-
Notifications
You must be signed in to change notification settings - Fork 157
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
This PR fixes the Bethe energy loss function in `Interactions.cpp` `Acts::computeEnergyLossBethe` and `Acts::computeEnergyLossLandau` is implemented from equation 33.5 and 33.11 of [Review in Particle Physics](https://journals.aps.org/prd/abstract/10.1103/PhysRevD.98.030001) And their first term is computed with `computeEpsilon` function, following the notation of equation 33.11: ```c++ /// Compute epsilon energy pre-factor for RPP2018 eq. 33.11. /// /// Defined as /// /// (K/2) * (Z/A)*rho * x * (q²/beta²) /// /// where (Z/A)*rho is the electron density in the material and x is the /// traversed length (thickness) of the material. inline float computeEpsilon(float molarElectronDensity, float thickness, const RelativisticQuantities& rq) { return 0.5f * K * molarElectronDensity * thickness * rq.q2OverBeta2; } ``` Problem is that the epsilon term of equation 33.5 for Bethe function doesnt have the `0.5f` term Eq. 33.5 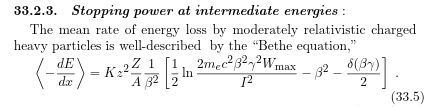 Eq. 33.11  If we are going to use the same epsilon term, we need to multiply 2 in the next term of Bethe energy loss function After the change, the output of `Acts::computeEnergyLossBethe` gets consistent with Figure 33.2 of the reference. ### Update July 18th Looks like there is a _literature_ error in Landau energy loss function (eq. 33.11). It used the mass term of the incident particle (`m`), but I guess it should be the mass of electron (`m_e`) as equation 33.5. **I need a cross-check from someone who can access [Straggling in thin silicon detectors](https://journals.aps.org/rmp/abstract/10.1103/RevModPhys.60.663), which I cannot** (Update: It is confirmed that it should be the rest mass of electron) Finally, to get a consistent result of energy loss function, we need to fix the `computeDeltaHalf` term as well. But this is already mentioned in the comment which says: "Should we use RPP2018 eq. 33.7 instead w/ tabulated constants?" ```c++ /// Compute the density correction factor delta/2. /// /// Uses RPP2018 eq. 33.6 which is only valid for high energies. /// /// @todo Should we use RPP2018 eq. 33.7 instead w/ tabulated constants? inline float computeDeltaHalf(float meanExitationPotential, float molarElectronDensity, const RelativisticQuantities& rq) { ``` Yeah it does make big difference between 33.6 (valid for very high energy particles) and 33.7. **I am not going to include delatHalf term correction in this PR**, but it is included in acts-project/detray#282 After fixing codes (including the deltaHalf term), the Bethe energy loss function matches to the value in ([PDG](https://pdg.lbl.gov/2022/AtomicNuclearProperties/index.html)) In the following figure, Bethe energy loss (dE/dx) in Silicon is compared for Acts main, this PR, acts-project/detray#282, and PDG value. The value from this PR is not accurate yet due to the incorrect deltaHalf term <img src="https://user-images.githubusercontent.com/63090140/179622322-6c7170c9-7773-4eb4-a621-63e7429e3425.png" width="700"> For the most probable energy loss from Landau equation under the same condition of Figure 33.7 of [Review in Particle Physics](https://journals.aps.org/prd/abstract/10.1103/PhysRevD.98.030001) , detray#282 got 0.526 MeV, which is very close to the value in the figure. This PR got 0.739 MeV due to the incorrect delatHalf term
- Loading branch information
1 parent
1d6aeb8
commit fcdc820
Showing
21 changed files
with
156 additions
and
87 deletions.
There are no files selected for viewing
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file modified
BIN
-128 Bytes
(100%)
CI/physmon/reference/performance_ckf_truth_estimated.root
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file not shown.
Binary file modified
BIN
-25 Bytes
(100%)
CI/physmon/reference/performance_seeding_truth_estimated.root
Binary file not shown.
Binary file not shown.
Binary file modified
BIN
+40 Bytes
(100%)
CI/physmon/reference/performance_vertexing_orthogonal_hist.root
Binary file not shown.
Binary file modified
BIN
-16 Bytes
(100%)
CI/physmon/reference/performance_vertexing_seeded_hist.root
Binary file not shown.
Binary file modified
BIN
-17 Bytes
(100%)
CI/physmon/reference/performance_vertexing_truth_estimated_hist.root
Binary file not shown.
Binary file modified
BIN
+19 Bytes
(100%)
CI/physmon/reference/performance_vertexing_truth_smeared_hist.root
Binary file not shown.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.