New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
How to seed the database by sequence? #838
Comments
I'm guessing this happens because createMany does the inserts asynchrously. Does the order they're inserted really matter to you as long as all the emails are there and they're unique? |
Hi @webdevian, The order is somehow matters when you want do some dirty hard code staff instead of keeping some logic inside configs or tables. |
Yes |
I don't want create new issue. Forgive me. Why does framework don't support batch like migration? |
Seeds are used to have initial data in your database, that you can use for manual testing. While development, if you your data requirements change, then you must remove everything from the database and then add it. So simply within the same seed file, truncate all tables and then add data to them. |
Run queries in parallel make things unpredictable, I don't think this worth the trade of performance while development, you don't always have tons of data to seed. |
I also would like to suggest make the seeding process more transparent, it's good for people to control which schema should been seeded first, just like the migrations. |
@thetutlage |
I believe it is a general programming question.
The both statements are not parallel in any form. Also there is an RFC for seeds adonisjs/lucid#307. Please leave your suggestions there |
When you are using MySQL and you have table with foreign constraints, truncate does not work. Instead run:
I thought maybe someone will find this useful, because I was solving the same problem now and google search got me here. |
I solved it by creating a new Ace command. There might be better ways of solving this but this is how I did it: Create a new Ace-command: Add the following code to the command:
Now, instead of running As previously mentioned this type of solution will take longer time to execute because of the seeds running asynchronously. For reference, a seed that usually takes ~3s now takes ~7s. Hopefully this might help others as well. |
hello there, first of all thank you for sharing this. But would you mind explaining this line to me please: |
That creates a promise object directly. |
hey sorry for bothering you again, it doesnt seem to work for me. the |
Update: |
@amit2maha1 Run it with promisify and it should work. I'm currently running a slightly updated version of that command. I'll post it here.
|
dude it still says "Nothing to seed" |
this is the stupidest thing ever, it only works when I use this as the command |
it works but it stops after the first seed, any help will be appreciated my friend |
@amit2maha1 One solution is to add all you seed-files in E.g.
It's in that sequence the files will be executed. |
This thread has been automatically locked since there has not been any recent activity after it was closed. Please open a new issue for related bugs. |
Let's say we have a
UserSeeder.js
like this:and
factory.js
looks like this:My expected result is:
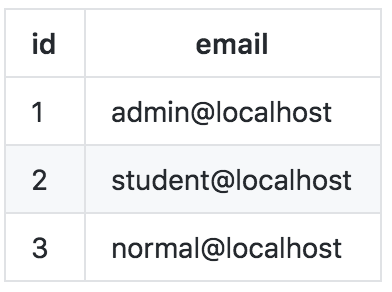
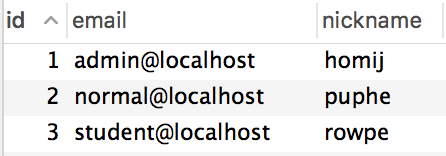
but the actual result is:
So am wondering if there's any way to approach this or have to seed them one by one?
Thanks!
The text was updated successfully, but these errors were encountered: