Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
yiyi.qi
committed
Oct 21, 2016
0 parents
commit 67c2e65
Showing
37 changed files
with
1,389 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,9 @@ | ||
*.iml | ||
.gradle | ||
/local.properties | ||
/.idea/workspace.xml | ||
/.idea/libraries | ||
.DS_Store | ||
/build | ||
/captures | ||
.externalNativeBuild |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,124 @@ | ||
本工程为基于高德地图Android SDK进行封装,实现了Marker聚合效果的例子 | ||
## 前述 ## | ||
- [高德官网申请key](http://lbs.amap.com/dev/#/). | ||
- 阅读[参考手册](http://a.amap.com/lbs/static/unzip/Android_Map_Doc/index.html). | ||
- 工程基于Android 3D地图SDK实现 | ||
|
||
## 功能描述 ## | ||
基于3D地图SDK进行封装,在大量点现实的场景下可以对点进行聚合显示,提高地图响应显示效率。在之前的开源工程版本基础上做了一些修改,提高了显示速度和内存优化使用,支持的聚合点更多,效果更好。主要修改包括: | ||
-将marker处理线程和聚合算法线程进行分离,提高效率。 | ||
-聚合过程中减少不必要的运算,提高聚合显示速度。 | ||
-适配新版SDK应用场景,对用到的图标,统一管理,减少内存使用和提高效率, | ||
-加入了动画效果,聚合切换过程更为平滑 | ||
|
||
## 效果图如下 ## | ||
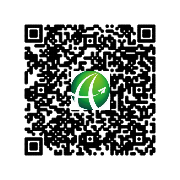 | ||
|
||
## 扫一扫安装## | ||
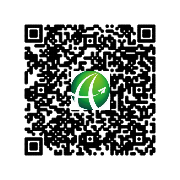 | ||
|
||
|
||
## 使用方法## | ||
###1:配置搭建AndroidSDK工程### | ||
-[Android Studio工程搭建方法](http://lbs.amap.com/api/android-sdk/guide/creat-project/android-studio-creat-project/#add-jars). | ||
-[通过maven库引入SDK方法](http://lbsbbs.amap.com/forum.php?mod=viewthread&tid=18786). | ||
###2:接口使用### | ||
-初始化聚合和加入元素 | ||
1:批量初始化,适合一次大量加入 | ||
``` java | ||
|
||
List<ClusterItem> items = new ArrayList<ClusterItem>(); | ||
|
||
//随机10000个点 | ||
for (int i = 0; i < 10000; i++) { | ||
|
||
double lat = Math.random() + 39.474923; | ||
double lon = Math.random() + 116.027116; | ||
|
||
LatLng latLng = new LatLng(lat, lon, false); | ||
RegionItem regionItem = new RegionItem(latLng, | ||
"test" + i); | ||
items.add(regionItem); | ||
|
||
} | ||
mClusterOverlay = new ClusterOverlay(mAMap, items, | ||
dp2px(getApplicationContext(), clusterRadius), | ||
getApplicationContext()); | ||
``` | ||
2:初始化之后,有新的聚合点加入 | ||
``` java | ||
|
||
double lat = Math.random() + 39.474923; | ||
double lon = Math.random() + 116.027116; | ||
|
||
LatLng latLng1 = new LatLng(lat, lon, false); | ||
RegionItem regionItem = new RegionItem(latLng1, | ||
"test"); | ||
mClusterOverlay.addClusterItem(regionItem); | ||
``` | ||
-设置渲染render和聚合点点击事件监听 | ||
``` java | ||
mClusterOverlay.setClusterRenderer(MainActivity.this); | ||
mClusterOverlay.setOnClusterClickListener(MainActivity.this); | ||
``` | ||
-自定义渲染 | ||
``` java | ||
public Drawable getDrawAble(int clusterNum) { | ||
int radius = dp2px(getApplicationContext(), 80); | ||
if (clusterNum == 1) { | ||
Drawable bitmapDrawable = mBackDrawAbles.get(1); | ||
if (bitmapDrawable == null) { | ||
bitmapDrawable = | ||
getApplication().getResources().getDrawable( | ||
R.drawable.icon_openmap_mark); | ||
mBackDrawAbles.put(1, bitmapDrawable); | ||
} | ||
|
||
return bitmapDrawable; | ||
} else if (clusterNum < 5) { | ||
|
||
Drawable bitmapDrawable = mBackDrawAbles.get(2); | ||
if (bitmapDrawable == null) { | ||
bitmapDrawable = new BitmapDrawable(null, drawCircle(radius, | ||
Color.argb(159, 210, 154, 6))); | ||
mBackDrawAbles.put(2, bitmapDrawable); | ||
} | ||
|
||
return bitmapDrawable; | ||
} else if (clusterNum < 10) { | ||
Drawable bitmapDrawable = mBackDrawAbles.get(3); | ||
if (bitmapDrawable == null) { | ||
bitmapDrawable = new BitmapDrawable(null, drawCircle(radius, | ||
Color.argb(199, 217, 114, 0))); | ||
mBackDrawAbles.put(3, bitmapDrawable); | ||
} | ||
|
||
return bitmapDrawable; | ||
} else { | ||
Drawable bitmapDrawable = mBackDrawAbles.get(4); | ||
if (bitmapDrawable == null) { | ||
bitmapDrawable = new BitmapDrawable(null, drawCircle(radius, | ||
Color.argb(235, 215, 66, 2))); | ||
mBackDrawAbles.put(4, bitmapDrawable); | ||
} | ||
|
||
return bitmapDrawable; | ||
} | ||
} | ||
``` | ||
-聚合点击事件 | ||
``` java | ||
public void onClick(Marker marker, List<ClusterItem> clusterItems) { | ||
mClusterItems = clusterItems; | ||
mCenterLat = marker.getPosition(); | ||
LatLngBounds.Builder builder = new LatLngBounds.Builder(); | ||
for (ClusterItem clusterItem : clusterItems) { | ||
builder.include(clusterItem.getPosition()); | ||
|
||
|
||
} | ||
LatLngBounds latLngBounds = builder.build(); | ||
mAMap.animateCamera(CameraUpdateFactory.newLatLngBounds(latLngBounds, 0) | ||
); | ||
} | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1 @@ | ||
/build |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,35 @@ | ||
apply plugin: 'com.android.application' | ||
|
||
android { | ||
compileSdkVersion 23 | ||
buildToolsVersion "23.0.3" | ||
defaultConfig { | ||
applicationId "com.amap.apis.cluster" | ||
minSdkVersion 15 | ||
targetSdkVersion 23 | ||
versionCode 1 | ||
versionName "1.0" | ||
testInstrumentationRunner "android.support.test.runner.AndroidJUnitRunner" | ||
ndk { | ||
abiFilters 'armeabi' | ||
} | ||
} | ||
buildTypes { | ||
release { | ||
minifyEnabled false | ||
|
||
} | ||
} | ||
} | ||
|
||
dependencies { | ||
compile fileTree(include: ['*.jar'], dir: 'libs') | ||
androidTestCompile('com.android.support.test.espresso:espresso-core:2.2.2', { | ||
exclude group: 'com.android.support', module: 'support-annotations' | ||
}) | ||
compile 'com.android.support:appcompat-v7:23.4.0' | ||
compile 'com.amap.api:map3d:latest.integration' | ||
compile 'com.amap.api:map3d-native:latest.integration' | ||
testCompile 'junit:junit:4.12' | ||
compile 'com.google.android.gms:play-services-appindexing:8.4.0' | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,17 @@ | ||
# Add project specific ProGuard rules here. | ||
# By default, the flags in this file are appended to flags specified | ||
# in /Users/yiyi.qi/Downloads/DeveloperSoft/android-sdk-macosx/tools/proguard/proguard-android.txt | ||
# You can edit the include path and order by changing the proguardFiles | ||
# directive in build.gradle. | ||
# | ||
# For more details, see | ||
# http://developer.android.com/guide/developing/tools/proguard.html | ||
|
||
# Add any project specific keep options here: | ||
|
||
# If your project uses WebView with JS, uncomment the following | ||
# and specify the fully qualified class name to the JavaScript interface | ||
# class: | ||
#-keepclassmembers class fqcn.of.javascript.interface.for.webview { | ||
# public *; | ||
#} |
26 changes: 26 additions & 0 deletions
26
app/src/androidTest/java/com/amap/apis/cluster/ExampleInstrumentedTest.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,26 @@ | ||
package com.amap.apis.cluster; | ||
|
||
import android.content.Context; | ||
import android.support.test.InstrumentationRegistry; | ||
import android.support.test.runner.AndroidJUnit4; | ||
|
||
import org.junit.Test; | ||
import org.junit.runner.RunWith; | ||
|
||
import static org.junit.Assert.*; | ||
|
||
/** | ||
* Instrumentation test, which will execute on an Android device. | ||
* | ||
* @see <a href="http://d.android.com/tools/testing">Testing documentation</a> | ||
*/ | ||
@RunWith(AndroidJUnit4.class) | ||
public class ExampleInstrumentedTest { | ||
@Test | ||
public void useAppContext() throws Exception { | ||
// Context of the app under test. | ||
Context appContext = InstrumentationRegistry.getTargetContext(); | ||
|
||
assertEquals("com.amap.apis.cluster", appContext.getPackageName()); | ||
} | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,30 @@ | ||
<?xml version="1.0" encoding="utf-8"?> | ||
<manifest xmlns:android="http://schemas.android.com/apk/res/android" | ||
package="com.amap.apis.cluster"> | ||
|
||
<application | ||
android:allowBackup="true" | ||
android:icon="@mipmap/ic_launcher" | ||
android:label="@string/app_name" | ||
android:supportsRtl="true" | ||
> | ||
<activity android:name=".MainActivity" android:screenOrientation="portrait"> | ||
<intent-filter> | ||
<action android:name="android.intent.action.MAIN" /> | ||
|
||
<category android:name="android.intent.category.LAUNCHER" /> | ||
</intent-filter> | ||
</activity> | ||
<meta-data android:name="com.amap.api.v2.apikey" android:value="您的key"> | ||
</meta-data> | ||
</application> | ||
<uses-permission android:name="android.permission.INTERNET" /> | ||
<uses-permission android:name="android.permission.WRITE_EXTERNAL_STORAGE" /> | ||
<uses-permission android:name="android.permission.ACCESS_COARSE_LOCATION" /> | ||
<uses-permission android:name="android.permission.ACCESS_NETWORK_STATE" /> | ||
<uses-permission android:name="android.permission.ACCESS_FINE_LOCATION" /> | ||
<uses-permission android:name="android.permission.READ_PHONE_STATE" /> | ||
<uses-permission android:name="android.permission.CHANGE_WIFI_STATE" /> | ||
<uses-permission android:name="android.permission.ACCESS_WIFI_STATE" /> | ||
<uses-permission android:name="android.permission.CHANGE_CONFIGURATION" /> | ||
</manifest> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,55 @@ | ||
package com.amap.apis.cluster; | ||
|
||
import android.graphics.Point; | ||
|
||
import com.amap.api.maps.AMapUtils; | ||
import com.amap.api.maps.model.LatLng; | ||
import com.amap.api.maps.model.Marker; | ||
|
||
import java.util.ArrayList; | ||
import java.util.List; | ||
|
||
/** | ||
* Created by yiyi.qi on 16/10/10. | ||
*/ | ||
|
||
public class Cluster { | ||
|
||
|
||
private LatLng mLatLng; | ||
private List<ClusterItem> mClusterItems; | ||
private Marker mMarker; | ||
|
||
|
||
Cluster( LatLng latLng) { | ||
|
||
mLatLng = latLng; | ||
mClusterItems = new ArrayList<ClusterItem>(); | ||
} | ||
|
||
void addClusterItem(ClusterItem clusterItem) { | ||
mClusterItems.add(clusterItem); | ||
} | ||
|
||
int getClusterCount() { | ||
return mClusterItems.size(); | ||
} | ||
|
||
|
||
|
||
LatLng getCenterLatLng() { | ||
return mLatLng; | ||
} | ||
|
||
void setMarker(Marker marker) { | ||
mMarker = marker; | ||
} | ||
|
||
Marker getMarker() { | ||
return mMarker; | ||
} | ||
|
||
List<ClusterItem> getClusterItems() { | ||
return mClusterItems; | ||
} | ||
} |
21 changes: 21 additions & 0 deletions
21
app/src/main/java/com/amap/apis/cluster/ClusterClickListener.java
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,21 @@ | ||
package com.amap.apis.cluster; | ||
|
||
import com.amap.api.maps.model.Marker; | ||
|
||
import java.util.List; | ||
|
||
/** | ||
* Created by yiyi.qi on 16/10/10. | ||
*/ | ||
|
||
public interface ClusterClickListener{ | ||
/** | ||
* 点击聚合点的回调处理函数 | ||
* | ||
* @param marker | ||
* 点击的聚合点 | ||
* @param clusterItems | ||
* 聚合点所包含的元素 | ||
*/ | ||
public void onClick(Marker marker, List<ClusterItem> clusterItems); | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,16 @@ | ||
package com.amap.apis.cluster; | ||
|
||
import com.amap.api.maps.model.LatLng; | ||
|
||
/** | ||
* Created by yiyi.qi on 16/10/10. | ||
*/ | ||
|
||
public interface ClusterItem { | ||
/** | ||
* 返回聚合元素的地理位置 | ||
* | ||
* @return | ||
*/ | ||
LatLng getPosition(); | ||
} |
Oops, something went wrong.