ||
/** | ||
* Display the password reset view. | ||
*/ | ||
public function create(Request $request): View | ||
{ | ||
return view('auth.reset-password', ['request' => $request]); | ||
} | ||
|
||
/** | ||
* Handle an incoming new password request. | ||
* | ||
* @throws \Illuminate\Validation\ValidationException | ||
*/ | ||
public function store(Request $request): RedirectResponse | ||
{ | ||
$request->validate([ | ||
'token' => ['required'], | ||
'email' => ['required', 'email'], | ||
'password' => ['required', 'confirmed', Rules\Password::defaults()], | ||
]); | ||
|
||
// Here we will attempt to reset the user's password. If it is successful we | ||
// will update the password on an actual user model and persist it to the | ||
// database. Otherwise we will parse the error and return the response. | ||
$status = Password::reset( | ||
$request->only('email', 'password', 'password_confirmation', 'token'), | ||
function ($user) use ($request) { | ||
$user->forceFill([ | ||
'password' => Hash::make($request->password), | ||
'remember_token' => Str::random(60), | ||
])->save(); | ||
|
||
event(new PasswordReset($user)); | ||
} | ||
); | ||
|
||
// If the password was successfully reset, we will redirect the user back to | ||
// the application's home authenticated view. If there is an error we can | ||
// redirect them back to where they came from with their error message. | ||
return $status == Password::PASSWORD_RESET | ||
? redirect()->route('login')->with('status', __($status)) | ||
: back()->withInput($request->only('email')) | ||
->withErrors(['email' => __($status)]); | ||
} | ||
} |
Oops, something went wrong.