-
Notifications
You must be signed in to change notification settings - Fork 2
Home
Welcome to the Bite Programming Language wiki!
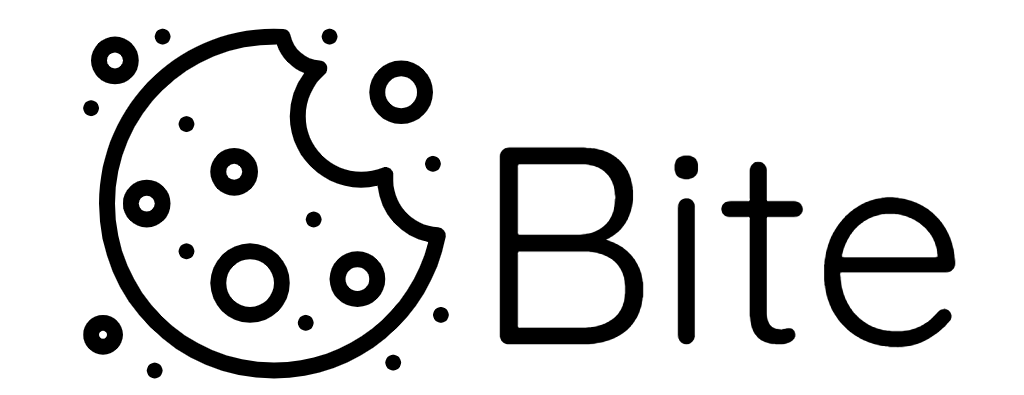
Bite (ˈbīt, pronounced the same as the word "byte") is a dynamically-typed object-oriented programming language that syntactically resembles C# and a bit of JavaScript.
The initial goal of Bite is to be a simple runtime scripting language for Unity, and any C# application, and to be able to have access to C# types and objects from within the Bite language.
Bite compiles to a bytecode that is run on a virtual machine. The reference virtual machine, BiteVM, is a stack-based virtual machine written in C#, and implemented as a library that you can import into your C# and Unity applications.
BiteVM runs on top of .NET, and supports .NET 4.6.2 as a .NET Framework library, and .NET Core 3.1 up to .NET 6.0 as a netstandard 2.0 library.
To try out Bite, you will need to download the BiteVM. It's a command line program that will compile and interpret Bite programs. You can also execute Bite code directly in BiteVM's REPL (Read Eval Print Loop) mode.
You can use your favorite editor to create Bite programs, but we have a Visual Studio Code Extension. that gives .bite programs syntax highlighting.
If you want to use the Bite virtual machine itself to add scripting capabilities to your Unity or C# application, see here
Bite code revolves around modules. To create a module, you define it with the module
directive, and then you can start writing code in it.
To try it out, create a new file named hello.bite
and enter the following code.
// My first Bite program
module HelloWorld;
import System;
System.PrintLine("Hello World!");
Save the file, then run the program using the command bitevm.exe -i hello.bite
. Congratulations, you just wrote and executed your first Bite program!
The first line of code module HelloWorld;
defines the module we are in, and should always be the first, non-comment line of code in you module.
You'll notice that the second line of code says import System;
. Importing a module makes the methods and variables of that module available to the module importing it through the module namespace. It will also run any code inside the module, if there is any. More on that later.
System
is a special module that contains useful methods, one of which is PrintLine
, which, you guessed it, prints a line of text.
The third line of code invokes Printline
through the System
module namespace.
A module encapsulates variables, functions, classes, and even code, as we just saw. Let's create a variable and a function and use it to display a message. We will also introduce the using
directive to make things easier for us.
// My second Bite program
module Example2;
import System;
using System; // so that we don't have to keep typing System before PrintLine
// declare a function
function SayMessage(message) {
PrintLine(message);
}
// declare a variable
var hello = "Hello World";
// call the function with the variable
SayMessage(hello);
One restriction of Bite is that you must declare something before you can use it. (Sorry JavaScript folks, no hoisting here!) So you cannot place the function declaration after the code that uses it.
Bite is object-oriented, meaning we can create classes within modules to further manage our code. When creating a class, create a function
with the same name as the class to define the constructor. You can define properties as variables in the class, and of course functions as well.
module Example3;
import System;
using System;
class Messenger {
// declare property "_message"
var _message;
// declare constructor
function Messager(message) {
_message = message;
}
// declare function
function SayMessage() {
PrintLine(_message);
}
}
Create an instance of a class using the new
keyword. You can pass arguments to the constructor here.
var msgr = new Messenger("Bite rocks!");
msgr.SayMessage();
If you are looking to use BiteVM in your Unity or C# application, see here