-
Notifications
You must be signed in to change notification settings - Fork 157
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
feat: adding layer building infrastructure for DetectorVolume objects (…
…#2043) This PR adds the infrastructure to build layer structures to be filled into DetectorVoluems, it relies on the IndexeSurfaces infrastructure. ### In **Core**: - a new Interfaces ```IInternalStructureBuilder``` is defined, which provides the internal objects and updators of a `DetectorVolume` - `Acts::Surface` objects are provided to this, e.g through a new `KDTree` provider - The `LayerStructureBuilder` class (which extends IInternalStructueBuilder) then creates - the binned structure of the surfaces - additional support surfaces (via a new Helper `SupportBuilder`) *Note*: if configured the support surfaces can be approximated by `N` planar surfaces in order to allow intersection vectorisation. ### In **Tests**: - All of the classes are unit tested extensively ### As **Visualizations**: - An indexed Disc from the UnitTests: 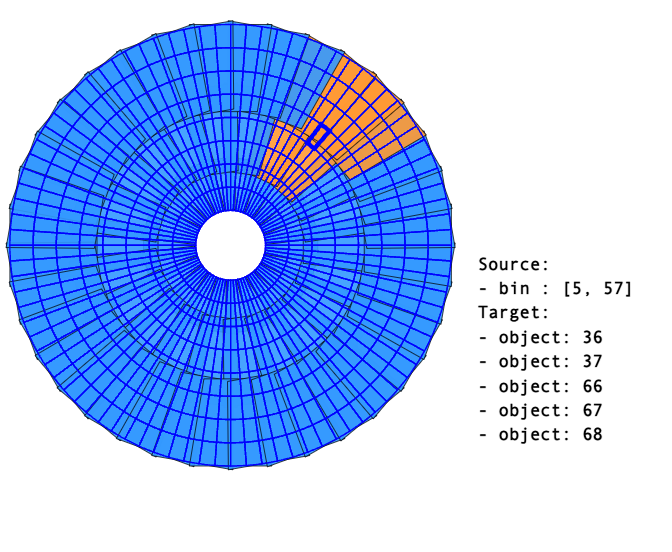 - A cylindrical support surfrace modelled as planes: 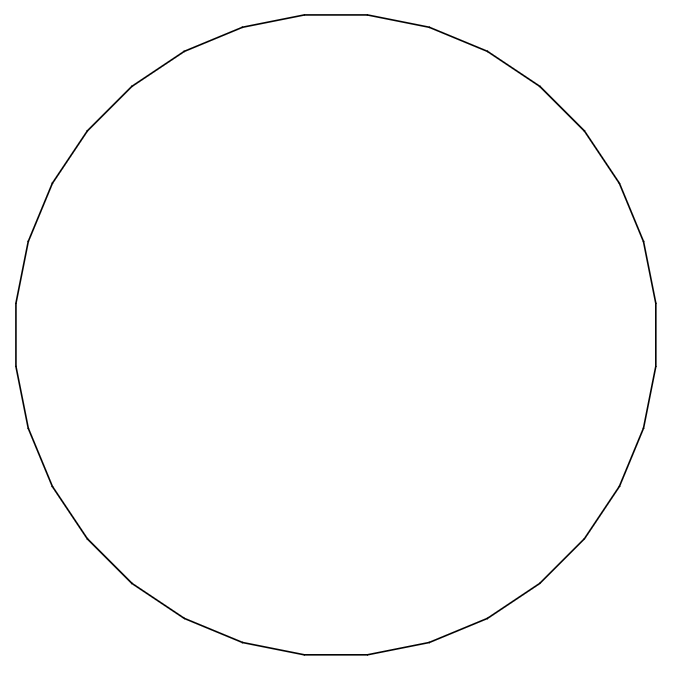 - A sectoral disk-like support surface modelled as trapezoids: 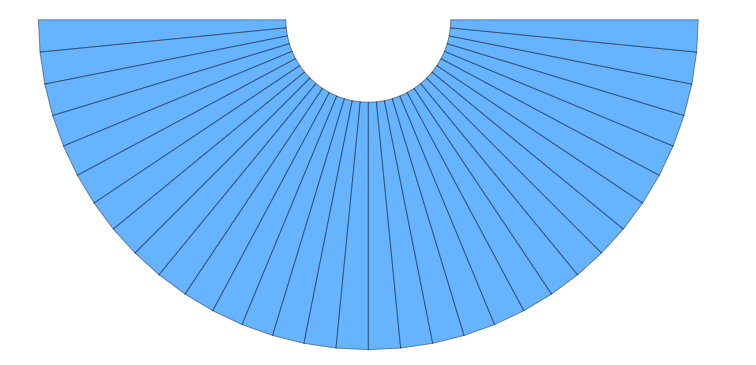 ### In **Examples**: In order to enable visual inspection of the surface binning generated, a new `SurfaceIndexing` inspector in `Examples/Detectors/DetectorInspectors` with appropriate python bindings is added, here is an example for the OpenDataDetector negative short strip endcap. ```python >>> import acts >>> from acts import examples as aex >>> ci = aex.CylindricalDetectorIndexing("odd-sensitives.json") ``` Now inspect a possible indexing: ```python ci.inspect("ss_outermost_disk_n", [[-3100., -2900. ], [200., 700.]] , [["binR", "open", "equidistant", 4, [200., 700.], 0], ["binPhi", "closed", "equidistant", 24, [ -3.142, 3.142 ], 1] ], [] ) ``` With the following results: 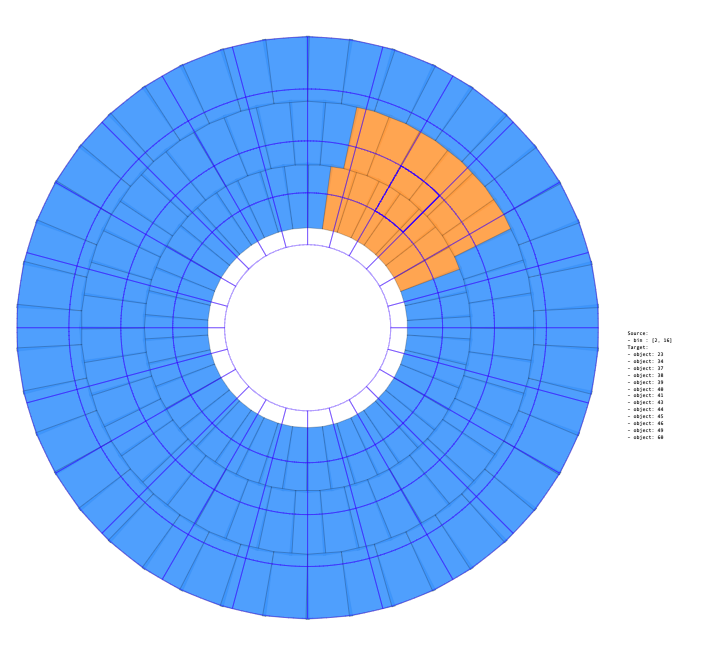 Now, the same with adapted inner radius to 240 and overcommitting the phi binning: ```python >>> ci.inspect("ss_outermost_disk_n", [[-3100., -2900. ], [200., 700.]] , [["binR", "open", "equidistant", 4, [240., 700.], 0], ["binPhi", "closed", "equidistant", 48, [ -3.142, 3.142 ], 1] ], [] ) ``` 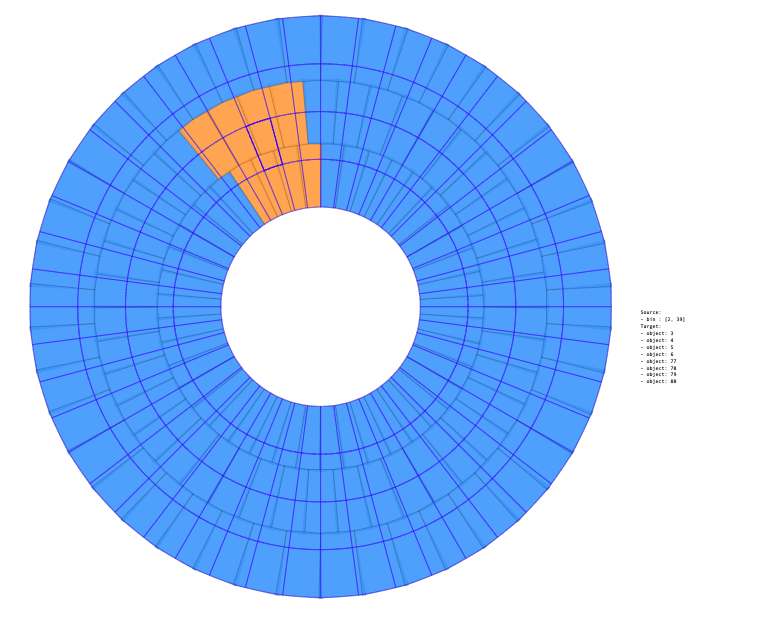
- Loading branch information
1 parent
b442bb9
commit 320be79
Showing
24 changed files
with
1,720 additions
and
7 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,61 @@ | ||
// This file is part of the Acts project. | ||
// | ||
// Copyright (C) 2023 CERN for the benefit of the Acts project | ||
// | ||
// This Source Code Form is subject to the terms of the Mozilla Public | ||
// License, v. 2.0. If a copy of the MPL was not distributed with this | ||
// file, You can obtain one at http://mozilla.org/MPL/2.0/. | ||
|
||
#pragma once | ||
|
||
#include "Acts/Geometry/GeometryContext.hpp" | ||
#include "Acts/Navigation/DetectorVolumeUpdators.hpp" | ||
#include "Acts/Navigation/SurfaceCandidatesUpdators.hpp" | ||
|
||
#include <memory> | ||
#include <tuple> | ||
#include <vector> | ||
|
||
namespace Acts { | ||
class Surface; | ||
} | ||
|
||
namespace Acts { | ||
namespace Experimental { | ||
|
||
class DetectorVolume; | ||
|
||
/// Holder struct for the internal structure components of a DetectorVolume | ||
/// | ||
/// @note the surface surfacesUpdator needs to handle also portal providing | ||
/// of contained volumes. | ||
struct InternalStructure { | ||
/// Contained surfaces of this volume, handled by the surfacesUpdator | ||
std::vector<std::shared_ptr<Surface>> surfaces = {}; | ||
/// Contained volumes of this volume, handled by the volumeUpdator | ||
std::vector<std::shared_ptr<DetectorVolume>> volumes = {}; | ||
/// Navigation delegate for surfaces | ||
SurfaceCandidatesUpdator surfacesUpdator; | ||
/// Navigaiton delegate for voluems | ||
DetectorVolumeUpdator volumeUpdator; | ||
}; | ||
|
||
/// @brief This is the interface definition of internal structure | ||
/// builders for DetectorVolume construction. | ||
/// | ||
/// It is assumed that each builder returns a consistent set of | ||
/// DetectorVolume internals, which in turn can be directly provided | ||
/// to a DetectorVolume constructor. | ||
class IInternalStructureBuilder { | ||
public: | ||
virtual ~IInternalStructureBuilder() = default; | ||
/// The interface definition for internal structure creation | ||
/// | ||
/// @param gctx the geometry context at the creation of the internal structure | ||
/// | ||
/// @return a consistent set of detector volume internals | ||
virtual InternalStructure create(const GeometryContext& gctx) const = 0; | ||
}; | ||
|
||
} // namespace Experimental | ||
} // namespace Acts |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,105 @@ | ||
// This file is part of the Acts project. | ||
// | ||
// Copyright (C) 2023 CERN for the benefit of the Acts project | ||
// | ||
// This Source Code Form is subject to the terms of the Mozilla Public | ||
// License, v. 2.0. If a copy of the MPL was not distributed with this | ||
// file, You can obtain one at http://mozilla.org/MPL/2.0/. | ||
|
||
#pragma once | ||
|
||
#include "Acts/Definitions/Algebra.hpp" | ||
#include "Acts/Detector/IInternalStructureBuilder.hpp" | ||
#include "Acts/Geometry/GeometryContext.hpp" | ||
#include "Acts/Utilities/Logger.hpp" | ||
|
||
#include <functional> | ||
#include <optional> | ||
|
||
namespace Acts { | ||
namespace Experimental { | ||
|
||
/// @brief This is a builder of layer structures to be contained | ||
/// within a DetectorVolume. | ||
/// | ||
/// It uses the IndexedSurfaceGrid to bin the internal surfaces, | ||
/// and allows for additional support surfaces that are added to the | ||
/// structure and indexing mechanism. Those support structures can | ||
/// also be approximated by planar surfaces, in order to facilitate | ||
/// vectorization of surface intersection calls. | ||
/// | ||
/// No sub volumes are added to this structure builders, hence, | ||
/// the DetectorVolumeFinder navigation delegate uses the "NoopFinder" | ||
/// breakpoint to indicate the bottom of the volume hierarchy. | ||
/// | ||
class LayerStructureBuilder : public IInternalStructureBuilder { | ||
public: | ||
/// @brief Support parameter defintions | ||
struct Support { | ||
/// Define whether you want to build support structures | ||
std::array<ActsScalar, 5u> values = {}; | ||
/// The surface type to be built | ||
Surface::SurfaceType type = Surface::SurfaceType::Other; | ||
/// Define in which values the support should be constrained | ||
std::vector<BinningValue> constraints = s_binningValues; | ||
/// Potential splits into planar approximations | ||
unsigned int splits = 1u; | ||
/// The (optional) layer transform | ||
std::optional<Transform3> transform = std::nullopt; | ||
}; | ||
|
||
/// @brief The surface binning definition | ||
struct Binning { | ||
/// Define the binning | ||
BinningData data; | ||
/// An expansion for the filling | ||
size_t expansion = 0u; | ||
}; | ||
|
||
/// @brief Configuration struct for the LayerStructureBuilder | ||
/// | ||
/// It contain: | ||
/// - a source of the surfaces to be built | ||
/// - a definition of surface binning on this layer | ||
/// - a definition of supports to be built | ||
struct Config { | ||
/// Connection point for a function to provide surfaces | ||
std::function<std::vector<std::shared_ptr<Surface>>()> surfaces; | ||
/// Definition of Supports | ||
std::vector<Support> supports = {}; | ||
/// Definition of Binnings | ||
std::vector<Binning> binnings = {}; | ||
/// Polyhedron approximations | ||
unsigned int nSegments = 1u; | ||
/// Extra information | ||
std::string auxilliary = ""; | ||
}; | ||
|
||
/// Constructor | ||
/// | ||
/// @param lConfig is the configuration struct | ||
/// @param logger logging instance for screen output | ||
LayerStructureBuilder(const Config& lConfig, | ||
std::unique_ptr<const Logger> logger = getDefaultLogger( | ||
"LayerStructureBuilder", Logging::INFO)); | ||
|
||
/// The interface definition for internal structure creation | ||
/// | ||
/// @param gctx the geometry context at the creation of the internal structure | ||
/// | ||
/// @return a consistent set of detector volume internals | ||
InternalStructure create(const GeometryContext& gctx) const final; | ||
|
||
private: | ||
/// configuration object | ||
Config m_cfg; | ||
|
||
/// Private acces method to the logger | ||
const Logger& logger() const { return *m_logger; } | ||
|
||
/// logging instance | ||
std::unique_ptr<const Logger> m_logger; | ||
}; | ||
|
||
} // namespace Experimental | ||
} // namespace Acts |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,71 @@ | ||
// This file is part of the Acts project. | ||
// | ||
// Copyright (C) 2023 CERN for the benefit of the Acts project | ||
// | ||
// This Source Code Form is subject to the terms of the Mozilla Public | ||
// License, v. 2.0. If a copy of the MPL was not distributed with this | ||
// file, You can obtain one at http://mozilla.org/MPL/2.0/. | ||
|
||
#pragma once | ||
|
||
#include "Acts/Definitions/Algebra.hpp" | ||
#include "Acts/Surfaces/Surface.hpp" | ||
#include "Acts/Utilities/Helpers.hpp" | ||
|
||
#include <array> | ||
#include <vector> | ||
|
||
namespace Acts { | ||
|
||
namespace Experimental { | ||
|
||
/// @brief This file contains helper methods to build common support structures | ||
/// such as support cylinders or discs. | ||
/// | ||
/// It allows to model those as Disc/CylinderSurface objects, but also - if | ||
/// configured such - as approximations built from palanr surfaces | ||
namespace SupportBuilder { | ||
|
||
/// @brief Helper method to build cylindrical support structure | ||
/// | ||
/// @param transform the transform where this cylinder should be located | ||
/// @param bounds the boundary values: r, halfZ, halfPhi, avgPhi, bevell parameters | ||
/// @param splits the number of surfaces through which the surface is approximated (1u ... cylinder) | ||
/// | ||
/// @return a vector of surfaces that represent this support | ||
std::vector<std::shared_ptr<Surface>> cylindricalSupport( | ||
const Transform3& transform, const std::array<ActsScalar, 6u>& bounds, | ||
unsigned int splits = 1u); | ||
|
||
/// @brief Helper method to build disc support structure | ||
/// | ||
/// @param transform the transform where this disc should be located | ||
/// @param bounds the boundary values: minR, maxR, halfPhi, avgPhi | ||
/// @param splits the number of surfaces through which the surface is approximated (1u ... disc) | ||
/// | ||
/// @return a vector of surfaces that represent this support | ||
std::vector<std::shared_ptr<Surface>> discSupport( | ||
const Transform3& transform, const std::array<ActsScalar, 4u>& bounds, | ||
unsigned int splits = 1u); | ||
|
||
/// Add support to already existing surfaces | ||
/// | ||
/// @param layerSurfaces [in, out] the surfaces to which those are added | ||
/// @param assignToAll [in, out] indices that are assigned to all bins in the indexing | ||
/// @param layerExtent the externally provided layer Extent | ||
/// @param layerRepresentation the shape of the layer | ||
/// @param layerSupportValues the support structue in numbers | ||
/// @param layerTransform is an optional value of the layer transform | ||
/// @param supportSplits the number of splits if splitting is configured | ||
/// | ||
/// @note this modifies the layerSurfaces and toAllIndices | ||
void addSupport(std::vector<std::shared_ptr<Surface>>& layerSurfaces, | ||
std::vector<size_t>& assignToAll, const Extent& layerExtent, | ||
Surface::SurfaceType layerRepresentation, | ||
const std::array<ActsScalar, 5u>& layerSupportValues, | ||
std::optional<Transform3> layerTransform = std::nullopt, | ||
unsigned int supportSplits = 1u); | ||
|
||
} // namespace SupportBuilder | ||
} // namespace Experimental | ||
} // namespace Acts |
Oops, something went wrong.