-
-
Notifications
You must be signed in to change notification settings - Fork 460
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
precision of CWT #531
Comments
Nice detective work! Argument for changing the default value seems convincing to me. |
@grlee77 Thank you for the 'detective work', it was essential to understanding the implementation. I've written a thorough breakdown of the implementation in three parts: (1) general; (2) resampling vs recomputing wavelet; (3) normalization. In (3), I found that the coefficients are actually L2-normalized, even though the wavelet is initially L1-normalized, and that L1 norm seems preferable. Further, the I'll dig into |
@grlee77 Thanks for this comment. I came across this after I opened a similar issue #705 . The MATLAB algorithm you referenced above seems to involve two convolutions with the intwave function - one with int_psi(k+1) and another with int_psi(k) followed by elementwise differencing of the two arrays. The cwt implementation in pywt just does one convolution with int_psi(k) followed by a finite difference which results in finite differencing the adjacent coefficients. This feels incorrect. I believe it should be simply replaced by psi(k) i.e. the discretized wavelet function as proposed in #574 Here's an example of cwt computed using the current implemention for a synthetic signal
you can clearly see lot of artifacts especially at lower frequencies. Using the solution proposed in #574 we get the following |
I was looking at the internals of CWT to understand why it takes the integral of the wavelet here:
pywt/pywt/_cwt.py
Lines 125 to 126 in 20f67ab
while SciPy's implementation does not. This appears to be because the PyWavelets implementation is doing things the way Matlab's original
cwt
function was implemented. Specifically it is following the algorithm listed in this old version of their toolbox documentation (The algorithm does not appear to be listed in the current version of Matlab's online documentation).Here is a concrete example comparing to
scipy.signal.cwt
with themorlet2
wavelet from scipy/scipy#7076 to illustrate the issue:This gives the following results:
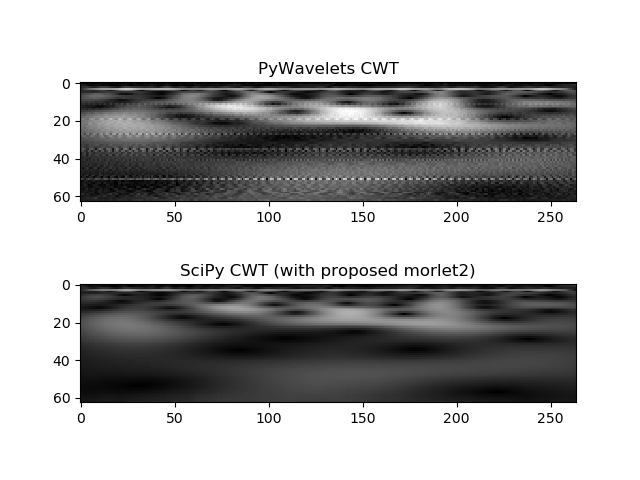
Note that there is quite a bit of zipper-like artifact in the
pywt.cwt
output. It seems that increasing theprecision
argument in the call tointwave
resolves the issue. I think the value of 10 highlighted above was chosen to match Matlab, but I think we should probably switch to a larger value. For most signals, the call to intwave is probably substantially shorter than the convolution itself, so I think it should not be problematic from a computation time standpoint to increase it a bit. (The length ofint_psi
will be2**precision
, but this will not change the eventual downsampledint_psi_scale
signal that is used during the convolutions.The reason the zipper-like artifact occurs seems to be because this
int_psi
waveform is computed once, but then integer indices are used to get versions at different scales:pywt/pywt/_cwt.py
Lines 149 to 154 in 20f67ab
The actual indices corresponding to the scales would actually be floating point, not int, so rounding them to integers gives a non-uniform step size across
int_psi
when computingint_psi_scale
. The moreint_psi
is oversampled, the less this is an issue.Increasing
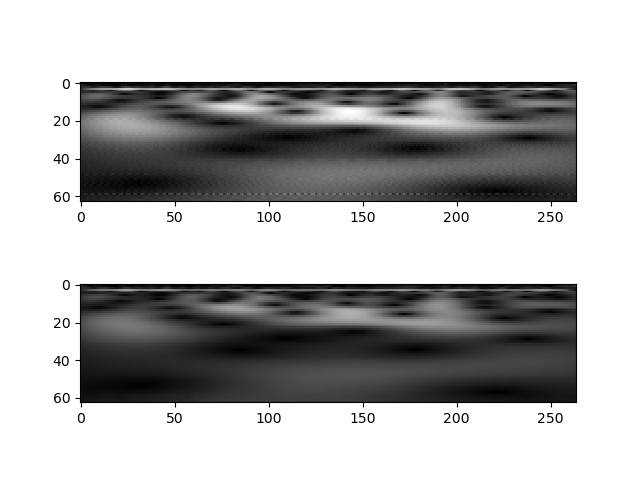
precision
from 10 to 12 reduces the artifact:and further increasing to 14 makes it no longer visible:
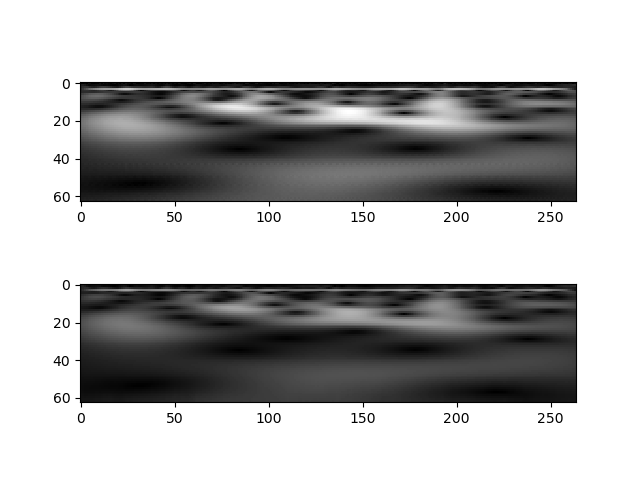
A separate issue from the artifact is the normalization convention used
In the figures above, the overall pattern looks the same, but there is some intensity difference across scales. I think this may be due to a different convention used for normalization of the wavelets. PyWavelets (and Matlab) use a normalization constant chosen to give unit L1 norm of the wavelet, while SciPy and some textbook/literature definitions use unit L2 norm. Matlab explains the rationale for their choice here. So, I wouldn't say either toolbox is "wrong", it just seems to be a matter of the convention used. We should probably make this a bit more explicit in the docs, though.
The text was updated successfully, but these errors were encountered: