-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
ce3cf98
commit 5f5f3d3
Showing
12 changed files
with
200 additions
and
108 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
File renamed without changes
File renamed without changes
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,46 @@ | ||
# Installation | ||
|
||
## Prerequisites | ||
|
||
While it is possible to use this library with the service run by W3C at [validator.w3.org/nu](https://validator.w3.org/nu/), | ||
I would recommend running your own instance. You will eliminate a lot of network latency if it runs on the same machine as your code, and you will not hit any rate limits that might exist for [validator.w3.org/nu](https://validator.w3.org/nu/). | ||
|
||
The source of the Checker can be found in the repository [validator/validator](https://github.com/validator/validator). | ||
Follow their instructions on how to download it and [run it as a web server](https://github.com/validator/validator#standalone-web-server). | ||
|
||
The easiest option is to use the Docker image, like this: | ||
```bash | ||
docker run -it --rm -p 8888:8888 ghcr.io/validator/validator:latest | ||
``` | ||
|
||
The command might require an additional `--platform linux/amd64` flag on M1 macs. | ||
|
||
Check if the server is running: | ||
```bash | ||
$ curl localhost:8888 -I | ||
HTTP/1.1 200 OK | ||
``` | ||
|
||
## Installation | ||
|
||
Make sure to read about the [prerequisites](#prerequisites) first. | ||
|
||
Add Vnu as a dependency to your project's `mix.exs`. To use the built-in, Hackney-based HTTP client adapter, also add `:hackney`: | ||
|
||
```elixir | ||
defp deps do | ||
[ | ||
{:vnu, "~> 1.1", only: [:dev, :test], runtime: false}, | ||
{:hackney, "~> 1.17"} | ||
] | ||
end | ||
``` | ||
|
||
TODO: describe what to do when you don't want to use hackney. | ||
|
||
And run: | ||
|
||
```bash | ||
$ mix deps.get | ||
``` | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,2 +1,17 @@ | ||
# Overview | ||
|
||
[](https://circleci.com/gh/angelikatyborska/vnu-elixir) | ||
 | ||
 | ||
 | ||
[](https://coveralls.io/github/angelikatyborska/vnu-elixir?branch=master) | ||
|
||
An Elixir client for [the Nu HTML Checker (v.Nu)](https://validator.w3.org/nu/). | ||
|
||
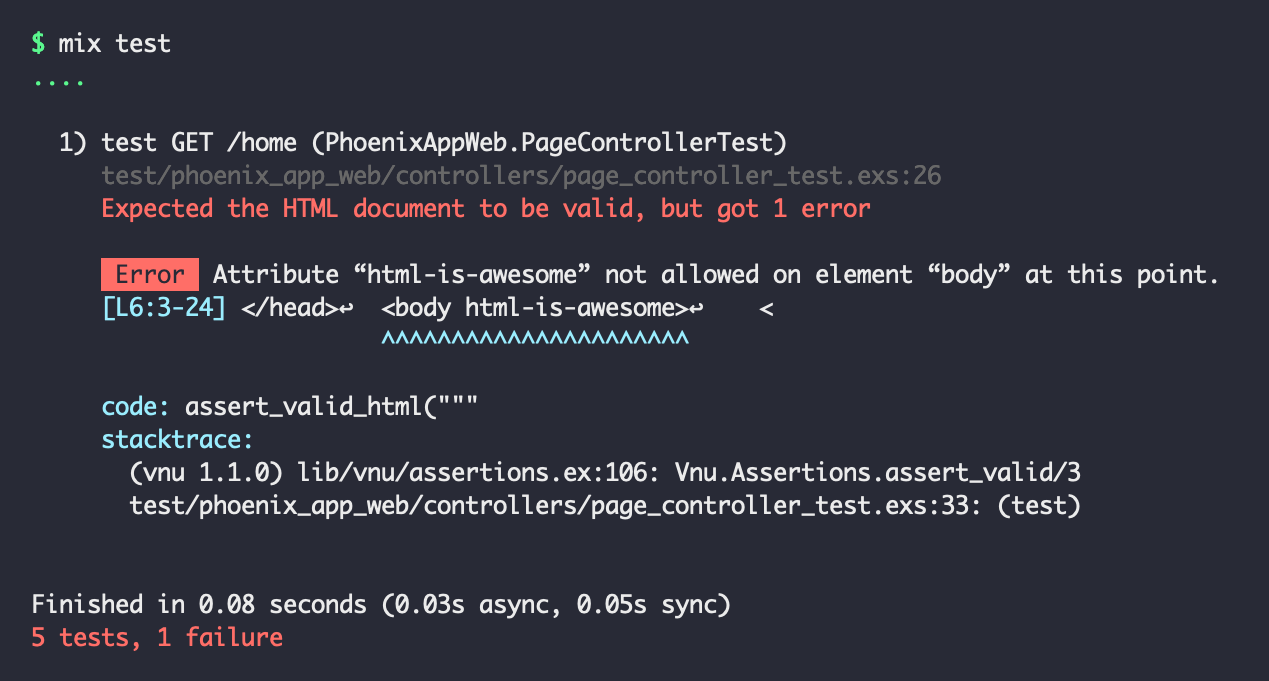 | ||
|
||
[v.Nu](https://validator.w3.org/nu/) is a document validity checker used by the W3C. | ||
It offers validating HTML, CSS, and SVG documents. | ||
|
||
This library brings that functionality to Elixir by using the Checker's JSON API. | ||
It offers ExUnit assertions for validating dynamic content in tests, Mix tasks for validating static content, and general purpose functions to fulfill other needs. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1,11 +1,136 @@ | ||
# Usage | ||
|
||
There are 3 different ways of using this library: | ||
- ExUnit assertions, | ||
- mix tasks for your static assets, | ||
- or generic function calls for all other needs. | ||
|
||
## ExUnit assertions | ||
|
||
### Phoenix controller tests | ||
If you are building an application that generates HTML, CSS, or SVG files, you might want to use those validations in your tests. | ||
|
||
- `Vnu.Assertions.assert_valid_html/2` | ||
- `Vnu.Assertions.assert_valid_css/2` | ||
- `Vnu.Assertions.assert_valid_svg/2` | ||
|
||
### Phoenix example | ||
|
||
I would recommend defining a local `assert_valid_html` function in your [`ConnCase`](https://hexdocs.pm/phoenix/testing.html#the-conncase) to have a single place to pass your default options. | ||
|
||
```elixir | ||
defmodule PhoenixAppWeb.ConnCase do | ||
use ExUnit.CaseTemplate | ||
|
||
using do | ||
quote do | ||
# ... | ||
def assert_valid_html(html, vnu_opts \\ []) do | ||
default_vnu_opts = [ | ||
server_url: "http://localhost:8888/", | ||
filter: PhoenixAppWeb.VnuHTMLMessageFilter, | ||
fail_on_warnings: true | ||
] | ||
|
||
Vnu.Assertions.assert_valid_html(html, Keyword.merge(default_vnu_opts, vnu_opts)) | ||
end | ||
end | ||
end | ||
end | ||
``` | ||
|
||
Then, you can use this function in your controller tests, LiveView tests, and integration tests. | ||
|
||
#### Controller tests | ||
|
||
```elixir | ||
defmodule PhoenixAppWeb.PageControllerTest do | ||
use PhoenixAppWeb.ConnCase | ||
|
||
test "GET /", %{conn: conn} do | ||
conn = get(conn, "/") | ||
|
||
html_response = | ||
conn | ||
|> html_response(200) | ||
|> assert_valid_html(fail_on_warnings: false) | ||
|
||
assert html_response =~ "Peace of mind from prototype to production" | ||
end | ||
end | ||
``` | ||
|
||
 | ||
|
||
### Phoenix LiveView tests | ||
|
||
#### LiveView tests | ||
|
||
TODO: add to example phoenix app and describe | ||
|
||
#### Hound integration tests | ||
|
||
TODO: add to example phoenix app and describe | ||
|
||
## Mix tasks | ||
|
||
If you have static HTML, CSS, or SVG files in your project, you might want to validate them with those mix tasks: | ||
|
||
- `mix vnu.validate.html` | ||
- `mix vnu.validate.css` | ||
- `mix vnu.validate.svg` | ||
- | ||
### Example | ||
|
||
```bash | ||
$ mix vnu.validate.css --server-url http://localhost:8888 assets/**/*.css | ||
``` | ||
|
||
 | ||
|
||
## Other needs | ||
|
||
If you need HTML, CSS, or SVG validation for something else, try one of those functions: | ||
|
||
- `Vnu.validate_html/2` | ||
- `Vnu.validate_css/2` | ||
- `Vnu.validate_svg/2` | ||
|
||
### Example | ||
|
||
```elixir | ||
iex> {:ok, result} = Vnu.validate_html("<!DOCTYPE html><html><head></head></html>", | ||
server_url: "http://localhost:8888") | ||
{:ok, | ||
%Vnu.Result{ | ||
messages: [ | ||
%Vnu.Message{ | ||
extract: "tml><head></head></html", | ||
first_column: 28, | ||
first_line: 1, | ||
hilite_length: 7, | ||
hilite_start: 10, | ||
last_column: 34, | ||
last_line: 1, | ||
message: "Element “head” is missing a required instance of child element “title”.", | ||
offset: nil, | ||
sub_type: nil, | ||
type: :error | ||
}, | ||
%Vnu.Message{ | ||
extract: "TYPE html><html><head>", | ||
first_column: 16, | ||
first_line: 1, | ||
hilite_length: 6, | ||
hilite_start: 10, | ||
last_column: 21, | ||
last_line: 1, | ||
message: "Consider adding a “lang” attribute to the “html” start tag to declare the language of this document.", | ||
offset: nil, | ||
sub_type: :warning, | ||
type: :info | ||
} | ||
] | ||
}} | ||
|
||
iex> Vnu.valid?(result) | ||
false | ||
``` |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters