Rencana project yang ingin saya buat adalah pembelian tiket bioskop. User diminta untuk mengisikan identitas diri kemudian memilih tipe teater yang ingin dipesan. User juga menginput hari, jumlah tiket yang ingin dibeli. Kemudian diberikan beberapa judul film dengan harga yang bervariasi bergantung pada tipe teater dan hari yang ingin dipesan. lalu user memilih waktu yang telah disediakan. kemudian proses untuk total pembayaran. cetak struk pembayaran, total, dan kode untuk pembayaran
Referensi : https://www.youtube.com/watch?v=-Y6PwX6IQwQ&t=1214s
Demo Aplikasi : https://youtu.be/nYeEtwfgVYE
Nama file : dashboardController.java
Source Code :
detmov_ticketprice.setText("Rp " + Integer.toString(ti.getTicketPrice())); |
salah satu casting yang dilakukan yaitu dari tipe Integer diubah ke tipe String
Nama file : Ticket.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/Ticket.java
Lines 15 to 37 in 168b0cb
public void Ticket(String genre, String cinema) | |
{ | |
switch(genre) | |
{ | |
case "Horror": | |
g = new horror(cinema); | |
break; | |
case "Romance": | |
g = new romance(cinema); | |
break; | |
case "Comedy": | |
g = new comedy(cinema); | |
break; | |
case "Action": | |
g = new action(cinema); | |
break; | |
default: | |
{ | |
System.out.println("Invalid Genre, Please try again"); | |
System.exit(0); | |
} | |
} | |
} |
Nama file : Action.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/action.java
Lines 11 to 18 in 168b0cb
public class action extends Genre{ | |
action(String cinema) | |
{ | |
if(cinema == "Regular") | |
setTicketPrice(50000); | |
else if(cinema == "Exclusive") | |
setTicketPrice(120000); | |
} |
Nama file : Action.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/action.java
Lines 20 to 25 in 168b0cb
@Override | |
public String printGenre() | |
{ | |
return "Action"; | |
} | |
} |
Nama file : getData.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/getData.java
Lines 14 to 82 in 168b0cb
public class getData { | |
private static String movieName; | |
private static String username; | |
private static LocalDate date; | |
private static int quantity; | |
/** | |
* @return the username | |
*/ | |
public static String getUsername() { | |
return username; | |
} | |
/** | |
* @param username the username to set | |
*/ | |
public static void setUsername(String user) { | |
username = user; | |
} | |
/** | |
* @return the quantity | |
*/ | |
public static int getQuantity() { | |
return quantity; | |
} | |
public static int getQueantity(String dsc) | |
{ | |
return quantity + 1; | |
} | |
/** | |
* @param aQuantity the quantity to set | |
*/ | |
public static void setQuantity(int aQuantity) { | |
quantity = aQuantity; | |
} | |
/** | |
* @return the date | |
*/ | |
public static LocalDate getDate() { | |
return date; | |
} | |
/** | |
* @param aDate the date to set | |
*/ | |
public static void setDate(LocalDate aDate) { | |
date = aDate; | |
} | |
/** | |
* @return the movieName | |
*/ | |
public static String getMovieName() { | |
return movieName; | |
} | |
/** | |
* @param aMovieName the movieName to set | |
*/ | |
public static void setMovieName(String aMovieName) { | |
movieName = aMovieName; | |
} | |
} |
Nama file : Genre.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/Genre.java
Lines 11 to 35 in 168b0cb
public class Genre extends abstractGenre{ | |
private int ticketPrice; | |
/** | |
* @return the ticketPrice | |
*/ | |
@Override | |
public int getTicketPrice() { | |
return ticketPrice; | |
} | |
/** | |
* @param ticketPrice the ticketPrice to set | |
*/ | |
@Override | |
public void setTicketPrice(int ticketPrice) { | |
this.ticketPrice = ticketPrice; | |
} | |
@Override | |
public String printGenre() | |
{ | |
return "Invalid"; | |
} | |
} |
Nama file : abstractGenre.java
Source Code :
abstract public class abstractGenre { | |
abstract public int getTicketPrice(); | |
abstract public void setTicketPrice(int ticketPrice); | |
abstract public String printGenre(); | |
} |
Nama file : interfaceTicket.java
Source Code :
interface InterfaceTicket { | |
public void Ticket(String genre, String cinema); | |
public void ResetPrice(); | |
} |
Nama file : dashboardController.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/dashboardController.java
Lines 186 to 187 in 168b0cb
HashMap<String,String> movieGenre = new HashMap(); | |
HashMap<String,Image> movieImage = new HashMap(); |
Nama file : FXMLDocumentController.java
Source Code :
File f = new File("Logins"); |
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/FXMLDocumentController.java
Lines 95 to 108 in 168b0cb
public void readFile() | |
{ | |
try { | |
FileReader fr = new FileReader(f+"\\Accounts.txt"); | |
//System.out.println("file exists!"); | |
} catch (FileNotFoundException ex) { | |
try { | |
FileWriter fw = new FileWriter(f+"\\Accounts.txt"); | |
//System.out.println("file created"); | |
} catch (IOException ex1) { | |
Logger.getLogger(FXMLDocumentController.class.getName()).log(Level.SEVERE, null, ex1); | |
} | |
} | |
} |
Nama file : FXMLDocumentController.java
Source Code :
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/FXMLDocumentController.java
Lines 97 to 107 in 168b0cb
try { | |
FileReader fr = new FileReader(f+"\\Accounts.txt"); | |
//System.out.println("file exists!"); | |
} catch (FileNotFoundException ex) { | |
try { | |
FileWriter fw = new FileWriter(f+"\\Accounts.txt"); | |
//System.out.println("file created"); | |
} catch (IOException ex1) { | |
Logger.getLogger(FXMLDocumentController.class.getName()).log(Level.SEVERE, null, ex1); | |
} | |
} |
Nama file : FXMLDocument.fxml dan dashboard.fxml
FP-OOP2022/MovieTheatreSystem/src/movietheatresystem/dashboard.fxml
Lines 1 to 501 in 168b0cb
<?xml version="1.0" encoding="UTF-8"?> | |
<?import de.jensd.fx.glyphs.fontawesome.FontAwesomeIcon?> | |
<?import javafx.scene.control.Button?> | |
<?import javafx.scene.control.ComboBox?> | |
<?import javafx.scene.control.DatePicker?> | |
<?import javafx.scene.control.Hyperlink?> | |
<?import javafx.scene.control.Label?> | |
<?import javafx.scene.control.Spinner?> | |
<?import javafx.scene.control.TextField?> | |
<?import javafx.scene.effect.Shadow?> | |
<?import javafx.scene.image.Image?> | |
<?import javafx.scene.image.ImageView?> | |
<?import javafx.scene.layout.AnchorPane?> | |
<?import javafx.scene.layout.BorderPane?> | |
<?import javafx.scene.shape.Line?> | |
<?import javafx.scene.text.Font?> | |
<?import javafx.scene.text.Text?> | |
<AnchorPane id="AnchorPane" fx:id="dashboardForm" prefHeight="656.0" prefWidth="1249.0" xmlns="http://javafx.com/javafx/19" xmlns:fx="http://javafx.com/fxml/1" fx:controller="movietheatresystem.dashboardController"> | |
<children> | |
<BorderPane layoutX="425.0" layoutY="92.0" prefHeight="656.0" prefWidth="1249.0" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<top> | |
<AnchorPane prefHeight="45.0" prefWidth="1250.0" BorderPane.alignment="CENTER"> | |
<children> | |
<AnchorPane fx:id="top_form" layoutX="437.0" layoutY="-77.0" prefHeight="123.2" prefWidth="1249.6" styleClass="top-form" stylesheets="@dashboardDesain.css" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<Button fx:id="close_btn" layoutX="1198.0" layoutY="1.0" mnemonicParsing="false" onAction="#close" prefHeight="24.0" prefWidth="50.0" styleClass="close_button" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<Button fx:id="minimize" layoutX="1148.0" layoutY="1.0" mnemonicParsing="false" onAction="#minimizebtn" prefHeight="24.0" prefWidth="50.0" styleClass="close_button" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="MINUS" /> | |
</graphic> | |
</Button> | |
<Label layoutX="43.0" layoutY="9.0" prefHeight="26.0" prefWidth="265.0" text="Movie Theatre Booking Ticket"> | |
<font> | |
<Font name="System Bold" size="16.0" /> | |
</font> | |
</Label> | |
<FontAwesomeIcon glyphName="PLAY_CIRCLE" layoutX="15.0" layoutY="28.0" size="18" wrappingWidth="19.828596115112305" /> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
</top> | |
<left> | |
<AnchorPane prefHeight="610.0" prefWidth="240.0" styleClass="bg_left" stylesheets="@dashboardDesain.css" BorderPane.alignment="CENTER"> | |
<children> | |
<FontAwesomeIcon fill="#191654" glyphName="USER" layoutX="75.0" layoutY="111.0" selectionFill="#252469" size="3cm" /> | |
<Label alignment="CENTER" layoutX="76.0" layoutY="135.0" text="Welcome" textFill="WHITE"> | |
<font> | |
<Font name="System Bold" size="19.0" /> | |
</font> | |
</Label> | |
<Button fx:id="nav_bookbtn" alignment="CENTER" layoutX="14.0" layoutY="241.0" mnemonicParsing="false" onAction="#navigationSelect" prefHeight="43.0" prefWidth="205.0" styleClass="left_button" stylesheets="@dashboardDesain.css" text="Booking" textFill="WHITE"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="DASHBOARD" size="18" /> | |
</graphic> | |
<font> | |
<Font name="System Bold" size="12.0" /> | |
</font> | |
</Button> | |
<Button fx:id="nav_nowShowingbtn" alignment="CENTER" layoutX="14.0" layoutY="284.0" mnemonicParsing="false" onAction="#navigationSelect" prefHeight="43.0" prefWidth="205.0" styleClass="left_button" stylesheets="@dashboardDesain.css" text="Now Showing"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="PLAY" size="18" /> | |
</graphic> | |
<font> | |
<Font name="System Bold" size="12.0" /> | |
</font> | |
</Button> | |
<Button fx:id="sign_out" layoutX="13.0" layoutY="559.0" mnemonicParsing="false" onAction="#signOut" prefHeight="38.0" prefWidth="104.0" styleClass="left_button" stylesheets="@dashboardDesain.css" text="Sign Out"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="SIGN_OUT" selectionFill="#b4b4b4" size="22" text="" /> | |
</graphic> | |
<font> | |
<Font name="System Bold" size="12.0" /> | |
</font> | |
</Button> | |
<Label fx:id="nav_username" alignment="TOP_CENTER" contentDisplay="CENTER" layoutX="37.0" layoutY="163.0" prefHeight="28.0" prefWidth="160.0" text="username" textAlignment="CENTER" textFill="WHITE"> | |
<font> | |
<Font name="System Bold" size="19.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
</left> | |
<center> | |
<AnchorPane prefHeight="200.0" prefWidth="200.0" styleClass="center_bg" stylesheets="@dashboardDesain.css" BorderPane.alignment="CENTER"> | |
<children> | |
<AnchorPane fx:id="page_booking" layoutX="68.0" layoutY="58.0" prefHeight="610.4" prefWidth="1009.6" visible="false" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<AnchorPane layoutX="14.0" layoutY="14.0" prefHeight="582.0" prefWidth="483.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<DatePicker fx:id="book_date" editable="false" layoutX="150.0" layoutY="170.0" onAction="#setDate" promptText="MM/DD/YY" /> | |
<Label layoutX="27.0" layoutY="44.0" text="Ticket booking"> | |
<font> | |
<Font name="System Bold" size="35.0" /> | |
</font> | |
</Label> | |
<Label alignment="CENTER_RIGHT" layoutX="72.0" layoutY="173.0" prefHeight="18.0" prefWidth="56.0" text="Date :" /> | |
<Label alignment="CENTER_RIGHT" layoutX="34.0" layoutY="139.0" prefHeight="18.0" prefWidth="93.0" text="Select Movie :" /> | |
<Label alignment="CENTER_RIGHT" layoutX="64.0" layoutY="206.0" prefHeight="18.0" prefWidth="64.0" text="Quantity :" /> | |
<Spinner fx:id="book_quantity" editable="true" layoutX="150.0" layoutY="202.0" prefHeight="26.0" prefWidth="174.0" visible="false" /> | |
<Button fx:id="book_buy" layoutX="171.0" layoutY="291.0" mnemonicParsing="false" onAction="#buy" prefHeight="73.0" prefWidth="141.0" styleClass="buy_btn" stylesheets="@dashboardDesain.css" text="Buy"> | |
<font> | |
<Font name="System Bold" size="27.0" /> | |
</font> | |
</Button> | |
<Button fx:id="book_clear" layoutX="198.0" layoutY="385.0" mnemonicParsing="false" onAction="#clear" prefHeight="25.0" prefWidth="88.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" text="Clear"> | |
<font> | |
<Font name="System Bold" size="16.0" /> | |
</font> | |
</Button> | |
<TextField fx:id="book_intQuantity" layoutX="151.0" layoutY="203.0" onAction="#quantityCheck" prefHeight="26.0" prefWidth="174.0" promptText="0" /> | |
<ComboBox fx:id="book_movies" layoutX="150.0" layoutY="135.0" onAction="#comboBox" prefHeight="26.0" prefWidth="174.0" promptText="Choose" styleClass="bg_white" stylesheets="@dashboardDesain.css" /> | |
<Button layoutX="332.0" layoutY="204.0" mnemonicParsing="false" onAction="#quantityCheck"> | |
<graphic> | |
<FontAwesomeIcon fx:id="qtycheck_btn" glyphName="CHECK" /> | |
</graphic> | |
</Button> | |
<Label layoutX="150.0" layoutY="232.0" text="*Please click the check box above to update" textFill="RED" /> | |
</children> | |
</AnchorPane> | |
<AnchorPane layoutX="513.0" layoutY="14.0" prefHeight="582.0" prefWidth="483.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<Label alignment="CENTER_RIGHT" layoutX="97.0" layoutY="369.0" text="Movie Title :" /> | |
<Label alignment="CENTER_RIGHT" layoutX="106.0" layoutY="400.0" prefHeight="18.0" prefWidth="56.0" text="Genre :" /> | |
<Label fx:id="detmov_title" layoutX="185.0" layoutY="366.0" prefHeight="18.0" prefWidth="211.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Movie Title" /> | |
<Label fx:id="detmov_genre" layoutX="185.0" layoutY="397.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Genre" /> | |
<Label alignment="CENTER_RIGHT" layoutX="106.0" layoutY="430.0" prefHeight="18.0" prefWidth="56.0" text="Date :" /> | |
<Label fx:id="detmov_date" layoutX="185.0" layoutY="427.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Date" /> | |
<Label alignment="CENTER_RIGHT" layoutX="64.0" layoutY="460.0" prefHeight="18.0" prefWidth="98.0" text="Ticket Price :" /> | |
<Label fx:id="detmov_ticketprice" layoutX="185.0" layoutY="457.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Ticket Price" /> | |
<Label alignment="CENTER_RIGHT" layoutX="64.0" layoutY="488.0" prefHeight="18.0" prefWidth="98.0" text="Total :" /> | |
<Label fx:id="detmov_total" layoutX="185.0" layoutY="485.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Total Price" /> | |
<ImageView fx:id="detmov_img" fitHeight="314.0" fitWidth="209.0" layoutX="137.0" layoutY="29.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<effect> | |
<Shadow /> | |
</effect> | |
</ImageView> | |
<FontAwesomeIcon glyphName="MONEY" layoutX="374.0" layoutY="501.0" /> | |
<FontAwesomeIcon glyphName="MONEY" layoutX="374.0" layoutY="473.0" /> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="page_select" prefHeight="610.4" prefWidth="1009.6" visible="false" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<Label alignment="CENTER" layoutX="348.0" layoutY="69.0" text="Select Theatre"> | |
<font> | |
<Font name="System Bold" size="47.0" /> | |
</font> | |
</Label> | |
<Button fx:id="select_regular" layoutX="236.0" layoutY="227.0" mnemonicParsing="false" onAction="#theatreSelect" prefHeight="204.0" prefWidth="222.0" styleClass="buy_btn" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="STAR" size="4cm" /> | |
</graphic> | |
</Button> | |
<Button fx:id="select_exclusive" layoutX="550.0" layoutY="227.0" mnemonicParsing="false" onAction="#theatreSelect" prefHeight="204.0" prefWidth="222.0" styleClass="buy_btn" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="DIAMOND" size="4cm" /> | |
</graphic> | |
</Button> | |
<Label alignment="CENTER" layoutX="292.0" layoutY="439.0" text="REGULAR"> | |
<font> | |
<Font name="System Bold" size="25.0" /> | |
</font> | |
</Label> | |
<Label alignment="CENTER" layoutX="597.0" layoutY="439.0" text="EXCLUSIVE"> | |
<font> | |
<Font name="System Bold" size="25.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="Page_receipt" prefHeight="610.4" prefWidth="1009.6" visible="false" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<AnchorPane fx:id="receipt" layoutX="158.0" layoutY="97.0" prefHeight="370.0" prefWidth="641.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<Label alignment="CENTER" layoutX="170.0" layoutY="24.0" text="Receipt"> | |
<font> | |
<Font name="System Bold" size="40.0" /> | |
</font> | |
</Label> | |
<Label alignment="CENTER_RIGHT" layoutX="84.0" layoutY="178.0" text="Movie Title :" /> | |
<Label fx:id="re_mov" layoutX="172.0" layoutY="175.0" prefHeight="18.0" prefWidth="211.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Movie Title" /> | |
<Label alignment="CENTER_RIGHT" layoutX="92.0" layoutY="208.0" prefHeight="18.0" prefWidth="56.0" text="Date :" /> | |
<Label fx:id="re_date" layoutX="171.0" layoutY="205.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Date" /> | |
<Label alignment="CENTER_RIGHT" layoutX="50.0" layoutY="238.0" prefHeight="18.0" prefWidth="98.0" text="Ticket Price :" /> | |
<Label fx:id="re_price" layoutX="171.0" layoutY="235.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Ticket Price" /> | |
<Label alignment="CENTER_RIGHT" layoutX="50.0" layoutY="270.0" prefHeight="18.0" prefWidth="98.0" text="Total :" /> | |
<Label fx:id="re_tot" layoutX="170.0" layoutY="267.0" prefHeight="18.0" prefWidth="210.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Total Price" /> | |
<Label alignment="CENTER_RIGHT" layoutX="65.0" layoutY="147.0" text="Payment Code :" /> | |
<Label fx:id="re_code" layoutX="171.0" layoutY="144.0" prefHeight="18.0" prefWidth="211.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="xxxxxxxxxxxxxx" /> | |
<Label alignment="CENTER_RIGHT" layoutX="109.0" layoutY="116.0" text="Name :" /> | |
<Label fx:id="re_user" layoutX="170.0" layoutY="113.0" prefHeight="18.0" prefWidth="211.0" styleClass="label_info" stylesheets="@dashboardDesain.css" text="Username" /> | |
<Line endX="100.0" layoutX="240.0" layoutY="82.0" startX="-100.0" /> | |
<Hyperlink fx:id="hype_close" layoutX="170.0" layoutY="310.0" onAction="#hyp_info" text="Information" /> | |
<Line endX="18.00006103515625" endY="231.39999389648438" layoutX="495.0" layoutY="147.0" startX="18.00006103515625" startY="-147.0" /> | |
<Line endX="18.00006103515625" endY="236.80001831054688" layoutX="593.0" layoutY="141.0" startX="18.00006103515625" startY="-140.0" /> | |
<Text layoutX="442.0" layoutY="207.0" strokeType="OUTSIDE" strokeWidth="0.0" styleClass="vertical_rotate" text="CINEMA 4X" wrappingWidth="232.21949768066406"> | |
<font> | |
<Font size="45.0" /> | |
</font> | |
</Text> | |
<AnchorPane fx:id="information_box" layoutX="123.0" layoutY="34.0" prefHeight="258.0" prefWidth="446.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" visible="false"> | |
<children> | |
<Label alignment="CENTER" layoutX="192.0" layoutY="24.0" text="Information" /> | |
<Button fx:id="info_close" layoutX="400.0" mnemonicParsing="false" onAction="#close_info" prefHeight="24.0" prefWidth="46.0"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<Text layoutX="42.0" layoutY="73.0" strokeType="OUTSIDE" strokeWidth="0.0" text="1. complete your payment immediately within 2 hours so that the booking can be processed" wrappingWidth="270.9366455078125" /> | |
<Text layoutX="42.0" layoutY="117.0" strokeType="OUTSIDE" strokeWidth="0.0" text="2. The payment code will be used when you want to pick up a ticket at the cinema, so please note it down" wrappingWidth="270.9366455078125" /> | |
<Text layoutX="42.0" layoutY="178.0" strokeType="OUTSIDE" strokeWidth="0.0" text="3. Seat selection will be made when picking up tickets at the cinema" wrappingWidth="270.9366455078125" /> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
<Button fx:id="re_back" layoutX="446.0" layoutY="503.0" mnemonicParsing="false" onAction="#back_dash" prefHeight="12.0" prefWidth="117.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" text="Back to dashboard" /> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="nowshowing" layoutX="81.0" layoutY="77.0" prefHeight="610.4" prefWidth="1009.6" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<AnchorPane layoutX="14.0" layoutY="14.0" prefHeight="582.0" prefWidth="983.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<Label layoutX="348.0" layoutY="37.0" prefHeight="88.0" prefWidth="286.0" text="Now Showing"> | |
<font> | |
<Font name="System Bold" size="42.0" /> | |
</font> | |
</Label> | |
<AnchorPane fx:id="fsg" layoutX="94.0" layoutY="154.0" onMouseClicked="#now_showingSelect" prefHeight="226.0" prefWidth="158.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<ImageView fx:id="ns_fsg" fitHeight="251.0" fitWidth="169.0" onMouseClicked="#now_showingSelect" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/horror_fsgame.jpg" /> | |
</image> | |
</ImageView> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="mt" layoutX="297.0" layoutY="154.0" prefHeight="226.0" prefWidth="158.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<ImageView fx:id="ns_mt" fitHeight="251.0" fitWidth="169.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/metimecomedy.jpg" /> | |
</image> | |
</ImageView> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="spd" layoutX="506.0" layoutY="154.0" prefHeight="226.0" prefWidth="158.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<ImageView fx:id="ns_spd" fitHeight="251.0" fitWidth="169.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/nowayhome.jpg" /> | |
</image> | |
</ImageView> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="yn" layoutX="720.0" layoutY="154.0" prefHeight="226.0" prefWidth="158.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<children> | |
<ImageView fx:id="ns_yn" fitHeight="251.0" fitWidth="169.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/romance_yourname.jpg" /> | |
</image> | |
</ImageView> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="fsg_preview" layoutX="94.0" layoutY="37.0" prefHeight="508.0" prefWidth="795.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" visible="false"> | |
<children> | |
<Button fx:id="fsg_close" layoutX="372.0" layoutY="480.0" mnemonicParsing="false" prefHeight="24.0" prefWidth="51.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<ImageView fx:id="img_fsg" fitHeight="337.0" fitWidth="231.0" layoutX="58.0" layoutY="53.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/horror_fsgame.jpg" /> | |
</image> | |
</ImageView> | |
<Line endX="-100.0" endY="389.0000305175781" layoutX="417.0" layoutY="59.0" startX="-100.0" /> | |
<Label layoutX="81.0" layoutY="404.0" text="The Friendship Game"> | |
<font> | |
<Font name="System Bold" size="18.0" /> | |
</font> | |
</Label> | |
<Text layoutX="343.0" layoutY="135.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Teens in a small town come across an object with mysterious cosmic powers and a baffling sort of consciousness. Over the course of a night, the object tests their loyalties and unravels the mystery about their missing friend." wrappingWidth="416.2808837890625"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Text> | |
<Label layoutX="343.0" layoutY="89.0" text="Synopsis :"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="344.0" layoutY="245.0" text="Director : Scooter Corkle"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="272.0" text="Producer : Daniel Bekerman"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="299.0" text="Language : English"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="345.0" layoutY="326.0" text="Duration : 87 min"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="mt_preview" layoutX="94.0" layoutY="38.0" prefHeight="508.0" prefWidth="795.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" visible="false"> | |
<children> | |
<Button fx:id="mt_close" layoutX="372.0" layoutY="480.0" mnemonicParsing="false" prefHeight="24.0" prefWidth="51.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<ImageView fx:id="img_mt" fitHeight="337.0" fitWidth="231.0" layoutX="58.0" layoutY="53.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/metimecomedy.jpg" /> | |
</image> | |
</ImageView> | |
<Line endX="-100.0" endY="389.0000305175781" layoutX="417.0" layoutY="59.0" startX="-100.0" /> | |
<Label alignment="CENTER" layoutX="133.0" layoutY="405.0" text="Me Time"> | |
<font> | |
<Font name="System Bold" size="18.0" /> | |
</font> | |
</Label> | |
<Text layoutX="343.0" layoutY="135.0" strokeType="OUTSIDE" strokeWidth="0.0" text="With his family away, a stay-at-home dad enjoys his first me-time in years by reconnecting with an old friend for a wild weekend that may upend his life." wrappingWidth="416.2808837890625"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Text> | |
<Label layoutX="343.0" layoutY="89.0" text="Synopsis :"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="344.0" layoutY="245.0" text="Director : John Hamburg"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="272.0" text="Producer : Kevin Hart, John Hamburg, Bryan Smiley"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="299.0" text="Language : English"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="345.0" layoutY="326.0" text="Duration : 101 min"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="spd_preview" layoutX="94.0" layoutY="39.0" prefHeight="508.0" prefWidth="795.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" visible="false"> | |
<children> | |
<Button fx:id="spd_close" layoutX="372.0" layoutY="480.0" mnemonicParsing="false" prefHeight="24.0" prefWidth="51.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<ImageView fx:id="img_spd" fitHeight="337.0" fitWidth="231.0" layoutX="58.0" layoutY="53.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/nowayhome.jpg" /> | |
</image> | |
</ImageView> | |
<Line endX="-100.0" endY="389.0000305175781" layoutX="417.0" layoutY="59.0" startX="-100.0" /> | |
<Label alignment="CENTER" layoutX="54.0" layoutY="402.0" text="Spider Man: No Way Home"> | |
<font> | |
<Font name="System Bold" size="18.0" /> | |
</font> | |
</Label> | |
<Text layoutX="343.0" layoutY="135.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Spider-Man seeks the help of Doctor Strange to forget his exposed secret identity as Peter Parker. However, Strange's spell goes horribly wrong, leading to unwanted guests entering their universe." wrappingWidth="416.2808837890625"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Text> | |
<Label layoutX="343.0" layoutY="89.0" text="Synopsis :"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="344.0" layoutY="245.0" text="Director : Jon Watts"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="272.0" text="Producer : Kevin Feige, Amy Pascal"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="299.0" text="Language : English"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="345.0" layoutY="326.0" text="Duration : 148 min"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
<AnchorPane fx:id="yn_preview" layoutX="94.0" layoutY="39.0" prefHeight="508.0" prefWidth="795.0" styleClass="bg_white" stylesheets="@dashboardDesain.css" visible="false"> | |
<children> | |
<Button fx:id="yn_close" layoutX="372.0" layoutY="480.0" mnemonicParsing="false" prefHeight="24.0" prefWidth="51.0" styleClass="bg_white" stylesheets="@dashboardDesain.css"> | |
<graphic> | |
<FontAwesomeIcon glyphName="CLOSE" /> | |
</graphic> | |
</Button> | |
<ImageView fx:id="img_yn" fitHeight="337.0" fitWidth="231.0" layoutX="58.0" layoutY="53.0" pickOnBounds="true" preserveRatio="true" styleClass="bg_white"> | |
<image> | |
<Image url="@../../movie/romance_yourname.jpg" /> | |
</image> | |
</ImageView> | |
<Line endX="-100.0" endY="389.0000305175781" layoutX="417.0" layoutY="59.0" startX="-100.0" /> | |
<Label alignment="CENTER" layoutX="122.0" layoutY="404.0" text="Your Name"> | |
<font> | |
<Font name="System Bold" size="18.0" /> | |
</font> | |
</Label> | |
<Text layoutX="343.0" layoutY="135.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Two teenagers share a profound, magical connection upon discovering they are swapping bodies. Things manage to become even more complicated when the boy and girl decide to meet in person." wrappingWidth="416.2808837890625"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Text> | |
<Label layoutX="343.0" layoutY="89.0" text="Synopsis :"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="344.0" layoutY="245.0" text="Director : Makoto Shinkai"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="272.0" text="Producer : Koichiro Ito, Katsuhiro Takei"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="343.0" layoutY="299.0" text="Language : Japanese"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
<Label layoutX="345.0" layoutY="326.0" text="Duration : 107 min"> | |
<font> | |
<Font size="18.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
<Text layoutX="109.0" layoutY="431.0" strokeType="OUTSIDE" strokeWidth="0.0" text="The Friendship Game" textAlignment="CENTER"> | |
<font> | |
<Font name="System Bold" size="14.0" /> | |
</font> | |
</Text> | |
<Text layoutX="352.0" layoutY="430.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Me Time" textAlignment="CENTER"> | |
<font> | |
<Font name="System Bold" size="14.0" /> | |
</font> | |
</Text> | |
<Text layoutX="499.0" layoutY="429.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Spider-Man: No Way Home" textAlignment="CENTER"> | |
<font> | |
<Font name="System Bold" size="14.0" /> | |
</font> | |
</Text> | |
<Text layoutX="767.0" layoutY="430.0" strokeType="OUTSIDE" strokeWidth="0.0" text="Your Name" textAlignment="CENTER"> | |
<font> | |
<Font name="System Bold" size="14.0" /> | |
</font> | |
</Text> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
</center> | |
</BorderPane> | |
</children> | |
</AnchorPane> |
<?xml version="1.0" encoding="UTF-8"?> | |
<?import de.jensd.fx.glyphs.fontawesome.FontAwesomeIcon?> | |
<?import javafx.scene.control.Button?> | |
<?import javafx.scene.control.Hyperlink?> | |
<?import javafx.scene.control.Label?> | |
<?import javafx.scene.control.PasswordField?> | |
<?import javafx.scene.control.TextField?> | |
<?import javafx.scene.layout.AnchorPane?> | |
<?import javafx.scene.text.Font?> | |
<AnchorPane id="AnchorPane" fx:id="mainform" prefHeight="464.0" prefWidth="732.0" xmlns="http://javafx.com/javafx/19" xmlns:fx="http://javafx.com/fxml/1" fx:controller="movietheatresystem.FXMLDocumentController"> | |
<children> | |
<AnchorPane fx:id="sign_form" prefHeight="464.0" prefWidth="732.0" styleClass="bg-form" stylesheets="@loginDesain.css" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<Button fx:id="sign_close" layoutX="678.0" layoutY="-1.0" minWidth="26.0" mnemonicParsing="false" onAction="#signIn_closes" prefHeight="26.0" prefWidth="54.0" styleClass="close" stylesheets="@loginDesain.css" textFill="WHITE"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="CLOSE" selectionFill="BLACK" size="16" text="" /> | |
</graphic> | |
</Button> | |
<FontAwesomeIcon fill="#191654" glyphName="USER" layoutX="307.0" layoutY="155.0" selectionEnd="0" size="4cm" /> | |
<Label layoutX="321.0" layoutY="180.0" text="Sign In" textFill="WHITE"> | |
<font> | |
<Font name="System Bold" size="30.0" /> | |
</font> | |
</Label> | |
<Button fx:id="sign_login" layoutX="307.0" layoutY="335.0" mnemonicParsing="false" onAction="#login" prefHeight="46.0" prefWidth="125.0" styleClass="close" stylesheets="@loginDesain.css" text="Login" textFill="WHITE" /> | |
<Hyperlink fx:id="sign_hyperlink" layoutX="342.0" layoutY="427.0" onAction="#signinpop" text="Sign Up" /> | |
<TextField fx:id="sign_username" alignment="CENTER" layoutX="273.0" layoutY="244.0" onAction="#login" prefHeight="26.0" prefWidth="194.0" promptText="Username" styleClass="textfield" stylesheets="@loginDesain.css" /> | |
<PasswordField fx:id="sign_pass" alignment="CENTER" layoutX="272.0" layoutY="292.0" prefHeight="26.0" prefWidth="194.0" promptText="Password" styleClass="textfield" stylesheets="@loginDesain.css" /> | |
<AnchorPane fx:id="signup" prefHeight="464.0" prefWidth="732.4" styleClass="bg-form" stylesheets="@loginDesain.css" visible="false" AnchorPane.bottomAnchor="0.0" AnchorPane.leftAnchor="0.0" AnchorPane.rightAnchor="0.0" AnchorPane.topAnchor="0.0"> | |
<children> | |
<Button fx:id="sign_close1" layoutX="678.0" layoutY="-1.0" minWidth="26.0" mnemonicParsing="false" onAction="#signIn_closes" prefHeight="26.0" prefWidth="54.0" styleClass="close" stylesheets="@loginDesain.css" textFill="WHITE"> | |
<graphic> | |
<FontAwesomeIcon fill="WHITE" glyphName="CLOSE" selectionFill="BLACK" size="16" text="" /> | |
</graphic> | |
</Button> | |
<FontAwesomeIcon fill="#191654" glyphName="USERS" layoutX="285.0" layoutY="147.0" selectionEnd="0" size="4cm" /> | |
<PasswordField fx:id="up_password" alignment="CENTER" layoutX="268.0" layoutY="268.0" prefHeight="26.0" prefWidth="194.0" promptText="Password" styleClass="textfield" stylesheets="@loginDesain.css" /> | |
<TextField fx:id="up_username" alignment="CENTER" layoutX="268.0" layoutY="232.0" prefHeight="26.0" prefWidth="194.0" promptText="Username" styleClass="textfield" stylesheets="@loginDesain.css" /> | |
<TextField fx:id="up_email" alignment="CENTER" layoutX="268.0" layoutY="305.0" prefHeight="26.0" prefWidth="194.0" promptText="Email" styleClass="textfield" stylesheets="@loginDesain.css" /> | |
<Hyperlink fx:id="up_hyperlink" layoutX="344.0" layoutY="427.0" onAction="#signinpop" text="Sign In" /> | |
<Button fx:id="up_create" layoutX="300.0" layoutY="351.0" mnemonicParsing="false" onAction="#singup_action" prefHeight="46.0" prefWidth="130.0" styleClass="close" stylesheets="@loginDesain.css" text="Create a new account" textFill="WHITE" /> | |
<Label alignment="CENTER" layoutX="294.0" layoutY="178.0" prefWidth="144.0" text="Sign Up" textFill="WHITE"> | |
<font> | |
<Font name="System Bold" size="30.0" /> | |
</font> | |
</Label> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> | |
</children> | |
</AnchorPane> |
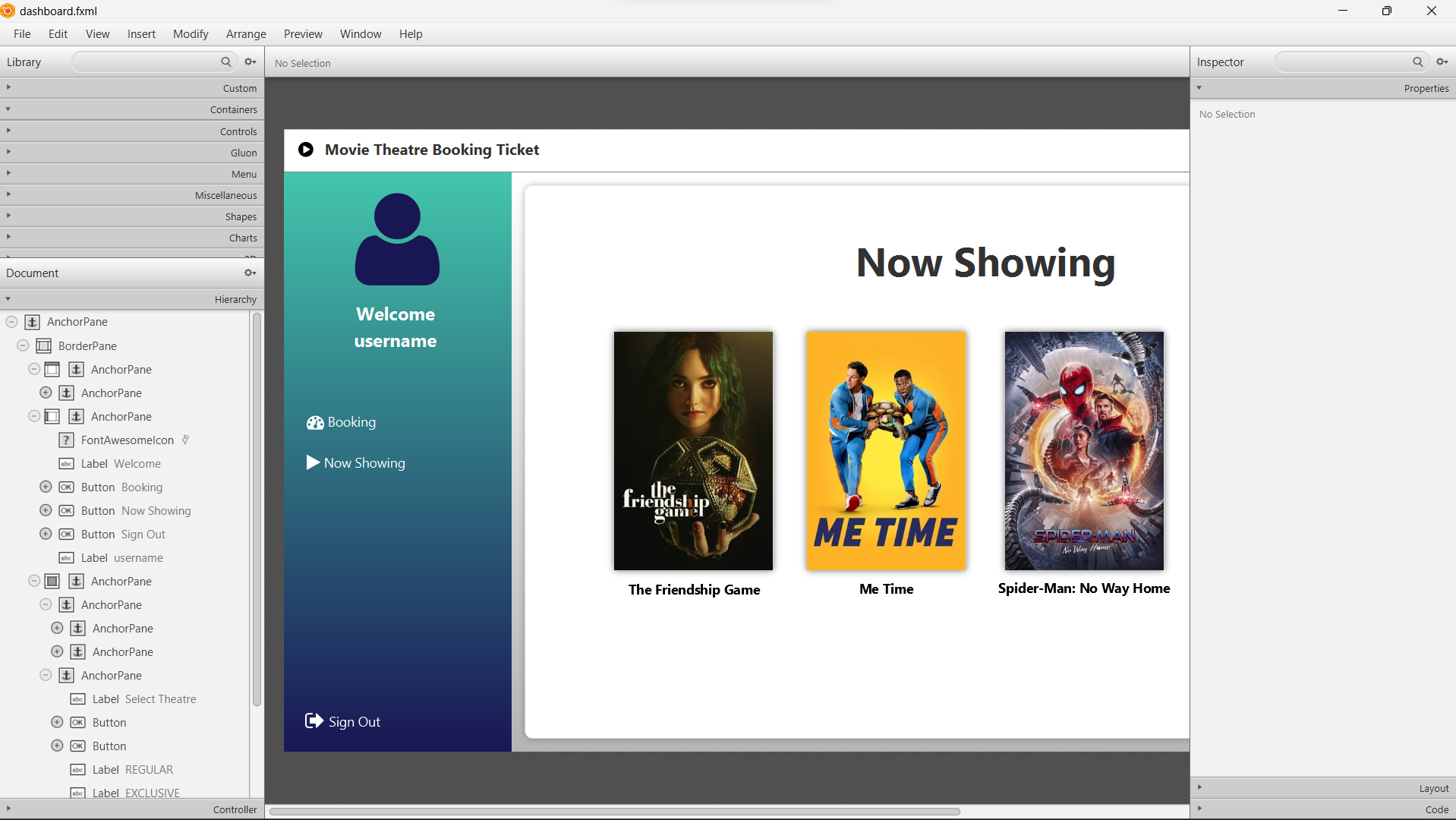
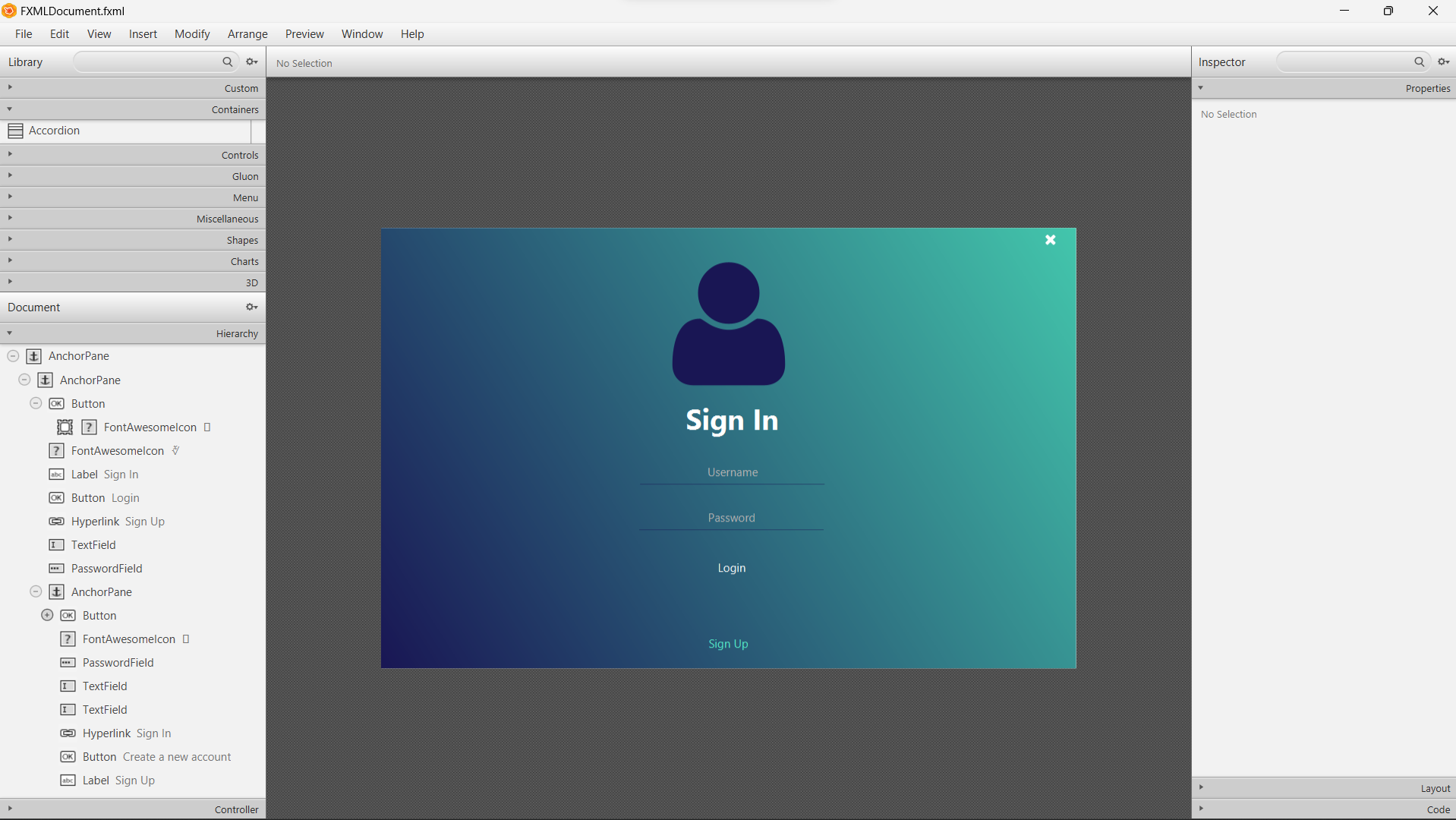
membuat user interface menggunakan sceneBuilder dan library tambahan yaitu fontawesomefx-8.2.jar
Nama file : dashboardController.java
ObservableList<String> list = FXCollections.observableArrayList(movieList); |