-
Notifications
You must be signed in to change notification settings - Fork 6
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
* include rendering classes - GSDevices null - DX12 - enums for devices - adjusted example to obtain instance pointers { device, renderer, thread } * restructure PCSX2 Package Moved all PCSX2 logic to its own package which will contain Classes, Structs, Methods and enums. GamePackage Game packages have been moved to their own contained subfolder in the sdk directory. This was done to maintain some level of neatness and exposure as the SDK grows in size * Update README.md * dx11 present hook template * update engine class Engine - include dx11 hook template Readme - included examples * readme * static memory class adjusted the entirety of the memory class for static access. Constructor method will populate both EEMemory and PS2BaseAddress. Being that these 2 values will always be the same for the lifetime of the module , there is no need to obtain it consistently. Tools class was also adjusted to be a static class all pointers for accessing members have been removed due to increased confusion * Update dllmain.cpp
- Loading branch information
Showing
22 changed files
with
710 additions
and
294 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,21 +1,100 @@ | ||
# PCSX2 Menu Trainer Development Kit | ||
## CODE EXAMPLE | ||
PCSX2 Menu Trainer Development Kit is a C++ library designed to simplify the process of creating cheats for the PCSX2 emulator. | ||
It aims to bridge the gap between modern cheat development practices and the classic era of cheat codes. | ||
This framework provides tools to port older codes to a more modern approach, using additional libraries such as dear imgui and minhook. | ||
|
||
|  | 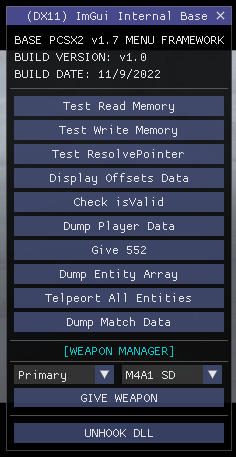 | 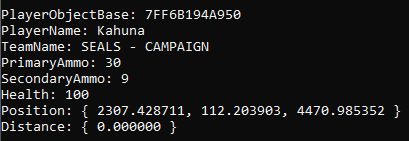 | | ||
| :---: | :---: | :---: | | ||
|
||
## GENERAL USAGE | ||
1. include the SDK header as well as any required packages. | ||
*NOTE: Engine_Package.h is a required package and needs to be manually included.* | ||
- `SDK.h` // Including the SDK header will provide access to all the most necessary features. | ||
- `Engine_Package.cpp` // Core Package and must be included for basic usage | ||
- `PCSX2_Package.cpp` // Contains PCSX2 specific members and functions. Like the rendering API's for instance | ||
|
||
2. Initialize the SDK | ||
- `PlayStation2::InitSDK();` | ||
|
||
3. Do Stuff | ||
https://github.com/NightFyre/PCSX2-CheatFrameWork/blob/1cdaa7f5a22868f86a9a7beeb7a637c6f58a8f8e/dllmain.cpp#L8-L44 | ||
|
||
## CODE EXAMPLES | ||
|
||
- Get Physical Address | ||
```cpp | ||
// - Example Pnach: Save Anywhere by Codejunkies ( Kingdom Hearts (NTSC-UC) ) | ||
// - patch=1,EE,204865E0,extended,00114288 | ||
// - Raw Address -> 204865E0 | ||
|
||
// Extract offset from raw address (remove "0x20") | ||
unsigned int offset = 0x4865E0; | ||
|
||
// Get Physical Address | ||
auto addr = PlayStation2::Memory::GetPS2Address(offset); | ||
``` | ||
|
||
- Read Memory | ||
```cpp | ||
// Read Memory | ||
// - Example Offset: Tidus Health - FFX | ||
// - 0x10DF34C | ||
|
||
// Get Offset Physical Address | ||
auto addr = PlayStation2::Memory::GetPS2Address(0x10DF34C); | ||
|
||
// Read Memory | ||
auto result = PlayStation2::Memory::PS2Read<int>(addr); | ||
``` | ||
|
||
- Write Memory | ||
```cpp | ||
// Write Memory | ||
// - Example Pnach: Infinite Health by Codejunkies ( Star Wars Battlefront ) | ||
// - patch=1,EE,20519060,extended,00000001 | ||
// - Raw Address -> 20519060 | ||
// - Patch -> 00000001 | ||
|
||
// Get Physical Address | ||
auto addr = PlayStation2::Memory::GetPS2Address(0x519060); | ||
|
||
// Write Memory | ||
PlayStation2::Memory::PS2Write<int>(addr, 1); | ||
``` | ||
|
||
## ADVANCED TECHNIQUES | ||
- Access Class Members via GamePackages | ||
```cpp | ||
/// Access SealObject & Modify Weapon Ammo | ||
/// SOCOM 1 -> Access SealObject & Modify Weapon Ammo | ||
{ | ||
// Create an instance of the Offsets Class | ||
SOCOM1::Offsets offsets; // Initializing the class will auto resolve any of our offsets placed in the header | ||
SOCOM1::Offsets offsets; // Initializing the class will auto resolve all offsets placed in the class constructor | ||
auto base = (SOCOM1::CPlayer*)offsets.SEALPointer; // Get SEALObject Class | ||
if (base->isValid()) // Check if our player object is valid, generally the value will be NULL if not in a game | ||
base.PrimaryMag1 = 1337; // Set Ammo Count in MAG1 | ||
if (base->isValid()) // Check if player object is valid, generally the value will be NULL if not in a game | ||
base.PrimaryMag1 = 1337; // Set Ammo Count in MAG1 | ||
} | ||
``` | ||
| 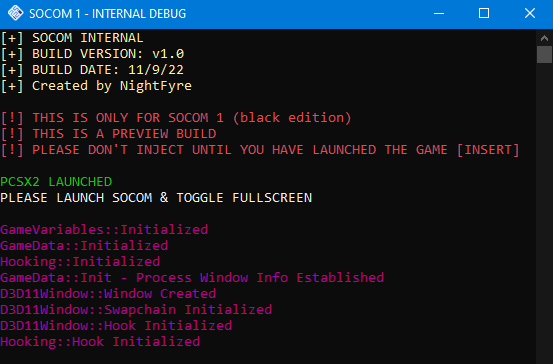 | 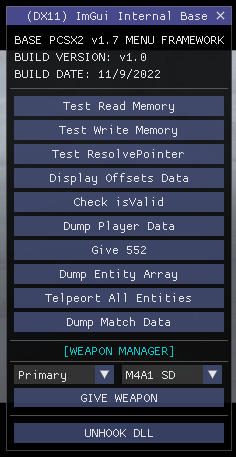 | 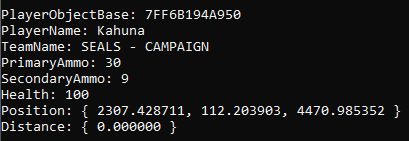 | | ||
| :---: | :---: | :---: | | ||
|
||
- Hook Rendering API | ||
```cpp | ||
/// PCSX2 -> Hook DirectX11 Present | ||
PlayStation2::GSDevice* device = *PlayStation2::CGlobals::g_gs_device; // Obtains the current render api device instance | ||
auto d3d11 = reinterpret_cast<PlayStation2::GSDevice11*>(device); // Cast D3D11 Rendering Device | ||
pSwapChain = d3d11->GetSwapChain(); // Obtain SwapChain Pointer | ||
if (pSwapChain) | ||
PlayStation2::hkVFunction(pSwapChain, 8, oIDXGISwapChainPresent, hkPresent); // Hook present | ||
``` | ||
## References & Credits | ||
- [Accessing PCSX2 Memory](https://nightfyre.github.io/PCSX2_Trainer/) | ||
- [Dear ImGui](https://github.com/ocornut/imgui) | ||
- [Minhook Hooking Library](https://github.com/TsudaKageyu/minhook) | ||
- [PCSX2](https://github.com/PCSX2/pcsx2) | ||
- [GameHacking.org](https://gamehacking.org/system/ps2) | ||
- [Sly Cooper Modding Community](https://discord.com/invite/2GSXcEzPJA) | ||
- [SOCOM Modding Community](https://discord.com/invite/PCJGrwMdUS) | ||
- [A General Guide for Making Cheats & Trainers for PCSX2](https://www.unknowncheats.me/forum/general-programming-and-reversing/569991-pcsx2-guide-cheats-trainers.html) | ||
## License | ||
This framework is distributed under the MIT License. | ||
## Disclaimer | ||
1. *This framework is intended for educational and single-player use.* | ||
2. *Use cheats responsibly and respect the terms of use of the games you are modifying.* |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
File renamed without changes.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.