-
Notifications
You must be signed in to change notification settings - Fork 3
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
36 changed files
with
1,001 additions
and
6 deletions.
There are no files selected for viewing
2 changes: 1 addition & 1 deletion
2
LeetCodeNet.Tests/G0101_0200/S0102_binary_tree_level_order_traversal/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
2 changes: 1 addition & 1 deletion
2
LeetCodeNet.Tests/G0101_0200/S0104_maximum_depth_of_binary_tree/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
2 changes: 1 addition & 1 deletion
2
LeetCodeNet.Tests/G0101_0200/S0124_binary_tree_maximum_path_sum/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
29 changes: 29 additions & 0 deletions
29
LeetCodeNet.Tests/G0201_0300/S0206_reverse_linked_list/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
namespace LeetCodeNet.G0201_0300.S0206_reverse_linked_list { | ||
|
||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void ReverseList() { | ||
ListNode headActual = new ListNode(1); | ||
headActual.next = new ListNode(2); | ||
headActual.next.next = new ListNode(3); | ||
headActual.next.next.next = new ListNode(4); | ||
headActual.next.next.next.next = new ListNode(5); | ||
Assert.Equal("5, 4, 3, 2, 1", new Solution().ReverseList(headActual).ToString()); | ||
} | ||
|
||
[Fact] | ||
public void ReverseList2() { | ||
ListNode headActual = new ListNode(1); | ||
headActual.next = new ListNode(2); | ||
Assert.Equal("2, 1", new Solution().ReverseList(headActual).ToString()); | ||
} | ||
|
||
[Fact] | ||
public void ReverseList3() { | ||
Assert.Null(new Solution().ReverseList(null)); | ||
} | ||
} | ||
} |
16 changes: 16 additions & 0 deletions
16
LeetCodeNet.Tests/G0201_0300/S0207_course_schedule/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
namespace LeetCodeNet.G0201_0300.S0207_course_schedule { | ||
|
||
using Xunit; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void CanFinish() { | ||
Assert.True(new Solution().CanFinish(2, new int[][] { new int[] { 1, 0 } })); | ||
} | ||
|
||
[Fact] | ||
public void CanFinish2() { | ||
Assert.False(new Solution().CanFinish(2, new int[][] { new int[] { 1, 0 }, new int[] { 0, 1 } })); | ||
} | ||
} | ||
} |
21 changes: 21 additions & 0 deletions
21
LeetCodeNet.Tests/G0201_0300/S0208_implement_trie_prefix_tree/TrieTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
namespace LeetCodeNet.G0201_0300.S0208_implement_trie_prefix_tree { | ||
|
||
using Xunit; | ||
|
||
public class TrieTest { | ||
[Fact] | ||
public void Trie() { | ||
Trie trie = new Trie(); | ||
trie.Insert("apple"); | ||
// return True | ||
Assert.True(trie.Search("apple")); | ||
// return False | ||
Assert.False(trie.Search("app")); | ||
// return True | ||
Assert.True(trie.StartsWith("app")); | ||
trie.Insert("app"); | ||
// return True | ||
Assert.True(trie.Search("app")); | ||
} | ||
} | ||
} |
16 changes: 16 additions & 0 deletions
16
LeetCodeNet.Tests/G0201_0300/S0215_kth_largest_element_in_an_array/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
namespace LeetCodeNet.G0201_0300.S0215_kth_largest_element_in_an_array { | ||
|
||
using Xunit; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void FindKthLargest() { | ||
Assert.Equal(5, new Solution().FindKthLargest(new int[] { 3, 2, 1, 5, 6, 4 }, 2)); | ||
} | ||
|
||
[Fact] | ||
public void FindKthLargest2() { | ||
Assert.Equal(4, new Solution().FindKthLargest(new int[] { 3, 2, 3, 1, 2, 4, 5, 5, 6 }, 4)); | ||
} | ||
} | ||
} |
29 changes: 29 additions & 0 deletions
29
LeetCodeNet.Tests/G0201_0300/S0221_maximal_square/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,29 @@ | ||
namespace LeetCodeNet.G0201_0300.S0221_maximal_square { | ||
|
||
using Xunit; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void MaximalSquare() { | ||
char[][] input = { | ||
new[] {'1', '0', '1', '0', '0'}, | ||
new[] {'1', '0', '1', '1', '1'}, | ||
new[] {'1', '1', '1', '1', '1'}, | ||
new[] {'1', '0', '0', '1', '0'} | ||
}; | ||
Assert.Equal(4, new Solution().MaximalSquare(input)); | ||
} | ||
|
||
[Fact] | ||
public void MaximalSquare2() { | ||
char[][] input = { new[] {'0', '1'}, new[] {'1', '0'} }; | ||
Assert.Equal(1, new Solution().MaximalSquare(input)); | ||
} | ||
|
||
[Fact] | ||
public void MaximalSquare3() { | ||
char[][] input = { new[] {'0'} }; | ||
Assert.Equal(0, new Solution().MaximalSquare(input)); | ||
} | ||
} | ||
} |
19 changes: 19 additions & 0 deletions
19
LeetCodeNet.Tests/G0201_0300/S0226_invert_binary_tree/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,19 @@ | ||
namespace LeetCodeNet.G0201_0300.S0226_invert_binary_tree { | ||
|
||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void InvertTree() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 4, 2, 7, 1, 3, 6, 9 }); | ||
Assert.Equal("4,7,9,6,2,3,1", new Solution().InvertTree(root).ToString()); | ||
} | ||
|
||
[Fact] | ||
public void InvertTree2() { | ||
TreeNode root = TreeUtils.ConstructBinaryTree(new List<int?> { 2, 1, 3 }); | ||
Assert.Equal("2,3,1", new Solution().InvertTree(root).ToString()); | ||
} | ||
} | ||
} |
19 changes: 19 additions & 0 deletions
19
LeetCodeNet.Tests/G0201_0300/S0230_kth_smallest_element_in_a_bst/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,19 @@ | ||
namespace LeetCodeNet.G0201_0300.S0230_kth_smallest_element_in_a_bst { | ||
|
||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void KthSmallest() { | ||
TreeNode root = TreeNode.Create(new List<int?> { 3, 1, 4, null, 2 }); | ||
Assert.Equal(1, new Solution().KthSmallest(root, 1)); | ||
} | ||
|
||
[Fact] | ||
public void KthSmallest2() { | ||
TreeNode root = TreeNode.Create(new List<int?> { 5, 3, 6, 2, 4, null, null, 1 }); | ||
Assert.Equal(3, new Solution().KthSmallest(root, 3)); | ||
} | ||
} | ||
} |
23 changes: 23 additions & 0 deletions
23
LeetCodeNet.Tests/G0201_0300/S0234_palindrome_linked_list/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,23 @@ | ||
namespace LeetCodeNet.G0201_0300.S0234_palindrome_linked_list { | ||
|
||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void IsPalindrome() { | ||
ListNode headActual = new ListNode(1); | ||
headActual.next = new ListNode(2); | ||
headActual.next.next = new ListNode(2); | ||
headActual.next.next.next = new ListNode(1); | ||
Assert.True(new Solution().IsPalindrome(headActual)); | ||
} | ||
|
||
[Fact] | ||
public void IsPalindrome2() { | ||
ListNode headActual = new ListNode(1); | ||
headActual.next = new ListNode(2); | ||
Assert.False(new Solution().IsPalindrome(headActual)); | ||
} | ||
} | ||
} |
36 changes: 36 additions & 0 deletions
36
LeetCodeNet.Tests/G0201_0300/S0236_lowest_common_ancestor_of_a_binary_tree/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
namespace LeetCodeNet.G0201_0300.S0236_lowest_common_ancestor_of_a_binary_tree { | ||
|
||
using Xunit; | ||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void LowestCommonAncestor() { | ||
TreeNode leftNodeLeftNode = new TreeNode(6); | ||
TreeNode leftNodeRightNode = new TreeNode(2, new TreeNode(7), new TreeNode(4)); | ||
TreeNode leftNode = new TreeNode(5, leftNodeLeftNode, leftNodeRightNode); | ||
TreeNode rightNode = new TreeNode(1, new TreeNode(0), new TreeNode(8)); | ||
TreeNode root = new TreeNode(3, leftNode, rightNode); | ||
Assert.Equal(3, new Solution().LowestCommonAncestor(root, new TreeNode(5), new TreeNode(1)).val); | ||
} | ||
|
||
[Fact] | ||
public void LowestCommonAncestor2() { | ||
TreeNode leftNodeLeftNode = new TreeNode(6); | ||
TreeNode leftNodeRightNode = new TreeNode(2, new TreeNode(7), new TreeNode(4)); | ||
TreeNode leftNode = new TreeNode(5, leftNodeLeftNode, leftNodeRightNode); | ||
TreeNode rightNode = new TreeNode(1, new TreeNode(0), new TreeNode(8)); | ||
TreeNode root = new TreeNode(3, leftNode, rightNode); | ||
Assert.Equal(5, new Solution().LowestCommonAncestor(root, new TreeNode(5), new TreeNode(4)).val); | ||
} | ||
|
||
[Fact] | ||
public void LowestCommonAncestor3() { | ||
Assert.Equal(2, | ||
new Solution().LowestCommonAncestor( | ||
new TreeNode(2, new TreeNode(1), null), | ||
new TreeNode(2), | ||
new TreeNode(1)).val); | ||
} | ||
} | ||
} |
22 changes: 22 additions & 0 deletions
22
LeetCodeNet.Tests/G0201_0300/S0238_product_of_array_except_self/SolutionTest.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,22 @@ | ||
namespace LeetCodeNet.G0201_0300.S0238_product_of_array_except_self { | ||
|
||
using Xunit; | ||
|
||
public class SolutionTest { | ||
[Fact] | ||
public void ProductExceptSelf() { | ||
Assert.Equal( | ||
new int[] { 24, 12, 8, 6 }, | ||
new Solution().ProductExceptSelf(new int[] { 1, 2, 3, 4 }) | ||
); | ||
} | ||
|
||
[Fact] | ||
public void ProductExceptSelf2() { | ||
Assert.Equal( | ||
new int[] { 0, 0, 9, 0, 0 }, | ||
new Solution().ProductExceptSelf(new int[] { -1, 1, 0, -3, 3 }) | ||
); | ||
} | ||
} | ||
} |
34 changes: 34 additions & 0 deletions
34
LeetCodeNet/G0201_0300/S0206_reverse_linked_list/Solution.cs
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
namespace LeetCodeNet.G0201_0300.S0206_reverse_linked_list { | ||
|
||
// #Easy #Top_100_Liked_Questions #Top_Interview_Questions #Linked_List #Recursion | ||
// #Data_Structure_I_Day_8_Linked_List #Algorithm_I_Day_10_Recursion_Backtracking | ||
// #Level_1_Day_3_Linked_List #Udemy_Linked_List #Big_O_Time_O(N)_Space_O(1) | ||
// #2024_01_10_Time_57_ms_(95.02%)_Space_40.9_MB_(19.99%) | ||
|
||
using LeetCodeNet.Com_github_leetcode; | ||
|
||
/** | ||
* Definition for singly-linked list. | ||
* public class ListNode { | ||
* public int val; | ||
* public ListNode next; | ||
* public ListNode(int val=0, ListNode next=null) { | ||
* this.val = val; | ||
* this.next = next; | ||
* } | ||
* } | ||
*/ | ||
public class Solution { | ||
public ListNode ReverseList(ListNode head) { | ||
ListNode prev = null; | ||
ListNode curr = head; | ||
while (curr != null) { | ||
ListNode next = curr.next; | ||
curr.next = prev; | ||
prev = curr; | ||
curr = next; | ||
} | ||
return prev; | ||
} | ||
} | ||
} |
34 changes: 34 additions & 0 deletions
34
LeetCodeNet/G0201_0300/S0206_reverse_linked_list/readme.md
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,34 @@ | ||
206\. Reverse Linked List | ||
|
||
Easy | ||
|
||
Given the `head` of a singly linked list, reverse the list, and return _the reversed list_. | ||
|
||
**Example 1:** | ||
|
||
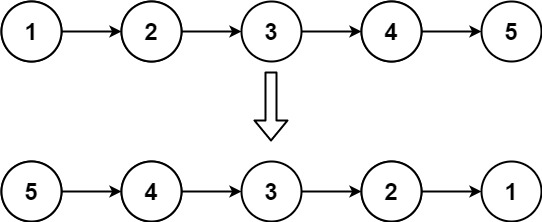 | ||
|
||
**Input:** head = [1,2,3,4,5] | ||
|
||
**Output:** [5,4,3,2,1] | ||
|
||
**Example 2:** | ||
|
||
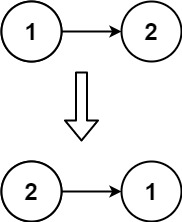 | ||
|
||
**Input:** head = [1,2] | ||
|
||
**Output:** [2,1] | ||
|
||
**Example 3:** | ||
|
||
**Input:** head = [] | ||
|
||
**Output:** [] | ||
|
||
**Constraints:** | ||
|
||
* The number of nodes in the list is the range `[0, 5000]`. | ||
* `-5000 <= Node.val <= 5000` | ||
|
||
**Follow up:** A linked list can be reversed either iteratively or recursively. Could you implement both? |
Oops, something went wrong.